using System;
using System.Collections.Generic;
using System.Text;
using System.IO;
using System.Web;
using System.Drawing;
using System.Drawing.Imaging;
using System.Drawing.Drawing2D;
using System.Collections;
using System.Net;
using System.Text.RegularExpressions;
using System.Configuration;
namespace SyntacticSugar
{
/// <summary>
/// ** 描述:图片上传类、支持水印、缩略图
/// ** 创始时间:2015-5-28
/// ** 修改时间:-
/// ** 修改人:sunkaixuan
/// </summary>
public class UploadImage
{
#region 属性
/// <summary>
/// 允许图片格式
/// </summary>
public string SetAllowFormat { get; set; }
/// <summary>
/// 允许上传图片大小
/// </summary>
public double SetAllowSize { get; set; }
/// <summary>
/// 文字水印字符
/// </summary>
public string SetWordWater { get; set; }
/// <summary>
/// 图片水印
/// </summary>
public string SetPicWater { get; set; }
/// <summary>
/// 水印图片的位置 0居中、1左上角、2右上角、3左下角、4右下角
/// </summary>
public int SetPositionWater { get; set; }
/// <summary>
/// 缩略图宽度多个逗号格开(例如:200,100)
/// </summary>
public string SetSmallImgWidth { get; set; }
/// <summary>
/// 缩略图高度多个逗号格开(例如:200,100)
/// </summary>
public string SetSmallImgHeight { get; set; }
/// <summary>
/// 是否限制最大宽度,默认为true
/// </summary>
public bool SetLimitWidth { get; set; }
/// <summary>
/// 最大宽度尺寸,默认为600
/// </summary>
public int SetMaxWidth { get; set; }
/// <summary>
/// 是否剪裁图片,默认true
/// </summary>
public bool SetCutImage { get; set; }
/// <summary>
/// 限制图片最小宽度,0表示不限制
/// </summary>
public int SetMinWidth { get; set; }
#endregion
public UploadImage()
{
SetAllowFormat = ".jpeg|.jpg|.bmp|.gif|.png"; //允许图片格式
SetAllowSize = 1; //允许上传图片大小,默认为1MB
SetPositionWater = 4;
SetCutImage = true;
}
#region main method
/// <summary>
/// 裁剪图片
/// </summary>
/// <param name="PostedFile">HttpPostedFile控件</param>
/// <param name="SavePath">保存路径【sys.config配置路径】</param>
/// <param name="oImgWidth">图片宽度</param>
/// <param name="oImgHeight">图片高度</param>
/// <param name="cMode">剪切类型</param>
/// <param name="ext">返回格式</param>
/// <returns>返回上传信息</returns>
public ResponseMessage FileCutSaveAs(System.Web.HttpPostedFile PostedFile, string SavePath, int oImgWidth, int oImgHeight, CutMode cMode)
{
ResponseMessage rm = new ResponseMessage();
try
{
//获取上传文件的扩展名
string sEx = System.IO.Path.GetExtension(PostedFile.FileName);
if (!CheckValidExt(SetAllowFormat, sEx))
{
TryError(rm, 2);
return rm;
}
//获取上传文件的大小
double PostFileSize = PostedFile.ContentLength / 1024.0 / 1024.0;
if (PostFileSize > SetAllowSize)
{
TryError(rm, 3);
return rm; //超过文件上传大小
}
if (!System.IO.Directory.Exists(SavePath))
{
System.IO.Directory.CreateDirectory(SavePath);
}
//重命名名称
string NewfileName = DateTime.Now.Year.ToString() + DateTime.Now.Month.ToString() + DateTime.Now.Day.ToString() + DateTime.Now.Hour.ToString() + DateTime.Now.Minute.ToString() + DateTime.Now.Second.ToString() + DateTime.Now.Millisecond.ToString("000");
string fName = "s" + NewfileName + sEx;
string fullPath = Path.Combine(SavePath, fName);
PostedFile.SaveAs(fullPath);
//重命名名称
string sNewfileName = DateTime.Now.Year.ToString() + DateTime.Now.Month.ToString() + DateTime.Now.Day.ToString() + DateTime.Now.Hour.ToString() + DateTime.Now.Minute.ToString() + DateTime.Now.Second.ToString() + DateTime.Now.Millisecond.ToString("000");
string sFName = sNewfileName + sEx;
rm.IsError = false;
rm.FileName = sFName;
string sFullPath = Path.Combine(SavePath, sFName);
rm.filePath = sFullPath;
rm.WebPath = "/"+sFullPath.Replace(HttpContext.Current.Server.MapPath("~/"), "").Replace("\\", "/");
CreateSmallPhoto(fullPath, oImgWidth, oImgHeight, sFullPath, SetPicWater, SetWordWater, cMode);
if (File.Exists(fullPath))
{
File.Delete(fullPath);
}
//压缩
if (PostFileSize > 100)
{
CompressPhoto(sFullPath, 100);
}
}
catch (Exception ex)
{
TryError(rm, ex.Message);
}
return rm;
}
/// <summary>
/// 通用图片上传类
/// </summary>
/// <param name="PostedFile">HttpPostedFile控件</param>
/// <param name="SavePath">保存路径【sys.config配置路径】</param>
/// <param name="finame">返回文件名</param>
/// <param name="fisize">返回文件大小</param>
/// <returns>返回上传信息</returns>
public ResponseMessage FileSaveAs(System.Web.HttpPostedFile PostedFile, string SavePath)
{
ResponseMessage rm = new ResponseMessage();
try
{
if (string.IsNullOrEmpty(PostedFile.FileName))
{
TryError(rm, 4);
return rm;
}
Random rd = new Random();
int rdInt = rd.Next(1000, 9999);
//重命名名称
string NewfileName = DateTime.Now.Year.ToString() + DateTime.Now.Month.ToString() + DateTime.Now.Day.ToString() + DateTime.Now.Hour.ToString() + DateTime.Now.Minute.ToString() + DateTime.Now.Second.ToString() + DateTime.Now.Millisecond.ToString() + rdInt;
//获取上传文件的扩展名
string sEx = System.IO.Path.GetExtension(PostedFile.FileName);
if (!CheckValidExt(SetAllowFormat, sEx))
{
TryError(rm, 2);
return rm;
}
//获取上传文件的大小
double PostFileSize = PostedFile.ContentLength / 1024.0 / 1024.0;
if (PostFileSize > SetAllowSize)
{
TryError(rm, 3);
return rm;
}
if (!System.IO.Directory.Exists(SavePath))
{
System.IO.Directory.CreateDirectory(SavePath);
}
rm.FileName = NewfileName + sEx;
string fullPath = SavePath.Trim('\\') + "\\" &
一款非常不错的asp.net图片处理类,自己用的时候需要做相应的修改(水印、剪裁、缩略图)
最新推荐文章于 2024-05-12 20:28:22 发布
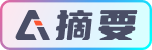