java 创建对象方法
Java is an object-oriented language, everything revolve around the object. An object represents runtime entity of a class and is essential to call variables and methods of the class.
Java是一种面向对象的语言,一切都围绕对象。 对象代表类的运行时实体 ,并且对于调用类的变量和方法至关重要 。
To create an object Java provides various ways that we are going to discuss in this topic.
创建对象Java提供了本主题将要讨论的各种方法。
New keyword
新关键字
New instance
新实例
Clone method
克隆方法
Deserialization
反序列化
NewInstance() method
NewInstance()方法
1)新关键字 (1) new Keyword)
In Java, creating objects using new keyword is very popular and common. Using this method user or system defined default constructor is called that initialize instance variables. And new keyword creates a memory area in heap to store created object.
在Java中,使用new关键字创建对象非常普遍。 使用此方法,将调用用户或系统定义的默认构造函数来初始化实例变量。 并且new关键字在堆中创建一个存储区来存储创建的对象。
Example:
例:
In this example, we are creating an object by using new keyword.
在此示例中,我们使用new关键字创建一个对象。
public class NewKeyword
{
String s = "studytonight";
public static void main(String as[])
{
NewKeyword a = new NewKeyword();
System.out.println(a.s);
}
}
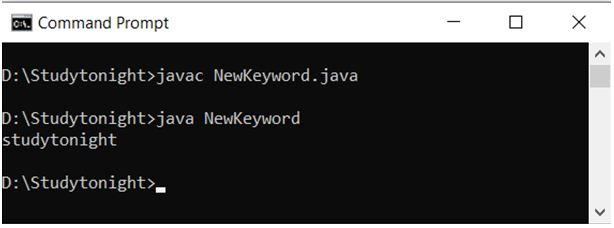
2)新实例 (2) New Instance)
In this case, we use a static method forName()
of Class class. This method loads the class and returns an object of type Class. That further we cast in our required type to get required type of object. This is what we do in this case.
在这种情况下,我们使用Class类的静态方法forName()
。 此方法加载类并返回Class类型的对象。 此外,我们强制转换了所需的类型以获得所需的对象类型。 在这种情况下,我们就是这样做的。
Example:
例:
You can see the example and understand that we loaded our class NewInstance by using Class.forName() method that returns the type Class object.
您可以看到该示例,并了解我们已经使用返回类型Class对象的Class.forName()方法加载了类NewInstance 。
public class NewInstance
{
String a = "studytonight";
public static void main(String[] args)
{
try
{
Class b = Class.forName("NewInstance");
NewInstance c = (NewInstance) b.newInstance();
System.out.println(c.a);
}
catch (ClassNotFoundException e)
{
e.printStackTrace();
}
catch (InstantiationException e)
{
e.printStackTrace();
}
catch (IllegalAccessException e)
{
e.printStackTrace();
}
}
}
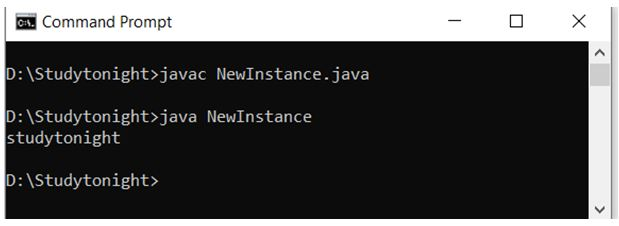
3)Clone()方法 (3) Clone() method)
In Java, clone() is called on an object. When a clone() method is called JVM creates a new object and then copy all the content of the old object into it. When an object is created using the clone() method, a constructor is not invoked. To use the clone() method in a program the class implements the cloneable and then define the clone() method.
在Java中,对对象调用clone()。 调用clone()方法时,JVM将创建一个新对象,然后将旧对象的所有内容复制到其中。 使用clone()方法创建对象时,不会调用构造函数。 要在程序中使用clone()方法,该类将实现可克隆对象,然后定义clone()方法。
Example:
例:
In this example, we are creating an object using clone()
method.
