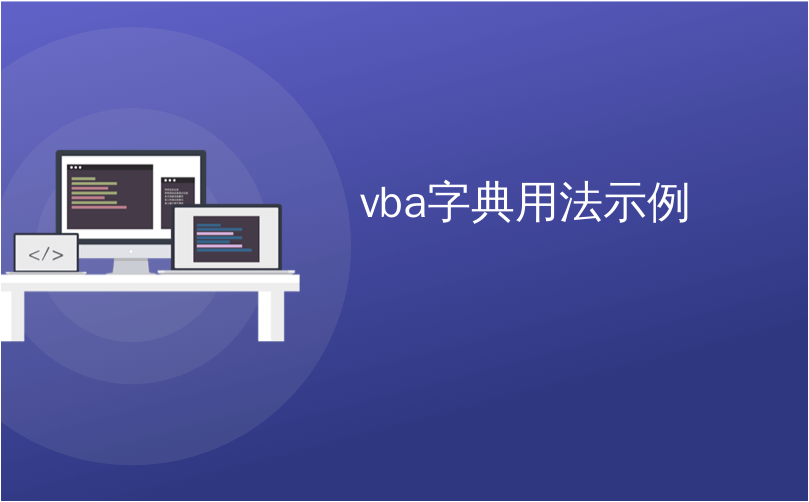
vba字典用法示例
Dictionary is a data type that can be used for different purposes. Dictionaries provide one key and one value matched together. JavaScript programming language does not provide the Dictionary data structure natively. But a dictionary data structure can be implemented with the help of JavaScript Object
type. In this tutorial, we will learn how to create a dictionary, add/remove items, get items, iterate over items, etc.
字典是一种可以用于不同目的的数据类型。 词典提供一个键和一个匹配在一起的值。 JavaScript编程语言本身不提供Dictionary数据结构。 但是,可以借助JavaScript Object
type来实现字典数据结构。 在本教程中,我们将学习如何创建字典,添加/删除项目,获取项目,遍历项目等。
字典或键/值对或项目 (Dictionary or Key/Value Pair or Item)
Before starting to learn the dictionary in JavaScript we have to make clear some terms used with the dictionary data type. Dictionary is consist of single or multiple items which are just key and value pair.
在开始使用JavaScript学习字典之前,我们必须弄清楚与字典数据类型一起使用的一些术语。 词典由单个或多个项目组成,它们只是键和值对。
- `Dictionary` may contain single or multiple items. “字典”可能包含单个或多个项目。
- `Item` consists of Key and Value pairs. Item由键和值对组成。
- `Key` is used to select, search and filter an item. “键”用于选择,搜索和过滤项目。
- `Value` is used to store some data inside an item. “值”用于在项目内部存储一些数据。
用JavaScript创建字典 (Create Dictionary In JavaScript)
As the dictionary is not supported natively we will use the Object type where we will simply create a new object with the dictionary variable name. First, we will create a dictionary just by creating an object whose variable name is dict
but this name can be different.
由于本机不支持字典,因此我们将使用“对象”类型,我们将仅使用字典变量名称创建一个新对象。 首先,我们将通过创建一个变量名称为dict
但该名称可以不同的对象来创建dict
。
//Create Dictionary with Object
var dict = Object();
Alternatively, we can create a dictionary by using curly brackets which will also create an Object
另外,我们可以使用大括号创建字典,这也会创建一个对象
//Create Dictionary with Object
var dict = {};
Another alternative way is creating a dictionary by setting some items or key/value pairs.
另一种替代方法是通过设置一些项或键/值对来创建字典。
var dict = {
ismail: "Baydan",
ahmet: "Ali",
elif: 1,
"ilknur": "Baydan",
10: "Val",
};
We can see from the example that the key and values can get a different type of data like string, integer, etc.
从示例中我们可以看到,键和值可以获取不同类型的数据,例如字符串,整数等。
在字典中添加/填充项目 (Add/Populate Item To Dictionary)
We can add or populate a dictionary very easily by using the key and value like below.
我们可以使用下面的键和值很容易地添加或填充字典。
dict["ismail"] = "baydan";
dict[30]= "This ";
We can also create an item or key/value pair by using dot notation like below. We will add a new key FirstName
to the dictionary named dict
with a value John
.
我们还可以通过如下所示的点符号来创建项目或键/值对。 我们将向名为dict
的字典中添加一个名为John
的新键FirstName
。
dict.FirstName="John";
删除/删除项目到字典 (Remove/Delete Item To Dictionary)
We can also remove a dictionary item by using JavaScript delete
keyword where we will provide the item with dot format or square format. Below we will delete two items those keys are ismail
and 10.
我们还可以使用JavaScript delete
关键字删除字典项,在字典项中,我们将以点格式或正方形格式提供该项。 在下面,我们将删除两项,即密钥ismail
和10。
//Delete Item with Key ismail
delete dict.ismail;
//Delete Item with Key 10
delete dict[10];
迭代/循环字典项 (Iterate/Loop Over Dictionary Items)
Generally, a dictionary will have multiple keys where we may need to iterate or loop over them. We can use the JavaScript for
loop in order to iterate over keys and return the value with the current key.
通常,字典将具有多个键,我们可能需要在这些键上进行迭代或循环。 我们可以使用JavaScript for
循环来遍历键并使用当前键返回值。
//Iterate over dict and get key for each step
for(var k in dict){
//Return the value for the current key
var v = dict[k];
}
获取/访问字典中的项目 (Get/Access Item In Dictionary)
We can get or access a specific item by using the key. We will just provide the key with a square parenthesis or dot usage below.
我们可以使用密钥获取或访问特定项目。 我们仅在下面提供带有方括号或点用法的密钥。
//Access using squre paranthesis
var val1 = dict["Name"];
//Access using dot
var val2 = dict.Surname;
更改/更新字典中的值 (Change/Update Value In Dictionary)
We can also change an existing item value by using its key. This will not delete the item it will just update. In this following example, we will change the Name key value to Elif
and 10 key value to ten
.
我们还可以使用其键更改现有项的值。 这不会删除只会更新的项目。 在下面的示例中,我们将Name键值更改为Elif
,将10键值更改为ten
。
//Change or Update Value
dict.Name = "Elif";
dict[10] = "ten";
检查密钥是否存在 (Check If Key Exist)
We can check if an item or key exists by using a simple if
statement. We will just provide the key to the if statement. If the item or key exists the if block will be executed.
我们可以使用简单的if
语句检查项目或键是否存在。 我们将只提供if语句的密钥。 如果项或键存在,则将执行if块。
if(dict.name){
//The dict.name exist
console.log(dict.name);
}
if(dict[10]){
//The dict 10 exist
console.log(dict[10]);
}
翻译自: https://www.poftut.com/how-create-and-use-dictionary-in-javascript-tutorial-with-examples/
vba字典用法示例