Loops are a very important part of the programming languages. Loops are used to iterate over given collection, array, list, etc. There are mainly two types of loops named for loop and while loop. There are other types of loops but they can specially design for the specific programming languages.
循环是编程语言中非常重要的部分。 循环用于遍历给定的集合,数组,列表等。循环主要有两种类型,分别为for循环和while循环。 还有其他类型的循环,但是它们可以为特定的编程语言专门设计。
循环类型 (Loop Types)
As stated previously there are two types of general loop. They are while
and for
loops. For
loop is more popular than while
loop. Loops need some start case, continue condition and end condition which will end the loop to prevent infinite loop.
如前所述,一般循环有两种类型。 它们是while
和for
循环的。 For
循环比while
循环更流行。 循环需要一些开始情况,继续条件和结束条件,这将结束循环以防止无限循环。
对于循环 (For Loop)
For loop will have the general syntax below.
For循环将具有以下常规语法。
for(START_STATEMENT; END_CONDITION ; EXECUTION_STATEMENT){
CODE
}
- `START_STATEMENT` is used for the first statement during execution and start of the for loop. After the first execution, it will not use again. It is like an initializer. 在执行和开始for循环期间,“ START_STATEMENT”用于第一条语句。 第一次执行后,将不再使用。 它就像一个初始化程序。
- `EXECUTION_STATEMENT` will be executed in every step of the loop. It will help to change the END_CONDITION variables to reach END_CONDITION. EXECUTION_STATEMENT将在循环的每个步骤中执行。 它将有助于更改END_CONDITION变量以达到END_CONDITION。
- `END_CONDITION` specifies the end condition for the loop. If the end condition met the loop will end. END_CONDITION指定循环的结束条件。 如果满足结束条件,则循环将结束。
While循环 (While Loop)
While loop will have the following general syntax below. As we can see while loop is more simple then for loop where it has END_CONDITION
.
While循环将在下面具有以下常规语法。 如我们所见,while循环比具有END_CONDITION
for循环更简单。
while(END_CONDITION){
CODE
}
- `END_CONDITION` is the condition used in While loop where it specifies the end condition by using variables. When the end condition met the while loop will end. END_CONDITION是While循环中使用的条件,它通过使用变量指定结束条件。 当满足结束条件时,while循环将结束。
Java循环 (Java Loop)
Java programming language provides both for and while loops. It is very similar to the C/C++ and C# programming languages.
Java编程语言提供了for和while循环。 它与C / C ++和C#编程语言非常相似。
Java For循环 (Java For Loop)
Java programming language for loop will have the following syntax. It is the same which is described at the beginning of the post.
用于循环的Java编程语言将具有以下语法。 与帖子开头所述的内容相同。
for(START_STATEMENT; END_CONDITION ; EXECUTION_STATEMENT){
CODE
}
and as an example, we will start counting from 1 to the 10 with the following Java for a loop.
并以一个示例为例,我们将使用以下Java循环从1到10开始计数。
for (int i = 1; i < 11; i++) {
System.out.println(i);
}
- `int i` is the start statement of the loop. It will create an integer variable named `i` and set its initialization value to the `1`. “ int i”是循环的开始语句。 它将创建一个名为“ i”的整数变量,并将其初始化值设置为“ 1”。
- `i < 11` is the end condition which will be checked in every loop step whether it meets or nor. If the end condition is met which means `i` is lower then 11 the loop will continue where when `i` is higher then 11 the loop will end. “ i <11”是结束条件,将在每个循环步骤中检查是否满足。 如果满足结束条件,这意味着“ i”低于11,则循环将继续,而当“ i”高于11则循环将结束。
- `i++` is the execution statement which is executed in every step of the loop. This is used to reach `i` to the end condition by increasing it. “ i ++”是在循环的每一步中执行的执行语句。 用来通过增加i达到最终条件。
Java While循环 (Java While Loop)
Java programming language also provides while loop like below.
