Python provides different methods and functions in order to remove files and directories. As python provides a lot of functionalities we can remove files and directories according to our needs. For example, we can remove files those sizes are bigger than 1 MB.
Python提供了不同的方法和功能,以删除文件和目录。 由于python提供了许多功能,因此我们可以根据需要删除文件和目录。 例如,我们可以删除大小大于1 MB的文件。
检查文件或目录是否存在 (Check If File or Directory Exist)
Before removing a file or directory checking if it exist is very convenient way. We can check a file is exist with the exists()
function of the os.path
module. In the following example we will check different files for their existence.
在删除文件或目录之前,检查它是否存在是非常方便的方法。 我们可以使用os.path
模块的exist exists()
函数检查文件是否存在。 在下面的示例中,我们将检查不同文件的存在。
import os
if os.path.exists("test.txt"):
print("test.txt exist")
else:
print("test.txt do NOT exist")
test.txt exist
status = os.path.exists("test.txt")
#status will be True
status = os.path.exists("text.txt")
#status will be False
status = os.path.exists("/")
#status will be True
status = os.path.exists("/home/ismail")
#status will be True
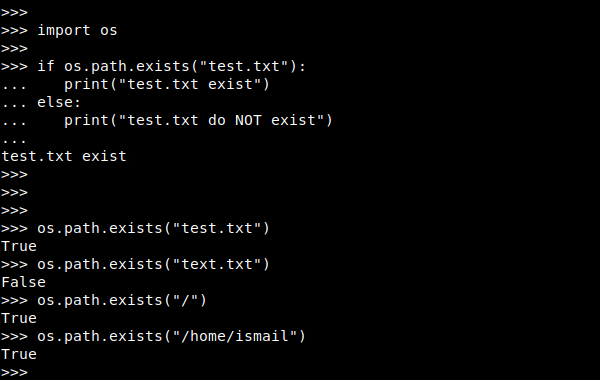
使用remove()方法删除文件(Remove File with remove() Method)
We can use os.remove()
function in order to remove a file. We should import the os
module in order to use remove
function. In this example, we will remove the file named trash
.
我们可以使用os.remove()
函数来删除文件。 我们应该导入os
模块以使用remove
功能。 在此示例中,我们将删除名为trash
的文件。
import os
os.remove("/home/ismail/readme.txt")
os.remove("/home/ismail/test.txt")
os.remove("/home/ismail/Pictures")
#Traceback (most recent call last):
# File "<stdin>", line 1, in <module>
#IsADirectoryError: [Errno 21] Is a directory: '/home/ismail/Pictures'
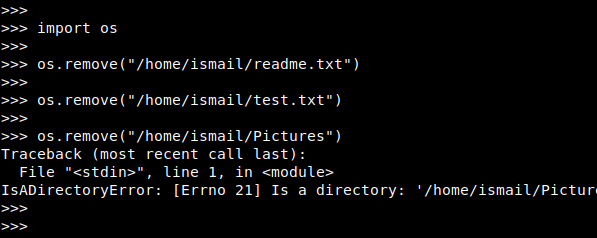
We can see that when we try to remove a directory or folder named “Pictures” we get an error because the remove() method can not be used for removing or deleting directory or folders.
我们可以看到,当我们尝试删除名为“ Pictures”的目录或文件夹时,会收到错误消息,因为remove()方法不能用于删除或删除目录或文件夹。
If the specified file is not exist the FileNotFoundError
will be thrown as an exception. Another error or exception is if the current user do not have rights to delete file running remove()
function will throw the PermissionError
. In order to handle this type of errors and exceptions we should use a try-catch
mechamism and handle them properly.
如果指定的文件不存在,则将引发FileNotFoundError
异常。 另一个错误或异常是,如果当前用户没有删除文件的权限,则运行remove()
函数将抛出PermissionError
。 为了处理这种类型的错误和异常,我们应该使用try-catch
机制,并对其进行正确处理。
处理文件删除操作的异常和错误 (Handle Exceptions and Errors For File Delete Operation)
We can handle previously defined errors and exceptions with the try-catch block. In this part we will hand different exceptions and errors related IsADirectory
, FileNotFound
, PermissionError
.
我们可以使用try-catch块处理先前定义的错误和异常。 在这一部分中,我们将处理与IsADirectory
, FileNotFound
, PermissionError
相关的不同异常和错误。
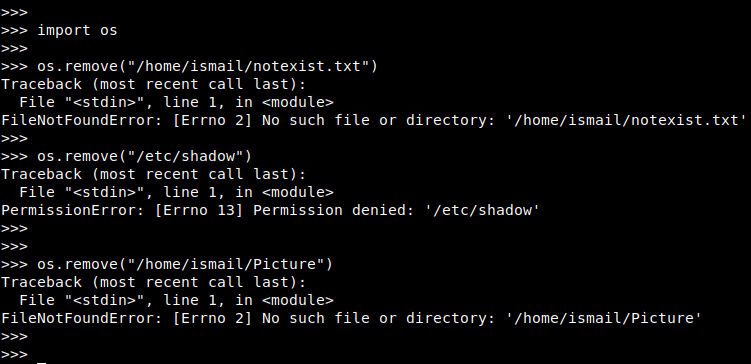
We can in the above that every remote operations created an error or exception. Now we we will handle all these exception properly and print some information about the exceptions.
