java类包装器有什么用
In Java, Wrapper Class is used for converting primitive data type into object and object into a primitive data type. For each primitive data type, a pre-defined class is present which is known as Wrapper class. From J2SE 5.0 version the feature of autoboxing and unboxing is used for converting primitive data type into object and object into a primitive data type automatically.
在Java中,包装程序类用于将原始数据类型转换为对象,并将对象转换为原始数据类型。 对于每种原始数据类型,存在一个预定义的类,称为Wrapper类。 从J2SE 5.0版本开始,自动装箱和拆箱功能用于将原始数据类型转换为对象,并将对象自动转换为原始数据类型。
为什么要使用包装器类? (Why Use Wrapper Classes?)
As we knew that in Java when input is given by the user, it is in the form of String. To convert a string into different data types, Wrapper classes are used.
众所周知,在Java中,当用户提供输入时,它采用String的形式。 要将字符串转换为不同的数据类型,请使用Wrapper类。
We can use wrapper class each time when want to convert primitive to object or vice versa.
每次要将原语转换为对象时都可以使用包装器类,反之亦然。
The following are the Primitive Data types with their Name of wrapper class and the method used for the conversation.
以下是原始数据类型及其包装类名称和用于对话的方法。
Primitive DataType | Wrapper ClassName | Conversion methods |
---|---|---|
byte | byte | public static byte parseByte(String) |
short | Short | public static short parseShort(String) |
int | Integer | public static int parseInt(String) |
long | Long | public static long parseLong(String) |
float | Float | public static float parseFloat(String) |
double | Double | public static double parseDouble(String) |
char | Character | |
boolean | Boolean | public static boolean parseBoolean(String) |
原始数据类型 | 包装类名称 | 转换方式 |
---|---|---|
字节 | 字节 | 公共静态字节parseByte(String) |
短 | 短 | 公共静态短parseShort(String) |
整型 | 整数 | 公共静态int parseInt(String) |
长 | 长 | 公共静态长parseLong(String) |
浮动 | 浮动 | 公共静态float parseFloat(String) |
双 | 双 | 公共静态double parseDouble(String) |
烧焦 | 字符 | |
布尔值 | 布尔型 | 公共静态布尔parseBoolean(String) |
In Java, all the primitive wrapper classes are immutable. When a new object is created the old object is not modified. Below is an example to demonstrate this concept.
在Java中,所有原始包装器类都是不可变的。 创建新对象时,不会修改旧对象。 下面是一个示例来演示此概念。
例: (Example:)
class WrapperPrimitiveDemo1
{
public static void main(String[] args)
{
Integer a = new Integer(12);
System.out.println("Old value = "+a);
xyz(a);
System.out.println("New Value = "+a);
}
private static void xyz(Integer a)
{
a = a + 10;
}
}
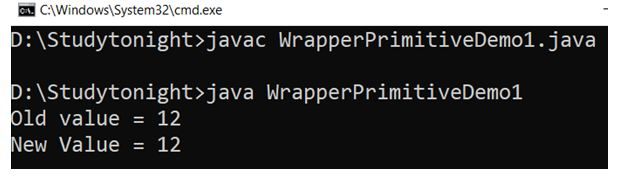
编号等级 (Number Class)
Java Number class is the super class of all the numeric wrapper classes. There are 6 sub classes, you can get the idea by following image.
Java Number类是所有数字包装器类的超类。 有6个子类,您可以通过下面的图像来了解这个想法。
The Number class contains some methods to provide the common operations for all the sub classes.
Number类包含一些方法,可为所有子类提供通用操作。
以下是带有示例的Number类的方法 (Following are the methods of Number class with there example)
1. Value()方法 (1. Value() Method)
This method is used for converting numeric object into a primitive data type. For example we can convert a Integer object to int or Double object to float type. The value() method is available for each primitive type and syntax are given below.