Java编程语言还提供如下的while循环。
while(END_CONDITION){
CODE
}
We will use the following example which will start counting from 1 to the 10 .
我们将使用以下示例,该示例将从1开始计数到10。
int i = 1;
while (i < 11) {
System.out.println(i);
i++;
}
- `int i = 1` is used to specify initialize `i`. “ int i = 1”用于指定初始化“ i”。
- `i < 11` is the end condition where the loop will end when `i` reaches to the 10. “ i <11”是结束条件,当“ i”达到10时,循环将结束。
C / C ++循环 (C/C++ Loop)
C/C++ is very similar languages to Java and C#.
C / C ++与Java和C#非常相似。
C / C ++ For循环 (C/C++ For Loop)
C/C++ programming languages for loop will have the following syntax. It is the same which is described at the beginning of the post.
用于循环的C / C ++编程语言将具有以下语法。 与帖子开头所述的内容相同。
for(START_STATEMENT; END_CONDITION ; EXECUTION_STATEMENT){
CODE
}
and as an example, we will start counting from 1 to the 10 with the following C/C++ for a loop.
举例来说,我们将使用以下C / C ++从1到10开始计数。
for (int i = 1; i < 11; i++) {
printf("%d",i);
}
- `int i` is the start statement of the loop. It will create an integer variable named `i` and set its initialization value to the `1`. “ int i”是循环的开始语句。 它将创建一个名为“ i”的整数变量,并将其初始化值设置为“ 1”。
- `i < 11` is the end condition which will be checked in every loop step whether it meets or nor. If the end condition is met which means `i` is lower then 11 the loop will continue where when `i` is higher then 11 the loop will end. “ i <11”是结束条件,将在每个循环步骤中检查是否满足。 如果满足结束条件,这意味着“ i”低于11,则循环将继续,而当“ i”高于11则循环将结束。
- `i++` is the execution statement that is executed in every step of the loop. This is used to reach `i` to the end condition by increasing it. “ i ++”是在循环的每一步中执行的执行语句。 通过增加i达到最终条件。
C / C ++ While循环 (C/C++ While Loop)
C/C++ programming languages also provide while loop like below.
C / C ++编程语言还提供如下while循环。
while(END_CONDITION){
CODE
}
We will use the following example which will start counting from 1 to the 10.
我们将使用以下示例,该示例将从1开始计数到10。
int i = 1;
while (i < 11) {
printf("%d",i);
i++;
}
- `int i = 1` is used to specify initialize `i`. “ int i = 1”用于指定初始化“ i”。
- `i < 11` is the end condition where the loop will end when `i` reaches to the 10. “ i <11”是结束条件,当“ i”达到10时,循环将结束。
PHP循环 (PHP Loop)
PHP programming language provides both for and while loops. It is very similar to the C/C++ and C# programming languages.
PHP编程语言提供了for和while循环。 它与C / C ++和C#编程语言非常相似。
PHP的循环 (PHP For Loop)
PHP programming language for loop will have the following syntax. It is the same which is described at the beginning of the post.
用于循环PHP编程语言将具有以下语法。 与帖子开头所述的内容相同。
for(START_STATEMENT; END_CONDITION ; EXECUTION_STATEMENT){
CODE
}
and as an example, we will start counting from 1 to the 10 with the following PHP for a loop.
举例来说,我们将从以下PHP开始从1到10计数。
for ($i = 1; $i < 11; $i++) {
echo $i;
}
- `int i` is the start statement of the loop. It will create an integer variable named `i` and set its initialization value to the `1`. “ int i”是循环的开始语句。 它将创建一个名为“ i”的整数变量,并将其初始化值设置为“ 1”。
- `i < 11` is the end condition which will be checked in every loop step whether it meets or nor. If the end condition is met which means `i` is lower then 11 the loop will continue where when `i` is higher then 11 the loop will end. “ i <11”是结束条件,将在每个循环步骤中检查是否满足。 如果满足结束条件,这意味着“ i”低于11,则循环将继续,而当“ i”高于11则循环将结束。
- `i++` is the execution statement that is executed in every step of the loop. This is used to reach `i` to the end condition by increasing it. “ i ++”是在循环的每一步中执行的执行语句。 用来通过增加i达到最终条件。
PHP While循环 (PHP While Loop)
PHP programming language also provides while loop like below.
