Multiprocessing is creating, running, ending multiple processes and threads from a single process for a specific purpose. Python programming language provides a lot of different features of multiprocessing.
多重处理是为了特定目的从单个进程创建,运行,结束多个进程和线程。 Python编程语言提供了许多不同的多重处理功能。
多处理功能 (Multiprocessing Features)
Multiprocessing provides a lot of features to the program or application developers. We will list some of the below.
多重处理为程序或应用程序开发人员提供了许多功能。 我们将在下面列出一些。
Performance
is the most popular feature of multiprocessing. A single process task can be divided into multiple ones where each process can complete faster. In the end, the complete task will be completed in less time.Performance
是多处理的最流行功能。 单个流程任务可以分为多个,每个流程可以更快地完成。 最后,完成任务将在更短的时间内完成。Reliability
is provided by creating simple tas pieces were even some piece corrupt we can create a little piece of task where other pieces to not interfere.通过创建简单的tas片段(即使某些片段已损坏)来提供
Reliability
我们可以创建一个小任务,而其他任务不会受到干扰。Efficiency
is done by using all CPU sources like threads r multiple CPUs. Modern CPU’s provides multiple processing units and threads to run multiple processes at the same time. With multiprocessing, we can use these resources efficiently.通过使用所有CPU资源(例如线程或多个CPU)来提高
Efficiency
。 现代CPU提供了多个处理单元和线程,以同时运行多个进程。 通过多处理,我们可以有效地使用这些资源。
导入多处理模块/库 (Import Multiprocessing Module/Library)
Python provides multiprocessing functions and capabilities with the multiprocessing
module. So in order to use multiprocessing functions and capabilities, we have to import multiprocessing
module like below.
Python通过多multiprocessing
模块提供了多处理功能。 因此,为了使用多处理功能,我们必须导入如下的multiprocessing
模块。
import multiprocessing

We can also see that multiprocessing
module provides process
and similar functions.
我们还可以看到, multiprocessing
模块提供了process
和类似的功能。
产生多个进程 (Spawn Multiple Processes)
We will start with a simple process spawn or create which will create a new process instance with the Process()
function. Process()
function basically needs two parameters where we need to specify the function name where the newly created function will run. Also, we can provide some parameters with the args
set like below.
我们将从一个简单的流程生成或创建开始,该流程将使用Process()
函数创建一个新的流程实例。 Process()
函数基本上需要两个参数,我们需要指定新创建的函数将在其中运行的函数名称。 另外,我们可以使用args
设置提供一些参数,如下所示。
import multiprocessing
def foo(message):
print(message)
if __name__ == '__main__':
p = multiprocessing.Process(target=foo, args=("This is a spawn",))
p.start()
p = multiprocessing.Process(target=foo, args=("This is a spawn 2",))
p.start()
p = multiprocessing.Process(target=foo, args=("This is a spawn 3",))
p.start()

We can see that we have created 3 processes to spawn and with the start()
function we started them. We can not start a single new process multiple times so we have to define a new process with the Process()
function like above.
我们可以看到我们已经创建了3个生成的进程,并使用start()
函数启动了它们。 我们不能多次启动一个新进程,因此我们必须使用上面的Process()
函数定义一个新进程。
为创建的流程设置名称 (Set Name For Created Processes)
Multiprocessing provides different useful features like setting a name for a new process. We will use the name
option which will be provided to the Process()
function. We can access this name from the newly created process with the multiprocessing.current_process().name
attribute like below.
多重处理提供了各种有用的功能,例如为新进程设置名称。 我们将使用name
选项,该选项将提供给Process()
函数。 我们可以使用下面的multiprocessing.current_process().name
属性从新创建的进程中访问此名称。
import multiprocessing
def daemon(message):
print(multiprocessing.current_process().name)
print(message)
if __name__ == '__main__':
p = multiprocessing.Process(name="This process name is daemon", target=daemon, args=("This is a spawn",))
p.start()

