目录
GitHub - bricke/Qt-AES: Native Qt AES encryption class
Qt 之 Aes CBC 加密 (有实例)
----------------------------------------------
关联参考:
Qt之Base64编解码 (***)
https://blog.csdn.net/ken2232/article/details/131990713
什么是base64
Base64是一种用64个字符来表示任意二进制数据的方法(就像ASCII码一样,是一种数据标准)
注:base64 是什么?
1. 说白了,就是 64进制。
和 十进制,二进制,八进制,十六进制一样,都是同样的东西,都属于数学计数系统,只不过它们之间的 基数 不同。如果仅仅从计数的角度而言,就是 基数不同,仅此而已。
2. 它们之间的根本区别,是什么?主要用途不同。
不同的计数基数,导致了它们的主要用途的不同。
十进制,主要用于人们的日常计数;
二进制,八进制,十六进制:主要应用在计算机领域;
base64:主要应用在计算机领域,可以将二进制的数据,采用可显示的字符来表示。
二进制数据,通常会包含不可显示的字符,如换行、回车等等,这类不可显示的数据,在某些应用场景里,会造成数据在通用型解释器中,一般都会产生错误,除非采用特别定制场景的专用数据解释器。
3. 只要想,任何进制的数学计数系统,人们都可以创造出来。
但是,是否实用,那就不一定了。
比如:人们日常采用的计数系统,是十进制;假如规定二进制作为人们日常计数的标准系统的话,那么,苹果:1111 块钱/斤 (二) == 15 块钱/斤 (十) ,这种计数系统,基数太少,其表达的效率就太低了。
绝大多数的人类,天生具有 十个手指,十个脚趾。因此,在日常生活中,采用十进制具有先天的优势。
而计算机的电路,通常采用 0/1来表示,因此,二进制则是计算机数学的基础。虽然人类曾经研发除了 采用 3种状态的电路,但这种电路没有流行起来,因此,三进制也就没有流行起来。世事无绝对,也许未来的量子计算机,可以让三进制,或是其他进制的计数系统流行起来呢?
//16进制字节流转为Base64
QByteArray toBase64(Base64Options options) const;
QByteArray toBase64() const; // ### Qt6 merge with previous//Base64转为16进制字节流
static QByteArray fromBase64(const QByteArray &base64, Base64Options options);
static QByteArray fromBase64(const QByteArray &base64); // ### Qt6 merge with previous
/// https://blog.51cto.com/wuquan1230/4760520
Qt中使用base64
// base64
// 编码
QByteArray base = "你好, 世界";
base = base.toBase64();
qDebug() << base;
// 解码
base= QByteArray::fromBase64(base);
qDebug() << base.data();
#include "aesni/qaesencryption.h"
// 加密
QString Dialog_login::AES_encryption(const QString &data, const QString &key)
{
QAESEncryption encryption(QAESEncryption::AES_128, QAESEncryption::ECB, QAESEncryption::PKCS7);
QByteArray enBA = encryption.encode(data.toUtf8(), key.toUtf8());
return QString::fromLatin1(enBA.toBase64()); //以便网络传输,或保存在 txt型文件中
}
// 解密
QString Dialog_login::AES_decryption(const QString &data, const QString &key)
{
QAESEncryption encryption(QAESEncryption::AES_128, QAESEncryption::ECB, QAESEncryption::PKCS7);
QByteArray enBA = QByteArray::fromBase64(data.toUtf8());
QByteArray deBA = encryption.decode(enBA, key.toUtf8());
return QString::fromLatin1(QAESEncryption::RemovePadding(deBA, QAESEncryption::PKCS7));
}
----------------------------------------------
参考:
https://blog.csdn.net/ken2232/article/details/131982924
AES五种加密模式(CBC、ECB、CTR、OCF、CFB)
分组密码有五种工作体制:
1.电码本模式(Electronic Codebook Book (ECB));
2.密码分组链接模式(Cipher Block Chaining (CBC));
3.计算器模式(Counter (CTR));
4.密码反馈模式(Cipher FeedBack (CFB));
5.输出反馈模式(Output FeedBack (OFB))。
----------------------------------------------
GitHub - bricke/Qt-AES: Native Qt AES encryption class
用法:
#include <QCoreApplication>
#include <QTest>#ifdef __cplusplus
#include "unit_test/aestest.h"
#endifint main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
AesTest test1;
return QTest::qExec(&test1);
}
#ifndef AESTEST_H
#define AESTEST_H#include <QObject>
#include <QByteArray>
#include <QTest>class AesTest : public QObject
{
Q_OBJECT
private slots:
