本教程将向您介绍Web API,并教您如何使用请求Python库来获取和更新Web API中的信息。 您还将作为工作示例学习如何与Twitter API进行交互。
Web API简介
API(应用程序编程接口)是用于构建可被各种客户端使用的HTTP服务的框架。 Web API使用HTTP协议来处理客户端和Web服务器之间的请求。
使开发人员能够集成和使用其基础架构的一些最常见的API包括:
- Google API
- Twitter API
- 亚马逊API
- Facebook API
与其他静态数据源相比,使用API的最重要原因之一是因为它是实时的。 例如,我们将要使用的Twitter API将从社交网络获取实时数据。
另一个优点是数据一直在变化,因此,如果您要定期下载数据,那将很耗时。
使用请求库
为了使用API,您将需要安装请求Python库。 Requests是Python中的HTTP库,使您可以使用Python发送HTTP请求。
安装要求
在您的终端中,键入:
pip install requests
要检查安装是否成功,请在您的Python解释器或终端中发出以下命令:
import requests
如果没有错误,则说明安装成功。
如何从Web API获取信息
GET方法用于从Web服务器获取信息。 让我们看看如何发出GET请求以获取GitHub的公共时间表。
我们使用变量req
来存储来自请求的响应。
import requests
req = requests.get('https://github.com/timeline.json')
现在,我们已经向GitHub时间轴发出了请求,让我们获取响应中包含的编码和内容。
import requests
req = requests.get('https://github.com/timeline.json')
req.text
u'{"message":"Hello there, wayfaring stranger. If you\u2019re reading this then you probably didn\u2019t see our blog post a couple of years back announcing that this API would go away: https://git.io/17AROg Fear not, you should be able to get what you need from the shiny new Events API instead.","documentation_url":"https://developer.github.com/v3/activity/events/#list-public-events"}
import requests
req = requests.get('https://github.com/timeline.json')
req.encoding
'utf-8'
请求具有内置的JSON解码,可用于获取JSON格式的请求的响应。
import requests
import json
req = requests.get('https://github.com/timeline.json')
req.json()
{u'documentation_url': u'https://developer.github.com/v3/activity/events/#list-public-events', u'message': u'Hello there, wayfaring stranger. If you\u2019re reading this then you probably didn\u2019t see our blog post a couple of years back announcing that this API would go away: http://git.io/17AROg Fear not, you should be able to get what you need from the shiny new Events API instead.'}
如何在Web API上创建和更新信息
POST和PUT方法都用于创建和更新数据。 尽管有相似之处,但需要注意的是,如果提交了两个相同的项目,使用POST请求更新数据将在数据存储区中导致两个条目。
创建数据(POST请求):
r = requests.post('http://127.0.0.1/api/v1/add_item', data = {'task':'Shopping'})
更新数据(PUT请求):
r = requests.put('http://127.0.0.1/api/v1/add_item', data = {'task':'Shopping at 2'})
使用Twitter REST API
在本部分中,您将学习如何获取Twitter API凭据,对Twitter API进行身份验证以及如何使用Python与Twitter API进行交互。
您还可以从公共Twitter帐户检索信息,例如推文,关注者等。
使用Twitter进行身份验证
我们需要先与Twitter API进行身份验证,然后才能进行交互。 为此,请按照下列步骤操作:
- 转到Twitter Apps页面 。
- 单击“ 创建新应用” (您需要登录Twitter才能访问此页面)。 如果您没有Twitter帐户,请创建一个 。
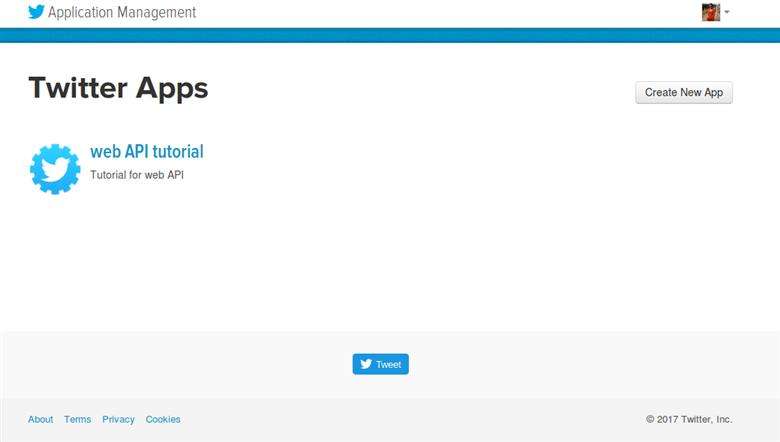
3.为您的应用和网站占位符创建名称和描述。
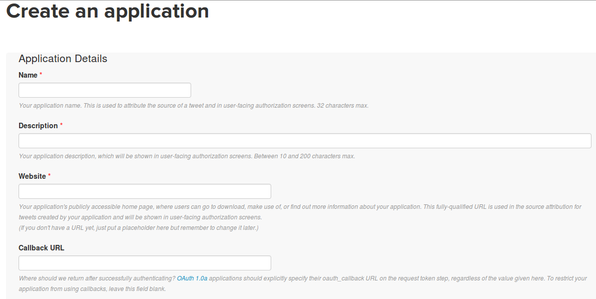
4.找到“ 密钥和访问令牌”选项卡,然后创建您的访问令牌。
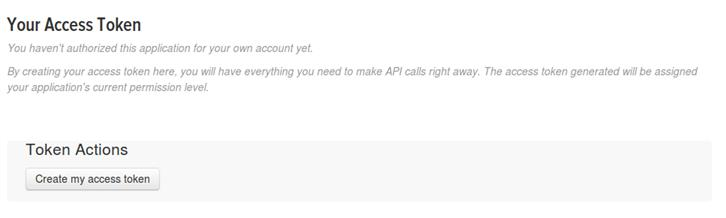
