1)显示我设定的目录
QFileSystemModel *pModel = new QFileSystemModel();
pModel->setReadOnly(false);
pModel->setRootPath("C:\\zib");
ui->treeView->setModel(pModel);
ui->treeView->header()->setStretchLastSection(true);
ui->treeView->header()->setSortIndicator(0,Qt::AscendingOrder);
ui->treeView->header()->setSortIndicatorShown(true);
QModelIndex index = pModel->index("C:\\zib");
ui->treeView->setRootIndex(index);
ui->treeView->expand(index);
ui->treeView->scrollTo(index);
ui->treeView->resizeColumnToContents(0);
以下转载的别人的,等以后有事件再看看
转载1
QFileSystemModel 类似QDitModel,只不过Qt不推荐使用QDirModel,推荐是使用QFileSystemModel,该模型允许我们在view中显示操作系统的目录结构。
directoryviewer.h文件:
#ifndef DIRECTORYVIEWER_H
#define DIRECTORYVIEWER_H
#include <QtGui/QDialog>
#include <QFileSystemModel>
#include <QTreeView>
class DirectoryViewer : public QDialog
{
Q_OBJECT
public:
DirectoryViewer(QWidget *parent = 0);
~DirectoryViewer();
private slots:
void createDirectory();
void remove();
private:
QFileSystemModel *model;
QTreeView *treeView;
};
#endif
directoryviewer.cpp文件:
#include "directoryviewer.h"
#include <QPushButton>
#include <QVBoxLayout>
#include <QHBoxLayout>
#include <QHeaderView>
#include <QInputDialog>
#include <QMessageBox>
DirectoryViewer::DirectoryViewer(QWidget *parent)
: QDialog(parent)
{
model = new QFileSystemModel;
model->setReadOnly(false); //设置可以修改
model->setRootPath(QDir::currentPath());
treeView = new QTreeView;
treeView->setModel(model);
treeView->header()->setStretchLastSection(true);
treeView->header()->setSortIndicator(0, Qt::AscendingOrder);
treeView->header()->setSortIndicatorShown(true);
treeView->header()->setClickable(true);
QModelIndex index = model->index(QDir::currentPath());
treeView->expand(index); //当前项展开
treeView->scrollTo(index); //定位到当前项
treeView->resizeColumnToContents(0);
QPushButton *createButton = new QPushButton("Create Directory", this);
QPushButton *removeButton = new QPushButton("Remove", this);
QHBoxLayout *hlayout = new QHBoxLayout;
hlayout->addWidget(createButton);
hlayout->addWidget(removeButton);
QVBoxLayout *vlayout = new QVBoxLayout;
vlayout->addWidget(treeView);
vlayout->addLayout(hlayout);
setLayout(vlayout);
connect(createButton, SIGNAL(clicked()), this, SLOT(createDirectory()));
connect(removeButton, SIGNAL(clicked()), this, SLOT(remove()));
}
DirectoryViewer::~DirectoryViewer()
{
}
void DirectoryViewer::createDirectory()
{
QModelIndex index = treeView->currentIndex();
if (!index.isValid())
{
return;
}
QString dirName = QInputDialog::getText(this, tr("Create Directory"), tr("Directory name"));
if (!dirName.isEmpty())
{
if (!model->mkdir(index, dirName).isValid())
{
QMessageBox::information(this, tr("Create Directory"), tr("Failed to create the directory"));
}
}
}
void DirectoryViewer::remove()
{
QModelIndex index = treeView->currentIndex();
if (!index.isValid())
{
return;
}
bool ok;
if (model->fileInfo(index).isDir())
{
ok = model->rmdir(index);
}
else
{
ok = model->remove(index);
}
if (!ok)
{
QMessageBox::information(this, tr("Remove"), tr("Failed to remove %1").arg(model->fileName(index)));
}
}
main.cpp文件:
#include <QtGui/QApplication>
#include "directoryviewer.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
DirectoryViewer w;
w.show();
w.setWindowTitle("QFileSystemModel Demo");
return a.exec();
}
转载2
QFileSystemModel will not fetch any files or directories until setRootPath() is called. This will prevent any unnecessary querying on the file system until that point such as listing the drives on Windows.
QFileSystemModel uses a separate thread to populate itself so it will not cause the main thread to hang as the file system is being queried.
Calls to rowCount() will return 0 until the model populates a directory. QFileSystemModel keeps a cache with file information. The cache is automatically kept up to date using the QFileSystemWatcher.
In this example, we'll use Qt Gui application with QDialog:
As we discussed in other ModelView tutorials, Qt's MVC may not be the same as the conventional MVC.