void initTestCase();void ECB128Crypt();
void ECB128Decrypt();void ECB192Crypt();
void ECB192Decrypt();void ECB256Crypt();
void ECB256Decrypt();void ECB256String();
void CBC128Crypt();
void CBC128Decrypt();void CFB256String();
void CFB256SmallSizeText();
void CFB256MediumSizeText();
void CFB256LargeSizeText();
void CFB256XLargeSizeText();void OFB128Crypt();
void OFB256String();void CBC256StringEvenISO();
void CBC256StringEvenPKCS7();void cleanupTestCase(){}
private:
QByteArray key16;
QByteArray key24;
QByteArray key32;
QByteArray iv;
QByteArray in;
QByteArray outECB128;
QByteArray outECB192;
QByteArray outECB256;
QByteArray inCBC128;
QByteArray outCBC128;
QByteArray outOFB128;
};#endif // AESTEST_H
注意:
1. 由于源码放置的目录不同,因此,用户需要自行修改头文件的目录。
2. 当采用用户自定义的目录时,由于个别的源码目录构造的问题,导致所获得的 fullFileName不正确;于是,个别的验证也就不正确了。
3.在跨平台使用时,文件名的编码,有时会被解压缩软件之类的东西,给修改了,导致莫名的错误。
///直接采用变量演示的函数:移植 OK
void AesTest::CFB256String()
{
QAESEncryption encryption(QAESEncryption::AES_256, QAESEncryption::CFB, QAESEncryption::PKCS7);QString inputStr("The Advanced Encryption Standard (AES), also known by its original name Rijndael ");
QString key("123456789123");QByteArray hashKey = QCryptographicHash::hash(key.toLocal8Bit(), QCryptographicHash::Sha256);
QByteArray encodeText = encryption.encode(inputStr.toLocal8Bit(), hashKey, iv);
QByteArray decodedText = encryption.removePadding(encryption.decode(encodeText, hashKey, iv));
QCOMPARE(QString(decodedText), inputStr);
}
///采用打开文件演示的函数:移植 NG,需要修改文件的路径
void AesTest::CFB256SmallSizeText()
{
QAESEncryption encryption(QAESEncryption::AES_256, QAESEncryption::CFB);QFile textFile(":/unit_test/alices-in-wonderland.txt");
QByteArray input;
if (textFile.open(QFile::ReadOnly))
input = textFile.readAll();
else
QFAIL("File alices-in-wonderland.txt not found!");QString key("123456789123");
QByteArray hashKey = QCryptographicHash::hash(key.toLocal8Bit(), QCryptographicHash::Sha256);
QByteArray encodeText = encryption.encode(input, hashKey, iv);
QByteArray decodedText = encryption.removePadding(encryption.decode(encodeText, hashKey, iv));
QCOMPARE(decodedText, input);
}
注意:
1. QFile textFile(":/unit_test/alices-in-wonderland.txt"); 使用的是资源路径,只能读。
2. 当该文件被删除之后,会重新建立一个空的、新文件。
3. 空文件问题
当文件是“空”的,可能会导致错误的结果,即会错误地通过验证,这个需要测试和调整。
=====================================
Qt 之 Aes CBC 加密 (有实例)
Qt笔记-AES加密_qt aes加密_IT1995的博客-CSDN博客
#include <QCoreApplication>
#include <QCryptographicHash>
#include <QVector>
#include <QDebug>
#include "QAesEncryption.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QString key = "0123456789012345";
qDebug() << "key.size : " << key.toUtf8().size();
QString string = "Hello World";
QAESEncryption encryption(QAESEncryption::AES_128, QAESEncryption::ECB, QAESEncryption::PKCS7);
QByteArray enBA = encryption.encode(string.toUtf8(), key.toUtf8());
QByteArray enBABase64 = enBA.toBase64();
qDebug() << "string : " << string;
qDebug() << "enBA : " << enBABase64;
enBA = QByteArray::fromBase64(enBABase64);
