Python is a feature-rich programming language that provides a lot of string or text-related functions. String manipulation provides different operations where Substring
operation is one of the most important.
Python是一种功能丰富的编程语言,可提供许多与字符串或文本相关的功能。 字符串操作提供了不同的操作,其中Substring
操作是最重要的操作之一。
什么是子串? (What Is Substring?)
Substring is an operation that will get some part of the specified string. Substring operations can be done in different ways and methods. For example “I love poftut.com” provides the substring “poftut.com” and “love” etc.
子字符串是将获取指定字符串的一部分的操作。 子串操作可以通过不同的方式和方法来完成。 例如,“ I love poftut.com”提供了子字符串“ poftut.com”和“ love”等。
字符串类型内置切片 (String Type Builtin Slicing)
The most popular, easy and practical way to get substring is using the String data type slicing operator. Strings are like character arrays and every character has an index number. So providing these index number some part or the string or a substring can be restrived from a string.
获取子字符串的最流行,简便和实用的方法是使用String数据类型切片运算符。 字符串就像字符数组,每个字符都有一个索引号。 因此,提供这些索引号可以从字符串中获取部分或字符串或子字符串。
SUBSTRING = STRING[START_INDEX:END_INDEX]
STRING
is the text or string which is the source of the SUBSTRING and contains characters.
STRING
是文本或字符串,是SUBSTRING的来源,包含字符。
START_INDEX
is the substring index start number where specifies the SUBSTRING first character. START_INDEX is optional and if not provided 0 is assumed.
START_INDEX
是子字符串索引的起始编号,其中指定SUBSTRING第一个字符。 START_INDEX是可选的,如果未提供,则假定为0。
END_INDEX
is the substring index end number where specifies the SUBSTRING last character. END_INDEX is optional and if not provided the last character of the STRING is assumed.
END_INDEX
是子字符串索引的结束编号,其中指定SUBSTRING最后一个字符。 END_INDEX是可选的,如果未提供,则假定为STRING的最后一个字符。
SUBSTRING
is the sub string which is returned with the START_INDEX and END_INDEX numbers from the STRING.
SUBSTRING
是从STRING返回的带有START_INDEX和END_INDEX数字的子字符串。
从指定索引到结尾的子字符串 (Substring From Specified Index To The End)
Lets start with a simple example about substring where we will specify the start index of the substring and do not provide the end index which will be assumed as the last character of the given string.
让我们从有关子字符串的简单示例开始,在该示例中,我们将指定子字符串的开始索引,而不提供将被视为给定字符串的最后一个字符的结束索引。
s1 = "I love poftut.com"
s1[0:]
# The output is 'I love poftut.com'
s1[1:]
# The output is ' love poftut.com'
s1[2:]
# The output is 'love poftut.com'
s1[5:]
# The output is 'e poftut.com'
s1[55:]
# The output is ''
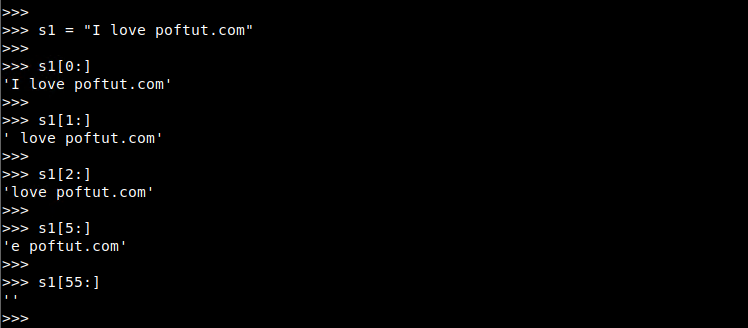
We can see that when we provide start index as 0 the whole comple string is returned as substring. If we provide start index as 55 which do not exist for the given string the substring is empty.
我们可以看到,当我们提供起始索引为0时,整个comple字符串将作为子字符串返回。 如果我们提供的起始索引为55(对于给定的字符串不存在),则子字符串为空。
从开始到指定索引的子串(Substring From Start To Specified Index)
As the start index is optional we can only specify the end index for the substring. The start index will be set as 0 by default.
