c语言中动态分配内存
The process of allocating memory at runtime is known as dynamic memory allocation. Library routines known as memory management functions are used for allocating and freeing memory during execution of a program. These functions are defined in stdlib.h header file.
在运行时分配内存的过程称为动态内存分配 。 被称为内存管理功能的库例程用于在程序执行期间分配和释放内存。 这些函数在stdlib.h头文件中定义。
Function | Description |
---|---|
malloc() | allocates requested size of bytes and returns a void pointer pointing to the first byte of the allocated space |
calloc() | allocates space for an array of elements, initialize them to zero and then returns a void pointer to the memory |
free | releases previously allocated memory |
realloc | modify the size of previously allocated space |
功能 | 描述 |
---|---|
malloc() | 分配请求的字节大小,并返回一个空指针,该指针指向分配空间的第一个字节 |
calloc() | 为元素数组分配空间,将其初始化为零,然后返回指向内存的空指针 |
free | 释放先前分配的内存 |
realloc | 修改先前分配的空间的大小 |
内存分配过程 (Memory Allocation Process)
Global variables, static
variables and program instructions get their memory in permanent storage area whereas local variables are stored in a memory area called Stack.
全局变量, static
变量和程序指令在永久存储区中获取其内存,而局部变量存储在称为Stack的存储区中。
The memory space between these two region is known as Heap area. This region is used for dynamic memory allocation during execution of the program. The size of heap keep changing.
这两个区域之间的存储空间称为堆区域。 该区域用于在程序执行期间进行动态内存分配。 堆的大小不断变化。
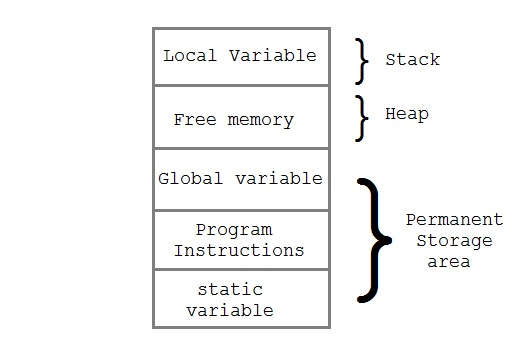
分配内存块 (Allocating block of Memory)
malloc()
function is used for allocating block of memory at runtime. This function reserves a block of memory of the given size and returns a pointer of type void
. This means that we can assign it to any type of pointer using typecasting. If it fails to allocate enough space as specified, it returns a NULL
pointer.
malloc()
函数用于在运行时分配内存块。 此函数保留给定大小的内存块,并返回void
类型的指针 。 这意味着我们可以使用类型转换将其分配给任何类型的指针。 如果未能按指定分配足够的空间,则返回NULL
指针。
Syntax:
句法:
void* malloc(byte-size)
示例时间:malloc() (Time for an Example: malloc())
int *x;
x = (int*)malloc(50 * sizeof(int)); //memory space allocated to variable x
free(x); //releases the memory allocated to variable x
calloc()
is another memory allocation function that is used for allocating memory at runtime. calloc
function is normally used for allocating memory to derived data types such as arrays and structures. If it fails to allocate enough space as specified, it returns a NULL
pointer.
calloc()
是另一个内存分配函数,用于在运行时分配内存。 calloc
函数通常用于将内存分配给派生的数据类型,例如数组和结构 。 如果未能按指定分配足够的空间,则返回NULL
指针。
Syntax:
句法:
void *calloc(number of items, element-size)
示例时间:calloc() (Time for an Example: calloc())
struct employee
{
char *name;
int salary;
};
typedef struct employee emp;
emp *e1;
e1 = (emp*)calloc(30,sizeof(emp));
realloc()
changes memory size that is already allocated dynamically to a variable.
realloc()
更改已动态分配给变量的内存大小。
Syntax:
句法:
void* realloc(pointer, new-size)
示例时间:realloc() (Time for an Example: realloc())
int *x;
x = (int*)malloc(50 * sizeof(int));
x = (int*)realloc(x,100); //allocated a new memory to variable x
malloc()
和calloc()
之间的差异 (Diffrence between malloc()
and calloc()
)
calloc() | malloc() |
---|---|
calloc() initializes the allocated memory with 0 value. | malloc() initializes the allocated memory with garbage values. |
Number of arguments is 2 | Number of argument is 1 |
Syntax : (cast_type *)calloc(blocks , size_of_block); | Syntax : (cast_type *)malloc(Size_in_bytes); |
calloc() | malloc() |
---|---|
calloc()使用0值初始化分配的内存。 | malloc()使用垃圾值初始化分配的内存。 |
参数数量为2 | 自变量数为1 |
句法 : (cast_type *)calloc(blocks,size_of_block); | 句法 : (cast_type *)malloc(Size_in_bytes); |
表示动态内存分配的程序(使用calloc()) (Program to represent Dynamic Memory Allocation(using calloc()))
#include <stdio.h>
#include <stdlib.h>
int main()
{
int i, n;
int *element;
printf("Enter total number of elements: ");
scanf("%d", &n);
/*
returns a void pointer(which is type-casted to int*)
pointing to the first block of the allocated space
*/
element = (int*) calloc(n,sizeof(int));
/*
If it fails to allocate enough space as specified,
it returns a NULL pointer.
*/
if(element == NULL)
{
printf("Error.Not enough space available");
exit(0);
}
for(i = 0; i < n; i++)
{
/*
storing elements from the user
in the allocated space
*/
scanf("%d", element+i);
}
for(i = 1; i < n; i++)
{
if(*element > *(element+i))
{
*element = *(element+i);
}
}
printf("Smallest element is %d", *element);
return 0;
}
Enter total number of elements: 5 4 2 1 5 3 Smallest element is 1
输入元素总数:5 4 2 1 5 3最小元素是1
翻译自: https://www.studytonight.com/c/dynamic-memory-allocation-in-c.php
c语言中动态分配内存