此方法用于将数字对象转换为原始数据类型。 例如,我们可以将Integer对象转换为int,或者将Double对象转换为float类型。 value()方法可用于每种基本类型,语法在下面给出。
句法: (Syntax:)
byte byteValue()
short shortValue()
int intValue()
long longValue()
float floatValue()
double doubleValue()
例: (Example:)
Here we are using several methods like: byteValue(), intValue(), floatValue()
etc for convert object type to primitive type. The double type object is used to fetch different types of primitive values.
这里我们使用几种方法: byteValue(), intValue(), floatValue()
等将对象类型转换为原始类型。 double类型的对象用于获取不同类型的原始值。
public class NumberDemo1
{
public static void main(String[] args)
{
Double d1 = new Double("4.2365");
byte b = d1.byteValue();
short s = d1.shortValue();
int i = d1.intValue();
long l = d1.longValue();
float f = d1.floatValue();
double d = d1.doubleValue();
System.out.println("converting Double to byte : " + b);
System.out.println("converting Double to short : " + s);
System.out.println("converting Double to int : " + i);
System.out.println("converting Double to long : " + l);
System.out.println("converting Double to float : " + f);
System.out.println("converting Double to double : " + d1);
}
}
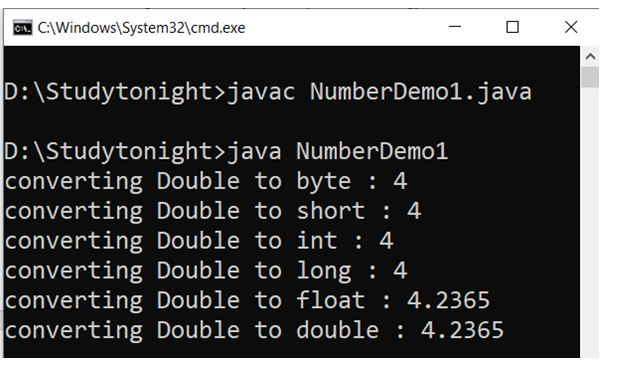
Java Integer类 (Java Integer class)
Java Integer class is used to handle integer objects. It provides methods that can be used for conversion primitive to object and vice versa.
Java Integer类用于处理整数对象。 它提供了可用于将原语转换为对象以及反之亦然的方法。
It wraps a value of the primitive type int in an object. This class provides several methods for converting an int to a String and a String to an int, as well as other constants and methods helpful while working with an int.
它将原始类型int的值包装在对象中。 此类提供了几种将int转换为String并将String转换为int的方法,以及在使用int时有用的其他常量和方法。
It is located into java.lang pakage and java.base module. Declaration of this class is given below.
它位于java.lang pakage和java.base模块中。 此类的声明在下面给出。
宣言 (Declaration)
public final class Integer extends Number implements Comparable<Integer>
Methods of Integer class with examples are discussed below.
下面讨论带有实例的Integer类的方法。
1. toString()方法 (1. toString() Method)
This method returns a String object representing this Integer's value. The value is converted to signed decimal representation and returned as a string. It overrides toString()
method of Object class.
此方法返回一个表示此Integer值的String对象。 该值将转换为带符号的十进制表示形式,并作为字符串返回。 它重写Object类的toString()
方法。
It does not take any argument but returns a string representation of the value of this object in base 10. Syntax of this method is given below.
它不带任何参数,但以10为基数返回此对象的值的字符串表示形式。下面给出了此方法的语法。
public String toString(int b)
例: (Example:)
In this example, we are using toString method to get string representation of Integer object.
在此示例中,我们使用toString方法获取Integer对象的字符串表示形式。
public class IntegerClassDemo1
{
public static void main(String args[])
{
int a = 95;
Integer x = new Integer(a);
System.out.println("toString(a) = " + Integer.toString(a));
}
}
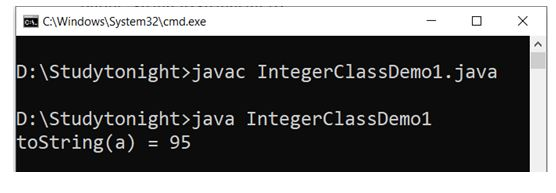
2. toHexString() (2. toHexString())
The toHexString()
method is used to get a string representation of the integer argument as an unsigned integer in base 16.
toHexString()
方法用于获取整数参数的字符串表示形式,以基数16为无符号整数。
This method takes an argument of int type and returns a hexadecimal string. Syntax of the method is given below.
