Problem Description
There was no donkey in the province of Gui Zhou, China. A trouble maker shipped one and put it in the forest which could be considered as an N×N grid. The coordinates of the up-left cell is (0,0) , the down-right cell is (N-1,N-1) and the cell below the up-left cell is (1,0)..... A 4×4 grid is shown below:
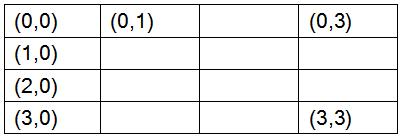
The donkey lived happily until it saw a tiger far away. The donkey had never seen a tiger ,and the tiger had never seen a donkey. Both of them were frightened and wanted to escape from each other. So they started running fast. Because they were scared, they were running in a way that didn't make any sense. Each step they moved to the next cell in their running direction, but they couldn't get out of the forest. And because they both wanted to go to new places, the donkey would never stepped into a cell which had already been visited by itself, and the tiger acted the same way. Both the donkey and the tiger ran in a random direction at the beginning and they always had the same speed. They would not change their directions until they couldn't run straight ahead any more. If they couldn't go ahead any more ,they changed their directions immediately. When changing direction, the donkey always turned right and the tiger always turned left. If they made a turn and still couldn't go ahead, they would stop running and stayed where they were, without trying to make another turn. Now given their starting positions and directions, please count whether they would meet in a cell.
Input
There are several test cases. In each test case: First line is an integer N, meaning that the forest is a N×N grid. The second line contains three integers R, C and D, meaning that the donkey is in the cell (R,C) when they started running, and it's original direction is D. D can be 0, 1, 2 or 3. 0 means east, 1 means south , 2 means west, and 3 means north. The third line has the same format and meaning as the second line, but it is for the tiger. The input ends with N = 0. ( 2 <= N <= 1000, 0 <= R, C < N)
Output
For each test case, if the donkey and the tiger would meet in a cell, print the coordinate of the cell where they meet first time. If they would never meet, print -1 instead.
Sample Input
2 0 0 0 0 1 2 4 0 1 0 3 2 0 0
Sample Output
-1 1 3
Source
题意:
在一个N*N的方格里,有一只驴和一只虎,两者以相同的速度(一格一格地走)同时开始走。走法是:往东南西北某一个初始方向走,两者都不能重复走自己走过的路,但是对方走过的路自己可以走,如果遇到墙壁或者自己走过的路,则驴向右转,虎向左转,如果还不能继续往前走,就停在原地不动。如果驴和老虎能同一时间在一个格子里面相遇,则输出坐标,否则输出-1 。
分析:
深搜。
1)当两者坐标相同时,相遇;
2)两者同时在不同地方都停下时,肯定不相遇;
3)如果有一个停下了,记录当前坐标,否则往初始方向继续遍历。


1 #pragma comment(linker, "/STACK:1024000000,1024000000") 2 #include<iostream> 3 #include<cstdio> 4 #include<cstring> 5 #include<cmath> 6 #include<math.h> 7 #include<algorithm> 8 #include<queue> 9 #include<set> 10 #include<bitset> 11 #include<map> 12 #include<vector> 13 #include<stdlib.h> 14 #include <stack> 15 using namespace std; 16 #define PI acos(-1.0) 17 #define max(a,b) (a) > (b) ? (a) : (b) 18 #define min(a,b) (a) < (b) ? (a) : (b) 19 #define ll long long 20 #define eps 1e-10 21 #define MOD 1000000007 22 #define N 1006 23 #define inf 1e12 24 int n; 25 int flag; 26 int p,q; 27 int dirx[]={0,1,0,-1}; 28 int diry[]={1,0,-1,0}; 29 int vis1[N][N],vis2[N][N]; 30 void dfs(int a,int b,int c,int x,int y,int z){ 31 vis1[a][b]=1; 32 vis2[x][y]=1; 33 if(flag==1){ 34 return; 35 } 36 if(a==x && b==y){ 37 flag=1; 38 printf("%d %d\n",a,b); 39 return; 40 } 41 42 if(p && q){ 43 flag=1; 44 printf("-1\n"); 45 return; 46 } 47 int aa,bb,xx,yy; 48 49 if(p){ 50 aa=a; 51 bb=b; 52 }else{ 53 aa=a+dirx[c]; 54 bb=b+diry[c]; 55 if(aa<0 || aa>=n || bb<0 || bb>=n || vis1[aa][bb]==1){ 56 c=(c+1)%4; 57 aa=a+dirx[c]; 58 bb=b+diry[c]; 59 if(aa<0 || aa>=n || bb<0 || bb>=n || vis1[aa][bb]==1){ 60 p=1; 61 aa=a; 62 bb=b; 63 } 64 } 65 } 66 67 if(q){ 68 xx=x; 69 yy=y; 70 }else{ 71 xx=x+dirx[z]; 72 yy=y+diry[z]; 73 if(xx<0 || xx>=n || yy<0 || yy>=n || vis2[xx][yy]==1){ 74 z=(z-1+4)%4; 75 xx=x+dirx[z]; 76 yy=y+diry[z]; 77 if(xx<0 || xx>=n || yy<0 || yy>=n || vis2[xx][yy]==1){ 78 q=1; 79 xx=x; 80 yy=y; 81 } 82 } 83 } 84 dfs(aa,bb,c,xx,yy,z); 85 } 86 int main() 87 { 88 while(scanf("%d",&n)==1){ 89 if(n==0){ 90 break; 91 } 92 memset(vis1,0,sizeof(vis1)); 93 memset(vis2,0,sizeof(vis2)); 94 int a,b,c,x,y,z; 95 scanf("%d%d%d",&a,&b,&c); 96 scanf("%d%d%d",&x,&y,&z); 97 if(a==x && b==y){ 98 printf("%d %d\n",a,b); 99 continue; 100 } 101 flag=0; 102 p=q=0; 103 dfs(a,b,c,x,y,z); 104 105 } 106 return 0; 107 }