- 学习目的:
通过进一步学习Nhibernate基础知识,掌握用Nhiberate实现多对多的业务逻辑
- 开发环境+必要准备
开发环境: windows 2003,Visual studio .Net 2005,Sql server 2005 developer edition
前期准备: 学习上两篇单表操作和many-to-one篇
3.对上篇文章的部分解释
1)bag节点:用于定义System.Collection.IList类型的集合元素。
属性 | 用法 | 举例 |
name | 映射的属性(必须) | name=”SalaryList” |
table | 映射的数据表(可选) | table=”Salary” |
lazy | 延迟加载(可选) | Lazy=true|false |
cascade | 指示级联操作方式(可选) | Cascade=all |
inverse | 关联由谁负责维护 | Inverse=”true” |
当lazy=”true”,父类初始化的时候不会自动加载子类集合
Cascade为级联操作方式,包括:
属性 | 用法说明 |
none | 默认值,不进行级联操作 |
save-update | save和update级联 |
delete | 删除级联 |
delete-orphan | 删除不相关的父对象的子对象 |
all | save/update/delete级联 |
all-delete-orphan | all+delete-arphan |
当inverse=”true”的时候代表由子类维护级联关系。这时候如果只往父类中添加子类,但不设定子类的父类,是不能保存子类的。
4.多对多业务模型
还是用户系统,1个用户职员隶属于多个部门,同时1个部门有多个不同的职员
用户和部门之间的数据关系图为:
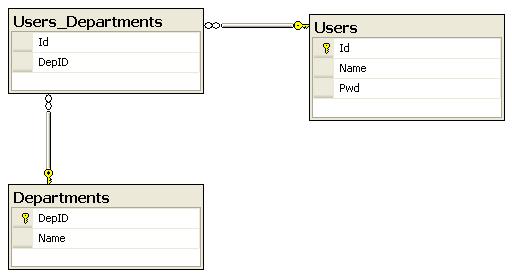
5. 实现步骤:
1)User.cs
User.cs
<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->
usingSystem;
usingSystem.Collections.Generic;
usingSystem.Text;

namespaceNhibernateSample1


{
publicclassUser


{
privateint_id;
privatestring_name;
privatestring_pwd;
privateSystem.Collections.IList_departmentsList;

/**////<summary>
///编号
///</summary>
publicvirtualintId


{
get


{
return_id;
}
set


{
_id=value;
}
}


/**////<summary>
///名称
///</summary>
publicvirtualstringName


{
get


{
return_name;
}
set


{
_name=value;
}
}


/**////<summary>
///密码
///</summary>
publicvirtualstringPwd


{
get


{
return_pwd;
}
set


{
_pwd=value;
}
}

/**////<summary>
///工资列表
///</summary>
publicSystem.Collections.IListDepartmentsList


{
get


{
return_departmentsList;
}
set


{
_departmentsList=value;
}
}
}
}
2)User.hbm.xml
User.hbm.xml
<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->
<?xmlversion="1.0"encoding="utf-8"?>
<hibernate-mappingxmlns="urn:nhibernate-mapping-2.2">
<classname="NhibernateSample1.User,NhibernateSample1"table="Users"lazy="false">
<idname="Id"column="Id"unsaved-value="0">
<generatorclass="native"/>
</id>
<propertyname="Name"column="Name"type="string"length="64"not-null="true"unique="true"></property>
<propertyname="Pwd"column="Pwd"type="string"length="64"not-null="true"></property>
<bagname="DepartmentsList"table="Users_Departments"inverse="true"lazy="false"cascade="all">
<keycolumn="Id"/>
<many-to-manyclass="NhibernateSample1.Departments,NhibernateSample1"column="DepID"></many-to-many>
</bag>
</class>
</hibernate-mapping>
3) Departments.cs
Departments
<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->
usingSystem;
usingSystem.Collections.Generic;
usingSystem.Text;
usingSystem.Collections;

namespaceNhibernateSample1


{
publicclassDepartments


{
int_depID;
string_name;
IList_usersList=newArrayList();
publicvirtualintDepID


{
get


{
return_depID;
}
set


{
_depID=value;
}
}
publicvirtualstringName


{
get


{
return_name;
}
set


{
_name=value;
}
}
publicvirtualIListUsersList


{
get


{
return_usersList;
}
set


{
_usersList=value;
}
}
}
}
4) Departments.hbm.xml
Departments.hbm.xml
<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->
<?xmlversion="1.0"encoding="utf-8"?>
<hibernate-mappingxmlns="urn:nhibernate-mapping-2.2">
<classname="NhibernateSample1.Departments,NhibernateSample1"table="Departments"lazy="false">
<idname="DepID"column="DepID"unsaved-value="0">
<generatorclass="native"/>
</id>
<propertyname="Name"column="Name"type="string"length="64"not-null="true"unique="true"></property>
<bagname="UsersList"table="Users_Departments"lazy="true">
<keycolumn="DepID"/>
<many-to-manyclass="NhibernateSample1.User,NhibernateSample1"column="Id"></many-to-many>
</bag>
</class>
</hibernate-mapping>
5) 数据操作类
UserDepartmentFixure.cs
<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->
usingSystem;
usingSystem.Collections.Generic;
usingSystem.Text;
usingSystem.Collections;
usingNHibernate;
usingNHibernate.Cfg;
usingNHibernate.Tool.hbm2ddl;

namespaceNhibernateSample1


{
publicclassUserDepartmentFixure


{
privateISessionFactory_sessions;
publicvoidConfigure()