PHP编程语言还提供如下的while循环。
while(END_CONDITION){
CODE
}
We will use the following example which will start counting from 1 to the 10 .
我们将使用以下示例,该示例将从1开始计数到10。
$i = 1;
while ($i < 11) {
echo $i;
$i++;
}
- `int i = 1` is used to specify initialize `i`. “ int i = 1”用于指定初始化“ i”。
- `i < 11` is the end condition where the loop will end when `i` reaches to the 10. “ i <11”是结束条件,当“ i”达到10时,循环将结束。
Python循环 (Python Loop)
Python is a programming language that is designed for novice users. Python provides a different syntax from other programming languages like C/C++, Java, C#.
Python是一种专为新手用户设计的编程语言。 Python提供了与其他编程语言(例如C / C ++,Java,C#)不同的语法。
Python的循环 (Python For Loop)
We can use for
loop by specifying a list or using range()
function which will create an iterable list for specified range with numbers.
我们可以通过指定列表或使用range()
函数来使用for
循环,该函数将为带有数字的指定范围创建一个可迭代的列表。
for ELEMENT in LIST:
print(ELEMENT)
We will loop over a list from 1 to 10 which is created with the range()
function like below.
我们将遍历一个从1到10的列表,该列表是使用如下的range()
函数创建的。
for x in range(1,10):
print(x)
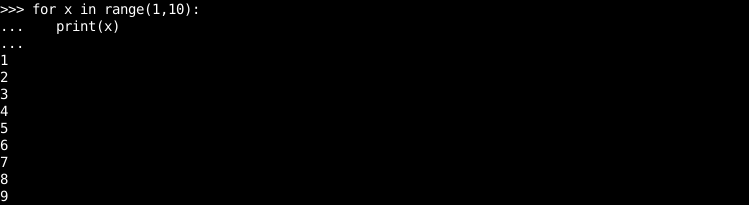
Python While循环(Python While Loop)
While loop will be similar to the for loop where we will create an x
variable with the 1
initialization value and then increment in the while loop one by one.
while循环类似于for循环,在for循环中,我们将使用1
初始化值创建一个x
变量,然后在while循环中逐个递增。
x=1
while(x<11):
print(x)
x=x+1
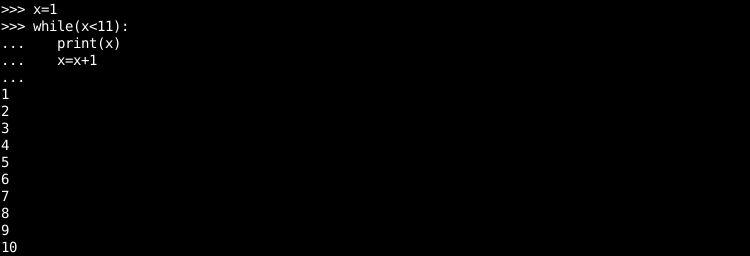
C#循环(C# Loop)
The C# programming language is very similar to the C/C++ and Java programming languages.
C#编程语言与C / C ++和Java编程语言非常相似。
C#For循环 (C# For Loop)
C# programming languages for the loop will have the following syntax. It is the same which is described at the beginning of the post.
循环的C#编程语言将具有以下语法。 与帖子开头所述的内容相同。
for(START_STATEMENT; END_CONDITION ; EXECUTION_STATEMENT){
CODE
}
and as an example, we will start counting from 1 to the 10 with the following C# for a loop.