QByteArray deBA = encryption.decode(enBA, key.toUtf8());
//移除填充
qDebug() << "deBA : " << QAESEncryption::RemovePadding(deBA, QAESEncryption::PKCS7);
return a.exec();
}
=====================
Qt MD5 和AES 加密 (有github源码)
一 、MD5 加密
#include <QCryptographicHash>
// MD5 加密
QString Dialog_login::MD5_encryption(const QString &data)
{
QCryptographicHash md5(QCryptographicHash::Md5); //使用MD5加密
md5.addData(data.toUtf8(),data.size()); // 添加数据
QByteArray bArry = md5.result(); //获取MD5加密后的密码
QString md5_date;
md5_data.append(bArry.toHex());
return md5_data;
}
二、AES 加密和解密
1、下载库:GitHub - bricke/Qt-AES: Native Qt AES encryption class
下载后需要下面5个文件,把这些文件复制出来放到一个文件夹下:
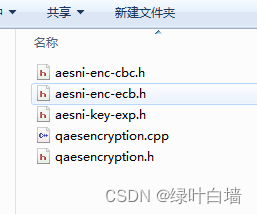
2、 qt 工程添加这些文件
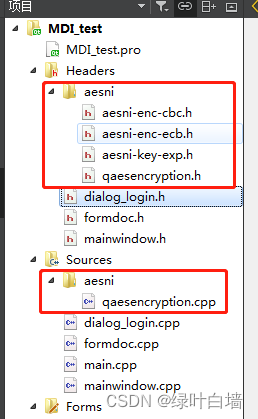
3. 编写加密解密代码
注意:
加密后的密文,大概率都会包含有不可见字符?在保存成 txt类型的密码文件后,会产生错误。
因此,需要将 QByteArray 型的密文转换为 Base64编码 来保存。
Base64编码的作用,是:把数据先做一个Base64编码,统统变成可见字符。
#include "aesni/qaesencryption.h"
// 加密
QString Dialog_login::AES_encryption(const QString &data, const QString &key)
{
QAESEncryption encryption(QAESEncryption::AES_128, QAESEncryption::ECB, QAESEncryption::PKCS7);
QByteArray enBA = encryption.encode(data.toUtf8(), key.toUtf8());
return QString::fromLatin1(enBA.toBase64());
}
// 解密
QString Dialog_login::AES_decryption(const QString &data, const QString &key)
{
QAESEncryption encryption(QAESEncryption::AES_128, QAESEncryption::ECB, QAESEncryption::PKCS7);
QByteArray enBA = QByteArray::fromBase64(data.toUtf8());
QByteArray deBA = encryption.decode(enBA, key.toUtf8());
return QString::fromLatin1(QAESEncryption::RemovePadding(deBA, QAESEncryption::PKCS7));
}
#include "aesni/qaesencryption.h"
// 加密
QString Dialog_login::AES_encryption(const QString &data, const QString &key)
{
QAESEncryption encryption(QAESEncryption::AES_128, QAESEncryption::ECB, QAESEncryption::PKCS7);
QByteArray enBA = encryption.encode(data.toUtf8(), key.toUtf8());
return QString::fromLatin1(enBA.toBase64());
}
// 解密
QString Dialog_login::AES_decryption(const QString &data, const QString &key)
{
QAESEncryption encryption(QAESEncryption::AES_128, QAESEncryption::ECB, QAESEncryption::PKCS7);
QByteArray enBA = QByteArray::fromBase64(data.toUtf8());
QByteArray deBA = encryption.decode(enBA, key.toUtf8());
return QString::fromLatin1(QAESEncryption::RemovePadding(deBA, QAESEncryption::PKCS7));
}
————————————————
版权声明:本文为CSDN博主「绿叶白墙」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明。
官方例子解析
目录结构
main.cpp
/// main.cpp
//
#include <QCoreApplication>
#include <QTest>#ifdef __cplusplus
#include "unit_test/aestest.h"
#endifint main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
AesTest test1; // 步骤 1:程序入口;定义 AesTest类
return QTest::qExec(&test1); // 步骤 2:执行测试程序
}
....../Qt-AES-master/unit_test/aestest.h
/// .../Qt-AES-master/unit_test/aestest.h
//
#ifndef AESTEST_H
#define AESTEST_H#include <QObject>
#include <QByteArray>
#include <QTest>class AesTest : public QObject // 步骤 x1:AesTest类 的声明
{
Q_OBJECT///