我们可以在上面看到,每个远程操作都会产生一个错误或异常。 现在,我们将正确处理所有这些异常,并打印一些有关异常的信息。
import os
try:
os.remove("/home/ismail/notexist.txt")
except OSError as err:
print("Exception handled: {0}".format(err))
# Exception handled: [Errno 2] No such file or directory: '/home/ismail/notexist.txt'
try:
os.remove("/etc/shadow")
except OSError as err:
print("Exception handled: {0}".format(err))
#Exception handled: [Errno 13] Permission denied: '/etc/shadow'
try:
os.remove("/home/ismail/Pictures")
except OSError as err:
print("Exception handled: {0}".format(err))
#Exception handled: [Errno 21] Is a directory: '/home/ismail/Pictures'
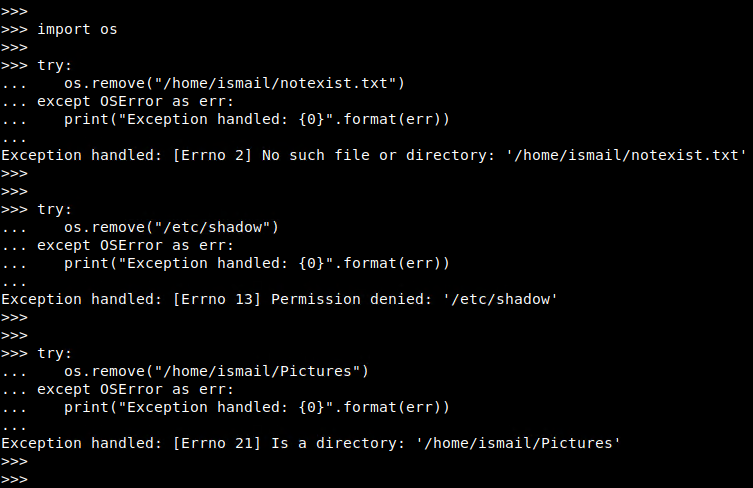
取消链接删除文件(Remove File with unlink)
unlink
is used to remove files. unlink
implements exact mechanisms of the remove
. unlink
is defined because of to implement Unix philosophy. Look remove
for more information.
unlink
用于删除文件。 unlink
实现remove
确切机制。 定义unlink
是因为要实现Unix哲学。 请参阅remove
以获取更多信息。
使用rmdir()方法删除空目录/文件夹 (Remove Empty Directory/Folder with rmdir() Mehtod)
As we know Linux provides rmdir
command which used to remove empty directories. Python provides the same function under os
module. We can only delete empty directories with rmdir
.
众所周知,Linux提供了rmdir
命令,该命令用于删除空目录。 Python在os
模块下提供了相同的功能。 我们只能使用rmdir
删除空目录。
import os
os.rmdir("/home/ismail/data")
使用rmtree()方法递归删除目录和内容 (Delete Directory and Contents Recursively with rmtree() Method)
How can we delete the directory and its contents? We can not use rmdir
because the directory is not empty. We can use shutil
module rmtree
function.
我们如何删除目录及其内容? 我们不能使用rmdir
因为目录不是空的。 我们可以使用shutil
模块的rmtree
函数。
import shutil
shutil.rmtree("/home/ismail/cache")

仅删除特定的文件类型或扩展名(Delete Only Specific File Types or Extensions)
While deleting files we may require only delete specific file types or extensions. We can use *
wildcard in order to specify file extensions. For example, in order to delete text files, we can specify the *.txt
extension. We should also use glob
module and functions to create a list of files.
在删除文件时,我们可能只需要删除特定的文件类型或扩展名。 我们可以使用*
通配符来指定文件扩展名。 例如,为了删除文本文件,我们可以指定*.txt
扩展名。 我们还应该使用glob
模块和函数来创建文件列表。
In this example, we will list all files with extensions .txt
by using glob
function. We will use the list name filelist
for these files. Then loop over the list to remove files with remove()
function one by one.
在此示例中,我们将使用glob
函数列出所有扩展名为.txt
的文件。 我们将对这些文件使用列表名称filelist
。 然后遍历列表,使用remove()
函数一一删除文件。
import glob
import os
filelist=glob.glob("/home/ismail/*.txt")
for file in filelist:
os.remove(file)

翻译自: https://www.poftut.com/delete-and-remove-file-and-directory-with-python/