java连接数据库步骤
The following 5 steps are the basic steps involve in connecting a Java application with Database using JDBC.
以下5个步骤是使用JDBC将Java应用程序与数据库连接所涉及的基本步骤。
Register the Driver
注册驱动程序
Create a Connection
建立连接
Create SQL Statement
创建SQL语句
Execute SQL Statement
执行SQL语句
Closing the connection
断开连接
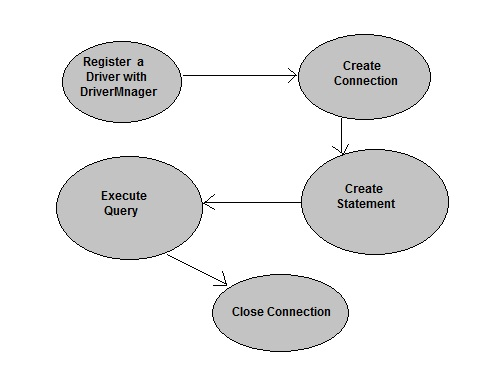
注册驱动程序 (Register the Driver)
It is first an essential part to create JDBC connection. JDBC API provides a method Class.forName()
which is used to load the driver class explicitly. For example, if we want to load a jdbc-odbc driver then the we call it like following.
首先,它是创建JDBC连接的重要部分。 JDBC API提供了一个Class.forName()
方法,该方法用于显式加载驱动程序类。 例如,如果我们要加载jdbc-odbc驱动程序,则我们将其命名如下。
向JDBC-ODBC驱动程序注册的示例 (Example to register with JDBC-ODBC Driver)
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
建立连接 (Create a Connection)
After registering and loading the driver in step1, now we will create a connection using getConnection()
method of DriverManager class. This method has several overloaded methods that can be used based on the requirement. Basically it require the database name, username and password to establish connection. Syntax of this method is given below.
在第一步中注册并加载驱动程序之后,现在我们将使用DriverManager类的getConnection()
方法创建一个连接。 此方法有几种可根据需要使用的重载方法。 基本上,它需要数据库名称,用户名和密码来建立连接。 该方法的语法如下。
句法 (Syntax)
getConnection(String url)
getConnection(String url, String username, String password)
getConnection(String url, Properties info)
This is a sample example to establish connection with Oracle Driver
这是建立与Oracle Driver的连接的示例示例
Connection con = DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:XE","username","password");
import java.sql.*;
class Test {
public static void main(String[] args) {
try {
//Loading driver
Class.forName("oracle.jdbc.driver.OracleDriver");
//creating connection
Connection con = DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:XE", "username", "password");
Statement s = con.createStatement(); //creating statement
ResultSet rs = s.executeQuery("select * from Student"); //executing statement
while (rs.next()) {
System.out.println(rs.getInt(1) + " " + rs.getString(2));
}
con.close(); //closing connection
} catch (Exception e) {
e.printStacktrace();
}
}
}
创建SQL语句 (Create SQL Statement)
In this step we will create statement object using createStatement() method. It is used to execute the sql queries and defined in Connection class. Syntax of the method is given below.
在这一步中,我们将使用createStatement()方法创建语句对象。 它用于执行sql查询,并在Connection类中定义。 该方法的语法如下。
句法 (Syntax)
public Statement createStatement() throws SQLException
创建SQL语句的示例 (Example to create a SQL statement)
Statement s=con.createStatement();
执行SQL语句 (Execute SQL Statement)
After creating statement, now execute using executeQuery()
method of Statement interface. This method is used to execute SQL statements. Syntax of the method is given below.
创建语句后,现在使用Statement接口的executeQuery()
方法执行。 此方法用于执行SQL语句。 该方法的语法如下。
句法 (Syntax)
public ResultSet executeQuery(String query) throws SQLException
执行SQL语句的示例 (Example to execute a SQL statement)
In this example, we are executing a sql query to select all the records from the user table and stored into resultset that further is used to display the records.
在此示例中,我们正在执行sql查询,以从用户表中选择所有记录,并将其存储到结果集中,该结果集还用于显示记录。
ResultSet rs=s.executeQuery("select * from user");
while(rs.next())
{
System.out.println(rs.getString(1)+" "+rs.getString(2));
}
断开连接 (Closing the connection)
This is final step which includes closing all the connection that we opened in our previous steps. After executing SQL statement you need to close the connection and release the session. The close()
method of Connection interface is used to close the connection.
这是最后一步,其中包括关闭在先前步骤中打开的所有连接。 执行SQL语句后,您需要关闭连接并释放会话。 Connection接口的close()
方法用于关闭连接。
句法 (Syntax)
public void close() throws SQLException
关闭连接的示例 (Example of closing a connection)
con.close();
Now lets combine all these steps into a single example and create a complete example of JDBC connectivity.
现在,将所有这些步骤组合为一个示例,并创建一个完整的JDBC连接示例。
示例:所有步骤合而为一 (Example: All Steps into one place)
import java.sql.*;
class Test {
public static void main(String[] args) {
try {
//Loading driver
Class.forName("oracle.jdbc.driver.OracleDriver");
//creating connection
Connection con = DriverManager.getConnection("jdbc:oracle:thin:@localhost:1521:XE", "username", "password");
Statement s = con.createStatement(); //creating statement
ResultSet rs = s.executeQuery("select * from Student"); //executing statement
while (rs.next()) {
System.out.println(rs.getInt(1) + " " + rs.getString(2));
}
con.close(); //closing connection
} catch (Exception e) {
e.printStacktrace();
}
}
}
翻译自: https://www.studytonight.com/java/steps-to-connect-to-database-in-java.php
java连接数据库步骤