import java.io.File;
import java.io.IOException;
import java.io.Writer;
import java.util.Map;
import org.hamcrest.CoreMatchers;
import org.junit.Assert;
import com.google.common.base.Charsets;
import com.google.common.base.Joiner;
import com.google.common.collect.Maps;
import com.google.common.io.CharSink;
import com.google.common.io.Files;
/**
* TODO 在此写上类的相关说明.<br>
* @author gqlpp<br>
* @version 1.0.0 2021年11月11日<br>
* @see
* @since JDK 1.5.0
*/
public class JoinerDemo {
/**
* @param args
*/
public static void main(String[] args) throws Exception {
throwNullException();
skipNull();
replaceNull();
appendToBuilder();
appendToWriter();
joinMap();
}
/**
* null-默认异常.
*/
static void throwNullException() {
try {
String[] values = new String[]{"foo",null,"bar"};
Joiner.on("#").join(values);//NullPointerException
} catch (NullPointerException e) {
System.out.println("NullPointerException");
}
}
/**
* 跳过null.
*/
static void skipNull() {
String[] values = new String[]{"foo",null,"bar"};
String returned = Joiner.on("#").skipNulls().join(values);
Assert.assertThat(returned, CoreMatchers.is("foo#bar"));
}
/**
* 替换null.
*/
static void replaceNull() {
String[] values = new String[]{"foo",null,"bar"};
String returned = Joiner.on("#").useForNull("missing").join(values);
Assert.assertThat(returned, CoreMatchers.is("foo#missing#bar"));
}
/**
* 添加到Builder.
*/
static void appendToBuilder() {
String[] values = new String[]{"foo", "bar","baz"};
StringBuilder builder = new StringBuilder();
StringBuilder returned = Joiner.on("|").appendTo(builder, values);
Assert.assertThat(returned.toString(), CoreMatchers.is("foo|bar|baz"));
}
/**
* 添加到Writer.
* @throws IOException
*/
static void appendToWriter() throws IOException {
File tempFile = new File("d:/data/temp.txt");
CharSink charSink = Files.asCharSink(tempFile, Charsets.UTF_8);
Writer writer = charSink.openStream();
String[] values = new String[]{"foo", "bar","baz"};
Joiner.on("|").appendTo(writer, values);
writer.close();
String fromFileString = Files.toString(tempFile, Charsets.UTF_8);
Assert.assertThat(fromFileString, CoreMatchers.is("foo|bar|baz"));
}
/**
* 拼接Map.
*/
static void joinMap() {
String expectedString = "Washington D.C=Redskins#New York City=Giants#Philadelphia=Eagles#Dallas=Cowboys";
Map<String, String> testMap = Maps.newLinkedHashMap();
testMap.put("Washington D.C", "Redskins");
testMap.put("New York City", "Giants");
testMap.put("Philadelphia", "Eagles");
testMap.put("Dallas", "Cowboys");
String returnedString = Joiner.on("#").withKeyValueSeparator("=").join(testMap);
Assert.assertThat(returnedString, CoreMatchers.is(expectedString));
}
}
Guava入门~Joiner
最新推荐文章于 2023-12-25 15:44:55 发布
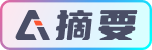