此方法采用int类型的参数,并返回一个十六进制字符串。 该方法的语法如下。
句法: (Syntax:)
public String toHexString(int b)
例: (Example:)
We are getting hexadecimal string value of an int value using the toHexString() method. In this example, we passed 95 as an argument and get 5f as a hexadecimal string. See the below example.
我们使用toHexString()方法获取一个int值的十六进制字符串值。 在此示例中,我们传递了95作为参数,并获得5f作为十六进制字符串。 请参见以下示例。
public class IntegerClassDemo1
{
public static void main(String args[])
{
int a = 95;
Integer x = new Integer(a);
System.out.println("toHexString(a) = " + Integer.toHexString(a));
}
}
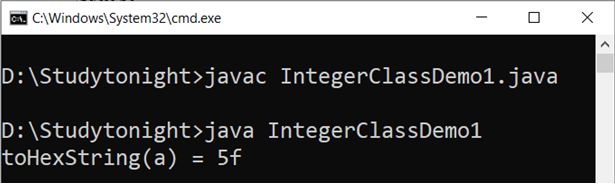
3. toOctalString() (3. toOctalString())
This method is helpful when we want to get an octal string representation of an int type value.
当我们要获取一个int类型值的八进制字符串表示形式时,此方法很有用。
It can be used to get a string representation of the integer argument as an unsigned integer in base 8.
它可以用来获取整数参数的字符串表示形式(以8为底的无符号整数)。
It takes an int type argument and returns an unsigned string representation of the argument. Syntax of this method is given below.
它接受一个int类型的参数,并返回该参数的无符号字符串表示形式。 该方法的语法如下。
句法: (Syntax:)
public String toOctalString(int b)
例: (Example:)
Lets take an example to get octal value of an int value. Here we are passing 95 to the toOctalString()
method and getting its octal value 135.
让我们举一个例子来获取一个int值的八进制值。 在这里,我们将95传递给toOctalString()
方法并获得其八进制值135。
public class IntegerClassDemo1
{
public static void main(String args[])
{
int a = 95;
Integer x = new Integer(a);
System.out.println("toOctalString(a) = " + Integer.toOctalString(a));
}
}
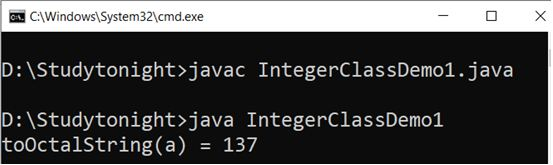
4. toBinaryString() (4. toBinaryString())
The toBinaryString()
method is used to get a string representation of the integer argument as an unsigned integer in base 2.
toBinaryString()
方法用于获取整数参数的字符串表示形式,以基数2为无符号整数。
It takes an integer argument and returns a string representation of the unsigned integer value. Syntax of the method is given below.
它使用整数参数,并返回无符号整数值的字符串表示形式。 该方法的语法如下。
句法: (Syntax:)
public String toBinaryString(int b)
例: (Example:)
In this example, we are using toBinaryString() method to get binary of an int value. It effective way to get binary of an int value.
