Ajax 上传图片并可以生成指定大小缩略图
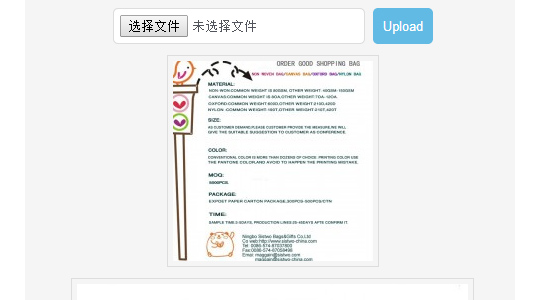
XML/HTML Code
- <div id="upload-wrapper">
- <div align="center">
- <form action="processupload.php" method="post" enctype="multipart/form-data" id="MyUploadForm">
- <input name="ImageFile" id="imageInput" type="file" />
- <input type="submit" id="submit-btn" value="Upload" />
- <img src="images/ajax-loader.gif" id="loading-img" style="display:none;" alt="Please Wait"/>
- </form>
- <div id="output"></div>
- </div>
- </div>
JavaScript Code
- <script type="text/javascript">
- $(document).ready(function() {
- var options = {
- target: '#output', // target element(s) to be updated with server response
- beforeSubmit: beforeSubmit, // pre-submit callback
- success: afterSuccess, // post-submit callback
- resetForm: true // reset the form after successful submit
- };
- $('#MyUploadForm').submit(function() {
- $(this).ajaxSubmit(options);
- // always return false to prevent standard browser submit and page navigation
- return false;
- });
- });
- function afterSuccess()
- {
- $('#submit-btn').show(); //hide submit button
- $('#loading-img').hide(); //hide submit button
- }
- //function to check file size before uploading.
- function beforeSubmit(){
- //check whether browser fully supports all File API
- if (window.File && window.FileReader && window.FileList && window.Blob)
- {
- if( !$('#imageInput').val()) //check empty input filed
- {
- $("#output").html("Are you kidding me?");
- return false
- }
- var fsize = $('#imageInput')[0].files[0].size; //get file size
- var ftype = $('#imageInput')[0].files[0].type; // get file type
- //allow only valid image file types
- switch(ftype)
- {
- case 'image/png': case 'image/gif': case 'image/jpeg': case 'image/pjpeg':
- break;
- default:
- $("#output").html("<b>"+ftype+"</b> Unsupported file type!");
- return false
- }
- //Allowed file size is less than 1 MB (1048576)
- if(fsize>1048576)
- {
- $("#output").html("<b>"+bytesToSize(fsize) +"</b> Too big Image file! <br />Please reduce the size of your photo using an image editor.");
- return false
- }
- $('#submit-btn').hide(); //hide submit button
- $('#loading-img').show(); //hide submit button
- $("#output").html("");
- }
- else
- {
- //Output error to older unsupported browsers that doesn't support HTML5 File API
- $("#output").html("Please upgrade your browser, because your current browser lacks some new features we need!");
- return false;
- }
- }
- //function to format bites bit.ly/19yoIPO
- function bytesToSize(bytes) {
- var sizes = ['Bytes', 'KB', 'MB', 'GB', 'TB'];
- if (bytes == 0) return '0 Bytes';
- var i = parseInt(Math.floor(Math.log(bytes) / Math.log(1024)));
- return Math.round(bytes / Math.pow(1024, i), 2) + ' ' + sizes[i];
- }
- </script>
processupload.php
PHP Code
- <?php
- if(isset($_POST))
- {
- ############ Edit settings ##############
- $ThumbSquareSize = 200; //Thumbnail will be 200x200
- $BigImageMaxSize = 500; //Image Maximum height or width
- $ThumbPrefix = "thumb_"; //Normal thumb Prefix
- $DestinationDirectory = '../upload/'; //specify upload directory ends with / (slash)
- $Quality = 90; //jpeg quality
- ##########################################
- //check if this is an ajax request
- if (!isset($_SERVER['HTTP_X_REQUESTED_WITH'])){
- die();
- }
- // check $_FILES['ImageFile'] not empty
- if(!isset($_FILES['ImageFile']) || !is_uploaded_file($_FILES['ImageFile']['tmp_name']))
- {
- die('Something wrong with uploaded file, something missing!'); // output error when above checks fail.
- }
- // Random number will be added after image name
- $RandomNumber = rand(0, 9999999999);
- $ImageName = str_replace(' ','-',strtolower($_FILES['ImageFile']['name'])); //get image name
- $ImageSize = $_FILES['ImageFile']['size']; // get original image size
- $TempSrc = $_FILES['ImageFile']['tmp_name']; // Temp name of image file stored in PHP tmp folder
- $ImageType = $_FILES['ImageFile']['type']; //get file type, returns "image/png", image/jpeg, text/plain etc.
- //Let's check allowed $ImageType, we use PHP SWITCH statement here
- switch(strtolower($ImageType))
- {
- case 'image/png':
- //Create a new image from file
- $CreatedImage = imagecreatefrompng($_FILES['ImageFile']['tmp_name']);
- break;
- case 'image/gif':
- $CreatedImage = imagecreatefromgif($_FILES['ImageFile']['tmp_name']);
- break;
- case 'image/jpeg':
- case 'image/pjpeg':
- $CreatedImage = imagecreatefromjpeg($_FILES['ImageFile']['tmp_name']);
- break;
- default:
- die('Unsupported File!'); //output error and exit
- }
- //PHP getimagesize() function returns height/width from image file stored in PHP tmp folder.
