Android的TextView在显示文字的时候有个问题就是一行还没显示满就跳到下一行,原因是:
1) TextView在显示中文的时候 标点符号不能显示在一行的行首和行尾,如果一个标点符号刚好在一行的行尾,该标点符号就会连同前一个字符跳到下一行显示;
2)一个英文单词不能被显示在两行中( TextView在显示英文时,标点符号是可以放在行尾的,但英文单词也不能分开 );
如果只是想让标点符号可以显示在行尾,有一个简单的方法就是在标点符号后加一个空格,则该标点符号就可以显示在行尾了;
如果想要两端对齐的显示效果,有两种方法:
1)修改Android源代码;将frameworks/base/core/Java/android/text下的StaticLayout.java文件中的如下代码:
- if (c == ' ' || c == '/t' ||
- ((c == '.' || c == ',' || c == ':' || c == ';') &&
- (j - 1 < here || !Character.isDigit(chs[j - 1 - start])) &&
- (j + 1 >= next || !Character.isDigit(chs[j + 1 - start]))) ||
- ((c == '/' || c == '-') &&
- (j + 1 >= next || !Character.isDigit(chs[j + 1 - start]))) ||
- (c >= FIRST_CJK && isIdeographic(c, true) &&
- j + 1 < next && isIdeographic(chs[j + 1 - start], false))) {
- okwidth = w;
- ok = j + 1;
-
- if (fittop < oktop)
- oktop = fittop;
- if (fitascent < okascent)
- okascent = fitascent;
- if (fitdescent > okdescent)
- okdescent = fitdescent;
- if (fitbottom > okbottom)
- okbottom = fitbottom;
- }
去掉就可以了。去掉后标点符号可以显示在行首和行尾,英文单词也可以被分开在两行中显示。
2)自定义View显示文本
网上就有达人采用自定义View来解决这个问题,我做了实验并总结了一下:
自定义View的步骤:
1)继承View类或其子类,例子继承了TextView类;
2)写构造函数,通过XML获取属性(这一步中可以自定义属性,见例程);
3)重写父类的某些函数,一般都是以on开头的函数,例子中重写了onDraw()和onMeasure()函数;
=========================StartCustomTextView.java=============================
- public class StartCustomTextView extends TextView {
- public static int m_iTextHeight;
- public static int m_iTextWidth;
-
- private Paint mPaint = null;
- private String string="";
- private float LineSpace = 0;
- private int left_Margin;
- private int right_Margin;
- private int bottom_Margin;
-
- public StartCustomTextView(Context context, AttributeSet set)
- {
- super(context,set);
- DisplayMetrics displayMetrics = getResources().getDisplayMetrics();
- TypedArray typedArray = context.obtainStyledAttributes(set, R.styleable.CYTextView);
- int width = displayMetrics.widthPixels;
- left_Margin = 29;
- right_Margin = 29;
- bottom_Margin = 29;
- width = width - left_Margin -right_Margin;
- float textsize = typedArray.getDimension(R.styleable.CYTextView_textSize, 34);
- int textcolor = typedArray.getColor(R.styleable.CYTextView_textColor, getResources().getColor(R.color.white));
- float linespace = typedArray.getDimension(R.styleable.CYTextView_lineSpacingExtra, 15);
- int typeface = typedArray.getColor(R.styleable.CYTextView_typeface, 0);
-
- typedArray.recycle();
-
-
- m_iTextWidth=width;
- LineSpace=linespace;
-
-
- mPaint = new Paint();
- mPaint.setAntiAlias(true);
- mPaint.setColor(textcolor);
- mPaint.setTextSize(textsize);
- switch(typeface){
- case 0:
- mPaint.setTypeface(Typeface.DEFAULT);
- break;
- case 1:
- mPaint.setTypeface(Typeface.SANS_SERIF);
- break;
- case 2:
- mPaint.setTypeface(Typeface.SERIF);
- break;
- case 3:
- mPaint.setTypeface(Typeface.MONOSPACE);
- break;
- default:
- mPaint.setTypeface(Typeface.DEFAULT);
- break;
- }
-
- }
-
- @Override
- protected void onDraw(Canvas canvas)
- {
- super.onDraw(canvas);
-
- char ch;
- int w = 0;
- int istart = 0;
- int m_iFontHeight;
- int m_iRealLine=0;
- int x=2;
- int y=30;
-
- Vector m_String=new Vector();
-
- FontMetrics fm = mPaint.getFontMetrics();
- m_iFontHeight = (int) Math.ceil(fm.descent - fm.top) + (int)LineSpace;
-
- for (int i = 0; i < string.length(); i++)
- {
- ch = string.charAt(i);
- float[] widths = new float[1];
