在这篇文章中,我将分享我的React Native项目结构,配置和一些技巧。 它包含了我在使用React Native进行1年的开发后学到的大多数东西,从创建到发行。
我创建了一个名为typescript-react-native-starter的Github存储库,该存储库现在用于我的所有项目。
因此,我希望它对使用React Native的其他新开发人员有所帮助。 欢迎您成为PR:D
特征
经过数个项目使我了解类型变量的重要性之后,我最近开始使用Typescript。 一开始可能需要花费一些时间来学习,但这是值得的。 借助Typescript,您可以避免数小时的调试工作。
另外,它还使您的代码能够自动记录文档,这对于具有多个开发人员的项目至关重要。
打字稿
助焊剂状态管理
Redux :可预测状态容器
Redux Persist :离线商店
Redux Saga : Redux的副作用模型
typesafe-actions :轻松创建类型安全动作
import { action } from 'typesafe-actions' ;
import * as types from './actionTypes' ;
export const myAction = payload => action(types.MY_ACTION_TYPE, payload);
导航
React Navigation :基于Java的易于使用的导航解决方案
单元测试
Codecov :覆盖率报告
CI / CD
运行掉毛预提交和单元测试预推用沙哑的钩
占位符应用程序图标:用于使用Fastlane快速将您的应用程序上载到Beta版
应用图标生成器 :生成所有需要的尺寸,标签和注释图标。占位符功能图形和屏幕截图可快速上传Beta版Android应用
林亭
使用Airbnb样式配置的Tslint
兼容Vscode Prettier
国际化和本地化
react-native-localization :易于使用的i18n软件包
其他
Cocoapods :iOS依赖项管理器
jetifier :用于React Native 0.60 AndroidX迁移的过渡工具
autobind-decorator :用装饰器轻松绑定组件的功能
// Before
handleClick() {...}
<button onClick={ this .handleClick.bind( this ) }> </ button >
// After
@boundMethod
handleClick() {...}
<button onClick={ this .handleClick }> </ button >
项目结构
我使用的结构灵感来自许多来源,因此您可能会对此感到熟悉。 除了某些特殊文件(例如App.tsx,store.ts等),我喜欢按类别将文件分开。
发布文件夹还包含一些有用的占位符图像,用于部署您的应用程序。
例如,为了在Google Play上部署您的应用程序,甚至进行内部测试,您都必须添加屏幕截图,功能图片等。起初还可以,但是经过几个项目,这有点烦人,所以我决定创建一些占位符图像。
├── __tests__ // Unit tests
│ ├── App.test.tsx // App component's tests
│ ├── components
│ │ └── MyComponent.test.txs
│ └── ...
├── android
├── app.json
├── assets // All assets: images, videos, ...
├── index.js
├── ios
├── publishing // Icon, screenshots, preview,... for App Store & Play Store
└── src
├── App.tsx
├── actions // Actions
│ ├── actionTypes.ts // Action types
│ └── app.ts // appReducer's actions
├── components // Components
│ └── MyComponent.tsx
├── constants // Colors, sizes, routes,...
│ └── strings.ts // i18n
├── containers // Screens, pages,...
├── lib // Libraries, services,...
├── index.tsx // Root component
├── reducers // Reducers
│ └── app.ts // appReducer
├── sagas // Redux sagas
├── store.ts
├── types // Type declarations
│ └── index.d.ts
└── utils // Utilities
有用的提示
本部分提供了完全随机但有用的提示,请随时在评论中分享您的观点或进行PR
NavigationService
你可以没有导航道具导航使用的NavigationService从SRC / lib中/ NavigationService.ts
import NavigationService from '../lib/NavigationService' ;
//...