在此示例中,我们使用toBinaryString()方法获取int值的二进制值。 它是获取int值的二进制值的有效方法。
public class IntegerClassDemo1
{
public static void main(String args[])
{
int a = 95;
Integer x = new Integer(a);
System.out.println("toBinaryString(a) = " + Integer.toBinaryString(a));
}
}
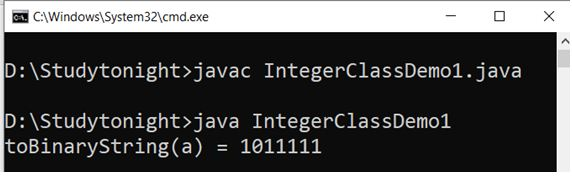
5. valueOf() (5. valueOf())
The valueOf()
method is used to get an Integer object representing the specified int value. It takes a single int type argument and returns an Integer instance. Syntax of the method is given below.
valueOf()
方法用于获取一个表示指定int值的Integer对象。 它使用一个int类型参数,并返回一个Integer实例。 该方法的语法如下。
句法: (Syntax:)
public static Integer valueOf(int b)
范例: (Exmaple:)
public class IntegerClassDemo1
{
public static void main(String args[])
{
int a = 95;
Integer x = Integer.valueOf(a);
System.out.println("valueOf(a) = " + x);
}
}
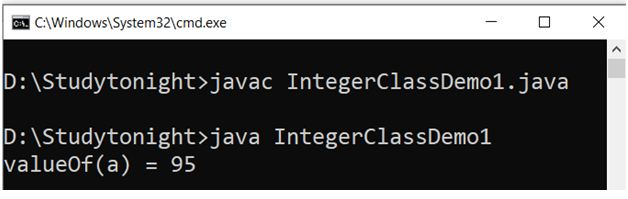
6. parseInt() (6. parseInt())
The parseInt()
method is used to parse the specified string argument as a signed decimal integer. The characters in the string must all be decimal digits.
parseInt()
方法用于将指定的字符串参数解析为带符号的十进制整数。 字符串中的字符必须全部为十进制数字。
It takes a single argument of string type and returns an int value.
它采用字符串类型的单个参数,并返回一个int值。
句法: (Syntax:)
public static intparseInt(String val, int radix) throws NumberFormatException
例: (Example:)
In the following example, we are passing a string that contains digits to parseInt()
method. The method returns an int value corresponding to the string.
在下面的示例中,我们将一个包含数字的字符串传递给parseInt()
方法。 该方法返回对应于字符串的int值。
public class IntegerClassDemo1
{
public static void main(String args[])
{
String a = "95";
Integer x = Integer.parseInt(a);
System.out.println("parseInt(a) = " + x);
}
}
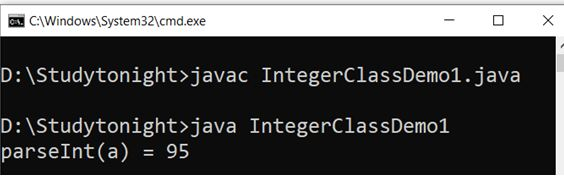
7. getInteger() (7. getInteger())
句法: (Syntax:)
public static Integer getInteger(String prop)
例: (Example:)
public class IntegerClassDemo1
{
public static void main(String args[])
{
int a = Integer.getInteger("sun.arch.data.model");
System.out.println("getInteger(sun.arch.data.model) = " + a);
}
}
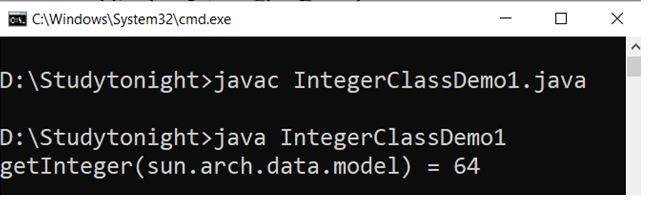
8,decode() (8. decode())
The decode()
method is used to decode a String into an Integer. It takes an string type argument that can be a decimal, hexadecimal, and octal number and returns an instance of Integer class. Syntax of the method is given below.
decode()
方法用于将String解码为Integer。 它采用可以是十进制,十六进制和八进制数字的字符串类型参数,并返回Integer类的实例。 该方法的语法如下。
句法: (Syntax:)
public static Integer decode(String s)
throws NumberFormatException
例: (Example:)
In this example, we are using different types of numerical values to decode it and get integer instance.