- String srt = String.valueOf(ch);
- mPaint.getTextWidths(srt, widths);
-
- if (ch == '\n'){
- m_iRealLine++;
- m_String.addElement(string.substring(istart, i));
- istart = i + 1;
- w = 0;
- }else{
- w += (int) (Math.ceil(widths[0]));
- if (w > m_iTextWidth){
- m_iRealLine++;
- m_String.addElement(string.substring(istart, i));
- istart = i;
- i--;
- w = 0;
- }else{
- if (i == (string.length() - 1)){
- m_iRealLine++;
- m_String.addElement(string.substring(istart, string.length()));
- }
- }
- }
- }
- m_iTextHeight=m_iRealLine*m_iFontHeight+2;
- canvas.setViewport(m_iTextWidth, m_iTextWidth);
- for (int i = 0, j = 0; i < m_iRealLine; i++, j++)
- {
- canvas.drawText((String)(m_String.elementAt(i)), x, y+m_iFontHeight * j, mPaint);
- }
- }
-
-
- protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec)
- {
- int measuredHeight = measureHeight(heightMeasureSpec);
- int measuredWidth = measureWidth(widthMeasureSpec);
- this.setMeasuredDimension(measuredWidth, measuredHeight);
- LayoutParams layout = new LinearLayout.LayoutParams(measuredWidth,measuredHeight);
- layout.leftMargin= left_Margin;
- layout.rightMargin= right_Margin;
- layout.bottomMargin= bottom_Margin;
- this.setLayoutParams(layout);
- super.onMeasure(widthMeasureSpec, heightMeasureSpec);
- }
-
- private int measureHeight(int measureSpec)
- {
- int specMode = MeasureSpec.getMode(measureSpec);
- int specSize = MeasureSpec.getSize(measureSpec);
-
- initHeight();
- int result = m_iTextHeight;
- if (specMode == MeasureSpec.AT_MOST){
-
-
-
-
- result = specSize;
- }else if (specMode == MeasureSpec.EXACTLY){
-
-
- }
- return result;
- }
-
- private void initHeight()
- {
-
- m_iTextHeight=0;
-
-
- FontMetrics fm = mPaint.getFontMetrics();
- int m_iFontHeight = (int) Math.ceil(fm.descent - fm.top) + (int)LineSpace;
- int line=0;
- int istart=0;
-
- int w=0;
- for (int i = 0; i < string.length(); i++)
- {
- char ch = string.charAt(i);
- float[] widths = new float[1];
- String srt = String.valueOf(ch);
- mPaint.getTextWidths(srt, widths);
-
- if (ch == '\n'){
- line++;
- istart = i + 1;
- w = 0;
- }else{
- w += (int) (Math.ceil(widths[0]));
- if (w > m_iTextWidth){
- line++;
- istart = i;
- i--;
- w = 0;
- }else{
- if (i == (string.length() - 1)){
- line++;
- }
- }
- }
- }
- m_iTextHeight=(line)*m_iFontHeight+2;
- }
-
- private int measureWidth(int measureSpec)
- {
- int specMode = MeasureSpec.getMode(measureSpec);
- int specSize = MeasureSpec.getSize(measureSpec);
-
-
- int result = 500;
- if (specMode == MeasureSpec.AT_MOST){
-
-
-
-
- result = specSize;
- }else if (specMode == MeasureSpec.EXACTLY){
-
- result = specSize;
- }
- return result;
- }
-
- public void SetText(String text)
- {
- string = text;
-
-
- }
- }
=======================attrs.xml===============================
该文件是自定义的属性,放在工程的res/values下
- <resources>
- <attr name="textwidth" format="integer"/>
- <attr name="typeface">
- <enum name="normal" value="0"/>
- <enum name="sans" value="1"/>
- <enum name="serif" value="2"/>
- <enum name="monospace" value="3"/>
- </attr>
-
- <declare-styleable name="CYTextView">
- <attr name="textwidth" />
- <attr name="textSize" format="dimension"/>
- <attr name="textColor" format="reference|color"/>
- <attr name="lineSpacingExtra" format="dimension"/>
- <attr name="typeface" />
- </declare-styleable>
- </resources>