由于开始索引是可选的,因此我们只能为子字符串指定结束索引。 默认情况下,起始索引将设置为0。
s1 = "I love poftut.com"
s1[:0]
# The output is ''
s1[:1]
# The output is 'I'
s1[:2]
# The output is 'I '
s1[:5]
# The output is 'I lov'
s1[:55]
# The output is 'I love poftut.com'
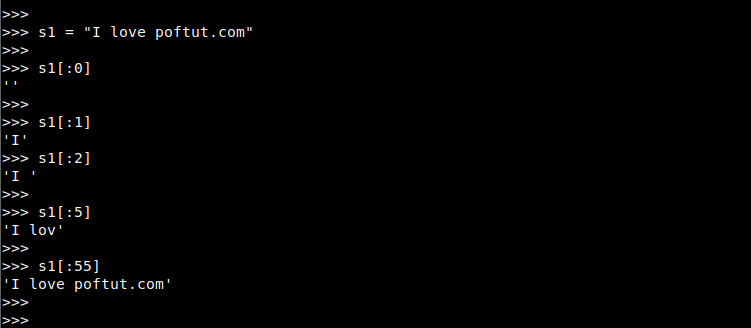
从指定索引到指定索引的子字符串(Substring From Start Specified Index To Specified Index)
Even both the start and end index is optional we can specify both of them. This will give us complete control over the substring where we can explicitly set the start and end index of the substring.
即使开始索引和结束索引都是可选的,我们也可以同时指定它们。 这将使我们能够完全控制子字符串,在此我们可以显式设置子字符串的开始和结束索引。
s1 = "I love poftut.com"
s1[0:16]
# The output is 'I love poftut.co'
s1[0:17]
# The output is 'I love poftut.com'
s1[5:17]
# The output is 'e poftut.com'
s1[5:7]
# The output is 'e '
1[7:5]
# The output is ''
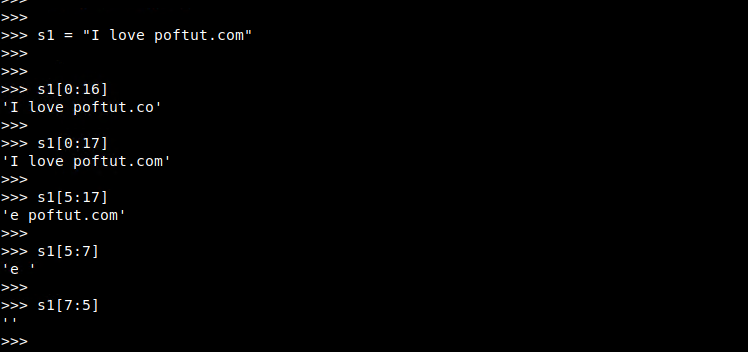
反向子串(Reverse Substring)
Reverse substring is an operation where negative index numbers are used to specify the start and end index of the substring. Using negative number will reverse the index.
反向子字符串是一种操作,其中负索引号用于指定子字符串的开始和结束索引。 使用负数将反转索引。
s1 = "I love poftut.com"
s1[5:]
# The output is 'e poftut.com'
s1[-5:]
# The output is 't.com'
s1[5:8]
# The output is 'e p'
s1[-5:-8]
# The output is ''
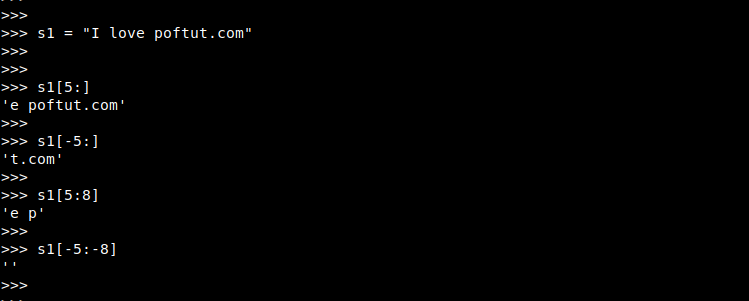
使用split()方法的具有指定字符的子字符串(Substring with Specified Character By Using split() Method)
split()
isa string builtin functin which can split and create substrings from the given string. Split requires a split character which will be used like a splitter or delimiter. By default space ” ” is the split character but it can be also provided explicitly to the split() function.
split()
是一个字符串内置函数,可以从给定的字符串拆分并创建子字符串。 分割需要一个分割字符,该字符将用作分割符或定界符。 默认情况下,空格“”是分割字符,但也可以显式提供给split()函数。
s1 = "I love poftut.com"
s1.split()
# The output is ['I', 'love', 'poftut.com']
s1.split('t')
# The output is ['I love pof', 'u', '.com']
s1.split('.')
# The output is ['I love poftut', 'com']
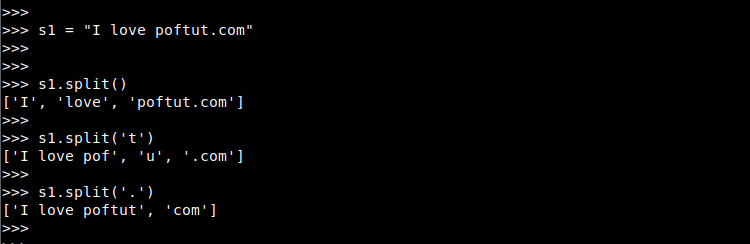