效果图差不多这样子
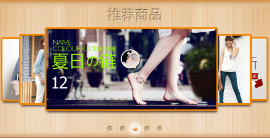
import android.content.Context; import android.graphics.Camera; import android.graphics.Matrix; import android.util.AttributeSet; import android.view.View; import android.view.animation.Transformation; import android.widget.Gallery; import android.widget.ImageView; public class CoverFlow extends Gallery { private Camera mCamera = new Camera(); private int mMaxRotationAngle = 50; private int mMaxZoom = -380; private int mCoveflowCenter; private boolean mAlphaMode = true; private boolean mCircleMode = false; public CoverFlow(Context context) { super(context); this.setStaticTransformationsEnabled(true); } public CoverFlow(Context context, AttributeSet attrs) { super(context, attrs); this.setStaticTransformationsEnabled(true); } public CoverFlow(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); this.setStaticTransformationsEnabled(true); } public int getMaxRotationAngle() { return mMaxRotationAngle; } public void setMaxRotationAngle(int maxRotationAngle) { mMaxRotationAngle = maxRotationAngle; } public boolean getCircleMode() { return mCircleMode; } public void setCircleMode(boolean isCircle) { mCircleMode = isCircle; } public boolean getAlphaMode() { return mAlphaMode; } public void setAlphaMode(boolean isAlpha) { mAlphaMode = isAlpha; } public int getMaxZoom() { return mMaxZoom; } public void setMaxZoom(int maxZoom) { mMaxZoom = maxZoom; } private int getCenterOfCoverflow() { return (getWidth() - getPaddingLeft() - getPaddingRight()) / 2 + getPaddingLeft(); } private static int getCenterOfView(View view) { return view.getLeft() + view.getWidth() / 2; } protected boolean getChildStaticTransformation(View child, Transformation t) { final int childCenter = getCenterOfView(child); final int childWidth = child.getWidth(); int rotationAngle = 0; t.clear(); t.setTransformationType(Transformation.TYPE_MATRIX); if (childCenter == mCoveflowCenter) { transformImageBitmap((ImageView) child, t, 0); } else { rotationAngle = (int) (((float) (mCoveflowCenter - childCenter) / childWidth) * mMaxRotationAngle); if (Math.abs(rotationAngle) > mMaxRotationAngle) { rotationAngle = (rotationAngle < 0) ? -mMaxRotationAngle : mMaxRotationAngle; } transformImageBitmap((ImageView) child, t, rotationAngle); } return true; } /** * This is called during layout when the size of this view has changed. If * you were just added to the view hierarchy, you're called with the old * values of 0. * * @param w * Current width of this view. * @param h * Current height of this view. * @param oldw * Old width of this view. * @param oldh * Old height of this view. */ protected void onSizeChanged(int w, int h, int oldw, int oldh) { mCoveflowCenter = getCenterOfCoverflow(); super.onSizeChanged(w, h, oldw, oldh); } /** * Transform the Image Bitmap by the Angle passed * * @param imageView * ImageView the ImageView whose bitmap we want to rotate * @param t * transformation * @param rotationAngle * the Angle by which to rotate the Bitmap */ private void transformImageBitmap(ImageView child, Transformation t, int rotationAngle) { mCamera.save(); final Matrix imageMatrix = t.getMatrix(); final int imageHeight = child.getLayoutParams().height; final int imageWidth = child.getLayoutParams().width; final int rotation = Math.abs(rotationAngle); mCamera.translate(0.0f, 0.0f, 100.0f); // As the angle of the view gets less, zoom in if (rotation <= mMaxRotationAngle) { float zoomAmount = (float) (mMaxZoom + (rotation * 1.5)); mCamera.translate(0.0f, 0.0f, zoomAmount); if (mCircleMode) { if (rotation < 40) mCamera.translate(0.0f, 155, 0.0f); else mCamera.translate(0.0f, (255 - rotation * 2.5f), 0.0f); } if (mAlphaMode) { ((ImageView) (child)).setAlpha((int) (255 - rotation * 2.5)); } } mCamera.rotateY(rotationAngle); mCamera.getMatrix(imageMatrix); imageMatrix.preTranslate(-(imageWidth / 2), -(imageHeight / 2)); imageMatrix.postTranslate((imageWidth / 2), (imageHeight / 2)); mCamera.restore(); } }
import android.app.Activity;
import android.content.Context;
import android.graphics.drawable.BitmapDrawable;
import android.os.Bundle;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import com.mmb.shop.R;
import com.mmb.shop.view.CoverFlow;
public class TestActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
CoverFlow cf = new CoverFlow(this);
