java线程 守护线程
Daemon threads is a low priority thread that provide supports to user threads. These threads can be user defined and system defined as well. Garbage collection thread is one of the system generated daemon thread that runs in background. These threads run in the background to perform tasks such as garbage collection. Daemon thread does allow JVM from existing until all the threads finish their execution. When a JVM founds daemon threads it terminates the thread and then shutdown itself, it does not care Daemon thread whether it is running or not.
守护程序线程是低优先级线程,为用户线程提供支持。 这些线程可以由用户定义,也可以由系统定义。 垃圾收集线程是在后台运行的系统生成的守护程序线程之一。 这些线程在后台运行以执行诸如垃圾收集之类的任务。 守护程序线程的确允许JVM从现有线程运行,直到所有线程完成其执行。 当JVM找到守护程序线程时,它将终止该线程,然后关闭自身,而不管守护程序线程是否正在运行。
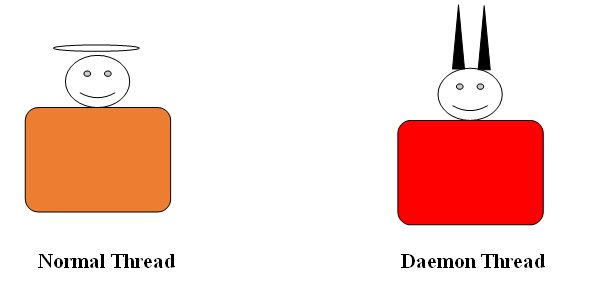
以下是守护进程线程中的方法 (Following are the methods in Daemon Thread)
1. void setDaemon(boolean status)
1. void setDaemon(布尔状态)
In Java, this method is used to create the current thread as a daemon thread or user thread. If there is a user thread as obj1 then obj1.setDaemon(true) will make it a Daemon thread and if there is a Daemon thread obj2 then calling obj2.setDaemon(false) will make it a user thread.
在Java中,此方法用于将当前线程创建为守护程序线程或用户线程。 如果存在作为obj1的用户线程,则obj1.setDaemon(true)将使其成为守护线程,如果存在daemon线程obj2,则调用obj2.setDaemon(false)将使其成为用户线程。
Syntax:
句法:
public final void setDaemon(boolean on)
2. boolean isDaemon()
2. boolean isDaemon()
In Java, this method is used to check whether the current thread is a daemon or not. It returns true if the thread is Daemon otherwise it returns false.
在Java中,此方法用于检查当前线程是否是守护程序。 如果线程是Daemon,则返回true,否则返回false。
Syntax:
句法:
public final booleanisDaemon()
例: (Example:)
Lets create an example to create daemon and user threads. To create daemon thread setdaemon() method is used. It takes boolean value either true or false.
让我们创建一个示例来创建守护程序和用户线程。 要创建守护程序线程,请使用setdaemon()方法。 它采用布尔值true或false。
public class DaemonDemo1 extends Thread
{
public DaemonDemo1(String name1)
{
super(name1);
}
public void run()
{
if(Thread.currentThread().isDaemon())
{
System.out.println(getName() + " is Daemon thread");
}
else
{
System.out.println(getName() + " is User thread");
}
}
public static void main(String[] args)
{
DaemonDemo1 D1 = new DaemonDemo1("D1");
DaemonDemo1 D2 = new DaemonDemo1("D2");
DaemonDemo1 D3 = new DaemonDemo1("D3");
D1.setDaemon(true);
D1.start();
D2.start();
D3.setDaemon(true);
D3.start();
}
}
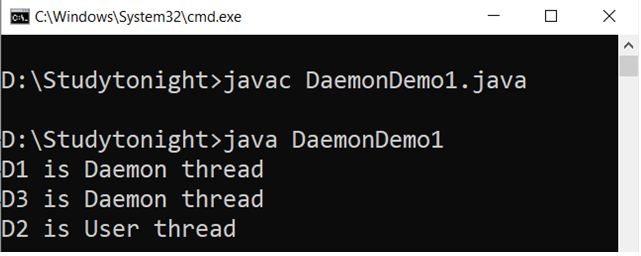
示例:守护进程线程优先级 (Example : Daemon thread Priority)
Since daemon threads are low level threads then lets check the priority of these threads. The priority we are getting is set by the JVM.
由于守护程序线程是低级线程,因此让我们检查这些线程的优先级。 我们获得的优先级是由JVM设置的。
public class DaemonDemo1 extends Thread
{
public DaemonDemo1(String name1)
{
super(name1);
}
public void run()
{
if(Thread.currentThread().isDaemon())
{
System.out.println(getName() + " is Daemon thread");
}
else
{
System.out.println(getName() + " is User thread");
}
System.out.println(getName()+" priority "+Thread.currentThread().getPriority());
}
public static void main(String[] args)
{
DaemonDemo1 D1 = new DaemonDemo1("D1");
DaemonDemo1 D2 = new DaemonDemo1("D2");
DaemonDemo1 D3 = new DaemonDemo1("D3");
D1.setDaemon(true);
D1.start();
D2.start();
D3.setDaemon(true);
D3.start();
}
}
D1 is Daemon thread D1 priority 5 D2 is User thread D3 is Daemon thread D2 priority 5 D3 priority 5
D1是守护程序线程D1优先级5 D2是用户线程D3是守护程序线程D2优先级5 D3优先级5
例 (Example)
While creating daemon thread make sure the setDaemon() is called before starting of the thread. Calling it after starting of thread will throw an exception and terminate the program execution.
创建守护程序线程时,请确保 在启动线程之前会调用setDaemon()。 在线程启动后调用它会引发异常并终止程序执行。
public class DaemonDemo1 extends Thread
{
public DaemonDemo1(String name1)
{
super(name1);
}
public void run()
{
if(Thread.currentThread().isDaemon())
{
System.out.println(getName() + " is Daemon thread");
}
else
{
System.out.println(getName() + " is User thread");
}
System.out.println(getName()+" priority "+Thread.currentThread().getPriority());
}
public static void main(String[] args)
{
DaemonDemo1 D1 = new DaemonDemo1("D1");
DaemonDemo1 D2 = new DaemonDemo1("D2");
DaemonDemo1 D3 = new DaemonDemo1("D3");
D1.setDaemon(true);
D1.start();
D2.start();
D3.start();
D3.setDaemon(true);
}
}
D1 is Daemon threadException in thread "main" D1 priority 5 D3 is User thread D2 is User thread D2 priority 5java.lang.IllegalThreadStateException D3 priority 5 at java.base/java.lang.Thread.setDaemon(Thread.java:1410) at myjavaproject.DaemonDemo1.main(DaemonDemo1.java:32)
D1是线程“主”中的守护程序threadException D1优先级5 D3是用户线程D2是用户线程D2优先级5java.lang.IllegalThreadStateException D3优先级5位于java.base / java.lang.Thread.setDaemon(Thread.java:1410) myjavaproject.DaemonDemo1.main(DaemonDemo1.java:32)
翻译自: https://www.studytonight.com/java/daemon-thread-in-java.php
java线程 守护线程