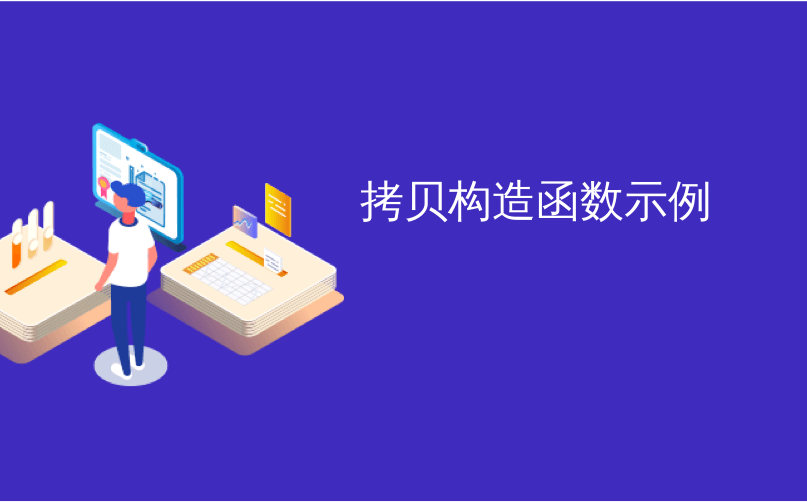
拷贝构造函数示例
A constructor is used in object-oriented programming languages in order to initialize an object which will be created from a class type. In C++ constructor is automatically called in order to initialize an object in different ways.
面向对象的编程语言中使用构造函数来初始化将由类类型创建的对象。 在C ++中,为了以不同方式初始化对象,会自动调用构造函数。
什么是构造函数? (What Is Constructor?)
A constructor is defined inside the class with the same name as the class. The name must be same with the class because it will be marked as a constructor automatically. The constructor should be public which means it should be put public definitions of the class. Constructor names are also case sensitive which should be the same case with the class name. The constructor also have the same name as the class.
在类内部定义了一个与该类同名的构造函数。 该名称必须与该类相同,因为它将被自动标记为构造函数。 构造函数应该是公共的,这意味着应该将其置于类的公共定义中。 构造函数名称也区分大小写,与类名称应相同。 构造函数也具有与类相同的名称。
构造函数如何工作? (How Does Constructor Work?)
The constructor will work like a function that will be called automatically during object initialization. The initialization can be done in different ways like below.
构造函数将像在对象初始化期间自动调用的函数一样工作。 初始化可以通过以下不同方式完成。
Point p = new Point();
Point p();
构造函数的类型 (Types Of Constructors)
Inside a class definition, we do not have to create a constructor explicitly where the compiler will create a default constructor implicitly which will just initialize the object. But we have different options in order to create constructor like the following:
在类定义内,我们不必显式创建构造函数,编译器将隐式创建默认构造函数,该构造函数将初始化对象。 但是,为了创建类似于以下内容的构造函数,我们有不同的选择:
- `Default Constructor` `默认构造函数`
- `Parameterized Constructor` 参数化构造函数
- `Dynamic Constructor`动态构造函数
默认构造函数(Default Constructor)
We will start with an explicit default constructor. We will put the Point()
constructor definition under the public:
part and we will also add curly braces { }
to create default constructor code block. We will put inside the default constructor code block the code we will run during object initialization or creation. The code is simple where we will set x and y variables to the 0.
我们将从一个显式的默认构造函数开始。 我们将把Point()
构造函数定义放在public:
部分下,还将添加花括号{ }
以创建默认的构造函数代码块。 我们将在默认的构造函数代码块中放入将在对象初始化或创建期间运行的代码。 代码很简单,我们将x和y变量设置为0。
// C++ Example Application To Explain Constructors
#include <iostream>
using namespace std;
class Point {
private:
int x, y;
public:
// Default Constructor
Point()
{
x = 0;
y = 0;
}
int getX()
{
return x;
}
int getY()
{
return y;
}
};
int main()
{
// Constructor called with a new object initilization from the Point class
Point p = Point();
// We can access values assigned by constructor
cout << "p.x = " << p.getX() << ", p.y = " << p.getY();
return 0;
}
参数化构造函数 (Parameterized Constructor)
In the previous example, we have just called the constructor to initialize the object. But we do not provide any value for the initialization. We can also use parameterized constructors in order to provide some values for the initialization which will set to the created object. A parameterized constructor is very same with the default constructor where we will add some parameters as arguments to the constructor function. In this following example, we will set the x and y by providing them to the constructor as value.
在前面的示例中,我们刚刚调用了构造函数来初始化对象。 但是我们不提供任何初始化值。 我们还可以使用参数化的构造函数,以便为初始化提供一些值,这些值将设置为创建的对象。 参数化构造函数与默认构造函数非常相似,在默认构造函数中,我们将向构造函数添加一些参数作为参数。 在下面的示例中,我们将x和y作为值提供给构造函数,以对其进行设置。
// C++ Example Application To Explain Constructors
#include <iostream>
using namespace std;
class Point {
private:
int x, y;
public:
// Default Constructor
Point(int new_x, int new_y)
{
x = new_x;
y = new_y;
}
int getX()
{
return x;
}
int getY()
{
return y;
}
};
int main()
{
// Constructor called with a new object initilization from the Point class
Point p = Point( 10 , 15 );
// We can access values assigned by constructor
cout << "p.x = " << p.getX() << ", p.y = " << p.getY();
return 0;
}
构造函数重载 (Constructor Overloading)
In the previous examples, we have defined only one constructor for each time but C++ provides the ability to define multiple constructors for different use cases. Defining multiple constructors for a single class is named constructor overloading. The most important restriction about constructor overloading is the same signature can not be used multiple times. This means the same parameter count and types can not be used multiple times. Let’s look to the example where we will define a constructor which do not requires a parameter and the second constructor which accepts two parameters.