创建守护进程(Create Daemon Processes)
When a normally created or spawned process completes its execution its ended. This is the normal behavior of all of the normal processes. But some special cases we need to run a process like a daemon or service where they will not stop even they have no task to complete. Daemons mainly wait for a specific event like connection, trigger some event to run. Python multiprocessing provides the daemon
option which will turn given process into a daemon which will run forever normally. But if the main process finishes the daemons will be excited too.
当正常创建或生成的进程完成其执行时,其结束。 这是所有正常过程的正常行为。 但是在某些特殊情况下,我们需要运行诸如守护程序或服务之类的进程,使它们即使没有任务完成也不会停止。 守护程序主要等待诸如连接之类的特定事件,触发某些事件运行。 Python多重处理提供了daemon
选项,该选项会将给定的进程转换为一个守护程序,该守护程序将永远正常运行。 但是,如果主进程完成,守护进程也将兴奋。
import multiprocessing
def daemon(message):
print(message)
if __name__ == '__main__':
p = multiprocessing.Process(target=daemon, args=("Waiting For Tasks",))
p.daemon = True
p.start()
print("This will not printed bec")
等待过程完成 (Wait For Process To Complete)
After the main process reached the end, the process will exit and all the child processes will stop. But we can change this behavior where the main process will wait for the child process to finish and exit. We will use join()
function where the specified child process exit will be waiting by the main process.
主进程结束后,该进程将退出,所有子进程将停止。 但是我们可以在主进程等待子进程完成并退出的情况下更改此行为。 我们将使用join()
函数,其中指定的子进程出口将由主进程等待。
import multiprocessing
def daemon(message):
print(message)
if __name__ == '__main__':
p = multiprocessing.Process(target=daemon, args=("Waiting For Tasks",))
p.daemon = True
p.start()
p.join()
print("This will not printed bec")
终止过程 (Terminate Process)
After a new child process is created it will exit after the task is completed. But In some cases, we may need to terminate the given child process before it finishes. We can use terminate()
function which will terminate the given process.
创建新的子进程后,它将在任务完成后退出。 但是在某些情况下,我们可能需要在给定的子进程完成之前终止它。 我们可以使用terminate()
函数来终止给定的进程。
import multiprocessing
def daemon(message):
print(message)
if __name__ == '__main__':
p = multiprocessing.Process(target=daemon, args=("Waiting For Tasks",))
p.daemon = True
p.start()
p.terminate()
print("Process Is Terminated")
检查给定的子进程正在运行还是正在运行 (Check If Given Child Process Is Running or Alive)
After creating a child process it will run according to its task or whether it is a daemon. We may need this process current situation whether it is running or alive from the main process. We will use is_alive()
function which will return boolean values True or False according to the child process situation.
创建子进程后,它将根据其任务或它是否是守护程序运行。 无论主进程正在运行还是处于活动状态,我们都可能需要此进程的当前状态。 我们将使用is_alive()
函数,该函数将根据子进程的情况返回布尔值True或False。
import multiprocessing
def daemon(message):
print(message)
if __name__ == '__main__':
p = multiprocessing.Process(target=daemon, args=("Waiting For Tasks",))
p.daemon = True
p.start()
print(p.is_alive())
打印过程退出状态 (Print Process Exit Status)
The process executes, completes, and exits. But the process will have some exit or finish reasons for different exit cases. For example, a process can be exit because it’s killed or it finishes its execution successfully. We can get or print the child process exit status with the exitcode
attribute.
该过程执行,完成并退出。 但是对于不同的退出情况,该过程将有一些退出或完成的原因。 例如,一个进程可能因为被杀死或成功完成执行而退出。 我们可以使用exitcode
属性获取或打印子进程的退出状态。
import multiprocessing
import time
def foo(message):
print(message)
if __name__ == '__main__':
p1 = multiprocessing.Process(target=foo, args=("Waiting For Tasks",))
p2 = multiprocessing.Process(target=foo, args=("Waiting For Tasks",))
p1.start()
p2.start()
p2.terminate()
time.sleep(3)
print(p1.exitcode)
print(p2.exitcode)

There are meaning about the exit status.
关于退出状态有一些含义。
- If equal to the 0 the process is executed and finished successfully without any error. 如果等于0,则该过程将成功执行并成功完成,没有任何错误。
- If bigger than 0 there is an error related to the code. 如果大于0,则与代码有关的错误。
- If lower than 0the process is killed with a signal. 如果小于0,则进程将被信号杀死。
记录多进程操作(Log Multiprocess Operations)
Every created process has some logging mechanism. We can redirect these logs into a file or standard output with the log_to_stferr(logging.DEBUG)
by specifying the level of the log verbosity which is DEBUG
in this case.
每个创建的进程都有一些日志记录机制。 通过指定日志详细程度(在这种情况下为DEBUG
log_to_stferr(logging.DEBUG)
,我们可以使用log_to_stferr(logging.DEBUG)
将这些日志重定向到文件或标准输出中。
def foo(message):
print(message)
if __name__ == '__main__':
multiprocessing.log_to_stderr(logging.DEBUG)
p1 = multiprocessing.Process(target=foo, args=("Waiting For Tasks",))
p2 = multiprocessing.Process(target=foo, args=("Waiting For Tasks",))
p1.start()
p2.start()
p2.terminate()
time.sleep(3)
print(p1.exitcode)
print(p2.exitcode)
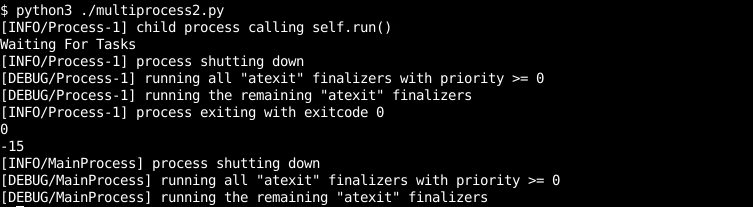
翻译自: https://www.poftut.com/multiprocessing-and-parallel-programming-in-python/