EditText方框验证码
项目中有这样一个需求:
验证码页是四个方框,输入验证码方框颜色改变,删除再变回原来颜色。
先看下效果,动图不太清晰,将就看吧
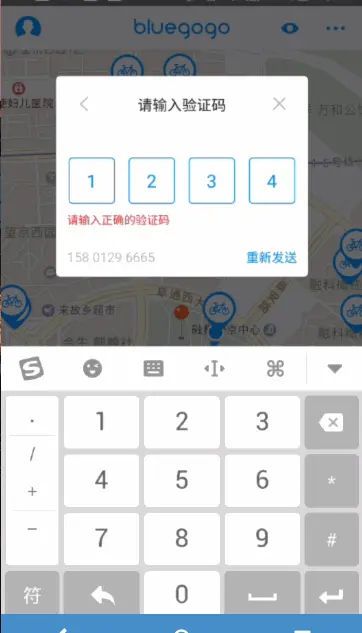
效果.gif
思路:
1.用一个透明的EditText与四个TextView重叠,并给TextView设置默认背景
2.监听EditText文本变化,获取输入内容,给TextView赋值并改变TextView背景
3. 4个TextView有值后添加输入完成回调,监听删除键添加删除回调
代码:
private EditText editText;
private TextView[] TextViews;
private StringBuffer stringBuffer = new StringBuffer();
private int count = 4;
private String inputContent;
public SecurityCodeView(Context context) {
this(context, null);
}
public SecurityCodeView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public SecurityCodeView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
TextViews = new TextView[4];
View.inflate(context, R.layout.view_security_code, this);
editText = (EditText) findViewById(R.id.item_edittext);
TextViews[0] = (TextView) findViewById(R.id.item_code_iv1);
TextViews[1] = (TextView) findViewById(R.id.item_code_iv2);
TextViews[2] = (TextView) findViewById(R.id.item_code_iv3);
TextViews[3] = (TextView) findViewById(R.id.item_code_iv4);
editText.setCursorVisible(false);//将光标隐藏
setListener();
}
private void setListener() {
editText.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence charSequence, int i, int i1, int i2) {
}
@Override
public void onTextChanged(CharSequence charSequence, int i, int i1, int i2) {
}
@Override
public void afterTextChanged(Editable editable) {
//重点 如果字符不为""时才进行操作
if (!editable.toString().equals("")) {
if (stringBuffer.length() > 3) {
//当文本长度大于3位时edittext置空
editText.setText("");
return;
} else {
//将文字添加到StringBuffer中
stringBuffer.append(editable);
editText.setText("");//添加后将EditText置空
// Log.e("TAG", "afterTextChanged: stringBuffer is " + stringBuffer);
count = stringBuffer.length();//记录stringbuffer的长度
inputContent = stringBuffer.toString();
if (stringBuffer.length() == 4) {
//文字长度位4 则调用完成输入的监听
if (inputCompleteListener != null) {
inputCompleteListener.inputComplete();
}
}
}
for (int i = 0; i < stringBuffer.length(); i++) {
TextViews[i].setText(String.valueOf(inputContent.charAt(i)));
TextViews[i].setBackgroundResource(R.mipmap.bg_verify_press);
}
}
}
});
editText.setOnKeyListener(new OnKeyListener() {
@Override
public boolean onKey(View v, int keyCode, KeyEvent event) {
if (keyCode == KeyEvent.KEYCODE_DEL
&& event.getAction() == KeyEvent.ACTION_DOWN) {
if (onKeyDelete()) return true;
return true;
}
return false;
}
});
}
public boolean onKeyDelete() {
if (count == 0) {
count = 4;
return true;
}
if (stringBuffer.length() > 0) {
//删除相应位置的字符
stringBuffer.delete((count - 1), count);
count--;
// Log.e(TAG, "afterTextChanged: stringBuffer is " + stringBuffer);
inputContent = stringBuffer.toString();
TextViews[stringBuffer.length()].setText("");
TextViews[stringBuffer.length()].setBackgroundResource(R.mipmap.bg_verify);
if (inputCompleteListener != null)
inputCompleteListener.deleteContent(true);//有删除就通知manger
}
return false;
}
/**
* 清空输入内容
*/
public void clearEditText() {
stringBuffer.delete(0, stringBuffer.length());
inputContent = stringBuffer.toString();
for (int i = 0; i < TextViews.length; i++) {
TextViews[i].setText("");
TextViews[i].setBackgroundResource(R.mipmap.bg_verify);
}
}
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
return super.onKeyDown(keyCode, event);
}
private InputCompleteListener inputCompleteListener;
public void setInputCompleteListener(InputCompleteListener inputCompleteListener) {
this.inputCompleteListener = inputCompleteListener;
}
public interface InputCompleteListener {
void inputComplete();
void deleteContent(boolean isDelete);
}
/**
* 获取输入文本
*
* @return
*/
public String getEditContent() {
return inputContent;
}
布局
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="47dp"
android:gravity="center"
android:orientation="horizontal"
android:weightSum="3">
<TextView
android:id="@+id/item_code_iv1"
style="@style/text_editStyle" />
<View
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/item_code_iv2"
style="@style/text_editStyle" />
<View
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/item_code_iv3"
style="@style/text_editStyle" />
<View
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<TextView
android:id="@+id/item_code_iv4"
style="@style/text_editStyle" />
</LinearLayout>
<EditText
android:id="@+id/item_edittext"
android:layout_width="match_parent"
android:layout_height="46dp"
android:background="@android:color/transparent"
android:inputType="number" />
</RelativeLayout>
使用:
<com.xiaviv.securitycodedemo.SecurityCodeView
android:id="@+id/scv_edittext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:layout_marginTop="20dp" />
<TextView
android:id="@+id/tv_text"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:layout_marginRight="20dp"
android:layout_marginTop="10dp"
android:layout_weight="4"
android:text="输入验证码表示同意《用户协议》" />
public class MainActivity extends AppCompatActivity implements SecurityCodeView.InputCompleteListener {
private SecurityCodeView editText;
private TextView text;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
findViews();
setListener();
}
private void setListener() {
editText.setInputCompleteListener(this);
}
private void findViews() {
editText = (SecurityCodeView) findViewById(R.id.scv_edittext);
text = (TextView) findViewById(R.id.tv_text);
}
@Override
public void inputComplete() {
Toast.makeText(getApplicationContext(), "验证码是:" + editText.getEditContent(), Toast.LENGTH_LONG).show();
if (!editText.getEditContent().equals("1234")) {
text.setText("验证码输入错误");
text.setTextColor(Color.RED);
}
}
@Override
public void deleteContent(boolean isDelete) {
if (isDelete){
text.setText("输入验证码表示同意《用户协议》");
text.setTextColor(Color.BLACK);
}
}
}