在本教程系列的上一部分中,您学习了如何实现编辑博客文章详细信息的功能。
在这一部分中,您将实现删除现有博客帖子以及实现用户注销功能的功能。
入门
让我们从克隆本系列最后一部分的源代码开始。
git clone https://github.com/royagasthyan/AngularBlogApp-EditUpdate DeletePost
导航到项目目录并安装所需的依赖项。
cd DeletePost/client
npm install
cd DeletePost/server
npm install
安装依赖项后,请重新启动客户端和服务器应用程序。
cd DeletePost/client
npm start
cd DeletePost/server
node app.js
将浏览器指向http:// localhost:4200 ,您将运行该应用程序。
添加删除确认
您已经将删除图标添加到列出的博客文章中。 当用户单击与任何博客文章对应的删除图标时,您需要显示一个删除确认弹出窗口。 如果用户确认删除过程,则仅需要删除博客帖子。
让我们开始在用户单击删除按钮时添加模式弹出确认。 将以下模式弹出式代码添加到show-post.component.html
文件。
<div class="modal fade" id="deleteModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Delete Post</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
Are you sure ?
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Cancel</button>
<button type="button" class="btn btn-primary">Delete</button>
</div>
</div>
</div>
</div>
修改删除图标以包括data-target
属性,如下所示:
<i data-toggle="modal" data-target="#deleteModal" title="Delete" class="fas fa-trash-alt" aria-hidden="true"></i>
保存以上更改,然后重新启动客户端服务器。 登录该应用程序,然后单击与任何博客文章相对应的删除图标,您将弹出确认模式。
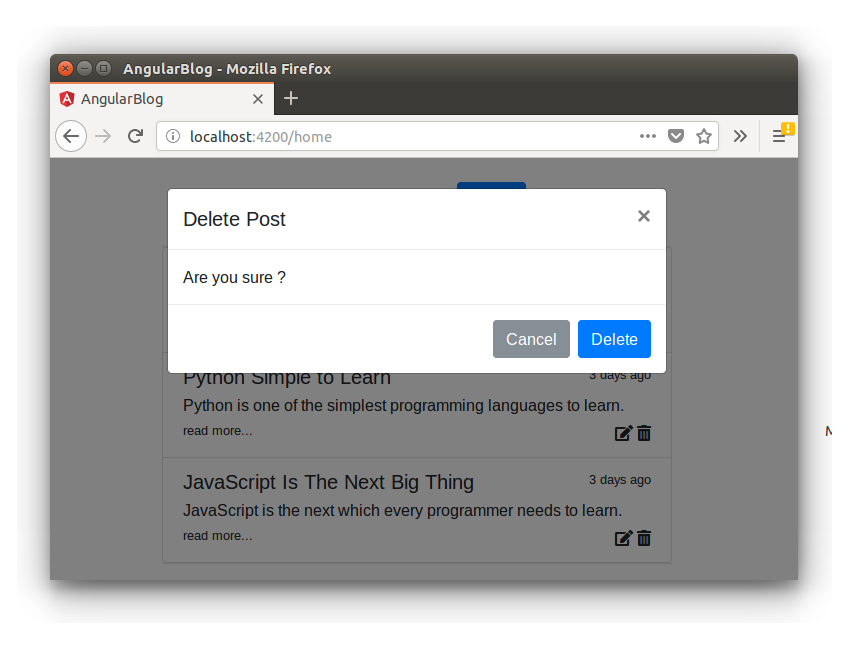
创建删除博客文章API
让我们创建一个REST API端点以删除博客文章。 在server/app.js
文件中,创建一个REST API终结点,以根据博客文章id
来处理博客文章删除。 REST API端点的外观如下:
app.post('/api/post/deletePost', (req, res) => {
})
首先使用Mongoose
客户端连接到MongoDB数据库。
mongoose.connect(url, { useMongoClient: true }, function(err){
// connection established
});
您将使用findByIdAndRemove
方法使用id
查找博客帖子并将其删除。 成功删除博客帖子后,您将返回status
作为响应。 REST API端点的外观如下:
app.post('/api/post/deletePost', (req, res) => {
mongoose.connect(url, { useMongoClient: true }, function(err){
if(err) throw err;
Post.findByIdAndRemove(req.body.id,
(err, doc) => {
if(err) throw err;
return res.status(200).json({
status: 'success',
data: doc
})
})
});
})
调用Delete API
用户单击删除图标时,您需要将帖子详细信息保留在变量中。 如果用户在确认后继续使用delete选项,则将调用delete REST API。
在删除按钮上添加一个名为setDelete
的方法,单击show-post.component.html
。 外观如下:
<i (click)="setDelete(post)" data-toggle="modal" data-target="#deleteModal" title="Delete" class="fas fa-trash-alt" aria-hidden="true"></i>
在show-post.component.ts
文件中,定义一个名为post_to_delete
的变量。
在show-post.component.ts
定义称为setDelete
的方法,以保留要删除的帖子详细信息。
setDelete(post: Post){
this.post_to_delete = post;
}
当用户点击取消弹出窗口的按钮,你需要调用一个名为方法unsetDelete
设置post_to_delete
为null。 外观如下:
unsetDelete(){
this.post_to_delete = null;
}
这是show-post.component.html
的“ Cancel
按钮HTML代码的外观:
<button #closeBtn (click)="unsetDelete()" type="button" class="btn btn-secondary" data-dismiss="modal">Cancel</button>
现在,让我们在show-post.service.ts
文件中定义称为deletePost
的服务方法。 外观如下:
deletePost(id){
return this.http.post('/api/post/deletePost',{id : id})
}
拨叫服务方法ShowPostComponent
,定义了一个名为方法deletePost
将订阅deletePost
从方法ShowPostService
。 这里是如何的deletePost
从方法ShowPostComponent
外观:
deletePost(){
this.showPostService.deletePost(this.post_to_delete._id).subscribe(res => {
this.getAllPost();
})
}
删除帖子后,您需要刷新帖子列表,因此需要调用getAllPost
方法。 一旦删除成功,您还需要关闭弹出窗口。
首先,在show-post.component.ts
文件中导入对ViewChild
和ElementRef
的引用。
import { Component, OnInit, ViewChild, ElementRef } from '@angular/core';
定义一个变量closeBtn
以创建对弹出关闭按钮的引用。
@ViewChild('closeBtn') closeBtn: ElementRef;
现在,删除呼叫成功后,您需要关闭删除确认弹出窗口。
这是修改后的deletePost
方法的外观:
deletePost(){
this.showPostService.deletePost(this.post_to_delete._id).subscribe(res => {
this.getAllPost();
this.closeBtn.nativeElement.click();
})
}
这是show-post.component.ts
文件的外观:
import { Component, OnInit, ViewChild, ElementRef } from '@angular/core';
import { ShowPostService } from './show-post.service';
import { Post } from '../models/post.model';
import { CommonService, } from '../service/common.service';
@Component({
selector: 'app-show-post',
templateUrl: './show-post.component.html',
styleUrls: ['./show-post.component.css'],
providers: [ ShowPostService ]
})
export class ShowPostComponent implements OnInit {
@ViewChild('closeBtn') closeBtn: ElementRef;
public posts : any [];
public post_to_delete;
constructor(private showPostService: ShowPostService, private commonService: CommonService) {
}
ngOnInit(){
this.getAllPost();
this.commonService.postAdded_Observable.subscribe(res => {
this.getAllPost();
});
}
setDelete(post: Post){
this.post_to_delete = post;
}
unsetDelete(){
this.post_to_delete = null;
}
getAllPost(){
this.showPostService.getAllPost().subscribe(result => {
console.log('result is ', result);
this.posts = result['data'];
});
}
editPost(post: Post){
this.commonService.setPostToEdit(post);
}
deletePost(){
this.showPostService.deletePost(this.post_to_delete._id).subscribe(res => {
this.getAllPost();
this.closeBtn.nativeElement.click();
})
}
}
保存以上更改,然后重新启动客户端和服务器应用程序。 登录该应用程序,然后单击与任何博客文章对应的删除图标。 您将弹出一个确认框。 确认删除博客文章,博客文章将被删除,博客文章列表将被更新。
登录期间处理用户会话
用户登录应用程序时,您将登录的用户名保留在localstorage
。 修改LoginComponent
内的validateLogin
方法,以将登录的用户名存储在localstorage
。
验证API调用的结果后,添加以下代码以存储登录的用户名。
localStorage.setItem('loggedInUser', this.user.username);
这是validateLogin
方法的外观:
validateLogin() {
if(this.user.username && this.user.password) {
this.loginService.validateLogin(this.user).subscribe(result => {
if(result['status'] === 'success') {
localStorage.setItem('loggedInUser', this.user.username);
this.router.navigate(['/home']);
} else {
alert('Wrong username password');
}
}, error => {
console.log('error is ', error);
});
} else {
alert('enter user name and password');
}
}
现在,在home.component.html
文件中,将一个名为logout
的方法添加到Log out按钮。
<button (click)="logout()" type="button" class="btn btn-link">
Logout
</button>
在home.component.ts
文件中,创建一个名为logout
的方法。 在logout
方法内部,您需要清除loggedInUser
的本地存储。 该方法的外观如下:
logout(){
localStorage.removeItem('loggedInUser');
this.router.navigate(['/']);
}
在HomeComponent
的构造方法中,您需要添加对loggedInUser
本地存储键的检查。 如果未找到,则需要重定向到登录页面。 这是home.component.ts
文件的外观:
import { Component, ViewChild, ElementRef } from '@angular/core';
import { CommonService } from '../service/common.service';
import { Router } from '@angular/router';
@Component({
selector: 'app-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.css']
})
export class HomeComponent {
@ViewChild('addPost') addBtn: ElementRef;
constructor(private commonService: CommonService, private router: Router){
if(!localStorage.getItem('loggedInUser')){
this.router.navigate(['/']);
}
this.commonService.postEdit_Observable.subscribe(res => {
this.addBtn.nativeElement.click();
});
}
logout(){
localStorage.removeItem('loggedInUser');
this.router.navigate(['/']);
}
}
保存以上更改,然后重新启动客户端服务器。 尝试通过在浏览器窗口中加载URL http:// localhost:4200 / home来访问主页。 您将被重定向到登录页面。
登录该应用程序,然后单击注销按钮。 您将被注销并重定向到登录页面。
包起来
在本系列教程的这一部分中,您学习了如何通过在博客文章列表中添加图标来实现博客文章删除。 您还创建了一个REST API,用于使用Mongoose
客户端从MongoDB数据库中删除博客文章详细信息。
您仅实现了博客应用程序的非常基本的功能,并且可以对该应用程序进行进一步开发以使其包含更多功能。
您如何学习使用Angular和MongoDB创建博客应用程序的经验? 请在下面的评论中告诉我们您的想法和建议。
该教程的源代码可在GitHub上获得 。
最后,请记住,JavaScript是网页的语言。 它并非没有学习曲线,但如果您正在寻找其他资源来学习或在工作中使用,请查看我们在Envato Market中可以找到的资源 。