NavigationService.navigate( 'ChatScreen' , { userName : 'Lucy' });
可可足
当您运行react-native链接并且链接的库具有podspec文件时,则链接将使用Podfile。 要禁用此功能,请删除
# Add new pods below this line
从ios / Podfile中的第24行开始
静态捆绑
每当您将物理设备作为目标时,都会生成静态捆绑包,即使在调试中也是如此。 为了节省时间, 在调试中禁用了捆绑包的生成
反应本机屏幕
您可以将react-native-screens与react-navigation一起使用,以提高内存消耗
从react-native屏幕上安装并遵循使用带有反应导航(无Expo)的步骤
打开./src/index.tsx并取消注释
// import { useScreens } from 'react-native-screens';
// useScreens();
反应性
尽量避免“绝对”位置和硬值(100、300、1680等),特别是对于大的位置和硬值。请改用flex box和%值。如果必须使用硬值,则可以使用此normalize函数来适应硬值对应于屏幕的宽度或高度。 我可以稍后将其上传到存储库中:
import { Dimensions, Platform, PixelRatio } from 'react-native' ;
export const { width : SCREEN_WIDTH, height : SCREEN_HEIGHT } = Dimensions.get(
'window' ,
);
// based on iphone X's scale
const wscale = SCREEN_WIDTH / 375 ;
const hscale = SCREEN_HEIGHT / 812 ;
export function normalize ( size, based = 'width' ) {
const newSize = based === 'height' ? size * hscale : size * wscale;
if (Platform.OS === 'ios' ) {
return Math .round(PixelRatio.roundToNearestPixel(newSize));
} else {
return Math .round(PixelRatio.roundToNearestPixel(newSize)) - 2 ;
}
}
所以现在我可以使用:
// iphone X
normalize( 100 ) // = 100
// iphone 5s
normalize( 100 ) // = maybe 80
// You can choose either "width" (default) or "height" depend on cases:
container = {
width : normalize( 100 , "width" ), // "width" is optional, it's default
height: normalize( 100 , "height" )
}
推送之前,请在3种不同的模拟器上测试您的应用程序:iphone5s(小),iphone 8(中)和iphone Xs Max(大)
Fastlane的Beta发行版
-安装快速通道
# Using RubyGems
sudo gem install fastlane -NV
# Alternatively using Homebrew
brew cask install fastlane
的iOS
-打开您的项目Xcode工作区并更新应用程序的捆绑包标识符和团队
-初始化快速通道
cd <PROJECT_NAME>/ios
fastlane init
-分发您的应用
fastlane beta
安卓系统
-使用Android Studio打开项目,并在build.gradle(模块:app)文件中更新应用的applicationId
-从“生成”菜单中选择“生成的签名包/ APK ...”
-下一步,然后在密钥存储路径下创建新...,然后单击下一步和完成
-首次部署应用程序时,必须手动将其上传到Google Play控制台。 Google不允许使用其API进行首次上传。请在Google Play控制台中创建您的应用程序(与iOS Fastlane不同,它无法为您完成此操作)
-确保这四个复选标记图标为绿色
推荐顺序:定价和发行,内容分级,商店详情和应用发布
您可以在Publishing / android文件夹中找到商店列表所需的资产
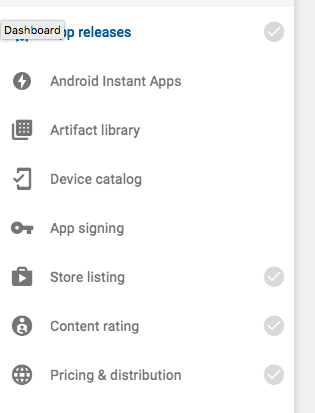
-初始化快速通道
cd <PROJECT_NAME>/android
fastlane init
-使用发布的Fastfile
cp publishing/android/fastlane/Fastfile android/fastlane
-分发您的应用
没有官方插件可以自动升级android版本代码(与iOS通道不同)。
在每次部署之前,请确保手动升级android / app / build.gradle中的versionCodevalue。
更多
-查看Fastlane的Beta发行指南 ,了解更多详细信息
-Fastlane的 React Native 文档
Apple Store Connect缺少合规性
如果您不使用Fastlane,并且不想在每次推送时都 提供Export Compliance Information ,则将其添加到Info.plist
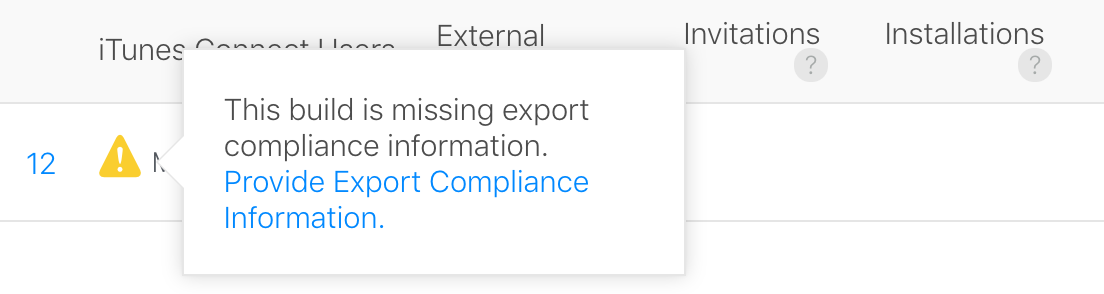
请注意,如果您的应用程序使用加密,则可能必须将其设置为<true />
From: https://hackernoon.com/my-react-native-stack-after-1-year-ctls30z9