java 序列化和反序列化
Serialization is a process of converting an object into a sequence of bytes which can be persisted to a disk or database or can be sent through streams. The reverse process of creating object from sequence of bytes is called deserialization.
序列化是将对象转换为字节序列的过程,这些字节序列可以保留到磁盘或数据库中,也可以通过流发送。 从字节序列创建对象的相反过程称为反序列化 。
A class must implement Serializable interface present in java.io
package in order to serialize its object successfully. Serializable is a marker interface that adds serializable behaviour to the class implementing it.
为了成功序列化其对象,类必须实现java.io
包中存在的Serializable接口。 可序列化是一个标记接口 ,可将可序列化行为添加到实现它的类中。
Java provides Serializable API encapsulated under java.io
package for serializing and deserializing objects which include,
Java提供了封装在java.io
包下的Serializable API,用于对对象进行序列化和反序列化,这些对象包括:
java.io.serializable
java.io.serializable
java.io.Externalizable
java.io.Externalizable
ObjectInputStream
ObjectInputStream
ObjectOutputStream
ObjectOutputStream
Java Marker接口 (Java Marker interface)
Marker Interface is a special interface in Java without any field and method. Marker interface is used to inform compiler that the class implementing it has some special behaviour or meaning. Some example of Marker interface are,
标记接口是Java中的一种特殊接口,没有任何字段和方法。 标记接口用于通知编译器实现它的类具有某些特殊的行为或含义。 Marker界面的一些示例是,
java.io.serializable
java.io.serializable
java.lang.Cloneable
java.lang.Cloneable
java.rmi.Remote
java.rmi.Remote
java.util.RandomAccess
java.util.RandomAccess
All these interfaces does not have any method and field. They only add special behavior to the classes implementing them. However marker interfaces have been deprecated since Java 5, they were replaced by Annotations. Annotations are used in place of Marker Interface that play the exact same role as marker interfaces did before.
所有这些接口都没有任何方法和字段。 它们仅向实现它们的类添加特殊行为。 但是,从Java 5开始,标记接口已被弃用,而由Annotations代替。 使用注释代替标记接口,其作用与标记接口完全相同。
To implement serialization and deserialization, Java provides two classes ObjectOutputStream and ObjectInputStream.
为了实现序列化和反序列化,Java提供了两个类ObjectOutputStream和ObjectInputStream。
ObjectOutputStream类 (ObjectOutputStream class)
It is used to write object states to the file. An object that implements java.io.Serializable interface can be written to strams. It provides various methods to perform serialization.
它用于将对象状态写入文件。 可以将实现java.io.Serializable接口的对象写入strams中。 它提供了多种执行序列化的方法。
ObjectInputStream类 (ObjectInputStream class)
An ObjectInputStream deserializes objects and primitive data written using an ObjectOutputStream.
ObjectInputStream反序列化使用ObjectOutputStream编写的对象和原始数据。
writeObject()
和readObject()
方法 (writeObject()
and readObject()
Methods)
The writeObject()
method of ObjectOutputStream
class serializes an object and send it to the output stream.
ObjectOutputStream
类的writeObject()
方法序列化一个对象,并将其发送到输出流。
publicfinal void writeObject(object x) throws IOException
The readObject()
method of ObjectInputStream
class references object out of stream and deserialize it.
ObjectInputStream
类的readObject()
方法从流中引用对象并将其反序列化。
public finalObject readObject() throws IOException,ClassNotFoundException
while serializing if you do not want any field to be part of object state then declare it either static or transient based on your need and it will not be included during java serialization process.
在序列化时,如果您不希望任何字段成为对象状态的一部分,则根据需要将其声明为静态或瞬态 ,并且在Java序列化过程中将不包括该字段。
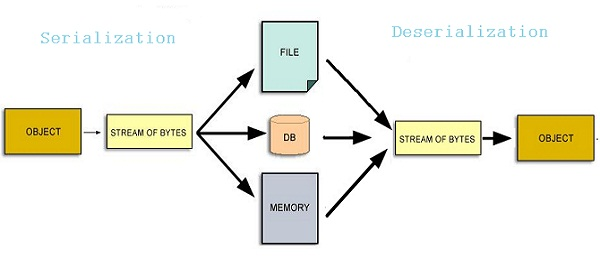
示例:使用Java序列化对象 (Example: Serializing an Object in Java)
In this example, we have a class that implements Serializable interface to make its object serialized.
在此示例中,我们有一个实现Serializable接口的类,以使其对象序列化。
import java.io.*;
class Studentinfo implements Serializable
{
String name;
int rid;
static String contact;
Studentinfo(String n, int r, String c)
{
this.name = n;
this.rid = r;
this.contact = c;
}
}
class Demo
{
public static void main(String[] args)
{
try
{
Studentinfo si = new Studentinfo("Abhi", 104, "110044");
FileOutputStream fos = new FileOutputStream("student.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(si);
oos.flush();
oos.close();
}
catch (Exception e)
{
System.out.println(e);
}
}
}
Object of Studentinfo class is serialized using writeObject()
method and written to student.txt
file.
使用writeObject()
方法序列化Studentinfo类的对象,并将其写入student.txt
文件。
示例:Java中对象的反序列化 (Example : Deserialization of Object in Java)
To deserialize the object, we are using ObjectInputStream class that will read the object from the specified file. See the below example.
为了反序列化对象,我们使用ObjectInputStream类,该类将从指定的文件中读取对象。 请参见以下示例。
import java.io.*;
class Studentinfo implements Serializable
{
String name;
int rid;
static String contact;
Studentinfo(String n, int r, String c)
{
this.name = n;
this.rid = r;
this.contact = c;
}
}
class Demo
{
public static void main(String[] args)
{
Studentinfo si=null ;
try
{
FileInputStream fis = new FileInputStream("/filepath/student.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
si = (Studentinfo)ois.readObject();
}
catch (Exception e)
{
e.printStackTrace(); }
System.out.println(si.name);
System.out. println(si.rid);
System.out.println(si.contact);
}
}
Abhi 104 null
Abhi 104空
Contact field is null because,it was marked as static and as we have discussed earlier static fields does not get serialized.
联系人字段为null,因为它被标记为静态,并且正如我们之前讨论的,静态字段不会被序列化。
NOTE: Static members are never serialized because they are connected to class not object of class.
注意:静态成员永远不会序列化,因为它们连接到类而不是类的对象。
transient
关键字 (transient
Keyword)
While serializing an object, if we don't want certain data member of the object to be serialized we can mention it transient. transient keyword will prevent that data member from being serialized.
在序列化对象时,如果我们不希望序列化对象的某些数据成员,则可以将其称为瞬态。 瞬态关键字将防止该数据成员被序列化。
class studentinfo implements Serializable
{
String name;
transient int rid;
static String contact;
}
Making a data member
transient
will prevent its serialization.使数据成员成为
transient
将阻止其序列化。In this example
rid
will not be serialized because it is transient, andcontact
will also remain unserialized because it is static.在此示例中,
rid
将不会被序列化,因为它是瞬时的 ,而contact
也将保持未序列化,因为它是静态的 。
翻译自: https://www.studytonight.com/java/serialization-and-deserialization.php
java 序列化和反序列化