- //Get first two values from image, width and height.
- //list assign svalues to $CurWidth,$CurHeight
- list($CurWidth,$CurHeight)=getimagesize($TempSrc);
- //Get file extension from Image name, this will be added after random name
- $ImageExt = substr($ImageName, strrpos($ImageName, '.'));
- $ImageExt = str_replace('.','',$ImageExt);
- //remove extension from filename
- $ImageName = preg_replace("/\.[^.\s]{3,4}$/", "", $ImageName);
- //Construct a new name with random number and extension.
- $NewImageName = $ImageName.'-'.$RandomNumber.'.'.$ImageExt;
- //set the Destination Image
- $thumb_DestRandImageName = $DestinationDirectory.$ThumbPrefix.$NewImageName; //Thumbnail name with destination directory
- $DestRandImageName = $DestinationDirectory.$NewImageName; // Image with destination directory
- //Resize image to Specified Size by calling resizeImage function.
- if(resizeImage($CurWidth,$CurHeight,$BigImageMaxSize,$DestRandImageName,$CreatedImage,$Quality,$ImageType))
- {
- //Create a square Thumbnail right after, this time we are using cropImage() function
- if(!cropImage($CurWidth,$CurHeight,$ThumbSquareSize,$thumb_DestRandImageName,$CreatedImage,$Quality,$ImageType))
- {
- echo 'Error Creating thumbnail';
- }
- /*
- We have succesfully resized and created thumbnail image
- We can now output image to user's browser or store information in the database
- */
- echo '<table width="100%" border="0" cellpadding="4" cellspacing="0">';
- echo '<tr>';
- echo '<td align="center"><img src="../upload/'.$ThumbPrefix.$NewImageName.'" alt="Thumbnail"></td>';
- echo '</tr><tr>';
- echo '<td align="center"><img src="../upload/'.$NewImageName.'" alt="Resized Image"></td>';
- echo '</tr>';
- echo '</table>';
- /*
- // Insert info into database table!
- mysql_query("INSERT INTO myImageTable (ImageName, ThumbName, ImgPath)
- VALUES ($DestRandImageName, $thumb_DestRandImageName, 'uploads/')");
- */
- }else{
- die('Resize Error'); //output error
- }
- }
- // This function will proportionally resize image
- function resizeImage($CurWidth,$CurHeight,$MaxSize,$DestFolder,$SrcImage,$Quality,$ImageType)
- {
- //Check Image size is not 0
- if($CurWidth <= 0 || $CurHeight <= 0)
- {
- return false;
- }
- //Construct a proportional size of new image
- $ImageScale = min($MaxSize/$CurWidth, $MaxSize/$CurHeight);
- $NewWidth = ceil($ImageScale*$CurWidth);
- $NewHeight = ceil($ImageScale*$CurHeight);
- $NewCanves = imagecreatetruecolor($NewWidth, $NewHeight);
- // Resize Image
- if(imagecopyresampled($NewCanves, $SrcImage,0, 0, 0, 0, $NewWidth, $NewHeight, $CurWidth, $CurHeight))
- {
- switch(strtolower($ImageType))
- {
- case 'image/png':
- imagepng($NewCanves,$DestFolder);
- break;
- case 'image/gif':
- imagegif($NewCanves,$DestFolder);
- break;
- case 'image/jpeg':
- case 'image/pjpeg':
- imagejpeg($NewCanves,$DestFolder,$Quality);
- break;
- default:
- return false;
- }
- //Destroy image, frees memory
- if(is_resource($NewCanves)) {imagedestroy($NewCanves);}
- return true;
- }
- }
- //This function corps image to create exact square images, no matter what its original size!
- function cropImage($CurWidth,$CurHeight,$iSize,$DestFolder,$SrcImage,$Quality,$ImageType)
- {
- //Check Image size is not 0
- if($CurWidth <= 0 || $CurHeight <= 0)
- {
- return false;
- }
- //abeautifulsite.net has excellent article about "Cropping an Image to Make Square bit.ly/1gTwXW9
- if($CurWidth>$CurHeight)
- {
- $y_offset = 0;
- $x_offset = ($CurWidth - $CurHeight) / 2;
- $square_size = $CurWidth - ($x_offset * 2);
- }else{
- $x_offset = 0;
- $y_offset = ($CurHeight - $CurWidth) / 2;
- $square_size = $CurHeight - ($y_offset * 2);
- }
- $NewCanves = imagecreatetruecolor($iSize, $iSize);
- if(imagecopyresampled($NewCanves, $SrcImage,0, 0, $x_offset, $y_offset, $iSize, $iSize, $square_size, $square_size))
- {
- switch(strtolower($ImageType))
- {
- case 'image/png':
- imagepng($NewCanves,$DestFolder);
- break;
- case 'image/gif':
- imagegif($NewCanves,$DestFolder);
- break;
- case 'image/jpeg':
- case 'image/pjpeg':
- imagejpeg($NewCanves,$DestFolder,$Quality);
- break;
- default:
- return false;
- }
- //Destroy image, frees memory
- if(is_resource($NewCanves)) {imagedestroy($NewCanves);}
- return true;
- }
- }