private slots: /// 这是测试函数群,在私有槽函数中 运行
void initTestCase(); // 步骤 x2:初始化
void ECB128Crypt(); // 步骤 x3:各种测试方法的成员函数声明
void ECB128Decrypt();
void ECB192Crypt();
void ECB192Decrypt();void ECB256Crypt();
void ECB256Decrypt();void ECB256String();
void CBC128Crypt();
void CBC128Decrypt();void CFB256String();
void CFB256SmallSizeText();
void CFB256MediumSizeText();
void CFB256LargeSizeText();
void CFB256XLargeSizeText();void OFB128Crypt();
void OFB256String();void CBC256StringEvenISO();
void CBC256StringEvenPKCS7();void cleanupTestCase(){}
private:
QByteArray key16;
QByteArray key24;
QByteArray key32;
QByteArray iv;
QByteArray in;
QByteArray outECB128;
QByteArray outECB192;
QByteArray outECB256;
QByteArray inCBC128;
QByteArray outCBC128;
QByteArray outOFB128;
};#endif // AESTEST_H
....../Qt-AES-master/unit_test/aestest.cpp
void AesTest::CFB256XLargeSizeText() // 步骤 x4:加密与解密 的测试函数
{
QAESEncryption encryption(QAESEncryption::AES_256, QAESEncryption::CFB);QFile textFile(":/unit_test/shakespeare-complete-works.txt"); // 步骤 x5:用于加密测试的明文文件
QByteArray input;
if (textFile.open(QFile::ReadOnly))
input = textFile.readAll();
else
QFAIL("File shakespeare-complete-works.txt not found!");QString key("123456789123"); // 步骤 x6:密钥
QByteArray hashKey = QCryptographicHash::hash(key.toLocal8Bit(), QCryptographicHash::Sha256);
QByteArray encodeText = encryption.encode(input, hashKey, iv); // 步骤 x6:加密
QByteArray decodedText = encryption.removePadding(encryption.decode(encodeText, hashKey, iv)); // 步骤 x7:解密
QCOMPARE(decodedText, input); // 步骤 x8:原始明文 与 解密后的明文 的比较
}
/// ":/unit_test/la-divina-commedia.txt" /// 附加 参考 的函数
void AesTest::CFB256MediumSizeText()
{
QAESEncryption encryption(QAESEncryption::AES_256, QAESEncryption::CFB);/// 读入外部文件
QFile textFile(":/unit_test/la-divina-commedia.txt");
QByteArray input;
if (textFile.open(QFile::ReadOnly))
input = textFile.readAll(); /// 读入的 明文
else
QFAIL("File la-divina-commedia.txt not found!");QString key("123456789123"); /// 密码
QByteArray hashKey = QCryptographicHash::hash(key.toLocal8Bit(), QCryptographicHash::Sha256);
/// 加密
QByteArray encodeText = encryption.encode(input, hashKey, iv); /// 加密的 密文/// 解密
QByteArray decodedText = encryption.removePadding(encryption.decode(encodeText, hashKey, iv)); /// 解密的 明文
QCOMPARE(decodedText, input); /// 比较:解密的明文 与 加密的密文
}
....../Qt-AES-master/Headers/qaesencryption.h
///....../Qt-AES-master/Headers/qaesencryption.h
//
#ifndef QAESENCRYPTION_H
#define QAESENCRYPTION_H#ifdef QtAES_EXPORTS
#include "qtaes_export.h"
#else
#define QTAESSHARED_EXPORT
#endif#include <QObject>
#include <QByteArray>#ifdef __linux__
#ifndef __LP64__
#define do_rdtsc _do_rdtsc
#endif
#endifclass QTAESSHARED_EXPORT QAESEncryption : public QObject
{
Q_OBJECT
public:
enum Aes {
AES_128,
AES_192,
AES_256
};
/// 参考:
/// https://blog.csdn.net/ken2232/article/details/131982924
/// AES五种加密模式(CBC、ECB、CTR、OCF、CFB)
enum Mode {
ECB,
CBC,
CFB,
OFB
};enum Padding {
ZERO,
PKCS7,
ISO
};//--------------------------
/*!
