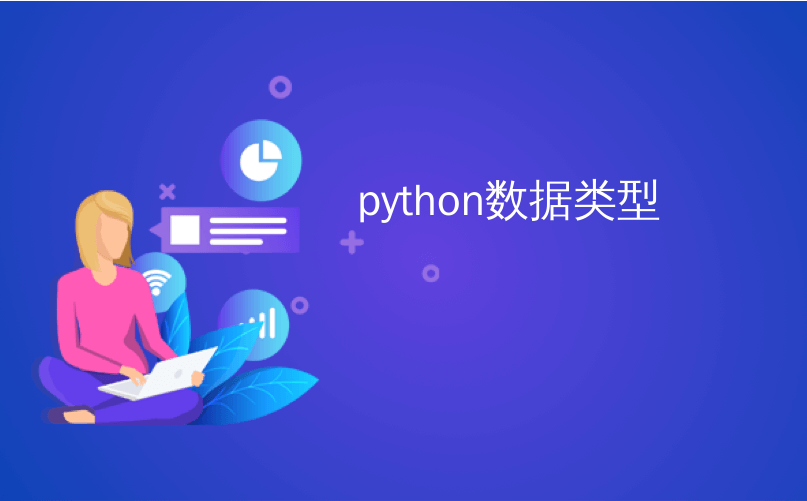
python数据类型
Python provides different data types in order to store values. Every data type in Python is actually an instance of classes. Python provides the following data types.
Python提供了不同的数据类型以存储值。 Python中的每种数据类型实际上都是类的实例。 Python提供以下数据类型。
- Numbers 号码
- Boolean布尔型
- String串
- List清单
- Tuple元组
- Set组
- Dictionary字典
- Binary Types二进制类型
Python数字(Python Numbers)
Python numbers are consist of two main types named integer, floating-point, and complex numbers.
Python数字由两种主要类型组成,分别是整数,浮点数和复数。
Integer
numbers can store any decimal numbers which can be positive or negative. Integer numbers can be any length which is limited with the available memory.
Integer
可以存储正或负的任何十进制数字。 整数可以是任何可用内存限制的长度。
a = 5
b = 10
c = a + b
print(c)
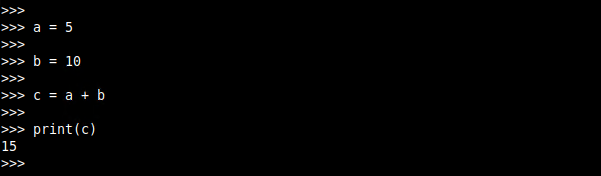
Floating point
numbers are useful to store floating-point values.
Floating point
对于存储浮点值很有用。
a = 1.2
b = 2.8
c = a + b
print(c)
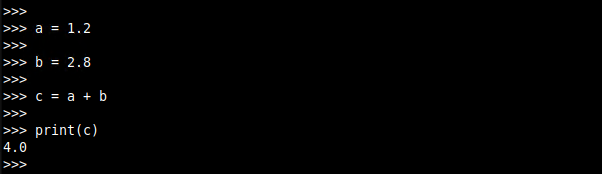
Complex
numbers are mainly used by mathematicians and data professional which are consist of real and imaginary parts.
Complex
主要由数学家和数据专业人员使用,它们由实部和虚部组成。
a = 1+2j
b = 2+1j
Python布尔 (Python Boolean)
Python Boolean values will store basic logic True
or False
.Boolean values also used for different boolean operations like AND, OR, XOR, NOT, etc.
Python布尔值将存储基本逻辑True
或False
。布尔值也用于不同的布尔运算,例如AND,OR,XOR,NOT等。
a = True
b = False
c = a | b
print(c)
#Output will be True
c = a & b
print(c)
#Output will be False
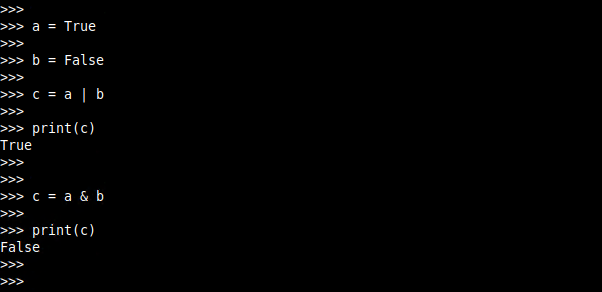
Python字符串(Python Strings)
Python string data type is a sequence of single or multiple Unicode characters. In order to provide the string value single or, double quotes can be used. For multi-line strings triple quotes should be used.
