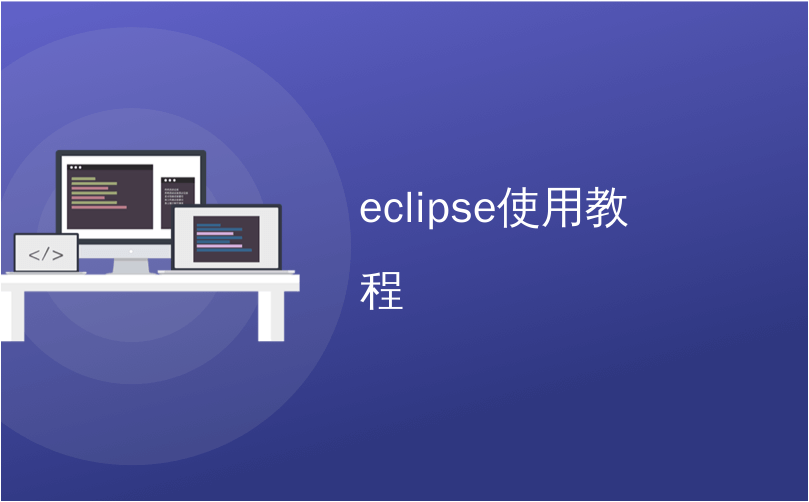
eclipse使用教程
Eclipse Collections是一个开放源代码Java Collections框架。 在此博客中,我将演示该框架的五个鲜为人知的功能。 我在去年的Java Advent Calendar中发布了类似的博客。 请参阅博客末尾的资源以获取有关该框架的更多信息。
1. countBy()
:当您要查找特定对象的计数时,可以使用countBy()
API来获取Bag。 Bag的目的是维护对象到计数的映射。 袋可用于查询O(1)
时间中的项目计数。 Bag还提供了其他有用的API,有助于计数。 在此博客中了解有关Bag数据结构的更多信息。
@Test
public void countBy()
{
MutableList<String> strings =
Lists.mutable.with( "A" , "B" , "C" , "A" , "B" , "A" );
Bag<String> stringToCount = strings.countBy(each -> each);
assertEquals( 3 , stringToCount.occurrencesOf( "A" ));
assertEquals( 2 , stringToCount.occurrencesOf( "B" ));
assertEquals( 1 , stringToCount.occurrencesOf( "C" ));
assertEquals( 3 , stringToCount.sizeDistinct());
assertEquals( 6 , stringToCount.size());
}
2. reject()
:当您要选择不满足谓词的元素时,可以使用reject()
API。 提供此API是为了增强可读性并使开发人员直观。 您可以使用reject()
代替使用select()
和取反布尔条件。 本质上,当使用reject()
时,将选择所有对于布尔条件不返回true
的元素。 reject(BooleanCondition)
的输出与通过执行select(!someBooleanCondition)
得到的输出相同。
@Test
public void reject()
{
MutableList<Integer> numbers =
Lists.mutable.with( 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 );
MutableList<Integer> odds = numbers.reject(num -> num % 2 == 0 );
// reject pattern used to find odd numbers.
// Notice there is no negation in the predicate.
assertEquals(Lists.mutable.with( 1 , 3 , 5 , 7 , 9 ), odds);
MutableList<Integer> oddsUsingSelect =
numbers.select(num -> num % 2 != 0 );
assertEquals(odds, oddsUsingSelect);
}
3. makeString()
:当您想要RichIterable
可配置字符串表示形式时,可以使用makeString()
。 如果使用不带定界符的makeString()
,则使用默认的定界符"comma space" ( ", " )
。 如果需要特定的定界符,可以将其传递给makeString()
,输出字符串将具有字符串表示形式,其中每个元素都由定界符分隔。 如果Iterable
的大小为1,则不使用定界符。
@Test
public void makeString()
{
MutableList<Integer> nums = Lists.mutable.with( 1 , 2 , 3 );
assertEquals( "[1, 2, 3]" , nums.toString());
// Notice the difference: toString() vs makeString().