5.您需要记下Access token
和Access Token secret
因为在身份验证过程中将需要它们。
6.您还需要注意Consumer Key
和Consumer Secret
。
我们可以使用一些库来访问Twitter API,但是在本教程中,我们将使用python-twitter库。
安装python-twitter
要安装python-twitter,请使用:
$ pip install python-twitter
Twitter API通过twitter.Api
类公开,因此让我们通过传递令牌和秘密密钥来创建该类:
import twitter
api = twitter.Api(consumer_key=[consumer key],
consumer_secret=[consumer secret],
access_token_key=[access token],
access_token_secret=[access token secret])
替换上面的凭据,并确保将其括在引号中,例如,consumer_key ='xxxxxxxxxx',...)
查询Twitter
与Twitter API交互的方法很多,包括:
>>> api.PostUpdates(status)
>>> api.PostDirectMessage(user, text)
>>> api.GetUser(user)
>>> api.GetReplies()
>>> api.GetUserTimeline(user)
>>> api.GetHomeTimeline()
>>> api.GetStatus(status_id)
>>> api.DestroyStatus(status_id)
>>> api.GetFriends(user)
>>> api.GetFollowers()
为了从Twitter获取数据,我们将在上面创建的api
对象的帮助下进行API调用。
我们将执行以下操作:
- 创建一个
user
变量,并将其设置为等于有效的Twitter句柄(用户名)。
在
api
对象上调用GetUserTimeline()
方法,并传递以下参数。
- 有效的Twitter句柄
- 您要检索的推文数(
count
) - 一个标记来排除转发(使用
include_rts = false
来完成)
让我们从Envato Tuts + Code时间轴获取最新的推文,不包括推文。
import twitter
api = twitter.Api(consumer_key="xxxxxxxxxxxx", consumer_secret="xxxxxxxxxxxxxx",
access_token_key="314746354-xxxxx", access_token_secret="xxxxxx")
user = "@TutsPlusCode"
statuses = api.GetUserTimeline(
screen_name=user, count=30, include_rts=False)
for s in statuses:
print s.text
GetUserTimeline()
方法将返回最新的30条推文的列表,因此我们遍历该列表并从每条推文中打印最重要的信息(内容)。
Here are 6 things that make Yarn the best #JavaScript package manager around. https://t.co/N4vzIJmSJi
Find out more about bar charts with part 3 of this series on creating interactive charts using Plotly.js. https://t.co/lyKMxSsicJ
See what's new with Git support in Xcode 9. https://t.co/7gGu0PV1rV
Here's how to create digital signatures with Swift. https://t.co/kCYYjShJkW
In this quick tip, discover how to use Atom as a Git GUI. https://t.co/8rfQyo42xM
Take a look at these 12 useful WordPress plugins for page layouts. https://t.co/T57QUjEpu5
Learn more about line charts in part 2 of our series on creating interactive charts using Plotly.js. https://t.co/nx51wOzSkF
Grab some great freebies with our special 10th birthday offer, https://t.co/AcIGTiC2re
In this latest in our series on coding a real-time app with NativeScript: Push notifications. https://t.co/qESFVGVF4L
Get started with end-to-end testing in Angular using Protractor. https://t.co/TWhQZe7ihE
Learn how to build a to-do API with Node, Express, and MongoDB. https://t.co/R4DvRYiM90
What is the Android activity lifecyle? https://t.co/VUHsucaC1X
