1
public
class
MyStack
{
2
private int[] a;
3
private int count = 0;
4
public final int MAX_SIZE;
5
6
public MyStack(int size)
{
7
MAX_SIZE = size;
8
a = new int[size];
9
count = 0;
10
}
11
12
public synchronized void push()
{
13
while(count==MAX_SIZE)
{//这里用while而不是if因为当被唤醒时,该线程处于锁池等待获取锁,这个时候可能会有别的该线改变数组的大小。所以唤醒时继续检查数组是否已满。
14
try
{
15
this.wait(); //释放掉当前的对象锁,在等待池等待
16
} catch (InterruptedException e)
{
17
e.printStackTrace();
18
}
19
}
20
a[count++]=count;
21
System.out.println(Thread.currentThread().getName()+"压入数据:"+count);
22
this.notify(); //唤醒生产者消费者线程
23
}
24
25
public synchronized int pop()
{
26
while(count ==0)
{
27
try
{
28
this.wait();
29
} catch (InterruptedException e)
{
30
e.printStackTrace();
31
}
32
}
33
this.notify();
34
System.out.println(Thread.currentThread().getName()+"弹出数据:"+count);
35
return a[--count];
36
}
37
}
38
/** */
/**
39
*生产者
40
**/
41
public
class
Producer
extends
Thread
{
42
43
private MyStack stack;
44
45
public Producer(MyStack stack)
{
46
this.stack = stack;
47
}
48
@Override
49
public void run()
{
50
while (true)
{
51
stack.push();
52
try
{
53
Thread.sleep(200);
54
} catch (InterruptedException e)
{
55
e.printStackTrace();
56
}
57
}
58
}
59
}
60
/** */
/**
61
*消费者
62
**/
63
public
class
Consumer
extends
Thread
{
64
65
private MyStack stack;
66
67
public Consumer(MyStack stack)
{
68
this.stack = stack;
69
}
70
71
@Override
72
public void run()
{
73
while(true)
{
74
stack.pop();
75
try
{
76
Thread.sleep(300);
77
} catch (InterruptedException e)
{
78
e.printStackTrace();
79
}
80
}
81
}
82
}



2

3

4

5

6



7

8

9

10

11

12



13



14



15

16



17

18

19

20

21

22

23

24

25



26



27



28

29



30

31

32

33

34

35

36

37

38


39

40

41



42

43

44

45



46

47

48

49



50



51

52



53

54



55

56

57

58

59

60


61

62

63



64

65

66

67



68

69

70

71

72



73



74

75



76

77



78

79

80

81

82

线程状态图
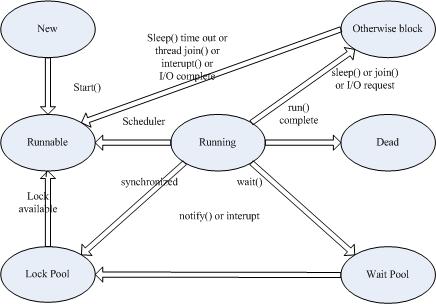