Python provides different types of List structures in order to hold multiple elements in a single variable like an array. Generally, the same type of data is held in these elements and we may want to sort them for value or key, incremental or decremental. In this tutorial, we will examine sorting Python List structure in different ways.
Python提供了不同类型的List结构,以便将多个元素保存在单个变量(如数组)中。 通常,这些元素中包含相同类型的数据,我们可能希望按值或键,增量或减量对它们进行排序。 在本教程中,我们将以不同的方式检查Python列表结构的排序。
创建Python列表 (Create Python List)
Before starting examples sort operation examples, we will create a simple list like below. This list consists of vowels as we can see below.
在开始示例排序操作示例之前,我们将创建一个如下所示的简单列表。 此列表由元音组成,如下所示。
mylist=['a','u','e','i']
sort()函数语法 (sort() Function Syntax)
sort() function has very simple syntax where parameters like reverse
, key
key be provided as key/value pair to pass parameter. There is no return value for the function sort() which means sorted list values will be stored in the current list.
sort()函数的语法非常简单,其中将诸如reverse
, key
key之类的参数作为键/值对提供以传递参数。 函数sort()没有返回值,这意味着排序后的列表值将存储在当前列表中。
LIST.sort(PARAMETER)
- LIST is the list variable that contains multiple items and sorted with the sort() function. LIST是包含多个项目并使用sort()函数排序的列表变量。
- PARAMETER is used to pass some arguments like reverse sort, sort according to key, etc. PARAMETER用于传递一些参数,例如反向排序,根据键排序等。
带sort()函数的排序列表 (Sort List with sort() Function)
In this example, we will use sort()
function in order to list the items of the list named mylist . After the sort operation, the elements of the list will be sorted. So we will print the list to see this.
在此示例中,我们将使用sort()
函数以列出名为mylist的列表中的项目。 排序操作后,将对列表中的元素进行排序。 因此,我们将打印列表以查看此内容。
mylist=['a','u','e','i']
mylist.sort()
print(mylist)

使用sorted()函数排序(Sort with sorted() Function)
There is also a separate function named sorted() which accepts List type variables as a parameter and return as a sorted list like below. We will provide mylist
and assign the sorted list as mysortedlist
like below. As we can see that the difference is that the function sort() will sort in-place but the function sorted() will sort given list and return the sorted list as a return value.
还有一个名为sorted()的单独函数,该函数接受List类型的变量作为参数,并返回如下所示的排序列表。 我们将提供mylist
并将排序后的列表分配为mysortedlist
如下所示。 正如我们所看到的,区别在于函数sort()将就地排序,而函数sort()将给定列表进行排序并将已排序的列表作为返回值返回。
mylist=['a','u','e','i']
sortedlist=sorted(mylist)
print(sortedlist)

使用sorted()函数对逆序排序(Sort Reverse Order with sorted() Function)
We have also the ability to sort in reverse order. We will use the parameter reverse with the value true. The reverse parameter will make the sort operation in reverse which means descending.
我们还可以按相反的顺序排序。 我们将使用值true的反向参数。 reverse参数将使排序操作反向进行,这意味着递减。
mylist=['a','u','e','i']
sortedlist=sorted(mylist,reverse=True)
print(sortedlist)
#Output will be ['u', 'i', 'e', 'a']
mylist=[3,9,5,7,2,0,4,1,2]
sortedlist=sorted(mylist,reverse=True)
print(sortedlist)
#Output will be [9, 7, 5, 4, 3, 2, 2, 1, 0]
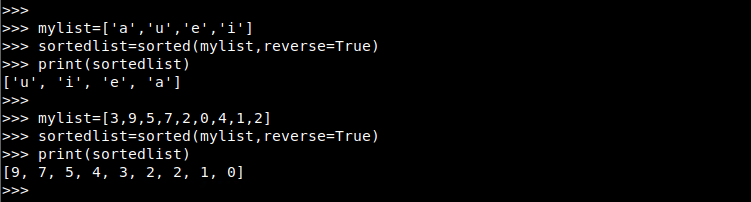
使用sort()函数对逆序排序(Sort Reverse Order with sort() Function)
Function sort() also provides the ability to reverse sort. We will use the parameter reverse
with the value True
in order to sort in a reverse or descending way. Below we will sort some characters and numbers in reverse order.
函数sort()还提供了反向排序的功能。 我们将使用带有值True
的参数reverse
,以反向或降序排序。 在下面,我们将按照相反的顺序对一些字符和数字进行排序。
mylist=['a','u','e','i']
mylist.sort(reverse=True)
print(mylist)
#Output will be ['u', 'i', 'e', 'a']
mylist=[3,9,5,7,2,0,4,1,2]
mylist.sort(reverse=True)
print(mylist)
#Output will be [9, 7, 5, 4, 3, 2, 2, 1, 0]
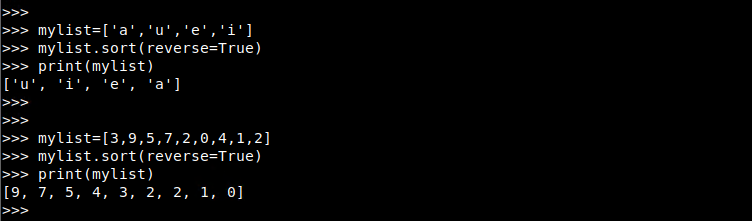
使用功能sorted()对元组进行排序(Sort Tuples with Function sorted())
Function sorted() can be used to sort List and List types like Tuples. Tuples are named as list type structure and can be sorted with the function sorted(). Below we will create a tuple named mytuple and sort it with the sorted() function.
函数sorted()可用于对List和List类型进行排序,例如Tuples。 元组被命名为列表类型结构,并且可以使用sorted()函数进行排序。 在下面,我们将创建一个名为mytuple的元组,并使用sorted()函数对其进行排序。
mytuple=('a','u','e','i')
mysortedtuple=tuple(sorted(mytuple))
print(mysortedtuple)
#Output will be ('a', 'e', 'i', 'u')
mytuple=(3,9,5,7,2,0,4,1,2)
mysortedtuple=tuple(sorted(mytuple))
print(mysortedtuple)
#Output will be (0, 1, 2, 2, 3, 4, 5, 7, 9)
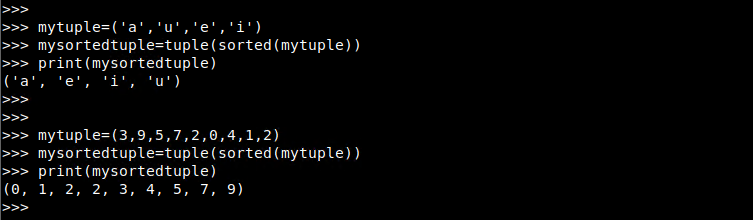
翻译自: https://www.poftut.com/how-to-sort-list-in-python-programming-language/