If the view and the controller objects are combined, the result is the model/view architecture. This still separates the way that data is stored from the way that it is presented to the user, but provides a simpler framework based on the same principles. This separation makes it possible to display the same data in several different views, and to implement new types of views, without changing the underlying data structures. To allow flexible handling of user input, we introduce the concept of the delegate. The advantage of having a delegate in this framework is that it allows the way items of data are rendered and edited to be customized.However, it's worth investigating their approach of using "delegate" with their Model/View pattern.
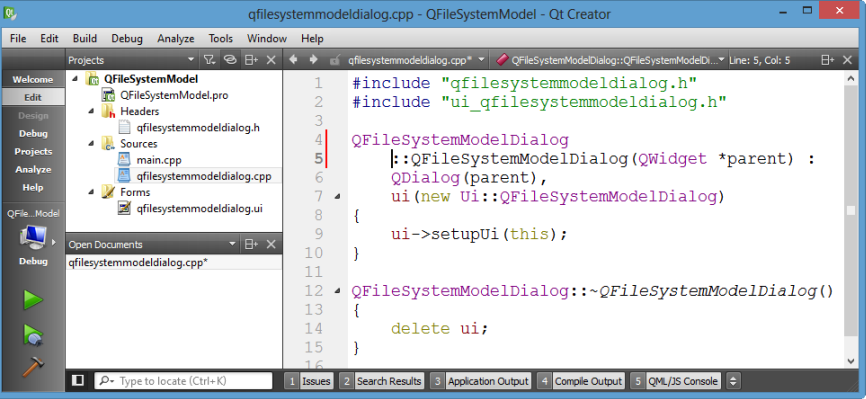
In this tutorial, we'll use Model-Based TreeView for folders on the left side and ListView for files on the right side:
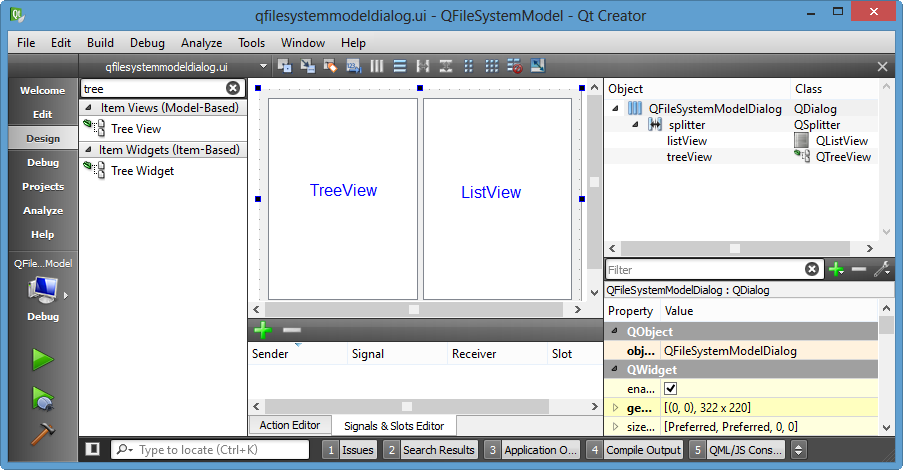
Since we finished layout, now is the time for coding.
Let's make two models in qfilesystemmodeldialog.h: one for files and the other for directories. We make two models because we need to filter them separately.
#ifndef QFILESYSTEMMODELDIALOG_H #define QFILESYSTEMMODELDIALOG_H #include <QDialog> #include <QFileSystemModel> namespace Ui { class QFileSystemModelDialog; } class QFileSystemModelDialog : public QDialog { Q_OBJECT public: explicit QFileSystemModelDialog(QWidget *parent = 0); ~QFileSystemModelDialog(); private: Ui::QFileSystemModelDialog *ui; // Make two models instead of one // to filter them separately QFileSystemModel *dirModel; QFileSystemModel *fileModel; }; #endif // QFILESYSTEMMODELDIALOG_H
Move on to the implementation file, qfilesystemmodeldialog.cpp:
#include "qfilesystemmodeldialog.h" #include "ui_qfilesystemmodeldialog.h" QFileSystemModelDialog::QFileSystemModelDialog(QWidget *parent) : QDialog(parent), ui(new Ui::QFileSystemModelDialog) { ui->setupUi(this); // Creates our new model and populate QString mPath = "C:/"; dirModel = new QFileSystemModel(this); // Set filter dirModel->setFilter(QDir::NoDotAndDotDot | QDir::AllDirs); // QFileSystemModel requires root path dirModel->setRootPath(mPath); // Attach the model to the view ui->treeView->setModel(dirModel); } QFileSystemModelDialog::~QFileSystemModelDialog() { delete ui; }
Let's run the code to see what we've done.
