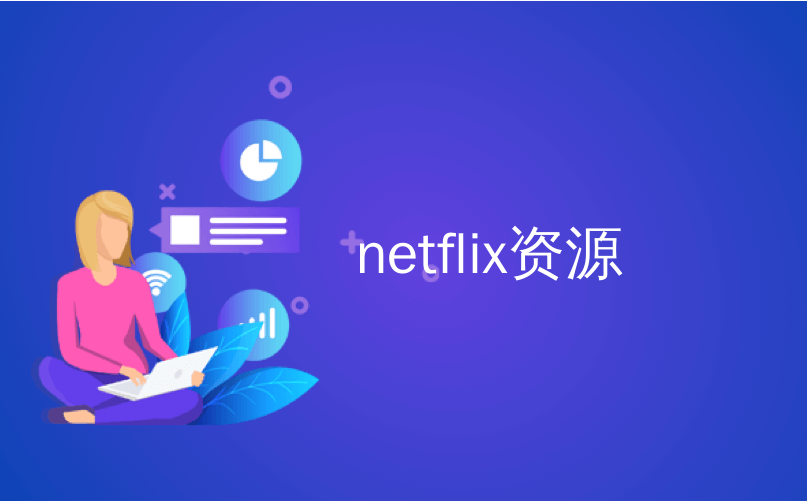
netflix资源
最近几天,我一直在与Netflix Governator合作,并尝试使用Governator尝试一个小样本,以将其与Spring Framework的依赖项注入功能集进行比较。 以下内容并不全面,我将在接下来的系列文章中对此进行扩展。
因此,对于没有经验的人来说,Governorator是Google Guice的扩展,它通过一些类似于Spring的功能对其进行了增强,引用Governator网站:
类路径扫描和自动绑定,生命周期管理,配置到字段映射,字段验证和并行化的对象预热。
在这里,我将演示两个功能,类路径扫描和自动绑定。
基本依赖注入
考虑一个BlogService,具体取决于BlogDao:
public class DefaultBlogService implements BlogService {
private final BlogDao blogDao;
public DefaultBlogService(BlogDao blogDao) {
this.blogDao = blogDao;
}
@Override
public BlogEntry get(long id) {
return this.blogDao.findById(id);
}
}
如果我使用Spring定义这两个组件之间的依赖关系,则将使用以下配置:
package sample.spring;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import sample.dao.BlogDao;
import sample.service.BlogService;
@Configuration
public class SampleConfig {
@Bean
public BlogDao blogDao() {
return new DefaultBlogDao();
}
@Bean
public BlogService blogService() {
return new DefaultBlogService(blogDao());
}
}
在Spring中,依赖项配置是在带有@Configuration注释的类中指定的。 用@Bean注释的方法返回组件,请注意如何通过blogService方法中的构造函数注入来注入blogDao。
此配置的单元测试如下:
package sample.spring;
import org.junit.Test;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import sample.service.BlogService;
import static org.hamcrest.MatcherAssert.*;
import static org.hamcrest.Matchers.*;
public class SampleSpringExplicitTest {
@Test
public void testSpringInjection() {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext();
context.register(SampleConfig.class);
context.refresh();
BlogService blogService = context.getBean(BlogService.class);
assertThat(blogService.get(1l), is(notNullValue()));
context.close();
}
}
请注意,Spring为单元测试提供了良好的支持,更好的测试如下:
package sample.spring;
package sample.spring;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import sample.service.BlogService;
import static org.hamcrest.MatcherAssert.*;
import static org.hamcrest.Matchers.*;
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration
public class SampleSpringAutowiredTest {
@Autowired
private BlogService blogService;
@Test
public void testSpringInjection() {
assertThat(blogService.get(1l), is(notNullValue()));
}
@Configuration
@ComponentScan("sample.spring")
public static class SpringConig {
}
}
这是基本的依赖项注入,因此不需要指定这种依赖关系,Governice本身就不需要管治者,这就是使用Guice Modules时配置的外观:
package sample.guice;
import com.google.inject.AbstractModule;
import sample.dao.BlogDao;
import sample.service.BlogService;
public class SampleModule extends AbstractModule{
@Override
protected void configure() {
bind(BlogDao.class).to(DefaultBlogDao.class);
bind(BlogService.class).to(DefaultBlogService.class);
}
}
此配置的单元测试如下:
package sample.guice;
import com.google.inject.Guice;
import com.google.inject.Injector;
import org.junit.Test;
import sample.service.BlogService;
import static org.hamcrest.Matchers.*;
import static org.hamcrest.MatcherAssert.*;
public class SampleModuleTest {
@Test
public void testExampleBeanInjection() {
Injector injector = Guice.createInjector(new SampleModule());
BlogService blogService = injector.getInstance(BlogService.class);
assertThat(blogService.get(1l), is(notNullValue()));
}
}
类路径扫描和自动绑定
类路径扫描是一种通过在类路径中查找标记来检测组件的方法。 使用Spring的样本应该澄清这一点:
@Repository
public class DefaultBlogDao implements BlogDao {
....
