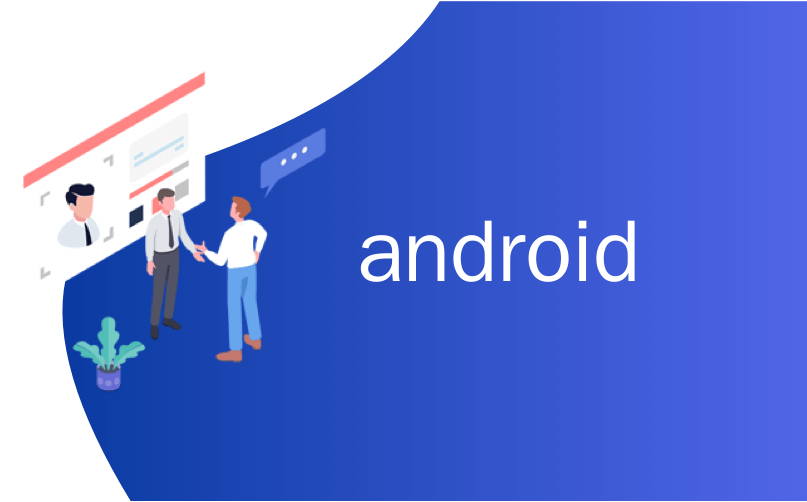
android
- 全屏启动Android应用程序
- 使用一个单独的线程来控制应用程序(游戏循环)
- 从资源加载图像
- 获取画布并在其上绘制图像
- 处理基本的触摸手势
我为此条目设置的任务很简单:使机器人在屏幕上移动。 它永远都不应离开表面,并且当它撞到屏幕边缘即墙壁时应该反弹。
如果您还记得,图像只是机器人的代表。 因此,我们将修改droid对象,并添加一些功能。 暂时只有一个。 我们的机器人是可移动的。 它可以移动。 这意味着它具有速度。 我们将赋予它移动的能力。 为此,我们将添加一个move()方法,该方法将仅基于其Speed更新X和Y坐标。 速度本身就是一个类,而Droid将包含它。 我现在将做一个具体的实现,但是稍后我将使用策略模式。
创建Speed.java类。
package net.obviam.droidz.model.components;
public class Speed {
public static final int DIRECTION_RIGHT = 1;
public static final int DIRECTION_LEFT = -1;
public static final int DIRECTION_UP = -1;
public static final int DIRECTION_DOWN = 1;
private float xv = 1; // velocity value on the X axis
private float yv = 1; // velocity value on the Y axis
private int xDirection = DIRECTION_RIGHT;
private int yDirection = DIRECTION_DOWN;
public Speed() {
this.xv = 1;
this.yv = 1;
}
public Speed(float xv, float yv) {
this.xv = xv;
this.yv = yv;
}
public float getXv() {
return xv;
}
public void setXv(float xv) {
this.xv = xv;
}
public float getYv() {
return yv;
}
public void setYv(float yv) {
this.yv = yv;
}
public int getxDirection() {
return xDirection;
}
public void setxDirection(int xDirection) {
this.xDirection = xDirection;
}
public int getyDirection() {
return yDirection;
}
public void setyDirection(int yDirection) {
this.yDirection = yDirection;
}
// changes the direction on the X axis
public void toggleXDirection() {
xDirection = xDirection * -1;
}
// changes the direction on the Y axis
public void toggleYDirection() {
yDirection = yDirection * -1;
}
}
我们将使用方向常数来确定轴上的移动方向。 机器人具有垂直和水平速度,并且在每次游戏更新时,都应考虑运动方向来设置坐标。机器人只能在画布区域上移动。 那是一个矩形和我们的2D坐标系。 与数学课程不同,原点在左上角。 因此,对于从屏幕左上角开始的机器人,其坐标将为0,0。 要沿对角线移动,速度矢量的X和Y分量的速度均为1 。 要向右下方移动,方向将是: X轴为1(右) , Y轴为1(下) 。
|
画布坐标系 |
要使机器人水平移动, Y向量的速度必须为0 。 0.5用于Y和1为X A的值就会使机器人行驶在22.5度到X轴。 简单的几何。
在Speed中,我们具有矢量分量(x和y)以及方向以及吸气剂和吸气剂。 两种方法( toggleXDirection()和toggleYDirection() )只需一次调用即可更改方向。 稍后我们将在碰撞检测(与屏幕墙一起)中看到它非常有用。
游戏循环( MainThread.java )获得了重要的修改,因为它引入了游戏更新方法。 以下代码段是更新的run()方法,仅添加了一行:
this.gamePanel.update();
run()方法:
public void run() {
Canvas canvas;
Log.d(TAG, "Starting game loop");
while (running) {
canvas = null;
// try locking the canvas for exclusive pixel editing
// in the surface
try {
canvas = this.surfaceHolder.lockCanvas();
synchronized (surfaceHolder) {
// update game state
this.gamePanel.update();
// render state to the screen
