这是向您展示如何通过JDBC将JSF 2.0与数据库集成的指南。 在此示例中,我们使用MySQL数据库和Tomcat Web容器。
本示例的目录结构
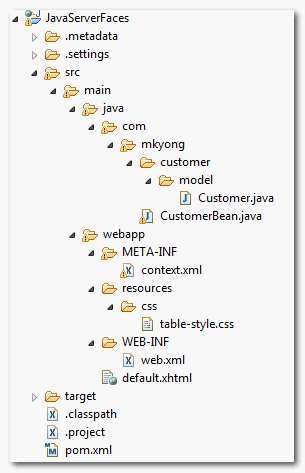
1.表结构
创建一个“ customer ”表,并插入五个虚拟记录。 稍后,通过JSF h:dataTable
显示它。
SQL命令
DROP TABLE IF EXISTS `mkyongdb`.`customer`;
CREATE TABLE `mkyongdb`.`customer` (
`CUSTOMER_ID` bigint(20) UNSIGNED NOT NULL AUTO_INCREMENT,
`NAME` varchar(45) NOT NULL,
`ADDRESS` varchar(255) NOT NULL,
`CREATED_DATE` datetime NOT NULL,
PRIMARY KEY (`CUSTOMER_ID`)
) ENGINE=InnoDB AUTO_INCREMENT=17 DEFAULT CHARSET=utf8;
insert into mkyongdb.customer(customer_id, name, address, created_date)
values(1, 'mkyong1', 'address1', now());
insert into mkyongdb.customer(customer_id, name, address, created_date)
values(2, 'mkyong2', 'address2', now());
insert into mkyongdb.customer(customer_id, name, address, created_date)
values(3, 'mkyong3', 'address3', now());
insert into mkyongdb.customer(customer_id, name, address, created_date)
values(4, 'mkyong4', 'address4', now());
insert into mkyongdb.customer(customer_id, name, address, created_date)
values(5, 'mkyong5', 'address5', now());
2. MySQL数据源
配置名为“ jdbc / mkyongdb ”的MySQL数据源,请按照本文– 如何在Tomcat 6中配置MySQL数据源
3.模型类
创建一个“ 客户 ”模型类来存储表记录。
文件:Customer.java
package com.mkyong.customer.model;
import java.util.Date;
public class Customer{
public long customerID;
public String name;
public String address;
public Date created_date;
//getter and setter methods
}
4. JDBC示例
一个JSF 2.0托管bean,通过@Resource
注入数据源“ jdbc / mkyongdb ”,并使用常规JDBC API从数据库中检索所有客户记录并将其存储到List中。
文件:CustomerBean.java
package com.mkyong;
import java.io.Serializable;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Resource;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.SessionScoped;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
import com.mkyong.customer.model.Customer;
@ManagedBean(name="customer")
@SessionScoped
public class CustomerBean implements Serializable{
//resource injection
@Resource(name="jdbc/mkyongdb")
private DataSource ds;
//if resource injection is not support, you still can get it manually.
/*public CustomerBean(){
try {
Context ctx = new InitialContext();
ds = (DataSource)ctx.lookup("java:comp/env/jdbc/mkyongdb");
} catch (NamingException e) {
e.printStackTrace();
}
}*/
//connect to DB and get customer list
public List<Customer> getCustomerList() throws SQLException{
if(ds==null)
throw new SQLException("Can't get data source");
//get database connection
Connection con = ds.getConnection();
if(con==null)
throw new SQLException("Can't get database connection");
PreparedStatement ps
= con.prepareStatement(
"select customer_id, name, address, created_date from customer");
//get customer data from database
ResultSet result = ps.executeQuery();
List<Customer> list = new ArrayList<Customer>();
while(result.next()){
Customer cust = new Customer();
cust.setCustomerID(result.getLong("customer_id"));
cust.setName(result.getString("name"));
cust.setAddress(result.getString("address"));
cust.setCreated_date(result.getDate("created_date"));
//store all data into a List
list.add(cust);
}
return list;
}
}
5. JSF页面数据表
JSF 2.0 xhtml页面使用h:dataTable
以表布局格式显示所有客户记录。
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
>
<h:head>
<h:outputStylesheet library="css" name="table-style.css" />
</h:head>
<h:body>
<h1>JSF 2.0 + JDBC Example</h1>
<h:dataTable value="#{customer.getCustomerList()}" var="c"
styleClass="order-table"
headerClass="order-table-header"
rowClasses="order-table-odd-row,order-table-even-row"
>
<h:column>
<f:facet name="header">
Customer ID
</f:facet>
#{c.customerID}
</h:column>
<h:column>
<f:facet name="header">
Name
</f:facet>
#{c.name}
</h:column>
<h:column>
<f:facet name="header">
Address
</f:facet>
#{c.address}
</h:column>
<h:column>
<f:facet name="header">
Created Date
</f:facet>
#{c.created_date}
</h:column>
</h:dataTable>
</h:body>
</html>
6.演示
运行它,查看输出
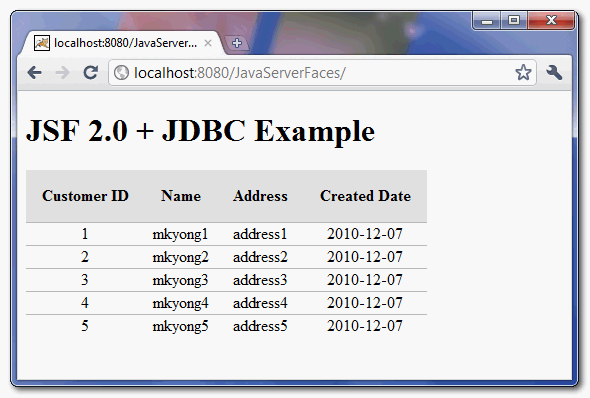
下载源代码
下载它– JSF-2-JDBC-Integration-Example.zip (12KB)
参考
翻译自: https://mkyong.com/jsf2/jsf-2-0-jdbc-integration-example/