双链表排序
Doubly linked list is a type of linked list in which each node apart from storing its data has two links. The first link points to the previous node in the list and the second link points to the next node in the list. The first node of the list has its previous link pointing to NULL similarly the last node of the list has its next node pointing to NULL.
双链表是一种链表,其中每个节点(除存储其数据外)都有两个链接。 第一个链接指向列表中的上一个节点,第二个链接指向列表中的下一个节点。 列表的第一个节点的上一个链接指向NULL,类似地,列表的最后一个节点的下一个节点指向NULL。
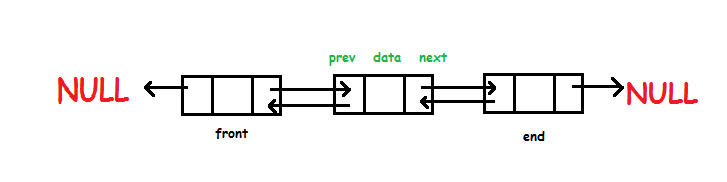
The two links help us to traverse the list in both backward and forward direction. But storing an extra link requires some extra space.
这两个链接可帮助我们在向前和向后的方向上遍历列表。 但是存储额外的链接需要一些额外的空间。
双链表的实现 (Implementation of Doubly Linked List)
First we define the node.
首先,我们定义节点。
struct node
{
int data; // Data
node *prev; // A reference to the previous node
node *next; // A reference to the next node
};
Now we define our class Doubly Linked List. It has the following methods:
现在,我们定义类“双重链接列表”。 它具有以下方法:
add_front: Adds a new node in the beginning of list
add_front:在列表的开头添加一个新节点
add_after: Adds a new node after another node
add_after:在另一个节点之后添加一个新节点
add_before: Adds a new node before another node
add_before:在另一个节点之前添加一个新节点
add_end: Adds a new node in the end of list
add_end:在列表末尾添加一个新节点
delete: Removes the node
delete:删除节点
forward_traverse: Traverse the list in forward direction
forward_traverse:向前遍历列表
backward_traverse: Traverse the list in backward direction
向后遍历:向后遍历列表
class Doubly_Linked_List
{
node *front; // points to first node of list
node *end; // points to first las of list
public:
Doubly_Linked_List()
{
front = NULL;
end = NULL;
}
void add_front(int );
void add_after(node* , int );
void add_before(node* , int );
void add_end(int );
void delete_node(node*);
void forward_traverse();
void backward_traverse();
};
在开头插入数据 (Insert Data in the beginning)
The prev pointer of first node will always be NULL and next will point to front.
第一节点的分组指针将始终是NULL和下一个将指向前方 。
If the node is inserted is the first node of the list then we make front and end point to this node.
如果插入的节点是列表的第一个节点,则我们将前端和终点指向该节点。
Else we only make front point to this node.
否则,我们仅将前端指向该节点。
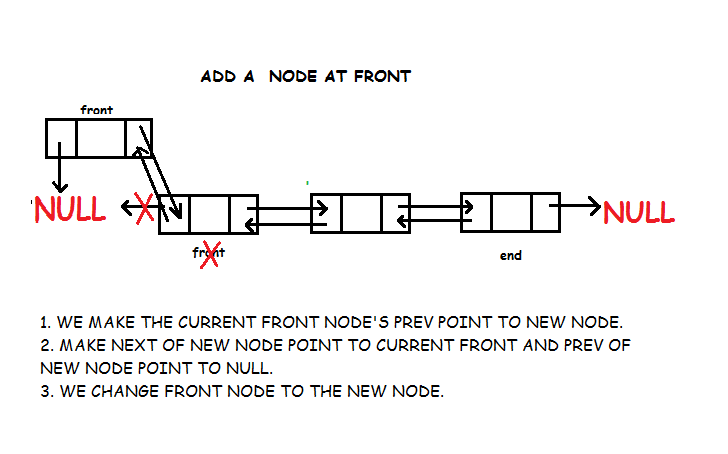
void Doubly_Linked_List :: add_front(int d)
{
// Creating new node
node *temp;
temp = new node();
temp->data = d;
temp->prev = NULL;
temp->next = front;
// List is empty
if(front == NULL)
end = temp;
else
front->prev = temp;
front = temp;
}
在节点之前插入数据 (Insert Data before a Node)
Let’s say we are inserting node X before Y. Then X’s next pointer will point to Y and X’s prev pointer will point the node Y’s prev pointer is pointing. And Y’s prev pointer will now point to X. We need to make sure that if Y is the first node of list then after adding X we make front point to X.