Python字符串数据类型是一个或多个Unicode字符的序列。 为了提供字符串值,可以使用单引号或双引号。 对于多行字符串,应使用三引号。
name ="ismail"
myname = 'ismail'
print(name)
#Output will beismail
print(myname)
#Output will be ismail
print(myname[1])
#Output will be s
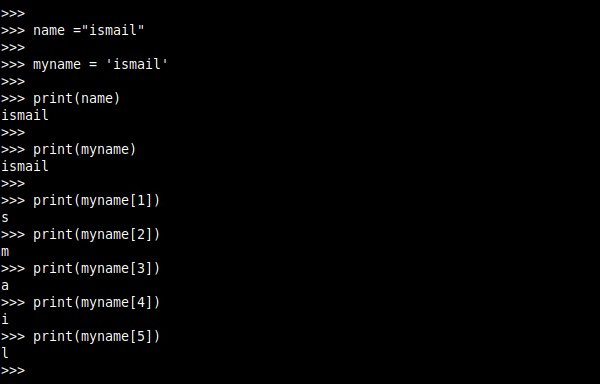
Python清单(Python List)
Python List data type of store a sequence of single or multiple objects or other data types. The list is also called as Array in other programming languages. The list is very flexible where multiple types of data can be stored inside a single list.
Python List数据类型存储一系列单个或多个对象或其他数据类型。 该列表在其他编程语言中也称为Array。 该列表非常灵活,可以在一个列表中存储多种类型的数据。
mylist = [ 1 , "two" , 3 , "four" , 5.0 ]
len(mylist)
#Output will 5 becuase there are 5 elements in this list
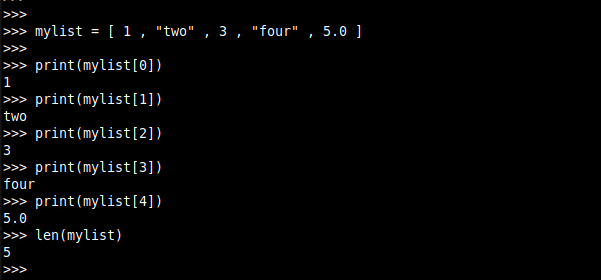
We can see that a first item is a number where second item is a string and the last item is a floating-point number.
我们可以看到第一个项目是一个数字,第二个项目是一个字符串,最后一个项目是一个浮点数。
Python元组 (Python Tuple)
Python Tuple is the very same as the Python List. Except tuple is read-only which means it can not be updated or changed after creation. But on the other side, a list can be updated or changed after creation. In order to update a tuple, a new tuple should be created with the old items.
Python元组与Python列表非常相同。 元组是只读的,这意味着在创建后不能对其进行更新或更改。 但另一方面,列表可以在创建后进行更新或更改。 为了更新元组,应使用旧项目创建一个新的元组。
mytuple = ( 1 , "two" , 3 , "four" , 5.0 )
len(mytuple)
#Output will be 5
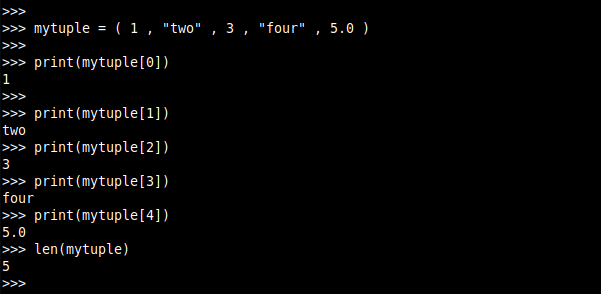
Python集(Python Set)
Python Set data type provides the features of the mathematical sets where every item is unique. Even elements defined multiple times only a single instance will be stored inside the set. Element items do not support indexing which means elements can not be accessed with index numbers.
Python Set数据类型提供了数学集的功能,其中每个项目都是唯一的。 即使多次定义的元素也只能将单个实例存储在集合中。 元素项不支持索引,这意味着无法使用索引号访问元素。
myset = { 1 , 1, 1, "two" , 3 , "four" , 5.0 }
len(myset)
#Output will be 5
print(myset)
#The output will be{1, 3, 5.0, 'two', 'four'}
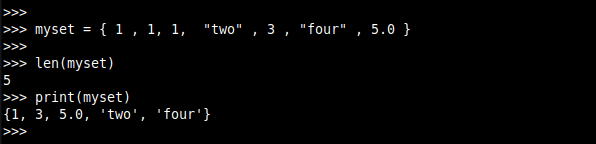
We can see that 1 is defined 3 times in the set named myset but stored only single 1 and the length of the myset is 5 even the definition provides 8 items.
我们可以看到1在名为myset的集合中定义了3次,但只存储了单个1,而即使定义提供了8个项目,myset的长度也为5。
Python字典 (Python Dictionary)
Python Dictionary is a data type which will store key-value pairs or items. It is called a dictionary because its structure is similar to a dictionary. Every key is related to value. Dictionary is optimized for retrieving values via using keys.