}
@Service
public class DefaultBlogService implements BlogService {
private final BlogDao blogDao;
@Autowired
public DefaultBlogService(BlogDao blogDao) {
this.blogDao = blogDao;
}
...
}
在这里,注释@ Service,@ Repository用作标记,以指示它们是组件,并且依赖项由DefaultBlogService的构造函数上的@Autowired注释指定。
鉴于现在已经简化了配置,我们只需要提供应该为此类带注释的组件进行扫描的软件包名称,这就是完整测试的样子:
package sample.spring;
...
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration
public class SampleSpringAutowiredTest {
@Autowired
private BlogService blogService;
@Test
public void testSpringInjection() {
assertThat(blogService.get(1l), is(notNullValue()));
}
@Configuration
@ComponentScan("sample.spring")
public static class SpringConig {}
}
总督提供了类似的支持:
@AutoBindSingleton(baseClass = BlogDao.class)
public class DefaultBlogDao implements BlogDao {
....
}
@AutoBindSingleton(baseClass = BlogService.class)
public class DefaultBlogService implements BlogService {
private final BlogDao blogDao;
@Inject
public DefaultBlogService(BlogDao blogDao) {
this.blogDao = blogDao;
}
....
}
在这里,@ AutoBindSingleton批注被用作标记批注来定义guice绑定,考虑到以下是对类路径扫描的测试:
package sample.gov;
import com.google.inject.Injector;
import com.netflix.governator.guice.LifecycleInjector;
import com.netflix.governator.lifecycle.LifecycleManager;
import org.junit.Test;
import sample.service.BlogService;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.is;
import static org.hamcrest.Matchers.notNullValue;
public class SampleWithGovernatorTest {
@Test
public void testExampleBeanInjection() throws Exception {
Injector injector = LifecycleInjector
.builder()
.withModuleClass(SampleModule.class)
.usingBasePackages("sample.gov")
.build()
.createInjector();
LifecycleManager manager = injector.getInstance(LifecycleManager.class);
manager.start();
BlogService blogService = injector.getInstance(BlogService.class);
assertThat(blogService.get(1l), is(notNullValue()));
}
}
查看如何使用Governator的LifecycleInjector组件指定要扫描的程序包,这将自动检测这些组件并将它们连接在一起。
只是为了包装类路径扫描和自动绑定功能,像Spring这样的Governor提供了对junit测试的支持,以下是更好的测试:
package sample.gov;
import com.google.inject.Injector;
import com.netflix.governator.guice.LifecycleTester;
import org.junit.Rule;
import org.junit.Test;
import sample.service.BlogService;
import static org.hamcrest.MatcherAssert.*;
import static org.hamcrest.Matchers.*;
public class SampleWithGovernatorJunitSupportTest {
@Rule
public LifecycleTester tester = new LifecycleTester();
@Test
public void testExampleBeanInjection() throws Exception {
tester.start();
Injector injector = tester
.builder()
.usingBasePackages("sample.gov")
.build()
.createInjector();
BlogService blogService = injector.getInstance(BlogService.class);
assertThat(blogService.get(1l), is(notNullValue()));
}
}
结论
如果您有兴趣进一步探索这个问题,那么我在此github项目中有一个示例,随着我对Governator的更多了解,我将扩展这个项目。
翻译自: https://www.javacodegeeks.com/2015/01/learning-netflix-governator-part-1.html
netflix资源