假设我们在Y之前插入节点X。然后X的下一个指针将指向Y,X的prev指针将指向节点Y的prev指针指向。 Y的prev指针现在将指向X。我们需要确保,如果Y是列表的第一个节点,那么在添加X之后,我们将指向X的前端。
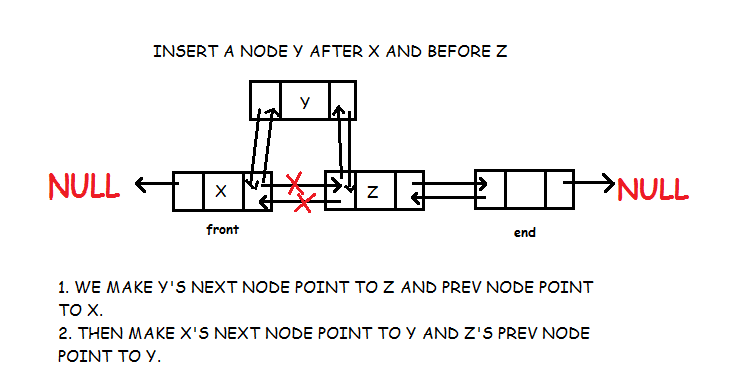
void Doubly_Linked_List :: add_before(node *n, int d)
{
node *temp;
temp = new node();
temp->data = d;
temp->next = n;
temp->prev = n->prev;
n->prev = temp;
//if node is to be inserted before first node
if(n->prev == NULL)
front = temp;
}
在节点之后插入数据 (Insert Data after a Node)
Let’s say we are inserting node Y after X. Then Y’s prev pointer will point to X and Y’s next pointer will point the node X’s next pointer is pointing. And X’s next pointer will now point to Y. We need to make sure that if X is the last node of list then after adding Y we make end point to Y.
假设我们在X之后插入节点Y。然后,Y的上一个指针将指向X,Y的下一个指针将指向节点X的下一个指针指向。 X的下一个指针现在将指向Y。我们需要确保,如果X是列表的最后一个节点,那么在添加Y之后,我们将端点指向Y。
void Doubly_Linked_List :: add_after(node *n, int d)
{
node *temp;
temp = new node();
temp->data = d;
temp->prev = n;
temp->next = n->next;
n->next = temp;
//if node is to be inserted after last node
if(n->next == NULL)
end = temp;
}
最后插入数据 (Insert Data in the end)
The next pointer of last node will always be NULL and prev will point to end.
最后一个节点的下指针将始终为NULL和prev将指向结束。
If the node is inserted is the first node of the list then we make front and end point to this node.
如果插入的节点是列表的第一个节点,则我们将前端和终点指向该节点。
Else we only make end point to this node.
