目录
setInitialValue()方法和initialValue()方法:
ThreadLocal理解:
如果在多线程并发环境中,一个可变对象涉及到共享与竞争,那么该可变对象就一定会涉及到线程间同步操作,这是多线程并发问题。
否则该可变对象将作为线程私有对象,可通过ThreadLocal进行管理,实现线程间私有对象隔离的目的。
可以发现,ThreadLocal并没有解决多线程并发的问题,因为ThreadLocal管理的可变对象的性质本来就不会涉及到多线程并发而引发的共享、竞争和同步问题,使用ThreadLocal管理只是方便了多线程获取和使用该私有可变对象的途径和方式。
ThreadLocal解决的问题:
将一个公共的可变对象,转换为线程私有的可变对象,防止出现不正确的共享。
《java并发变成实践》
线程本地(ThreadLocal)变量通常用于防止在基于可变的单体(Singleton)或全局变量的设计中,出现(不正确的)共享。
ThreadLocal的使用场景:
一个可变对象,在每一个线程(或者说多线程环境)中都会被使用,并且,该可变对象无需在各个线程间进行同步,那么,该可变对象就可以通过ThreadLocal进行管理,而此时该可变对象变成多实例对象,与线程一一对应。
ThreadLocal主要方法:
- public T get() { }返回当前线程所对应的线程局部变量
- public void set(T value) { }设置当前线程的线程局部变量的值
- public void remove(){} 将当前线程局部变量的值删除,目的是为了减少内存的占用,该方法是JDK 5.0新增的方法。需要指出的是,当线程结束后,对应该线程的局部变量将自动被垃圾回收,所以显式调用该方法清除线程的局部变量并不是必须的操作,但它可以加快内存回收的速度。
- protected T initialValue() { }返回该线程局部变量的初始值,该方法是一个protected的方法,显然是为了让子类覆盖而设计的。这个方法是一个延迟调用方法,在线程第1次调用get()或set(Object)时才执行,并且仅执行1次。ThreadLocal中的缺省实现直接返回一个null。
ThreadLocal源码分析:
get方法:
/**
* Returns the value in the current thread's copy of this
* thread-local variable. If the variable has no value for the
* current thread, it is first initialized to the value returned
* by an invocation of the {@link #initialValue} method.
*
* @return the current thread's value of this thread-local
*/
public T get() {
//获取当前线程对象
Thread t = Thread.currentThread();
//从当前线程对象中获取ThreadLocalMap类型实例对象
ThreadLocalMap map = getMap(t);
if (map != null) {
//this对象,也就是ThreadLocal对象作为map的key,获取ThreadLocal管理的可变对象
ThreadLocalMap.Entry e = map.getEntry(this);
if (e != null) {
@SuppressWarnings("unchecked")
T result = (T)e.value;
return result;
}
}
//map为空,延迟执行初始化方法
return setInitialValue();
}
getMap(t)方法:
/**
* Get the map associated with a ThreadLocal. Overridden in
* InheritableThreadLocal.
*
* @param t the current thread
* @return the map
*/
ThreadLocalMap getMap(Thread t) {
return t.threadLocals;
}
补充解释:
- 1、ThreadLocalMap,是ThreadLocal的静态内部类
- 2、ThreadLocalMap把其外部类ThreadLocal的实例对象作为key,把要管理的可变对象作为value
- 3、ThreadLocalMap的实例对象,由当前线程对象Thread的实例持有,而不是由ThreadLocal持有
如下图源码截图进行证明:
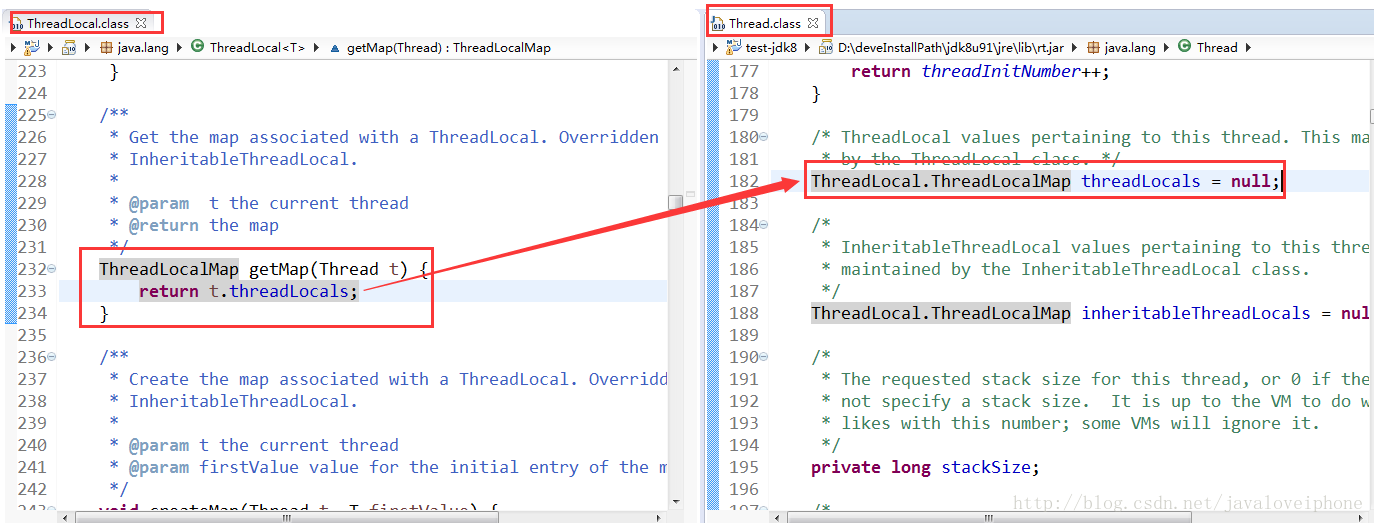
setInitialValue()方法和initialValue()方法:
/**
* Variant of set() to establish initialValue. Used instead
* of set() in case user has overridden the set() method.