在此示例中,我们使用不同类型的数值对其进行解码并获取整数实例。
public class IntegerClassDemo1
{
public static void main(String args[])
{
String a = "55";
String b = "004";
String c = "0x0f";
Integer a1 = Integer.decode(a);
System.out.println("decode(55) = " + a1);
a1 = Integer.decode(b);
System.out.println("decode(004) = " + a1);
a1 = Integer.decode(c);
System.out.println("decode(0x0f) = " + a1);
}
}
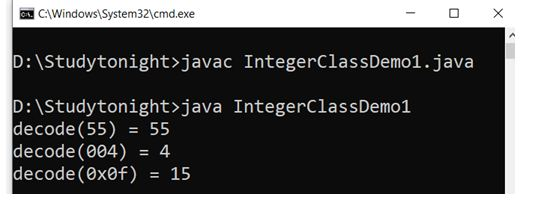
9. rotationLeft()和rotateRight() (9. rotateLeft() and rotateRight())
These two methods are used to rotate the two's complement binary representation of the specified int value left or right by the specified number of bits.
这两种方法用于将指定的int值的二进制补码二进制表示形式向左或向右旋转指定的位数。
句法: (Syntax:)
public static introtateLeft(intval, intdist)
public static introtateRight(intval, intdist)
例: (Example:)
public class IntegerClassDemo1
{
public static void main(String args[])
{
int a = 5;
System.out.println("rotateLeft = "+ Integer.rotateLeft(a, 5));
System.out.println("rotateRight = "+ Integer.rotateRight(a, 4));
}
}
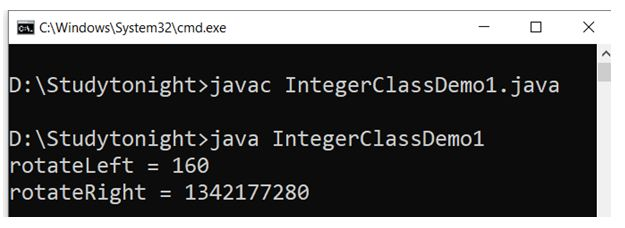
Java Long类 (Java Long class)
The Long class is a wrapper class that is used to wrap a value of the primitive type long in an object. An object of type Long contains a single field whose type is long.
Long类是一个包装器类,用于将long类型的原始类型的值包装在一个对象中。 Long类型的对象包含一个long类型的字段。
In addition, this class provides several methods for converting a long to a String and vice versa. Declaration of the class is given below.
另外,此类提供了几种将long转换为String的方法,反之亦然。 该类的声明在下面给出。
宣言 (Declaration)
public final class Long extends Number implements Comparable<Long>
This class is located into java.lang package and java.base module. Here we are discussing methods of Long class with their examples.
此类位于java.lang包和java.base模块中。 在这里,我们将讨论Long类的方法及其示例。
1. toString() (1. toString() )
This method returns a String object representing this Long's value. The value is converted to signed decimal representation and returned as a string. It overrides toString()
method of Object class.
此方法返回表示此Long值的String对象。 该值将转换为带符号的十进制表示形式,并作为字符串返回。 它重写Object类的toString()
方法。
It does not take any argument but returns a string representation of the value of this object in base 10. Syntax of this method is given below.
它不带任何参数,但以10为基数返回此对象的值的字符串表示形式。下面给出了此方法的语法。
句法: (Syntax:)
public String toString(long b)
例: (Example:)
public class LongDemo1
{
public static void main(String as[])
{
long a = 25;
System.out.println("toString(a) = " + Long.toString(a));
}
}
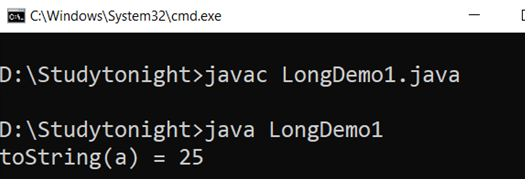
2. toHexString() (2. toHexString())
The toHexString()
method is used to get a string representation of the long argument.
toHexString()
方法用于获取long参数的字符串表示形式。
This method takes an argument of long type and returns a hexadecimal string. Syntax of the method is given below.
