Python provides a lot of modules for different operating system related operations. Running external command or shell command is a very popular Python developer. We can call Linux or Windows commands from python code or script and use output.
Python为与操作系统相关的不同操作提供了许多模块。 运行外部命令或shell命令是非常受欢迎的Python开发人员。 我们可以从python代码或脚本调用Linux或Windows命令并使用输出。
导入操作系统模块 (Import os Module)
We can use system()
function in order to run a shell command in Linux and Windows operating systems. system()
is provided by os
Module. So we will load this module like below.
我们可以使用system()
函数来在Linux和Windows操作系统中运行shell命令。 system()
由os
Module提供。 因此,我们将像下面那样加载此模块。
import os
使用系统功能运行命令 (Run Command with system Function)
After loading os
module we can use system()
function by providing the external command we want to run. In this example, we will run ls
command which will list current working directory content.
加载os
模块后,我们可以通过提供要运行的外部命令来使用system()
函数。 在此示例中,我们将运行ls
命令,该命令将列出当前的工作目录内容。
import os
os.system('ls')
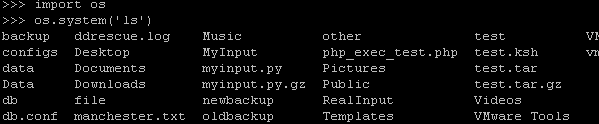
导入子流程模块(Import subprocess Module)
Another alternative for running an external shell command is subprocess
module. This module provides process-related functions. We will use call()
function but first, we need to load subprocess
module.
运行外部Shell命令的另一种选择是subprocess
模块。 此模块提供与过程相关的功能。 我们将使用call()
函数,但首先,我们需要加载subprocess
模块。
import subprocess
通过调用功能运行命令 (Run Command with call Function)
We will use call()
function which will create a separate process and run provided command in this process. In this example, we will create a process for ls
command. We should provide the exact path of the binary we want to call.
我们将使用call()
函数,该函数将创建一个单独的进程并在此进程中运行提供的命令。 在此示例中,我们将为ls
命令创建一个进程。 我们应该提供要调用的二进制文件的确切路径。
import subprocess
subprocess.call("/bin/ls")
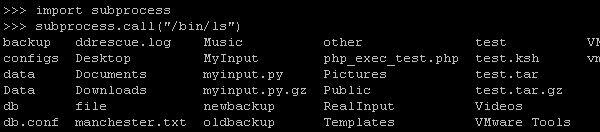
向命令提供参数(Provide Parameters To The Command)
We may need to provide parameters to the command we will call. We will provide a list where this list includes command or binary we will call and parameters as list items. In this example, we will call ls
for path /etc/
with the -l
parameter.
我们可能需要为将要调用的命令提供参数。 我们将提供一个列表,其中该列表包括我们将调用的命令或二进制文件以及作为列表项的参数。 在此示例中,我们将使用-l
参数为/etc/
路径调用ls
。
subprocess.call(['/bin/ls','-l','/etc'])
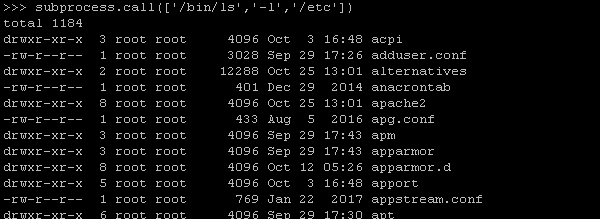
将命令输出保存到变量(Save Command Output To A Variable)
We may need to save the command output to a variable or a file. We will put the output variable named o
like below. We will use read()
function of popen()
returned object. read() will provide the whole output as a single string.
我们可能需要将命令输出保存到变量或文件中。 我们将像下面这样放置名为o
的输出变量。 我们将使用popen()
返回对象的read()
函数。 read()将整个输出作为单个字符串提供。
o=os.popen('ls').read()
print(o)
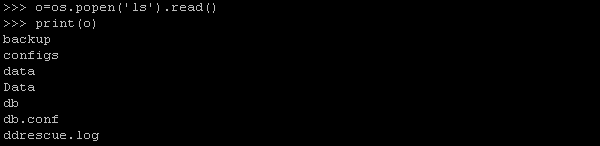
逐行保存命令输出(Save Command Output Line By Line)
Some commands execution can create a lot of outputs that can consist of multiple lines. Alternatively, we can save these command outputs line by line by using the readlines()
function. Also, we can iterate over the readlines() function to read output line by line. Below we will execute the ls
command which will produce multiple lines output. Then we will access these output which is saved into lines
in an array or list fashion.
