异常是应用程序中可能发生的运行时错误。 如果异常处理不当,正在执行的程序将终止。 本文讨论了如何在ASP.Net Core MVC中实现全局错误处理,以及相关的代码示例和用于说明概念的屏幕图像。
若要使用本文中的代码示例,您应该在系统中安装Visual Studio 2019。 如果您还没有副本,则可以在此处下载Visual Studio 2019 。
在Visual Studio中创建一个ASP.Net Core MVC项目
首先,让我们在Visual Studio 2019中创建一个ASP.Net Core项目。假设系统中安装了Visual Studio 2019,请按照下面概述的步骤在Visual Studio中创建一个新的ASP.Net Core项目。
- 启动Visual Studio IDE。
- 点击“创建新项目”。
- 在“创建新项目”窗口中,从显示的模板列表中选择“ ASP.Net Core Web应用程序”。
- 点击下一步。
- 在“配置新项目”窗口中,指定新项目的名称和位置。
- (可选)选中“将解决方案和项目放在同一目录中”复选框。
- 单击创建。
- 在接下来显示的“创建新的ASP.Net Core Web应用程序”窗口中,从顶部的下拉列表中选择.Net Core作为运行时,并选择ASP.Net Core 2.2(或更高版本)。
- 选择“ Web应用程序(Model-View-Controller)”作为项目模板,以创建新的ASP.Net Core MVC应用程序。
- 确保未选中“启用Docker支持”和“配置HTTPS”复选框,因为我们此处将不再使用这些功能。
- 确保将身份验证设置为“无身份验证”,因为我们也不会使用身份验证。
- 单击创建。
按照这些步骤应该在Visual Studio中创建一个新的ASP.Net Core MVC项目。 我们将在以下各节中使用该项目来说明如何在ASP.Net Core MVC中处理空响应。
ASP.Net Core MVC中的全局异常处理选项
ASP.Net Core MVC内置了对全局异常处理的支持。 您可以利用全局异常处理中间件来在一个中央位置配置异常处理,从而减少本应在应用程序中编写的异常处理代码量。
在ASP.Net Core MVC中全局处理异常的第二种方法是使用异常筛选器。 您可以在上一篇文章中阅读有关异常过滤器的信息 。 在本文中,我们将使用内置的全局异常处理中间件和下一节中讨论的UseExceptionHandler方法。
在ASP.Net Core中使用UseExceptionHandler扩展方法
UseExceptionHandler是一个扩展方法,用于向请求处理管道注册ExceptionHandler中间件。
您可以使用IExceptionHandlerFeature接口检索异常对象。 下面的代码片段说明了UseExceptionHandler方法如何用于全局处理异常。
app.UseExceptionHandler(
builder =>
{
builder.Run(
async context =>
{
context.Response.StatusCode =
(int)HttpStatusCode.InternalServerError;
context.Response.ContentType =
"application/json";
var exception =
context.Features.Get
<IExceptionHandlerFeature>();
if (exception != null)
{
var error = new ErrorMessage()
{
Stacktrace =
exception.Error.StackTrace,
Message = exception.Error.Message
};
var errObj =
JsonConvert.SerializeObject(error);
await context.Response.WriteAsync
(errObj).ConfigureAwait(false);
}
});
}
);
这是ErrorMessage类供您参考。
public class ErrorMessage
{
public string Message { get; set; }
public string Stacktrace { get; set; }
}
在ASP.Net Core MVC中配置全局异常处理中间件
创建新的ASP.Net Core MVC应用程序后,Startup.cs文件将包含一个Configure方法,该方法配置全局异常处理中间件,如下面的代码片段所示。
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Error");
}
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseMvc(routes =>
{
routes.MapRoute(
name: "default",
template:
"{controller=Home}/{action=Index}/{id?}");
});
}
注意语句app.UseExceptionHandler("/Error");
在上面的代码片段中。 UseExceptionHandler方法注册ExceptionHandler中间件,然后在应用程序中出现未处理的错误时将用户路由到"/Error"
。