在前面的示例中,我们每次仅定义一个构造函数,但是C ++提供了针对不同用例定义多个构造函数的功能。 为单个类定义多个构造函数称为构造函数重载。 关于构造函数重载的最重要限制是同一签名不能多次使用。 这意味着相同的参数计数和类型不能多次使用。 让我们看一下该示例,在该示例中,我们将定义一个不需要参数的构造函数和第二个接受两个参数的构造函数。
We will use the following constructors:
我们将使用以下构造函数:
- `Point()` constructor will do not get any parameter where during initialization it will set x and y variables to the 0. Point()构造函数将不会获得任何参数,因为在初始化期间它将x和y变量设置为0。
- `Point(int new_x, int new_y)` will get `new_x` and `new_y` arguments and set their values to the x and y. Point(int new_x,int new_y)将获取new_x和new_y参数并将其值设置为x和y。
// C++ Example Application To Explain Constructors
#include <iostream>
using namespace std;
class Point {
private:
int x, y;
public:
// Default Constructor
Point()
{
x = 0;
y = 0;
}
Point(int new_x, int new_y)
{
x = new_x;
y = new_y;
}
int getX()
{
return x;
}
int getY()
{
return y;
}
};
int main()
{
//Initialize without parameter
Point p1 = Point();
//Initialize with parameters
Point p2 = Point( 10 , 15 );
// We can access values assigned by constructor
cout << "p.x = " << p1.getX() << ", p.y = " << p1.getY();
cout << "p.x = " << p2.getX() << ", p.y = " << p2.getY();
return 0;
}
复制构造函数 (Copy Constructor)
As from a single class, multiple objects can be created and initialized. In some cases, we may need to use already created object values to be used in the newly created object. We can do this by using copy constructors which will get the existing object pointer and copy the existing object values to the newly created object during initialization inside the constructor.
从单个类开始,可以创建和初始化多个对象。 在某些情况下,我们可能需要使用已创建的对象值以在新创建的对象中使用。 我们可以通过使用复制构造函数来做到这一点,该构造函数将获取现有对象指针,并在构造函数内部进行初始化期间将现有对象值复制到新创建的对象。
// C++ Example Application To Explain Constructors
#include <iostream>
using namespace std;
class Point {
private:
int x, y;
char* c;
public:
// Default Constructor
Point(int new_x, int new_y)
{
x = new_x;
y = new_y;
}
Point(const Point &p2)
{
x = p2.x;
y = p2.y;
}
int getX()
{
return x;
}
int getY()
{
return y;
}
};
int main()
{
// Constructor called with a new object initilization from the Point class
Point p = Point(10,15);
Point new_p = Point(p);
// We can access values assigned by constructor
cout << "p.x = " << new_p.getX() << ", p.y = " << new_p.getY();
return 0;
}
动态构造器 (Dynamic Constructor)
In general, during the object initialization process, the memory allocation sizes are fixed. Every type like int, char, object etc has specific sizes. But we can also allocate memory dynamically during the contructor call or initialization. In the following example, we will create a char array dynamically allocated. The char array c memory is allocated and then put point
to this variable.
通常,在对象初始化过程中,内存分配大小是固定的。 每个类型(如int,char,object等)都有特定的大小。 但是我们也可以在构造函数调用或初始化期间动态分配内存。 在下面的示例中,我们将创建一个动态分配的char数组。 分配char数组c内存,然后将其point
此变量。
// C++ Example Application To Explain Constructors
#include <iostream>
using namespace std;
class Point {
private:
int x, y;
char* c;
public:
// Default Constructor
Point()
{
c = new char[6];
c = "point";
x = 0;
y = 0;
}
int getX()
{
return x;
}
int getY()
{
return y;
}
};
int main()
{
// Constructor called with a new object initilization from the Point class
Point p = Point();
// We can access values assigned by constructor
cout << "p.x = " << p.getX() << ", p.y = " << p.getY();
return 0;
}
构造函数与成员函数 (Constructor vs Member Function)
As constructors act similar to the member function of the class you may ask yourself that what is the difference between constructor and member function or similarities. Here are some of the most important of them.
当构造函数的行为类似于类的成员函数时,您可能会问自己,构造函数和成员函数之间的区别或相似之处是什么? 以下是其中一些最重要的内容。
- Constructors do not have a return value or type where member functions do have a return value or type even it is void. 构造函数没有返回值或类型,而成员函数确实具有返回值或类型,即使它是无效的。
- Constructors are may be called automatically or explicitly according to usage where member functions are called explicitly. 构造函数可以根据用法自动或显式调用,其中成员函数显式调用。
- Constructors do have the same name with its class where member functions can/should have different names then it’s class 构造函数的类确实具有相同的名称,其中成员函数可以/应该具有与该类不同的名称
- Constructors are created automatically if we do not specify one by the compiler where member function does not create implicitly.如果编译器未在成员函数未隐式创建的情况下未指定构造函数,则会自动创建构造函数。
翻译自: https://www.poftut.com/cpp-constructor-tutorial-with-examples/
拷贝构造函数示例