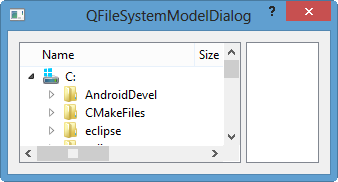
It shows the TreeView for directories successfully, and it looks like we're on track!
How about files?
We add similar line of code for files in the implementation file, qfilesystemmodeldialog.cpp:
// FILES fileModel = new QFileSystemModel(this); // Set filter fileModel->setFilter(QDir::NoDotAndDotDot | QDir::Files); // QFileSystemModel requires root path fileModel->setRootPath(mPath); // Attach the model to the view ui->listView->setModel(fileModel);
Let's setup a slot for the TreeView by "Go to slot..."->clicked(ModelIndex).
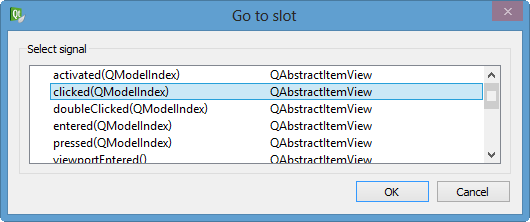
void QFileSystemModelDialog::on_treeView_clicked(const QModelIndex &index) { // TreeView clicked // 1. We need to extract path // 2. Set that path into our ListView // Get the full path of the item that's user clicked on QString mPath = dirModel->fileInfo(index).absoluteFilePath(); ui->listView->setRootIndex(fileModel->setRootPath(mPath)); }
Again, let's run the code:
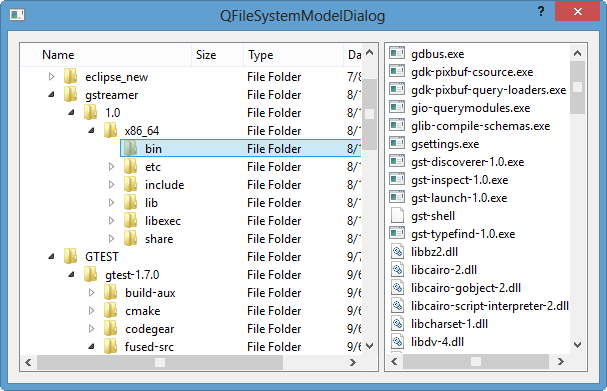
Great!
Here are the final codes:
qfilesystemmodeldialog.h:
#ifndef QFILESYSTEMMODELDIALOG_H
#define QFILESYSTEMMODELDIALOG_H
#include <QDialog>
#include <QFileSystemModel>
namespace Ui {
class QFileSystemModelDialog;
}
class QFileSystemModelDialog : public QDialog
{
Q_OBJECT
public:
explicit QFileSystemModelDialog(QWidget *parent = 0);
~QFileSystemModelDialog();
private slots:
void on_treeView_clicked(const QModelIndex &index);
private:
Ui::QFileSystemModelDialog *ui;
// Make two models instead of one
// to filter them separately
QFileSystemModel *dirModel;
QFileSystemModel *fileModel;
};
#endif // QFILESYSTEMMODELDIALOG_H
The implementation file, qfilesystemmodeldialog.cpp:
#include "qfilesystemmodeldialog.h" #include "ui_qfilesystemmodeldialog.h" QFileSystemModelDialog::QFileSystemModelDialog(QWidget *parent) : QDialog(parent), ui(new Ui::QFileSystemModelDialog) { ui->setupUi(this); // Creates our new model and populate QString mPath = "C:/"; // DIRECTORIES dirModel = new QFileSystemModel(this); // Set filter dirModel->setFilter(QDir::NoDotAndDotDot | QDir::AllDirs); // QFileSystemModel requires root path dirModel->setRootPath(mPath); // Attach the dir model to the view ui->treeView->setModel(dirModel); // FILES fileModel = new QFileSystemModel(this); // Set filter fileModel->setFilter(QDir::NoDotAndDotDot | QDir::Files); // QFileSystemModel requires root path fileModel->setRootPath(mPath); // Attach the file model to the view ui->listView->setModel(fileModel); } QFileSystemModelDialog::~QFileSystemModelDialog() { delete ui; } void QFileSystemModelDialog::on_treeView_clicked(const QModelIndex &index) { // TreeView clicked // 1. We need to extract path // 2. Set that path into our ListView // Get the full path of the item that's user clicked on QString mPath = dirModel->fileInfo(index).absoluteFilePath(); ui->listView->setRootIndex(fileModel->setRootPath(mPath)); }