数据结构堆栈 内存堆栈
Stack is an abstract data type with a bounded(predefined) capacity. It is a simple data structure that allows adding and removing elements in a particular order. Every time an element is added, it goes on the top of the stack and the only element that can be removed is the element that is at the top of the stack, just like a pile of objects.
堆栈是具有有限(预定义)容量的抽象数据类型。 它是一个简单的数据结构,允许按特定顺序添加和删除元素。 每次添加元素时,它都会位于堆栈的顶部 ,唯一可以删除的元素是位于堆栈顶部的元素,就像一堆对象一样。
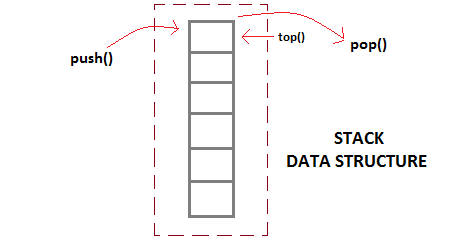
堆栈的基本功能 (Basic features of Stack)
Stack is an ordered list of similar data type.
堆栈是类似数据类型的有序列表 。
Stack is a LIFO(Last in First out) structure or we can say FILO(First in Last out).
Stack是LIFO ( 后进先出)结构,或者我们可以说FILO ( 后进先出)。
push()
function is used to insert new elements into the Stack andpop()
function is used to remove an element from the stack. Both insertion and removal are allowed at only one end of Stack called Top.push()
函数用于将新元素插入到堆栈中,而pop()
函数用于从堆栈中删除元素。 插入和移除都只能在Stack的称为Top的一端进行。Stack is said to be in Overflow state when it is completely full and is said to be in Underflow state if it is completely empty.
堆栈被认为是溢出状态,当它完全充满,被认为是下溢状态,如果它完全是空的。
堆栈的应用 (Applications of Stack)
The simplest application of a stack is to reverse a word. You push a given word to stack - letter by letter - and then pop letters from the stack.
堆栈最简单的应用是反转一个单词。 您将给定的单词按字母顺序推入堆栈,然后从堆栈中弹出字母。
There are other uses also like:
还有其他用途,例如:
Parsing
解析中
Expression Conversion(Infix to Postfix, Postfix to Prefix etc)
表达式转换(后缀为前缀,后缀为前缀等)
堆栈数据结构的实现 (Implementation of Stack Data Structure)
Stack can be easily implemented using an Array or a Linked List. Arrays are quick, but are limited in size and Linked List requires overhead to allocate, link, unlink, and deallocate, but is not limited in size. Here we will implement Stack using array.
使用数组或链接列表可以轻松实现堆栈。 数组速度很快,但是大小有限,“链接列表”需要开销来分配,链接,取消链接和取消分配,但大小不受限制。 在这里,我们将使用数组实现Stack。
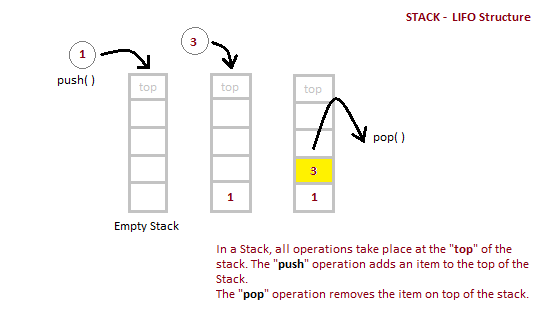
PUSH操作的算法 (Algorithm for PUSH operation)
Check if the stack is full or not.
检查堆栈是否已满 。
If the stack is full, then print error of overflow and exit the program.
如果堆栈已满,则打印溢出错误并退出程序。
If the stack is not full, then increment the top and add the element.
如果堆栈未满,则增加顶部并添加元素。
POP操作的算法 (Algorithm for POP operation)
Check if the stack is empty or not.
检查堆栈是否为空。
If the stack is empty, then print error of underflow and exit the program.
如果堆栈为空,则输出下溢错误并退出程序。
If the stack is not empty, then print the element at the top and decrement the top.
如果堆栈不为空,则在顶部打印元素,然后在顶部递减。
Below we have a simple C++ program implementing stack data structure while following the object oriented programming concepts.
下面我们有一个简单的C ++程序,它在遵循面向对象的编程概念的同时实现了堆栈数据结构。
/* Below program is written in C++ language */
# include<iostream>
using namespace std;
class Stack
{
int top;
public:
int a[10]; //Maximum size of Stack
Stack()
{
top = -1;
}
// declaring all the function
void push(int x);
int pop();
void isEmpty();
};
// function to insert data into stack
void Stack::push(int x)
{
if(top >= 10)
{
cout << "Stack Overflow \n";
}
else
{
a[++top] = x;
cout << "Element Inserted \n";
}
}
// function to remove data from the top of the stack
int Stack::pop()
{
if(top < 0)
{
cout << "Stack Underflow \n";
return 0;
}
else
{
int d = a[top--];
return d;
}
}
// function to check if stack is empty
void Stack::isEmpty()
{
if(top < 0)
{
cout << "Stack is empty \n";
}
else
{
cout << "Stack is not empty \n";
}
}
// main function
int main() {
Stack s1;
s1.push(10);
s1.push(100);
/*
preform whatever operation you want on the stack
*/
}
Position of Top
|
Status of Stack
|
---|---|
-1 | Stack is Empty |
0 | Only one element in Stack |
N-1 | Stack is Full |
N | Overflow state of Stack |
顶端位置
|
堆叠状态
|
---|---|
-1 | 堆栈为空 |
0 | 堆栈中只有一个元素 |
N-1 | 堆栈已满 |
N | 堆栈溢出状态 |
堆栈操作分析 (Analysis of Stack Operations)
Below mentioned are the time complexities for various operations that can be performed on the Stack data structure.
下面提到的是可以在堆栈数据结构上执行的各种操作的时间复杂度。
Push Operation : O(1)
推入操作 :O(1)
Pop Operation : O(1)
弹出操作 :O(1)
Top Operation : O(1)
最高操作 :O(1)
Search Operation : O(n)
搜索操作 :O(n)
The time complexities for push()
and pop()
functions are O(1)
because we always have to insert or remove the data from the top of the stack, which is a one step process.
push()
和pop()
函数的时间复杂度为O(1)
因为我们总是必须从堆栈顶部插入或删除数据,这是一个一步的过程。
翻译自: https://www.studytonight.com/data-structures/stack-data-structure
数据结构堆栈 内存堆栈