*
* @return the initial value
*/
private T setInitialValue() {
T value = initialValue();
Thread t = Thread.currentThread();
ThreadLocalMap map = getMap(t);
if (map != null)
map.set(this, value);
else
createMap(t, value);
return value;
}
protected T initialValue() {
return null;
}
补充说明:
- 1、initialValue()方法声明为protected,目的就是让子类覆盖重新的,如果不覆盖重写,则返回null
- 2、如果没有重写initialValue()方法,ThreadLocal对象直接调用get()方法,最终从setInitialValue()返回的对象为null
createMap(t, value):
/**
* Create the map associated with a ThreadLocal. Overridden in
* InheritableThreadLocal.
*
* @param t the current thread
* @param firstValue value for the initial entry of the map
*/
void createMap(Thread t, T firstValue) {
//直接new一个新的ThreadLocalMap实例,封装进入当前线程对象t
t.threadLocals = new ThreadLocalMap(this, firstValue);
}
从上面代码可以发现,每一个线程对象都有一个专属自己的ThreadLocalMap对象,而ThreadLocalMap对象存储了ThreadLocal对象与变量对象。线程对象、ThreadLocalMap对象、ThreadLocal对象、变量对象之间的关系如下图:
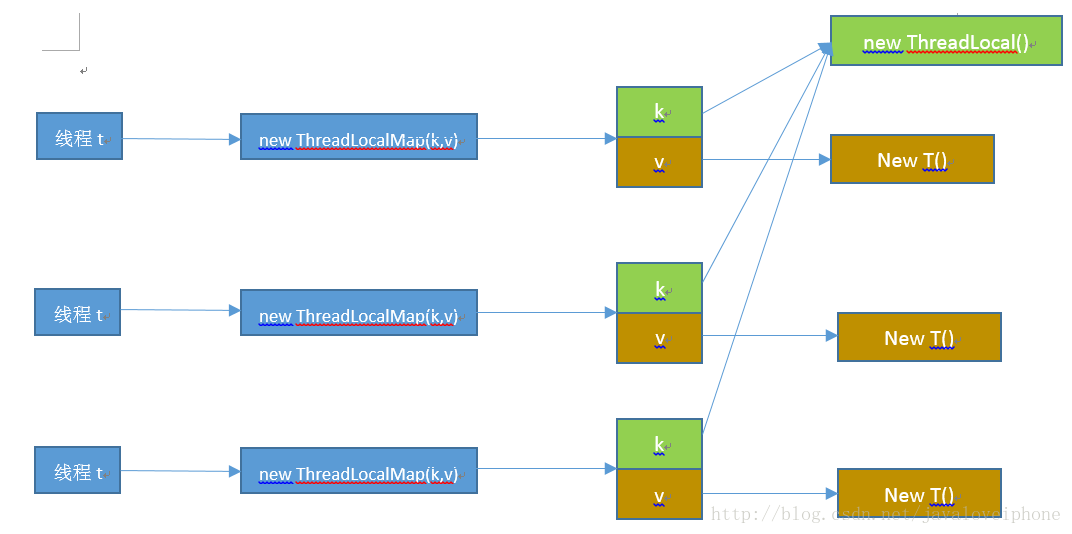
set(T value)方法:
/**
* Sets the current thread's copy of this thread-local variable
* to the specified value. Most subclasses will have no need to
* override this method, relying solely on the {@link #initialValue}
* method to set the values of thread-locals.
*
* @param value the value to be stored in the current thread's copy of
* this thread-local.
*/
public void set(T value) {
Thread t = Thread.currentThread();
ThreadLocalMap map = getMap(t);
if (map != null)
map.set(this, value);
else
createMap(t, value);
}
补充解释:
- 1、set方法用来修改或者初始化ThreadLocal管理的变量对象。
- 2、ThreadLocal对象调用get方法获取变量的,要么重写initialValue()方法,要么主动调用set方法,否则将返回null。
参考:
http://blog.csdn.net/lufeng20/article/details/24314381 (此文争议比较大,但依然可以作为理解ThreadLocal的素材)
http://www.cnblogs.com/dolphin0520/p/3920407.html
http://www.iteye.com/topic/103804