=======================main.xml==========================
- <?xml version="1.0" encoding="utf-8"?>
- <ScrollView
- xmlns:Android="http://schemas.android.com/apk/res/android"
- Android:layout_width="320px"
- Android:layout_height="320px"
- Android:background="#ffffffff"
- >
- <LinearLayout
- xmlns:Android="http://schemas.android.com/apk/res/android"
- Android:orientation="vertical"
- Android:layout_width="fill_parent"
- Android:layout_height="fill_parent">
- <com.cy.CYTextView.CYTextView
- xmlns:cy="http://schemas.Android.com/apk/res/ com.cy.CYTextView "
- Android:id="@+id/mv"
- Android:layout_height="wrap_content"
- Android:layout_width="wrap_content"
- cy :textwidth="320"
- cy :textSize="24sp"
- cy :textColor="#aa000000"
- cy :lineSpacingExtra="15sp"
- cy :typeface="serif">
- </com. cy .CYTextView.CYTextView>
- </LinearLayout>
- </ScrollView>
蓝色代码即为自定义View,其中以cy命名空间开头的属性是自定义属性;
=======================Main.java=============================
- public class Main extends Activity {
- CYTextView mCYTextView;
- String text = "Android提供了精巧和有力的组件化模型构建用户的UI部分。主要是基于布局类:View和 ViewGroup。在此基础上,android平台提供了大量的预制的View和xxxViewGroup子 类,即布局(layout)和窗口小部件(widget)。可以用它们构建自己的UI。";
-
-
- @Override
- public void onCreate(Bundle savedInstanceState) {
- super.onCreate(savedInstanceState);
- this.setContentView(R.layout.main);
-
- mCYTextView = (CYTextView)findViewById(R.id.mv);
- mCYTextView.SetText(text);
- }
-
- }
转自:http://hi.baidu.com/java_rose/blog/item/2940a030d1ec7f3e96ddd847.html
另外一个人的自定义TextView,学习一下
4.1 可以封装一个自定义的textview,直接包含自动排版换行的功能:
1 package cc.snser.test;
2
3 import android.content.Context;
4 import android.graphics.Paint;
5 import android.text.TextUtils;
6 import android.util.AttributeSet;
7 import android.widget.TextView;
8
9 public class AutoSplitTextView extends TextView {
10 private boolean mEnabled = true;
11
12 public AutoSplitTextView(Context context) {
13 super(context);
14 }
15
16 public AutoSplitTextView(Context context, AttributeSet attrs) {
17 super(context, attrs);
18 }
19
20 public AutoSplitTextView(Context context, AttributeSet attrs, int defStyle) {
21 super(context, attrs, defStyle);
22 }
23
24 public void setAutoSplitEnabled(boolean enabled) {
25 mEnabled = enabled;
26 }
27
28 @Override
29 protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
30 if (MeasureSpec.getMode(widthMeasureSpec) == MeasureSpec.EXACTLY
31 && MeasureSpec.getMode(heightMeasureSpec) == MeasureSpec.EXACTLY
32 && getWidth() > 0
33 && getHeight() > 0
34 && mEnabled) {
35 String newText = autoSplitText(this);
36 if (!TextUtils.isEmpty(newText)) {
37 setText(newText);
38 }
39 }
40 super.onMeasure(widthMeasureSpec, heightMeasureSpec);
41 }
42
43 private String autoSplitText(final TextView tv) {
44 final String rawText = tv.getText().toString(); //原始文本
45 final Paint tvPaint = tv.getPaint(); //paint,包含字体等信息
46 final float tvWidth = tv.getWidth() - tv.getPaddingLeft() - tv.getPaddingRight(); //控件可用宽度
47
48 //将原始文本按行拆分
49 String [] rawTextLines = rawText.replaceAll("\r", "").split("\n");
50 StringBuilder sbNewText = new StringBuilder();
51 for (String rawTextLine : rawTextLines) {
52 if (tvPaint.measureText(rawTextLine) <= tvWidth) {
53 //如果整行宽度在控件可用宽度之内,就不处理了
54 sbNewText.append(rawTextLine);
55 } else {
56 //如果整行宽度超过控件可用宽度,则按字符测量,在超过可用宽度的前一个字符处手动换行
57 float lineWidth = 0;
58 for (int cnt = 0; cnt != rawTextLine.length(); ++cnt) {