// draws the canvas on the panel
this.gamePanel.render(canvas);
}
} finally {
// in case of an exception the surface is not left in
// an inconsistent state
if (canvas != null) {
surfaceHolder.unlockCanvasAndPost(canvas);
}
} // end finally
}
}
我们将在MainGamePanel中创建相应的方法。 此方法负责更新应用程序中所有对象的状态。 目前只有机器人。 由于机器人在移动,因此我们将对墙壁进行基本的碰撞检测。 逻辑很简单。 检查机器人是否向左移动,然后检查机器人的位置是否在墙上,然后改变方向。 请记住,机器人的位置是图像的中心,因此我们需要使用图像的宽度和高度来获得正确的精度。我们还将更新机器人的位置。 为了使更新方法保持简单,我们将机器人位置的更新委托给机器人本身。 因此,机器人将获得一个update方法,如果该机器人没有被触摸手势拾取,它将继续更新其位置。 检查以前的帖子。
检查代码: MainGamePanel.java
public void update() {
// check collision with right wall if heading right
if (droid.getSpeed().getxDirection() == Speed.DIRECTION_RIGHT
&& droid.getX() + droid.getBitmap().getWidth() / 2 >= getWidth()) {
droid.getSpeed().toggleXDirection();
}
// check collision with left wall if heading left
if (droid.getSpeed().getxDirection() == Speed.DIRECTION_LEFT
&& droid.getX() - droid.getBitmap().getWidth() / 2 <= 0) {
droid.getSpeed().toggleXDirection();
}
// check collision with bottom wall if heading down
if (droid.getSpeed().getyDirection() == Speed.DIRECTION_DOWN
&& droid.getY() + droid.getBitmap().getHeight() / 2 >= getHeight()) {
droid.getSpeed().toggleYDirection();
}
// check collision with top wall if heading up
if (droid.getSpeed().getyDirection() == Speed.DIRECTION_UP
&& droid.getY() - droid.getBitmap().getHeight() / 2 <= 0) {
droid.getSpeed().toggleYDirection();
}
// Update the lone droid
droid.update();
}
getWidth()和getHeight()返回视图的宽度和高度。 面板是一个视图,还记得吗?
Droid.java文件的update()方法:
public void update() {
if (!touched) {
x += (speed.getXv() * speed.getxDirection());
y += (speed.getYv() * speed.getyDirection());
}
}
我还在MainThread.java中更改了渲染的名称,因此现在改为onDraw来渲染。 只是我更喜欢它,因为它遵循更新->渲染命名。
运行该应用程序,您应该会看到类似以下的屏幕,其中机器人以45度角移动并在碰到墙壁时从墙壁弹起。 您也可以拖动机器人。要退出该应用程序,请单击(触摸)屏幕下部。
|
移动机器人 |
在此处下载完整的源代码和eclipse项目。
参考:来自我们的JCG合作伙伴Tamas Jano的“使用Android在屏幕上移动图像”,来自“ Against The Grain ”博客。
- Android游戏开发教程简介
- Android游戏开发–游戏创意
- Android游戏开发–创建项目
- Android游戏开发–基本游戏架构
- Android游戏开发–基本游戏循环
- Android游戏开发–使用Android显示图像
- Android游戏开发–游戏循环
- Android游戏开发–测量FPS
- Android游戏开发–雪碧动画
- Android游戏开发–粒子爆炸
- Android游戏开发–设计游戏实体–策略模式
- Android游戏开发–使用位图字体
- Android游戏开发–从Canvas切换到OpenGL ES
- Android游戏开发–使用OpenGL ES显示图形元素(原语)
- Android游戏开发– OpenGL纹理映射
- Android游戏开发–设计游戏实体–状态模式
- Android游戏文章系列
翻译自: https://www.javacodegeeks.com/2011/07/android-game-development-moving-images.html
android