// the ", " delimiter is used by default
assertEquals( "1, 2, 3" , nums.makeString());
// Delimiter of choice can be passed
assertEquals( "1;2;3" , nums.makeString( ";" ));
MutableList<Integer> singleElement = Lists.mutable.with( 1 );
// Delimiter is not used for size = 1
assertEquals( "1" , singleElement.makeString());
assertEquals( "1" , singleElement.makeString( ";" ));
}
4. zip()
:要将两个OrderedIterable
缝合在一起时,可以使用zip()
。 zip()
API在两个OrderedIterable
上进行操作并将它们缝合在一起,这样您就获得了Pair
of elements的OrderedIterable
。 在Pair
,第一Pair
是从第一元件OrderedIterable
和第二Pair
从第二元件OrderedIterable
。 如果OrderedIterable
的大小不同, OrderedIterable
忽略较长OrderedIterable
中多余的元素。 zip()
的输出是一个OrderedIterable
,其大小与较小的OrderedIterable
相同。
@Test
public void zip()
{
MutableList<Integer> nums = Lists.mutable.with( 1 , 2 , 3 );
MutableList<String> strings =
Lists.mutable.with( "A" , "B" , "C" );
assertEquals(
Lists.mutable.with(Tuples.pair( 1 , "A" ),
Tuples.pair( 2 , "B" ),
Tuples.pair( 3 , "C" )),
nums.zip(strings));
assertEquals(
Lists.mutable.with(Tuples.pair( "A" , 1 ),
Tuples.pair( "B" , 2 ),
Tuples.pair( "C" , 3 )),
strings.zip(nums));
MutableList<Integer> numsSmallerSize =
Lists.mutable.with( 1 );
assertEquals(
Lists.mutable.with(Tuples.pair( 1 , "A" )),
numsSmallerSize.zip(strings));
assertEquals(
Lists.mutable.with(Tuples.pair( "A" , 1 )),
strings.zip(numsSmallerSize));
MutableList<String> stringsSmallerSize =
Lists.mutable.with( "A" , "B" );
assertEquals(
Lists.mutable.with(Tuples.pair( 1 , "A" ),
Tuples.pair( 2 , "B" )),
nums.zip(stringsSmallerSize));
assertEquals(
Lists.mutable.with(Tuples.pair( "A" , 1 ),
Tuples.pair( "B" , 2 )),
stringsSmallerSize.zip(nums));
}
5. OrderedIterable
corresponds()
:当您要根据Predicate
查找两个OrderedIterable
的所有元素是否相等时,可以使用corresponds()
API。 的corresponds()
API由第一检查操作如果两个OrderedIterable
■找相同的尺寸,如果它们具有那么相同的大小对应的两个元素OrderedIterable
S使用的被评估Predicate
传递给corresponds()
如果OrderedIterable
s的大小相等,并且Predicate
对所有元素返回true
,则corresponds()
elements corresponds()
返回true
。 如果OrderedIterable
s的大小不相等,或者Predicate
对任何元素返回false
,则corresponds()
的OrderedIterable
corresponds()
返回false
。
@Test
public void corresponds()
{
MutableList<Integer> lhs1 = Lists.mutable.with( 1 , 2 , 3 );
MutableList<Integer> rhs1 = Lists.mutable.with( 1 , 2 , 3 );
assertTrue(lhs1.corresponds(rhs1, Integer::equals));
MutableList<Integer> lhs2 = Lists.mutable.with( 1 , 2 , 3 );
MutableList<Integer> rhs2 = Lists.mutable.with( 2 , 4 , 6 );
assertTrue(
lhs2.corresponds(rhs2,
(lhs, rhs) -> rhs == 2 * lhs));
assertFalse(
lhs2.corresponds(rhs2,
(lhs, rhs) -> rhs == lhs * lhs));
assertFalse(lhs2.corresponds(rhs2, Integer::equals));
MutableList<Integer> lhs3 = Lists.mutable.with( 1 , 2 );
MutableList<Integer> rhs3 = Lists.mutable.with( 1 , 2 , 3 );
assertFalse(lhs3.corresponds(rhs3, Integer::equals));
}
Eclipse Collections资源:Eclipse Collections附带了List , Set和Map的自己的实现。 它还具有其他数据结构,例如Multimap , Bag和整个Primitive Collections层次结构。 我们的每个集合都有一个流利且丰富的API,可用于通常需要的迭代模式。
翻译自: https://www.javacodegeeks.com/2019/12/hidden-treasures-of-eclipse-collections-2019-edition.html
eclipse使用教程