此方法采用long类型的参数,并返回一个十六进制字符串。 该方法的语法如下。
句法: (Syntax:)
public String toHexString(long b)
3. toOctalString() (3. toOctalString() )
This method is helpful when we want to get an octal string representation of an long type value.
当我们要获取长类型值的八进制字符串表示形式时,此方法很有用。
It can be used to get a string representation of the long argument.
它可以用来获取long参数的字符串表示形式。
It takes an long type argument and returns an unsigned string representation of the argument. Syntax of this method is given below.
它使用一个长型参数,并返回该参数的无符号字符串表示形式。 该方法的语法如下。
句法: (Syntax:)
public String toOctalString(long b)
4. toBinaryString() (4. toBinaryString() )
The toBinaryString()
method is used to get a string representation of the long argument as an unsigned integer in base 2.
toBinaryString()
方法用于获取long参数的字符串表示形式,为基数2中的无符号整数。
It takes an long argument and returns a string representation of the unsigned long value. Syntax of the method is given below.
它使用long参数,并返回无符号long值的字符串表示形式。 该方法的语法如下。
句法: (Syntax:)
public String toBinaryString(long b)
例: (Example:)
We are getting hexadecimal string value of a long value using the toHexString()
method. In this example, we passed 25 as an argument and get its binary, hex and octal string. See the below example.
我们使用toHexString()
方法获取一个长值的十六进制字符串值。 在此示例中,我们传递25作为参数,并获取其二进制,十六进制和八进制字符串。 请参见以下示例。
public class LongDemo1
{
public static void main(String as[])
{
long a = 25;
System.out.println("toHexString(a) =" + Long.toHexString(a));
System.out.println("toOctalString(a) =" + Long.toOctalString(a));
System.out.println("toBinaryString(a) =" + Long.toBinaryString(a));
}
}
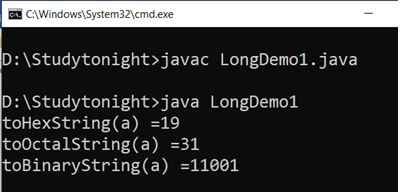
5. valueOf() (5. valueOf() )
The valueOf()
method is used to get an Long object representing the specified long value. It takes a single long type argument and returns a Long instance. Syntax of the method is given below.
valueOf()
方法用于获取表示指定长值的Long对象。 它使用一个long类型参数,并返回Long实例。 该方法的语法如下。
句法: (Syntax:)
public static Long valueOf(long b)
例: (Example:)
public class LongDemo1
{
public static void main(String as[])
{
long a = 25;
String b = "45";
Long x = Long.valueOf(a);
System.out.println("valueOf(a) = " + x);
x = Long.valueOf(b);
System.out.println("ValueOf(b) = " + x);
x = Long.valueOf(b, 6);
System.out.println("ValueOf(b,6) = " + x);
}
}
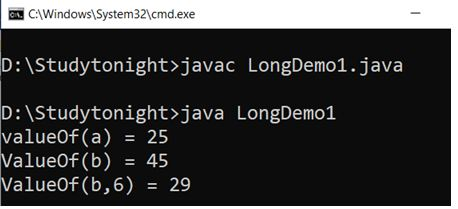
6. parseLong() (6. parseLong() )
The parseLong()
method is used to parse the specified string argument as a signed decimal Long. The characters in the string must all be decimal digits.
parseLong()
方法用于将指定的字符串参数解析为带符号的十进制Long。 字符串中的字符必须全部为十进制数字。
It takes a single argument of string type and returns a Long value.
它采用字符串类型的单个参数,并返回Long值。
句法: (Syntax: )
public static long parseInt(String val, int radix) throws NumberFormatException
例: (Example:)
In the following example, we are passing a string that contains digits to parseLong()
method. The method returns a long value corresponding to the string.