在此示例中,我们将使用clone()
方法创建一个对象。
public class CloneEg implements Cloneable
{
@Override
protected Object clone() throws CloneNotSupportedException
{
return super.clone();
}
String s = "studytonight";
public static void main(String[] args)
{
CloneEg a= new CloneEg();
try
{
CloneEg b = (CloneEg) a.clone();
System.out.println(b.s);
}
catch (CloneNotSupportedException e)
{
e.printStackTrace();
}
}
}
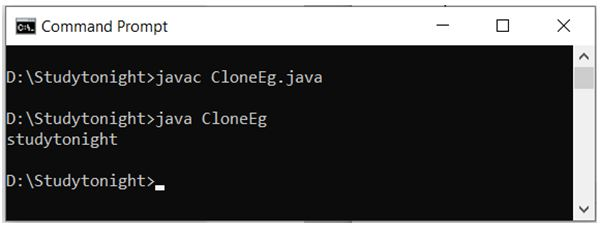
4)反序列化 (4) deserialization)
In Java, when an object is serialized and then deserialized, JVM create another separate object. When deserialization is performed JVM does not use any constructor for creating an object.
在Java中,当将对象序列化然后反序列化时,JVM将创建另一个单独的对象。 执行反序列化时,JVM不会使用任何构造函数来创建对象。
Example:
例:
Lets see an example of creating object by deserialization concept.
让我们看一个通过反序列化概念创建对象的示例。
import java.io.*;
class DeserializationEg implements Serializable
{
private String a;
DeserializationEg(String name)
{
this.a = a;
}
public static void main(String[] args)
{
try
{
DeserializationEg b = new DeserializationEg("studytonight");
FileOutputStream c = new FileOutputStream("CoreJava.txt");
ObjectOutputStream d = new ObjectOutputStream(c);
d.writeObject(b);
d.close();
d.close();
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
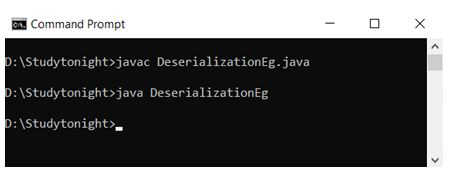
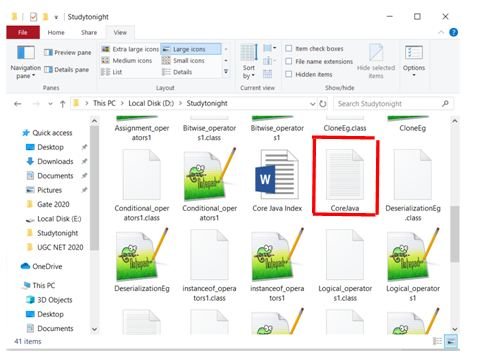
构造函数类的newInstance()方法 (newInstance() method of Constructor class)
In Java, Under java.lang.reflect package Constructor class is located. We can use it to create object. The Constructor class provides a method newInstance()
that can be used for creating an object. This method is also called a parameterized constructor.
在Java中,位于java.lang.reflect包下,构造函数类位于。 我们可以用它来创建对象。 Constructor类提供了一个newInstance()
方法,该方法可用于创建对象。 此方法也称为参数化构造函数。
Example:
例:
Lets create an example to create an object using newInstance() method of Constructor class.
让我们创建一个示例,以使用Constructor类的newInstance()方法创建对象。
import java.lang.reflect.*;
public class ReflectionEg
{
private String s;
ReflectionEg()
{
}
public void setName(String s)
{
this.s = s;
}
public static void main(String[] args)
{
try
{
Constructor
constructor = ReflectionEg.class.getDeclaredConstructor();
ReflectionEg r = constructor.newInstance();
r.setName("studytonight");
System.out.println(r.s);
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
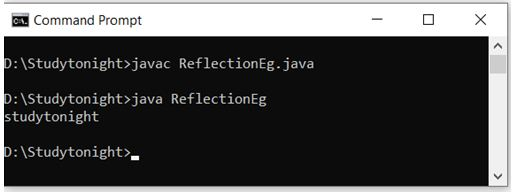
翻译自: https://www.studytonight.com/java/ways-to-create-object-in-java.php
java 创建对象方法