//cf.setBackgroundResource(R.drawable.icon);
cf.setAdapter(new ImageAdapter(this));
ImageAdapter imageAdapter = new ImageAdapter(this);
cf.setAdapter(imageAdapter);
// cf.setAlphaMode(false);
// cf.setCircleMode(false);
// cf.setSelection(3, true);
cf.setAnimationDuration(1000);
setContentView(cf);
}
public class ImageAdapter extends BaseAdapter {
private Context mContext;
private Integer[] mImageIds = {
R.drawable.a,
R.drawable.b,
R.drawable.c,
R.drawable.d,
R.drawable.e,
R.drawable.f,
R.drawable.g
};
public ImageAdapter(Context c) {
mContext = c;
}
public int getCount() {
return mImageIds.length;
}
public Object getItem(int position) {
return position;
}
public long getItemId(int position) {
return position;
}
public View getView(int position, View convertView, ViewGroup parent) {
//ReflectionImage image = new ReflectionImage(mContext);
ImageView image = new ImageView(mContext);
image.setImageResource(mImageIds[position]);
image.setLayoutParams(new CoverFlow.LayoutParams(96, 76));
image.setScaleType(ImageView.ScaleType.CENTER_INSIDE);
// Make sure we set anti-aliasing otherwise we get jaggies
BitmapDrawable drawable = (BitmapDrawable) image.getDrawable();
drawable.setAntiAlias(true);
return image;
}
public float getScale(boolean focused, int offset) {
return Math.max(0, 1.0f / (float) Math.pow(2, Math.abs(offset)));
}
}
}
ReflectionImage
import android.content.Context; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.graphics.Canvas; import android.graphics.LinearGradient; import android.graphics.Matrix; import android.graphics.Paint; import android.graphics.PorterDuffXfermode; import android.graphics.Bitmap.Config; import android.graphics.PorterDuff.Mode; import android.graphics.Shader.TileMode; import android.graphics.drawable.BitmapDrawable; import android.util.AttributeSet; import android.widget.ImageView; /** * * @author */ public class ReflectionImage extends ImageView { //是否为Reflection模式 private boolean mReflectionMode = true; public ReflectionImage(Context context) { super(context); } public ReflectionImage(Context context, AttributeSet attrs) { super(context, attrs); //取得原始图片的bitmap并重画 Bitmap originalImage = ((BitmapDrawable)this.getDrawable()).getBitmap(); doReflection(originalImage); } public ReflectionImage(Context context, AttributeSet attrs, int defStyle) { super(context, attrs, defStyle); Bitmap originalImage = ((BitmapDrawable)this.getDrawable()).getBitmap(); doReflection(originalImage); } public void setReflectionMode(boolean isRef) { mReflectionMode = isRef; } public boolean getReflectionMode() { return mReflectionMode; } //偷懒了,只重写了setImageResource,和构造函数里面干了同样的事情 @Override public void setImageResource(int resId) { Bitmap originalImage = BitmapFactory.decodeResource( getResources(), resId); doReflection(originalImage); //super.setImageResource(resId); } private void doReflection(Bitmap originalImage) { final int reflectionGap = 4; //原始图片和反射图片中间的间距 int width = originalImage.getWidth(); int height = originalImage.getHeight(); //反转 Matrix matrix = new Matrix(); matrix.preScale(1, -1); //reflectionImage就是下面透明的那部分,可以设置它的高度为原始的3/4,这样效果会更好些 Bitmap reflectionImage = Bitmap.createBitmap(originalImage, 0, 0, width, height, matrix, false); //创建一个新的bitmap,高度为原来的两倍 Bitmap bitmapWithReflection = Bitmap.createBitmap(width, (height + height), Config.ARGB_8888); Canvas canvasRef = new Canvas(bitmapWithReflection); //先画原始的图片 canvasRef.drawBitmap(originalImage, 0, 0, null); //画间距 Paint deafaultPaint = new Paint(); canvasRef.drawRect(0, height, width, height + reflectionGap, deafaultPaint); //画被反转以后的图片 canvasRef.drawBitmap(reflectionImage, 0, height + reflectionGap, null); // 创建一个渐变的蒙版放在下面被反转的图片上面 Paint paint = new Paint(); LinearGradient shader = new LinearGradient(0, originalImage.getHeight(), 0, bitmapWithReflection.getHeight() + reflectionGap, 0x80ffffff, 0x00ffffff, TileMode.CLAMP); // Set the paint to use this shader (linear gradient) paint.setShader(shader); // Set the Transfer mode to be porter duff and destination in paint.setXfermode(new PorterDuffXfermode(Mode.DST_IN)); // Draw a rectangle using the paint with our linear gradient canvasRef.drawRect(0, height, width, bitmapWithReflection.getHeight() + reflectionGap, paint); //调用ImageView中的setImageBitmap this.setImageBitmap(bitmapWithReflection); } }