一些命令执行可以创建很多输出,这些输出可以包含多行。 另外,我们可以使用readlines()
函数逐行保存这些命令输出。 另外,我们可以遍历readlines()函数逐行读取输出。 下面我们将执行ls
命令,该命令将产生多行输出。 然后,我们将访问这些输出,这些输出以数组或列表的方式保存到lines
中。
import os
lines = os.popen('ls').readlines()
print(lines[0])
#aiohttp-2.3.10-cp36-cp36m-manylinux1_x86_64.whl
print(lines[1])
#aiohttp_cors-0.5.3-py3-none-any.whl
print(lines[2])
#allclasses.html
print(lines[3])
#allclasses-index.html
print(lines[4])
#allpackages-index.html
print(lines[5])
#a.out
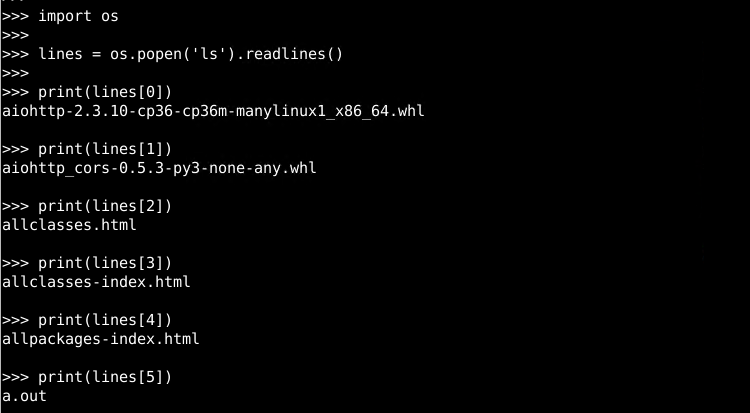
指定标准输入,输出,错误管道/变量(Specify the Standard Input, Output, Error Pipe/Variables)
By default, the output of the executed command is returned as a string with the Popen() function. Alternatively, we can specify pipes or variables to store the input and output pipes for the executed command. In the following example, we will use stdout
and stderr
variables to store standard output and standard error. Popen() function will create an instance where communicate()
function will return the standard output and standard error. This can be useful for commands running for long times where we need output interactively.
默认情况下,已执行命令的输出通过Popen()函数以字符串形式返回。 另外,我们可以指定管道或变量来存储已执行命令的输入和输出管道。 在以下示例中,我们将使用stdout
和stderr
变量存储标准输出和标准错误。 Popen()函数将创建一个实例,其中communicate()
函数将返回标准输出和标准错误。 这对于长时间运行需要交互输出的命令很有用。
import subprocess
c = subprocess.Popen(['ls','-l','.'], stdout = subprocess.PIPE, stderr = subprocess.PIPE)
stdout, stderr = c.communicate()
print(stdout)
print(stderr)
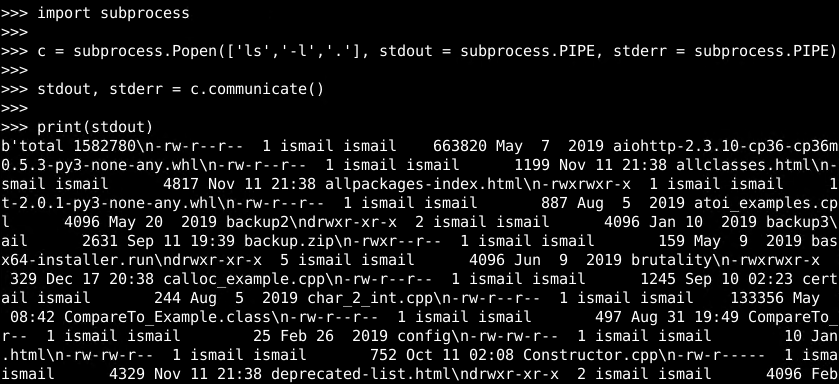
os.system()vs subprocess.run()vs subprocess.Popen()(os.system() vs subprocess.run() vs subprocess.Popen())
As we can see Python provides a lot of functions in order to run and execute system commands. But there are some differences during the usage of them and provides different features. Below we will compare and explain the differences and similarities of these commands.
如我们所见,Python提供了许多功能来运行和执行系统命令。 但是它们在使用过程中存在一些差异,并提供不同的功能。 下面我们将比较和解释这些命令的区别和相似之处。
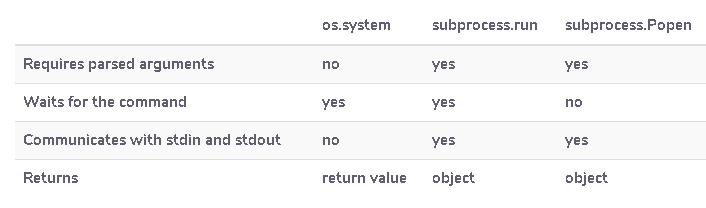
- If we require parsed arguments the os.system() function can not be used but subprocess.run() and subprocess.Popen() can be easily used. 如果我们需要解析的参数,则不能使用os.system()函数,但可以轻松使用subprocess.run()和subprocess.Popen()。
- If the need communication during the execution of the command with the standard input and standard output we should use subprocess.Popen() and subprocess.run() function. 如果在命令执行期间需要与标准输入和标准输出进行通信,则应使用subprocess.Popen()和subprocess.run()函数。
In general, we can see that os.system() function provides very basic and practical usage where subprocess.run() and subprocess.Popen() provides more advanced usage with features.
通常,我们可以看到os.system()函数提供了非常基本和实际的用法,其中subprocess.run()和subprocess.Popen()提供了具有功能的更高级用法。