Python字典是一种数据类型,它将存储键值对或项目。 之所以称为字典,是因为其结构类似于字典。 每个关键都与价值相关。 字典针对使用键检索值进行了优化。
mydict = { 1:'value' , 'key':2 , 'mykey':'myvalue' , 'name':'ismail'}
len(mydict)
#The output will be 4
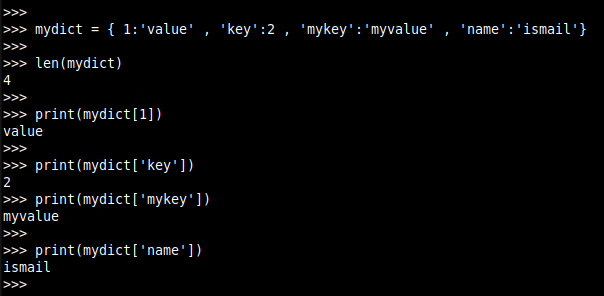
We can see in the example that we can access the values with their keys. The keys can be an integer, a string where values can be too.
在示例中我们可以看到可以使用其键访问值。 键可以是整数,值也可以是字符串。
Python二进制类型 (Python Binary Types)
Python Byte data type is used to store given data in a byte format. The byte data type is defined with the b
before the value like below.
Python Byte数据类型用于以字节格式存储给定的数据。 字节数据类型在值之前用b
定义,如下所示。
mybyte= b'Hello Poftut.com'
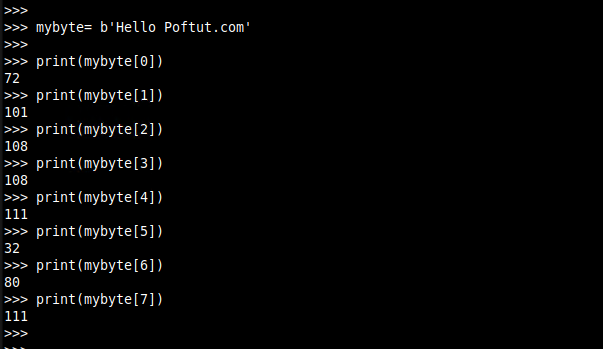
When we print the bytes one by one we can see that the string characters ASCII values are stores as a byte.
当我们一一打印字节时,可以看到字符串字符ASCII值存储为字节。
查找Python数据类型 (Find Python Data Type)
Python programming language data type is specified while setting initial value into a variable. so there is no specific sign about the variable data type. This makes finding a data type a bit complicated but type()
function can be used to get the data type of the provided variable or structure.
在将初始值设置为变量时,指定了Python编程语言数据类型。 因此,关于变量数据类型没有特定的符号。 这使得查找数据类型有些复杂,但是可以使用type()
函数来获取所提供的变量或结构的数据类型。
a = 1
b = 2.0
c = 1 + 2j
myname = "İsmail Baydan"
mylist = [ 1 , "two" , 3 , "four" , 5.0 ]
mytuple = ( 1 , "two" , 3 , "four" , 5.0 )
myset = { 1 , 1, 1, "two" , 3 , "four" , 5.0 }
mydict = { 1:'value' , 'key':2 , 'mykey':'myvalue' , 'name':'ismail'}
mybyte= b'Hello Poftut.com'
type(a)
#The output will <class 'int'>
type(b)
#The output will <class 'float'>
type(c)
#The output will <class 'complex'>
type(myname)
#The output will <class 'str'>
type(mylist)
#The output will <class 'list'>
type(mytuple)
#The output will <class 'tuple'>
type(myset)
#The output will <class 'set'>
type(mydict)
#The output will <class 'dict'>
type(mybyte)
#The output will <class 'bytes'>
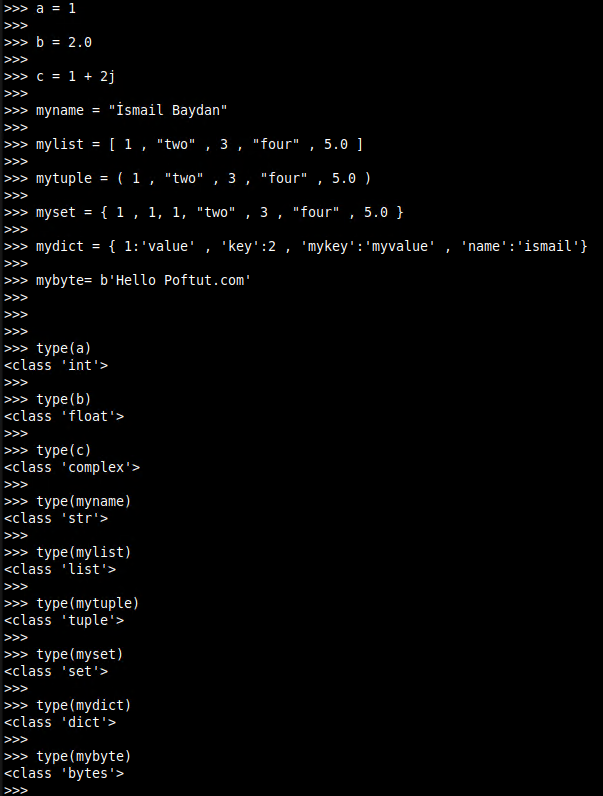
python数据类型