{
Configurationcfg=GetConfiguration();
_sessions=cfg.BuildSessionFactory();
}
ConfigurationGetConfiguration()


{
stringcfgPath=@"E:/myproject/nhibernatestudy/simle1/NHibernateStudy1/NhibernateSample1/hibernate.cfg.xml";
Configurationcfg=newConfiguration().Configure(cfgPath);
returncfg;
}
publicvoidExportTables()


{
Configurationcfg=GetConfiguration();
newSchemaExport(cfg).Create(true,true);
}
publicUserCreateUser(Stringname,stringpwd)


{
Useru=newUser();
u.Name=name;
u.Pwd=pwd;
u.DepartmentsList=newArrayList();

ISessionsession=_sessions.OpenSession();

ITransactiontx=null;

try


{
tx=session.BeginTransaction();
session.Save(u);
tx.Commit();
}
catch(HibernateExceptione)


{
if(tx!=null)tx.Rollback();
throwe;
}
finally


{
session.Close();
}

returnu;
}
publicDepartmentsCreateDepartments(Useru,stringname)


{
Departmentsitem=newDepartments();
item.Name=name;
u.DepartmentsList.Add(item);
item.UsersList.Add(u);
ISessionsession=_sessions.OpenSession();
ITransactiontx=null;

try


{
tx=session.BeginTransaction();
session.Save(item);
tx.Commit();
}
catch(HibernateExceptione)


{
if(tx!=null)tx.Rollback();
throwe;
}
finally


{
session.Close();
}
returnitem;
}
publicDepartmentsGetDepartments(intdepID)


{
ISessionsession=_sessions.OpenSession();
ITransactiontx=null;
try


{
tx=session.BeginTransaction();
Departmentsitem=(Departments)session.Load(typeof(Departments),
depID);
tx.Commit();
returnitem;
}
catch(HibernateExceptione)


{
if(tx!=null)tx.Rollback();
returnnull;
}
finally


{
session.Close();
}
returnnull;
}
publicUserGetUser(intuid)


{
ISessionsession=_sessions.OpenSession();
ITransactiontx=null;
try


{
tx=session.BeginTransaction();
Useritem=(User)session.Load(typeof(User),
uid);
tx.Commit();
returnitem;
}
catch(HibernateExceptione)


{
if(tx!=null)tx.Rollback();
returnnull;
}
finally


{
session.Close();
}
returnnull;
}

publicvoidDelete(intuid)


{
ISessionsession=_sessions.OpenSession();
ITransactiontx=null;
try


{
tx=session.BeginTransaction();
Departmentsitem=session.Load(typeof(Departments),uid)asDepartments;
session.Delete(item);
tx.Commit();
}
catch(HibernateExceptione)


{
if(tx!=null)tx.Rollback();
throwe;
}
finally


{
session.Close();
}
}

}
}
6)单元测试类
UnitTest1.cs
<!--<br><br>Code highlighting produced by Actipro CodeHighlighter (freeware)<br>http://www.CodeHighlighter.com/<br><br>-->
usingSystem;
usingSystem.Text;
usingSystem.Collections.Generic;
usingMicrosoft.VisualStudio.TestTools.UnitTesting;
usingNhibernateSample1;

namespaceTestProject1


{

/**////<summary>
///UnitTest1的摘要说明
///</summary>
[TestClass]
publicclassUnitTest1


{
publicUnitTest1()


{
//
//TODO:在此处添加构造函数逻辑
//
}
NhibernateSample1.UserDepartmentFixureusf=newUserDepartmentFixure();

其他测试属性#region其他测试属性
//
//您可以在编写测试时使用下列其他属性:
//
//在运行类中的第一个测试之前使用ClassInitialize运行代码
//[ClassInitialize()]
//publicstaticvoidMyClassInitialize(TestContexttestContext){}
//
//在类中的所有测试都已运行之后使用ClassCleanup运行代码
//[ClassCleanup()]
//publicstaticvoidMyClassCleanup(){}
//
//在运行每个测试之前使用TestInitialize运行代码
//[TestInitialize()]
//publicvoidMyTestInitialize(){}
//
//在运行每个测试之后使用TestCleanup运行代码
//[TestCleanup()]
//publicvoidMyTestCleanup(){}
//
#endregion

[TestMethod]
publicvoidTest1()


{
usf.Configure();
usf.ExportTables();
Useru=usf.CreateUser(Guid.NewGuid().ToString(),"ds");
Assert.IsTrue(u.Id>0);
Departmentss=usf.CreateDepartments(u,"政治部");
Assert.IsTrue(s.DepID>0);
Departmentss1=usf.CreateDepartments(u,"事业部");
Assert.IsTrue(s1.DepID>0);
usf.Delete(s1.DepID);
s1=usf.GetDepartments(s1.DepID);
Assert.IsNull(s1);
Useru1=usf.GetUser(1);
Assert.IsTrue(u1.DepartmentsList.Count>0);
}

}
}
到现在为止,终于更加体会到nhibernate的强大了。继续努力,fight!
files:
/Files/jillzhang/simple3.rar