59 char ch = rawTextLine.charAt(cnt);
60 lineWidth += tvPaint.measureText(String.valueOf(ch));
61 if (lineWidth <= tvWidth) {
62 sbNewText.append(ch);
63 } else {
64 sbNewText.append("\n");
65 lineWidth = 0;
66 --cnt;
67 }
68 }
69 }
70 sbNewText.append("\n");
71 }
72
73 //把结尾多余的\n去掉
74 if (!rawText.endsWith("\n")) {
75 sbNewText.deleteCharAt(sbNewText.length() - 1);
76 }
77
78 return sbNewText.toString();
79 }
80 }
1 package cc.snser.test;
2
3 import android.app.Activity;
4 import android.os.Bundle;
5
6 public class TestCActivity extends Activity {
7 private AutoSplitTextView mText;
8
9 @Override
10 protected void onCreate(Bundle savedInstanceState) {
11 super.onCreate(savedInstanceState);
12
13 setContentView(R.layout.testc);
14
15 mText = (AutoSplitTextView)findViewById(R.id.txt);
16 mText.setText("本文地址http://www.cnblogs.com/goagent/p/5159125.html本文地址啊本文。地址。啊http://www.cnblogs.com/goagent/p/5159125.html");
17 }
18 }
1 <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
2 xmlns:tools="http://schemas.android.com/tools"
3 android:layout_width="match_parent"
4 android:layout_height="match_parent"
5 android:background="@android:color/white"
6 android:orientation="vertical" >
7
8 <cc.snser.test.AutoSplitTextView
9 android:id="@+id/txt"
10 android:layout_width="match_parent"
11 android:layout_height="200dp"
12 android:layout_marginTop="11dp"
13 android:layout_marginLeft="11dp"
14 android:layout_marginRight="11dp"
15 android:background="@android:color/holo_blue_light"
16 android:textSize="20sp"
17 android:textColor="@android:color/black" />
18
19 </LinearLayout>
4.2 实现悬挂缩进
1 private String autoSplitText(final TextView tv, final String indent) {
2 final String rawText = tv.getText().toString(); //原始文本
3 final Paint tvPaint = tv.getPaint(); //paint,包含字体等信息
4 final float tvWidth = tv.getWidth() - tv.getPaddingLeft() - tv.getPaddingRight(); //控件可用宽度
5
6 //将缩进处理成空格
7 String indentSpace = "";
8 float indentWidth = 0;
9 if (!TextUtils.isEmpty(indent)) {
10 float rawIndentWidth = tvPaint.measureText(indent);
11 if (rawIndentWidth < tvWidth) {
12 while ((indentWidth = tvPaint.measureText(indentSpace)) < rawIndentWidth) {
13 indentSpace += " ";
14 }
15 }
16 }
17
18 //将原始文本按行拆分
19 String [] rawTextLines = rawText.replaceAll("\r", "").split("\n");
20 StringBuilder sbNewText = new StringBuilder();
21 for (String rawTextLine : rawTextLines) {
22 if (tvPaint.measureText(rawTextLine) <= tvWidth) {
23 //如果整行宽度在控件可用宽度之内,就不处理了
24 sbNewText.append(rawTextLine);
25 } else {
26 //如果整行宽度超过控件可用宽度,则按字符测量,在超过可用宽度的前一个字符处手动换行
27 float lineWidth = 0;
28 for (int cnt = 0; cnt != rawTextLine.length(); ++cnt) {
29 char ch = rawTextLine.charAt(cnt);
30 //从手动换行的第二行开始,加上悬挂缩进
31 if (lineWidth < 0.1f && cnt != 0) {
32 sbNewText.append(indentSpace);
33 lineWidth += indentWidth;
34 }
35 lineWidth += tvPaint.measureText(String.valueOf(ch));
36 if (lineWidth <= tvWidth) {
37 sbNewText.append(ch);
38 } else {
39 sbNewText.append("\n");
40 lineWidth = 0;
41 --cnt;
42 }
43 }
44 }
45 sbNewText.append("\n");
46 }
47
48 //把结尾多余的\n去掉
49 if (!rawText.endsWith("\n")) {
50 sbNewText.deleteCharAt(sbNewText.length() - 1);
51 }
52
53 return sbNewText.toString();
54 }
调用方式:
autoSplitText(tv, "1、");
悬挂缩进效果:
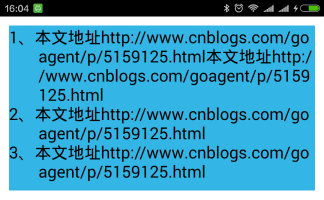
[转载请保留本文地址:http://www.cnblogs.com/snser/p/5159125.html]