队列 数据结构
Queue is also an abstract data type or a linear data structure, just like stack data structure, in which the first element is inserted from one end called the REAR(also called tail), and the removal of existing element takes place from the other end called as FRONT(also called head).
队列也是抽象数据类型或线性数据结构,就像堆栈数据结构一样 ,其中第一个元素从称为REAR的一端(也称为tail )插入,而现有元素的删除从另一端进行称为FRONT (也称为head )。
This makes queue as FIFO(First in First Out) data structure, which means that element inserted first will be removed first.
这使队列成为FIFO (先进先出)数据结构,这意味着首先插入的元素将被首先移除。
Which is exactly how queue system works in real world. If you go to a ticket counter to buy movie tickets, and are first in the queue, then you will be the first one to get the tickets. Right? Same is the case with Queue data structure. Data inserted first, will leave the queue first.
队列系统在现实世界中正是这样工作的。 如果您去售票处购买电影票,并且排在第一位,那么您将是第一个获得票的人。 对? 队列数据结构也是如此。 首先插入的数据,将首先离开队列。
The process to add an element into queue is called Enqueue and the process of removal of an element from queue is called Dequeue.
将元素添加到队列中的过程称为Enqueue ,从队列中删除元素的过程称为Dequeue 。
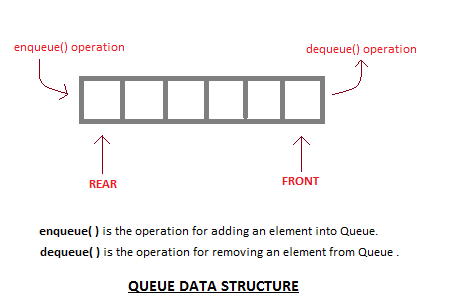