并以一个示例为例,我们将使用以下C#开始从1到10进行计数。
for (int i = 1; i < 11; i++) {
Console.WriteLine(i.ToString());
}
- `int i` is the start statement of the loop. It will create an integer variable named `i` and set its initialization value to the `1`. “ int i”是循环的开始语句。 它将创建一个名为“ i”的整数变量,并将其初始化值设置为“ 1”。
- `i < 11` is the end condition which will be checked in every loop step whether it meets or nor. If the end condition is met which means `i` is lower then 11 the loop will continue where when `i` is higher then 11 the loop will end. “ i <11”是结束条件,将在每个循环步骤中检查是否满足。 如果满足结束条件,这意味着“ i”低于11,则循环将继续,而当“ i”高于11则循环将结束。
- `i++` is the execution statement that is executed in every step of the loop. This is used to reach `i` to the end condition by increasing it. “ i ++”是在循环的每一步中执行的执行语句。 用来通过增加i达到最终条件。
C#While循环 (C# While Loop)
The C# programming language also provides while loop like below.
C#编程语言还提供了while循环,如下所示。
while(END_CONDITION){
CODE
}
We will use the following example which will start counting from 1 to the 10.
我们将使用以下示例,该示例将从1开始计数到10。
int i = 1;
while (i < 11) {
Console.WriteLine(i.ToString());
i++;
}
- `int i = 1` is used to specify initialize `i`. “ int i = 1”用于指定初始化“ i”。
- `i < 11` is the end condition where the loop will end when `i` reaches to the 10. “ i <11”是结束条件,当“ i”达到10时,循环将结束。
JavaScript循环 (JavaScript Loop)
The JavaScript programming language is very similar to the C/C++ and Java programming languages.
JavaScript编程语言与C / C ++和Java编程语言非常相似。
JavaScript的循环 (JavaScript For Loop)
JavaScript programming languages for the loop will have the following syntax. It is the same which is described at the beginning of the post.
循环JavaScript编程语言将具有以下语法。 与帖子开头所述的内容相同。
for(START_STATEMENT; END_CONDITION ; EXECUTION_STATEMENT){
CODE
}
and as an example, we will start counting from 1 to the 10 with the following JavaScript for a loop.
以一个示例为例,我们将使用以下JavaScript从一个循环开始从1到10计数。
for ( i = 1; i < 11; i++) {
console.log(i);
}
- `int i` is the start statement of the loop. It will create an integer variable named `i` and set its initialization value to the `1`. “ int i”是循环的开始语句。 它将创建一个名为“ i”的整数变量,并将其初始化值设置为“ 1”。
- `i < 11` is the end condition which will be checked in every loop step whether it meets or nor. If the end condition is met which means `i` is lower then 11 the loop will continue where when `i` is higher then 11 the loop will end. “ i <11”是结束条件,将在每个循环步骤中检查是否满足。 如果满足结束条件,这意味着“ i”低于11,则循环将继续,而当“ i”高于11则循环将结束。
- `i++` is the execution statement that is executed in every step of the loop. This is used to reach `i` to the end condition by increasing it. “ i ++”是在循环的每一步中执行的执行语句。 用来通过增加i达到最终条件。
JavaScript While循环 (JavaScript While Loop)
The JavaScript programming language also provides while loop like below.
JavaScript编程语言还提供while循环,如下所示。
while(END_CONDITION){
CODE
}
We will use the following example which will start counting from 1 to the 10.
我们将使用以下示例,该示例将从1开始计数到10。
int i = 1;
while (i < 11) {
console.log(i);
i++;
}
- `int i = 1` is used to specify initialize `i`. “ int i = 1”用于指定初始化“ i”。
- `i < 11` is the end condition where the loop will end when `i` reaches to the 10. “ i <11”是结束条件,当“ i”达到10时,循环将结束。