否则,我们仅将端点指向此节点。
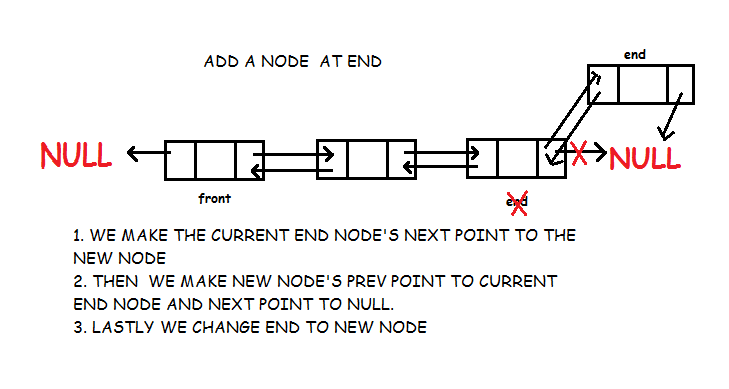
void Doubly_Linked_List :: add_end(int d)
{
// create new node
node *temp;
temp = new node();
temp->data = d;
temp->prev = end;
temp->next = NULL;
// if list is empty
if(end == NULL)
front = temp;
else
end->next = temp;
end = temp;
}
删除节点 (Remove a Node)
Removal of a node is quite easy in Doubly linked list but requires special handling if the node to be deleted is first or last element of the list. Unlike singly linked list where we require the previous node, here only the node to be deleted is needed. We simply make the next of the previous node point to next of current node (node to be deleted) and prev of next node point to prev of current node. Look code for more details.
在双向链接列表中,删除节点非常容易,但是如果要删除的节点是列表的第一个或最后一个元素,则需要特殊处理。 与我们需要上一个节点的单链表不同,这里仅需要删除的节点。 我们只需使上一个节点的下一个指向当前节点的下一个(要删除的节点),然后使下一个节点的prev指向当前节点的上一个。 查看代码以获取更多详细信息。
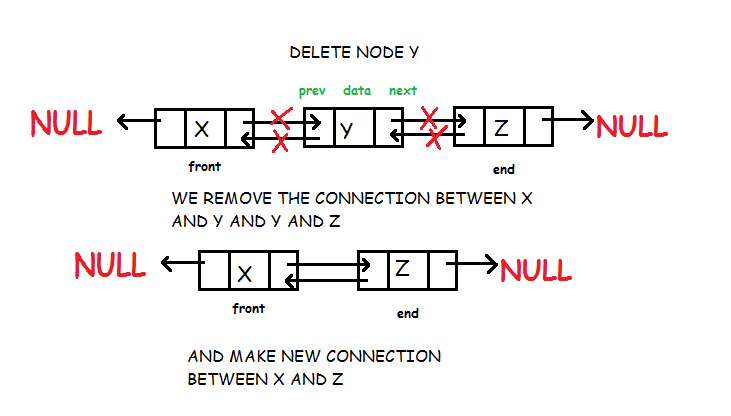
void Doubly_Linked_List :: delete_node(node *n)
{
// if node to be deleted is first node of list
if(n->prev == NULL)
{
front = n->next; //the next node will be front of list
front->prev = NULL;
}
// if node to be deleted is last node of list
else if(n->next == NULL)
{
end = n->prev; // the previous node will be last of list
end->next = NULL;
}
else
{
//previous node's next will point to current node's next
n->prev->next = n->next;
//next node's prev will point to current node's prev
n->next->prev = n->prev;
}
//delete node
delete(n);
}
向前遍历 (Forward Traversal)
Start with the front node and visit all the nodes untill the node becomes NULL.
从前端节点开始并访问所有节点,直到该节点变为NULL。
void Doubly_Linked_List :: forward_traverse()
{
node *trav;
trav = front;
while(trav != NULL)
{
cout<<trav->data<<endl;
trav = trav->next;
}
}
向后遍历 (Backward Traversal)
Start with the end node and visit all the nodes until the node becomes NULL.
从结束节点开始,并访问所有节点,直到该节点变为NULL。
void Doubly_Linked_List :: backward_traverse()
{
node *trav;
trav = end;
while(trav != NULL)
{
cout<<trav->data<<endl;
trav = trav->prev;
}
}
翻译自: https://www.studytonight.com/data-structures/doubly-linked-list
双链表排序