* \brief static method call to encrypt data given by rawText
* \param level: AES::Aes level
* \param mode: AES::Mode mode
* \param rawText: input text
* \param key: user-key (key.size either 128, 192, 256 bits depending on AES::Aes)
* \param iv: initialisation-vector (iv.size is 128 bits (16 Bytes))
* \param padding: AES::Padding standard
* \return encrypted cipher
*/
static QByteArray Crypt(QAESEncryption::Aes level,QAESEncryption::Mode mode,
const QByteArray &rawText,
const QByteArray &key,
const QByteArray &iv = QByteArray(),
QAESEncryption::Padding padding = QAESEncryption::ISO);
//--------------------------
/*!
* \brief static method call to decrypt data given by rawText
* \param level: AES::Aes level
* \param mode: AES::Mode mode
* \param rawText: input text
* \param key: user-key (key.size either 128, 192, 256 bits depending on AES::Aes)
* \param iv: initialisation-vector (iv.size is 128 bits (16 Bytes))
* \param padding: AES::Padding standard
* \return decrypted cipher with padding
*/
static QByteArray Decrypt(QAESEncryption::Aes level,QAESEncryption::Mode mode,
const QByteArray &rawText,
const QByteArray &key,
const QByteArray &iv = QByteArray(),QAESEncryption::Padding padding = QAESEncryption::ISO);
//--------------------------
/*!
* \brief static method call to expand the user key to fit the encrypting/decrypting algorithm
* \param level: AES::Aes level
* \param mode: AES::Mode mode
* \param key: user-key (key.size either 128, 192, 256 bits depending on AES::Aes)
* \param expKey: output expanded key
* \param isEncryptionKey: always 'true' || only 'false' when DECRYPTING in CBC or EBC mode with aesni (check if supported)
* \return AES-ready key
*/
static QByteArray ExpandKey(QAESEncryption::Aes level, QAESEncryption::Mode mode, const QByteArray &key, bool isEncryptionKey);//--------------------------
/*!
* \brief static method call to remove padding from decrypted cipher given by rawText
* \param rawText: inputText
* \param padding: AES::Padding standard
* \return decrypted cipher with padding removed
*/
static QByteArray RemovePadding(const QByteArray &rawText,QAESEncryption::Padding padding = QAESEncryption::ISO);
QAESEncryption(QAESEncryption::Aes level,
QAESEncryption::Mode mode,
QAESEncryption::Padding padding = QAESEncryption::ISO);//--------------------------
/*!
* \brief object method call to encrypt data given by rawText
* \param rawText: input text
* \param key: user-key (key.size either 128, 192, 256 bits depending on AES::Aes)
* \param iv: initialisation-vector (iv.size is 128 bits (16 Bytes))
* \return encrypted cipher
*/
QByteArray encode(const QByteArray &rawText,const QByteArray &key,
const QByteArray &iv = QByteArray());
//--------------------------
/*!
* \brief object method call to decrypt data given by rawText
* \param rawText: input text
* \param key: user-key (key.size either 128, 192, 256 bits depending on AES::Aes)
* \param iv: initialisation-vector (iv.size is 128 bits (16 Bytes))
* \param padding: AES::Padding standard
* \return decrypted cipher with padding
*/
QByteArray decode(const QByteArray &rawText,const QByteArray &key,
const QByteArray &iv = QByteArray());
//--------------------------
/*!
* \brief object method call to expand the user key to fit the encrypting/decrypting algorithm
* \param key: user-key (key.size either 128, 192, 256 bits depending on AES::Aes)
* \param isEncryptionKey: always 'true' || only 'false' when DECRYPTING in CBC or EBC mode with aesni (check if supported)
* \return AES-ready key
*/
QByteArray expandKey(const QByteArray &key, bool isEncryptionKey);//--------------------------
/*!