在下面的示例中,我们将包含数字的字符串传递给parseLong()
方法。 该方法返回对应于该字符串的long值。
public class LongDemo1
{
public static void main(String as[])
{
long a = 25;
String b = "45";
Long x = Long.parseLong(b);
System.out.println("parseLong(b) = " + x);
x = Long.parseLong(b, 6);
System.out.println("parseLong(b,6) = " + x);
}
}
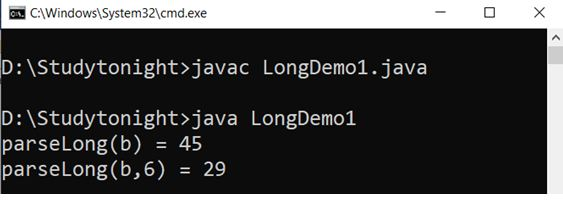
7. getLong() (7. getLong() )
The getLong()
method is used to determine the long value of the system property with the specified name. It takes a string argument that specifies name of a property and returns a Long value. Syntax of the method is given below.
getLong()
方法用于确定具有指定名称的系统属性的long值。 它使用一个字符串参数来指定属性名称,并返回Long值。 该方法的语法如下。
句法: (Syntax:)
public static Long getLong(String prop)
例: (Example:)
public class LongDemo1
{
public static void main(String as[])
{
long a = Long.getLong("sun.arch.data.model");
System.out.println("getLong(sun.arch.data.model) = " + a);
System.out.println("getLong(abcd) =" + Long.getLong("abcd"));
}
}
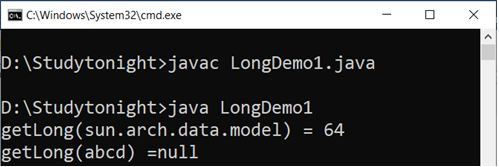
8.decode() (8.decode() )
The decode()
method is used to decode a String into Long. It takes an string type argument that can be a decimal, hexadecimal, and octal number and returns an instance of Long class. Syntax of the method is given below.
decode()
方法用于将String解码为Long。 它采用可以是十进制,十六进制和八进制数字的字符串类型参数,并返回Long类的实例。 该方法的语法如下。
句法: (Syntax:)
public static Long decode(String s) throws NumberFormatException
例: (Example:)
In this example, we are using different types of numerical values to decode and get long instance.
在此示例中,我们使用不同类型的数值来解码并获取长实例。
public class LongDemo1
{
public static void main(String as[])
{
String a = "25";
String b = "007";
String c = "0x0f";
Long x = Long.decode(a);
System.out.println("decode(25) = " + x);
x = Long.decode(b);
System.out.println("decode(007) = " + x);
x = Long.decode(c);
System.out.println("decode(0x0f) = " + x);
}
}
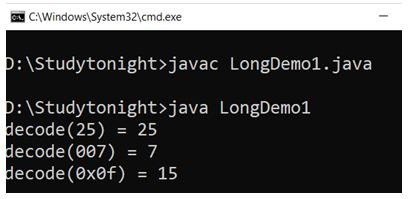
9. rotationLeft()和rotateRight() (9. rotateLeft() and rotateRight())
These two methods are used to rotate the two's complement binary representation of the specified long value left or right by the specified number of bits.
这两种方法用于将指定长值的二进制补码二进制表示形式向左或向右旋转指定的位数。
句法: (Syntax:)
public static long rotateLeft(long val, int dist)
public static long rotateRight(long val, int dist)
例: (Example:)
public class LongDemo1
{
public static void main(String as[])
{
long a = 3;
System.out.println("rotateLeft(0000 0000 0000 0011 , 3) =" + Long.rotateLeft(a, 3));
System.out.println("rotateRight(0000 0000 0000 0011 , 3) =" + Long.rotateRight(a, 3));
}
}
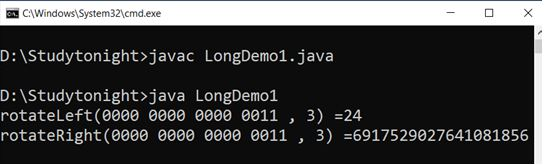
java类包装器有什么用