Powershell arrays are used to store a collection of elements in a single variable. These elements do not to be the same type and declared in different ways.
Powershell数组用于将元素集合存储在单个变量中。 这些元素不是同一类型,并且以不同的方式声明。
创建数组 (Create Array)
We will start with a simple example where we will create an array by providing the items. Items will be delimited with the comma. The array definition will start with a dollar sign $
.
我们将从一个简单的示例开始,在该示例中,我们将通过提供项目来创建一个数组。 项目将以逗号分隔。 数组定义将以美元符号$
开头。
PS> $ myArr=1,"İsmail",3.5,"Ahmet"

We can see that different type of elements like integer, float, string is used to create an array.
我们可以看到,使用了不同类型的元素(例如整数,浮点数,字符串)来创建数组。
使用范围创建数组 (Create Array with Range)
We can create an array by providing a range with a start and end number. The intermediate values will be created automatically. We will use parenthesis and two points between the start and end number. In this example, we will create an array with a range from 1 to 10 like below.
我们可以通过提供一个包含开始和结束编号的范围来创建数组。 中间值将自动创建。 我们将使用括号以及开始和结束编号之间的两个点。 在此示例中,我们将创建一个范围为1到10的数组,如下所示。
PS> $myArr=( 1 .. 10 )
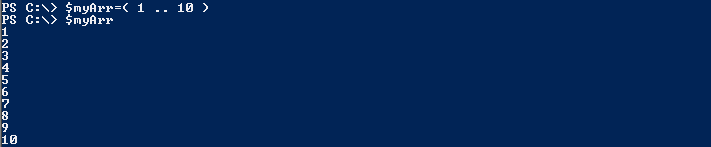
创建数值数组(Create Numeric Array)
We can create a numeric array by specifying the numeric array elements one by one. We will create a numeric array with even numbers like below.
我们可以通过逐个指定数字数组元素来创建数字数组。 我们将创建一个具有偶数的数字数组,如下所示。
PS> $myArr= 2, 4, 6, 8

创建空数组(Create Empty Array)
It may sound a bit non-sense but we may need to create an empty array. We will use @()
in order to create an empty array.
听起来有些废话,但我们可能需要创建一个空数组。 我们将使用@()
来创建一个空数组。
PS> [email protected]()
创建强类型数组 (Create Strongly Typed Array)
As Powershell is a scripting language the provided element values will be interpreted accordingly. This may create some confusion during the creation of array type. We can create a strongly typed array by providing the array type like an integer. In this example, we will create a strongly defined integer array where the elements will be only integer values.
由于Powershell是一种脚本语言,因此将相应地解释提供的元素值。 在创建数组类型期间,这可能会造成一些混乱。 我们可以通过提供类似于整数的数组类型来创建强类型数组。 在此示例中,我们将创建一个严格定义的整数数组,其中的元素将仅为整数值。
PS > [int[]] $myArr=10,20,30,40

创建多维数组(Create Multi-Dimensional Array)
As the problems become more complex we may need to use Multi-Dimensional arrays to express and solve complex problems with complex data types. We will use @
sign and parenthesis in order to create multi dimension arrays.
随着问题变得越来越复杂,我们可能需要使用多维数组来表达和解决具有复杂数据类型的复杂问题。 我们将使用@
符号和括号来创建多维数组。
PS> $myArr= @((1,2,3),(4,5,6),(7,8,9))
为数组增加价值 (Add Value To Array)
One of the most used operations is adding an element or item to the existing array. We can use +=
or Add()
function in order to add new element. For example, we will add 5 to the existing array.
最常用的操作之一是将元素或项目添加到现有数组。 我们可以使用+=
或Add()
函数来添加新元素。 例如,我们将5添加到现有数组。
PS> $myArr=10,20,30,40
PS> $myArr+=5

We can also use Add()
function to add 5 to the existing array named myArr
like below.
我们还可以使用Add()
函数将5添加到名为myArr
的现有数组中,如下所示。
PS> $myArr.Add(5)
使用索引打印指定的数组 (Print Specified Array with Index)
As array elements are stored in an indexed way we can print specified item by providing its index. In this example, we will print the second item in the array myArr
. Keep in mind that index starts from 0 in Powershell. So index 1 will be the second item in an array.
由于数组元素以索引方式存储,因此我们可以通过提供指定的索引来打印指定的项目。 在此示例中,我们将在数组myArr
打印第二个项目。 请记住,在Powershell中,索引从0开始。 因此索引1将是数组中的第二项。
PS> $myArr[1]

设置或更改指定的索引值(Set or Change Specified Index Value)
We can use index number in order to change given array specified element. In this case we will set index 1 as 100
like below.
我们可以使用索引号来更改给定数组的指定元素。 在这种情况下,我们将索引1设置为100
如下所示。
PS> $myArr[1]=100

从数组中检索指定范围的项目(Retrieve Specified Range Items From Array)
If we need to get a range of item from an array we can use ..
and provide the start and end index values. In this example, we will get range between 2 and 4 .
如果我们需要从数组中获取一定范围的项目,则可以使用..
并提供开始和结束索引值。 在此示例中,我们将获得2到4之间的范围。
PS> $myArr[2..4]

返回数组的所有项目(Return All Items Of Array)
We can return all items of the given array in different ways the most basic way is just providing the array.
我们可以以不同的方式返回给定数组的所有项目,最基本的方式就是提供数组。
PS> $myArr

获取数组长度(Get The Array Length)
Arrays have length according to the count of the elements. We can get the array length information with the length
option like below.
数组的长度取决于元素的数量。 我们可以使用length
选项获得数组长度信息,如下所示。
PS> $myArr.length

In this case our array named myArr
is 4 .
在这种情况下,名为myArr
的数组为4。
返回数组的最后一个元素 (Return Last Element Of Array)
If we need to get the last element of the given array we will use the negative index which means start from the last position. We will use -1
like below.
如果需要获取给定数组的最后一个元素,我们将使用负索引,这意味着从最后一个位置开始。 我们将使用-1
如下所示。
PS> $myArr[-1]
从多维数组返回项目 (Return Item From Multidimensional Array)
As we can create multi dimensional array we may need to get an element. We will provide multiple indexes according to dimension count. For two dimension we will use [][]
like below.
由于我们可以创建多维数组,因此我们可能需要获取一个元素。 我们将根据维数提供多个索引。 对于二维,我们将使用[][]
如下所示。
PS> $myArr[1][1]
检查给定元素是否存在 (Check If Given Element Exist)
We can use -eq
to check whether an array has specified item value. In this example, we will check if myArr
has the value 10
like below.
我们可以使用-eq
来检查数组是否具有指定的项目值。 在此示例中,我们将检查myArr
是否具有值10
如下所示。
PS> $myArr -eq 10

翻译自: https://www.poftut.com/powershell-array-tutorial-with-examples/