您可以利用UseStatusCodePagesWithReExecute扩展方法将状态代码页添加到管道中。 您也可以使用此方法来处理HTTP 404错误。 以下代码片段显示了更新的Configure方法。
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Error");
app.UseStatusCodePagesWithReExecute("/Error/NotFound/{0}");
}
//Other code
}
在ASP.Net Core MVC中使用ErrorController类
请注意,在HomeController类中,您将找到一个处理错误的操作方法。 我们不会使用此操作方法,因此您可以继续删除它。 这是ErrorController类,其中包含"/Error"
路由的操作方法。
public class ErrorController : Controller
{
[HttpGet("/Error")]
public IActionResult Index()
{
IExceptionHandlerPathFeature
iExceptionHandlerFeature =
HttpContext.Features.Get
<IExceptionHandlerPathFeature>();
if (iExceptionHandlerFeature != null)
{
string path = iExceptionHandlerFeature.Path;
Exception exception =
iExceptionHandlerFeature.Error;
//Write code here to log the exception details
return View("Error",
iExceptionHandlerFeature);
}
return View();
}
[HttpGet("/Error/NotFound/{statusCode}")]
public IActionResult NotFound(int statusCode)
{
var iStatusCodeReExecuteFeature =
HttpContext.Features.Get
<IStatusCodeReExecuteFeature>();
return View("NotFound",
iStatusCodeReExecuteFeature.OriginalPath);
}
}
您可以利用IExceptionHandlerPathFeature来检索异常元数据。 您还可以利用IStatusCodeReExecuteFeature检索发生HTTP 404异常的路径。 若要利用IExceptionHandlerPathFeature和IStatusCodeReExecuteFeature,请确保在项目中安装了Microsoft.AspNetCore.Diagnostics NuGet包。 以下语句说明了如何检索发生异常的路由。
string route = iExceptionHandlerFeature.Path;
要检索异常详细信息,您可以编写以下代码。
var exception = HttpContext.Features.Get<IExceptionHandlerPathFeature>();
检索到路由和异常详细信息后,您就可以编写自己的代码来记录详细信息。
创建视图以在ASP.Net Core MVC中显示错误消息
您需要一个视图来显示错误消息。 这是“错误”视图页面的代码。
@model Microsoft.AspNetCore.Diagnostics.IExceptionHandlerFeature
@{
ViewData["Title"] = "Index";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<div class="row">
<div class="text-danger">
<h3>Error: @Model.Error.Message</h3>
</div>
</div>
<div class="row">
<div class="col-12">
<p>@Model.Error.StackTrace</p>
<p>@Model.Error.InnerException</p>
</div>
</div>
这是NotFound视图页面的代码。
@model string
@{
ViewData["Title"] = "NotFound";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h1 class="text-danger">
Error: The requested URL @Model was not found!</h1>
<hr />
现在,当您执行应用程序时,应该分别看到以下输出的全局异常和HTTP 404错误。
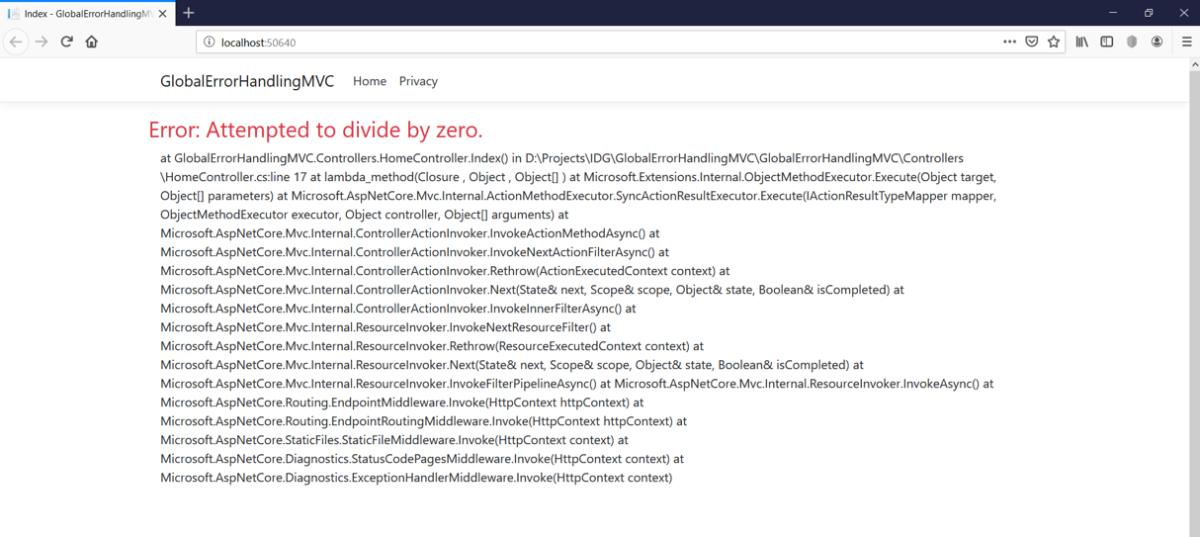
图1:在ASP.Net Core MVC中全局处理的异常。
并且,这是如果您尝试浏览不存在的URL时将如何处理HTTP 404错误的方法。
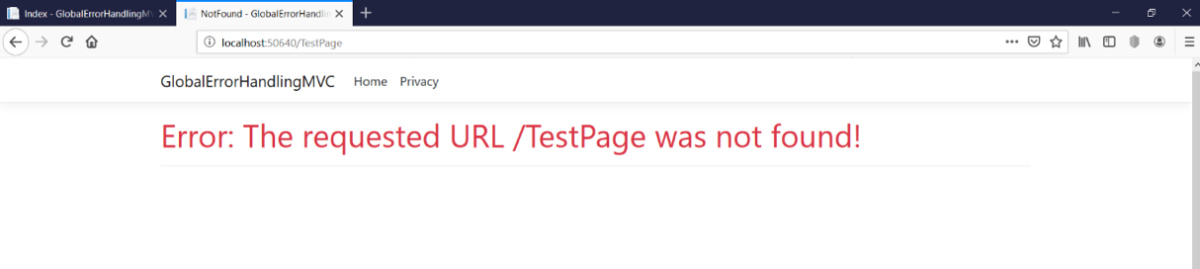
图2:在ASP.Net Core MVC中全局处理的HTTP 404错误。
ASP.Net Core内置了对全局异常处理的支持。 您可以利用ASP.Net Core中的全局异常处理来在应用程序的中央位置处理所有异常,并根据运行该应用程序的环境(开发,生产等)不同地处理异常。