* \brief object method call to remove padding from decrypted cipher given by rawText
* \param rawText: inputText
* \return decrypted cipher with padding removed
*/
QByteArray removePadding(const QByteArray &rawText);QByteArray printArray(uchar *arr, int size);
//--------------------------
Q_SIGNALS:public Q_SLOTS:
private:
int m_nb;
int m_blocklen;
int m_level;
int m_mode;
int m_nk;
int m_keyLen;
int m_nr;
int m_expandedKey;
int m_padding;
bool m_aesNIAvailable;
QByteArray* m_state;struct AES256{
int nk = 8;
int keylen = 32;
int nr = 14;
int expandedKey = 240;
int userKeySize = 256;
};struct AES192{
int nk = 6;
int keylen = 24;
int nr = 12;
int expandedKey = 209;
int userKeySize = 192;
};struct AES128{
int nk = 4;
int keylen = 16;
int nr = 10;
int expandedKey = 176;
int userKeySize = 128;
};//
quint8 getSBoxValue(quint8 num){return sbox[num];}
quint8 getSBoxInvert(quint8 num){return rsbox[num];}void addRoundKey(const quint8 round, const QByteArray &expKey);
void subBytes();
void shiftRows();
void mixColumns();
void invMixColumns();
void invSubBytes();
void invShiftRows();
QByteArray getPadding(int currSize, int alignment);
QByteArray cipher(const QByteArray &expKey, const QByteArray &in);
QByteArray invCipher(const QByteArray &expKey, const QByteArray &in);
QByteArray byteXor(const QByteArray &a, const QByteArray &b);//
const quint8 sbox[256] = {
//0 1 2 3 4 5 6 7 8 9 A B C D E F
0x63, 0x7c, 0x77, 0x7b, 0xf2, 0x6b, 0x6f, 0xc5, 0x30, 0x01, 0x67, 0x2b, 0xfe, 0xd7, 0xab, 0x76,
0xca, 0x82, 0xc9, 0x7d, 0xfa, 0x59, 0x47, 0xf0, 0xad, 0xd4, 0xa2, 0xaf, 0x9c, 0xa4, 0x72, 0xc0,
0xb7, 0xfd, 0x93, 0x26, 0x36, 0x3f, 0xf7, 0xcc, 0x34, 0xa5, 0xe5, 0xf1, 0x71, 0xd8, 0x31, 0x15,
0x04, 0xc7, 0x23, 0xc3, 0x18, 0x96, 0x05, 0x9a, 0x07, 0x12, 0x80, 0xe2, 0xeb, 0x27, 0xb2, 0x75,
0x09, 0x83, 0x2c, 0x1a, 0x1b, 0x6e, 0x5a, 0xa0, 0x52, 0x3b, 0xd6, 0xb3, 0x29, 0xe3, 0x2f, 0x84,
0x53, 0xd1, 0x00, 0xed, 0x20, 0xfc, 0xb1, 0x5b, 0x6a, 0xcb, 0xbe, 0x39, 0x4a, 0x4c, 0x58, 0xcf,
0xd0, 0xef, 0xaa, 0xfb, 0x43, 0x4d, 0x33, 0x85, 0x45, 0xf9, 0x02, 0x7f, 0x50, 0x3c, 0x9f, 0xa8,
0x51, 0xa3, 0x40, 0x8f, 0x92, 0x9d, 0x38, 0xf5, 0xbc, 0xb6, 0xda, 0x21, 0x10, 0xff, 0xf3, 0xd2,
0xcd, 0x0c, 0x13, 0xec, 0x5f, 0x97, 0x44, 0x17, 0xc4, 0xa7, 0x7e, 0x3d, 0x64, 0x5d, 0x19, 0x73,
0x60, 0x81, 0x4f, 0xdc, 0x22, 0x2a, 0x90, 0x88, 0x46, 0xee, 0xb8, 0x14, 0xde, 0x5e, 0x0b, 0xdb,
0xe0, 0x32, 0x3a, 0x0a, 0x49, 0x06, 0x24, 0x5c, 0xc2, 0xd3, 0xac, 0x62, 0x91, 0x95, 0xe4, 0x79,