Learn all about object-oriented programming with JavaScript. https://t.co/bI7ypANOx3
Have some fun with functions in this latest in our series on Kotlin. https://t.co/r2f2TzA5lM
Here's how to make your JavaScript code robust with Flow. https://t.co/rcdjybKL8L
Build your own to-do API with Node and Restify. https://t.co/gQeTSZ6C5k
Here's how to put your view controllers on a diet with MVVM. https://t.co/oJqNungt1O
Learn how to submit your new iOS app to the App Store. https://t.co/JQwsKovcaI
This guide is the perfect place to build your skills and start writing plugins in Go. https://t.co/68X5lLSNHp
Take a look at how to test components in Angular using Jasmine. https://t.co/V5OTNZgDkR
Learn to build your first #WordPress plugin in this awesome new course. https://t.co/695C6U6D7V
In this latest in our Android architecture components series: LiveData. https://t.co/gleDFbqeAi
Take a deep dive into the Go type system. https://t.co/AUM7ZyanRO
Take a look at serverless logic with realm functions. https://t.co/aYhfeMgAZc
Part 4 of React crash course for beginners is out! https://t.co/aG5NEa6yG9
Learn about social login and Firebase in this new addition to the series. https://t.co/oL5z0krQD3
Creating a blogging app using React part 6 is out! Tags https://t.co/OzUaPQEX8E
What is GenServer and why should you care? https://t.co/EmQeTBggUK
Check out part 3 in React crash course for beginners series. https://t.co/dflLCUqncO
Learn about packages and basic functions in this addition to our Kotlin from scratch series. https://t.co/JAo2ckSgZS
要检索关注者,我们使用GetFriends()
方法。
import twitter
api = twitter.Api(consumer_key="ftFL8G4yzQXUVzbUCdxGwKSjJ", consumer_secret="KxGwBe6GlgSYyC7PioIVuZ5tFAsZs7q1rseEYCOnTDIjulT0mZ",
access_token_key="314746354-Ucq36TRDnfGAxpOVtnK1qZxMfRKzFHFhyRqzNpTx",
access_token_secret="7wZ1qHS0qycy0aNjoMDpKhcfzuLm6uAbhB2LilxZzST8w")
user = "@TutsPlusCode"
friends = api.GetFriends(screen_name=user)
for friend in friends:
print friend.name
输出量
Derek Herman
Cyan Ta'eed
Dropbox
Stoyan Stefanov
The JavaScript Ninja
Dan Wellman
Brenley Dueck
Dave Ward
Packt
Karl Swedberg
Envato Tuts+ Web
Dev_Tips
Vahid Ta'eed
Jarel Remick
Envato Dev. Team
🖥 Drew Douglass 📈
Cameron Moll
SitePoint
John Resig
Skellie
Chris Coyier
Envato Tuts+
Envato Tuts+ Design
Collis
结论
Twitter的API可在数据分析中广泛使用。 它也可以用于复杂的大数据问题和身份验证应用程序。 在Twitter开发人员站点上了解有关Twitter API的更多信息。
另外,不要犹豫,看看我们在市场上有哪些出售和研究的产品 ,也不要犹豫,使用下面的提要来问任何问题并提供宝贵的反馈。
翻译自: https://code.tutsplus.com/articles/how-to-use-restful-web-apis-in-python--cms-29493