0xe7, 0xc8, 0x37, 0x6d, 0x8d, 0xd5, 0x4e, 0xa9, 0x6c, 0x56, 0xf4, 0xea, 0x65, 0x7a, 0xae, 0x08,
0xba, 0x78, 0x25, 0x2e, 0x1c, 0xa6, 0xb4, 0xc6, 0xe8, 0xdd, 0x74, 0x1f, 0x4b, 0xbd, 0x8b, 0x8a,
0x70, 0x3e, 0xb5, 0x66, 0x48, 0x03, 0xf6, 0x0e, 0x61, 0x35, 0x57, 0xb9, 0x86, 0xc1, 0x1d, 0x9e,
0xe1, 0xf8, 0x98, 0x11, 0x69, 0xd9, 0x8e, 0x94, 0x9b, 0x1e, 0x87, 0xe9, 0xce, 0x55, 0x28, 0xdf,
0x8c, 0xa1, 0x89, 0x0d, 0xbf, 0xe6, 0x42, 0x68, 0x41, 0x99, 0x2d, 0x0f, 0xb0, 0x54, 0xbb, 0x16 };const quint8 rsbox[256] = {
0x52, 0x09, 0x6a, 0xd5, 0x30, 0x36, 0xa5, 0x38, 0xbf, 0x40, 0xa3, 0x9e, 0x81, 0xf3, 0xd7, 0xfb,
0x7c, 0xe3, 0x39, 0x82, 0x9b, 0x2f, 0xff, 0x87, 0x34, 0x8e, 0x43, 0x44, 0xc4, 0xde, 0xe9, 0xcb,
0x54, 0x7b, 0x94, 0x32, 0xa6, 0xc2, 0x23, 0x3d, 0xee, 0x4c, 0x95, 0x0b, 0x42, 0xfa, 0xc3, 0x4e,
0x08, 0x2e, 0xa1, 0x66, 0x28, 0xd9, 0x24, 0xb2, 0x76, 0x5b, 0xa2, 0x49, 0x6d, 0x8b, 0xd1, 0x25,
0x72, 0xf8, 0xf6, 0x64, 0x86, 0x68, 0x98, 0x16, 0xd4, 0xa4, 0x5c, 0xcc, 0x5d, 0x65, 0xb6, 0x92,
0x6c, 0x70, 0x48, 0x50, 0xfd, 0xed, 0xb9, 0xda, 0x5e, 0x15, 0x46, 0x57, 0xa7, 0x8d, 0x9d, 0x84,
0x90, 0xd8, 0xab, 0x00, 0x8c, 0xbc, 0xd3, 0x0a, 0xf7, 0xe4, 0x58, 0x05, 0xb8, 0xb3, 0x45, 0x06,
0xd0, 0x2c, 0x1e, 0x8f, 0xca, 0x3f, 0x0f, 0x02, 0xc1, 0xaf, 0xbd, 0x03, 0x01, 0x13, 0x8a, 0x6b,
0x3a, 0x91, 0x11, 0x41, 0x4f, 0x67, 0xdc, 0xea, 0x97, 0xf2, 0xcf, 0xce, 0xf0, 0xb4, 0xe6, 0x73,
0x96, 0xac, 0x74, 0x22, 0xe7, 0xad, 0x35, 0x85, 0xe2, 0xf9, 0x37, 0xe8, 0x1c, 0x75, 0xdf, 0x6e,
0x47, 0xf1, 0x1a, 0x71, 0x1d, 0x29, 0xc5, 0x89, 0x6f, 0xb7, 0x62, 0x0e, 0xaa, 0x18, 0xbe, 0x1b,
0xfc, 0x56, 0x3e, 0x4b, 0xc6, 0xd2, 0x79, 0x20, 0x9a, 0xdb, 0xc0, 0xfe, 0x78, 0xcd, 0x5a, 0xf4,
0x1f, 0xdd, 0xa8, 0x33, 0x88, 0x07, 0xc7, 0x31, 0xb1, 0x12, 0x10, 0x59, 0x27, 0x80, 0xec, 0x5f,
0x60, 0x51, 0x7f, 0xa9, 0x19, 0xb5, 0x4a, 0x0d, 0x2d, 0xe5, 0x7a, 0x9f, 0x93, 0xc9, 0x9c, 0xef,
0xa0, 0xe0, 0x3b, 0x4d, 0xae, 0x2a, 0xf5, 0xb0, 0xc8, 0xeb, 0xbb, 0x3c, 0x83, 0x53, 0x99, 0x61,
0x17, 0x2b, 0x04, 0x7e, 0xba, 0x77, 0xd6, 0x26, 0xe1, 0x69, 0x14, 0x63, 0x55, 0x21, 0x0c, 0x7d };// The round constant word array, Rcon[i], contains the values given by
// x to th e power (i-1) being powers of x (x is denoted as {02}) in the field GF(2^8)
// Only the first 14 elements are needed
const quint8 Rcon[14] = {
0x8d, 0x01, 0x02, 0x04, 0x08, 0x10, 0x20, 0x40, 0x80, 0x1b, 0x36, 0x6c, 0xd8, 0xab};
};#endif // QAESENCRYPTION_H