Hibernate中,基于Annotation的简单树形结构的实现
1)Node.java
01 | package com.hibernate.model; |
03 | import java.util.HashSet; |
06 | import javax.persistence.CascadeType; |
07 | import javax.persistence.Entity; |
08 | import javax.persistence.FetchType; |
09 | import javax.persistence.GeneratedValue; |
10 | import javax.persistence.Id; |
11 | import javax.persistence.JoinColumn; |
12 | import javax.persistence.ManyToOne; |
13 | import javax.persistence.OneToMany; |
21 | private Set<Node> children = new HashSet<Node>(); |
28 | public void setId( int id) { |
31 | public String getName() { |
34 | public void setName(String name) { |
37 | @ManyToOne (cascade=CascadeType.ALL) |
38 | @JoinColumn (name= "nodeId" ) |
39 | public Node getParent() { |
42 | public void setParent(Node parent) { |
45 | @OneToMany (mappedBy= "parent" , cascade=CascadeType.ALL,fetch=FetchType.EAGER) |
46 | public Set<Node> getChildren() { |
49 | public void setChildren(Set<Node> children) { |
50 | this .children = children; |
2)hibernate.cfg.xml
01 | <?xml version= '1.0' encoding= 'UTF-8' ?> |
02 | <!DOCTYPE hibernate-configuration PUBLIC |
03 | "-//Hibernate/Hibernate Configuration DTD 3.0//EN" |
04 | "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd" > |
06 | <!-- Generated by MyEclipse Hibernate Tools. --> |
07 | <hibernate-configuration> |
10 | <property name= "dialect" >org.hibernate.dialect.MySQLDialect</property> |
11 | <property name= "connection.url" >jdbc:mysql: |
12 | <property name= "connection.username" >root</property> |
13 | <property name= "connection.password" > 123456 </property> |
14 | <property name= "connection.driver_class" >com.mysql.jdbc.Driver</property> |
15 | <property name= "myeclipse.connection.profile" >Mysql Driver</property> |
16 | <!-- Echo all executed SQL to stdout --> |
17 | <property name= "show_sql" > true </property> |
18 | <property name= "format_sql" > true </property> |
20 | <mapping class = "com.hibernate.model.Node" /> |
24 | </hibernate-configuration> |
3)log4j.properties
1 | log4j.rootLogger=info, stdout |
2 | log4j.logger.org.hibernate.test=info |
3 | log4j.appender.stdout=org.apache.log4j.ConsoleAppender |
4 | log4j.appender.stdout.Target=System.out |
5 | log4j.appender.stdout.layout=org.apache.log4j.PatternLayout |
6 | log4j.appender.stdout.layout.ConversionPattern=%d{ABSOLUTE} %5p %c{ 1 }:%L - %m%n |
7 | log4j.logger.org.hibernate.tool.hbm2ddl=debug |
4)HibernateTest.java
03 | import org.hibernate.Session; |
04 | import org.hibernate.SessionFactory; |
05 | import org.hibernate.cfg.AnnotationConfiguration; |
06 | import org.hibernate.tool.hbm2ddl.SchemaExport; |
07 | import org.junit.AfterClass; |
08 | import org.junit.BeforeClass; |
11 | import com.hibernate.model.Node; |
13 | public class HibernateTest { |
15 | private static SessionFactory sessionFactory; |
18 | public static void beforeClass() { |
19 | new SchemaExport( new AnnotationConfiguration().configure()).create( false , true ); |
20 | sessionFactory = new AnnotationConfiguration().configure().buildSessionFactory(); |
24 | public static void afterClass() { |
25 | sessionFactory.close(); |
28 | public void testSave() { |
29 | Session session = sessionFactory.openSession(); |
30 | Node node0 = new Node(); |
32 | Node node1 = new Node(); |
34 | Node node2 = new Node(); |
36 | Node node3 = new Node(); |
37 | node3.setName( "大儿子的大儿子" ); |
38 | Node node4 = new Node(); |
39 | node4.setName( "大儿子的小儿子" ); |
40 | Node node5 = new Node(); |
41 | node5.setName( "小儿子的大儿子" ); |
42 | Node node6 = new Node(); |
43 | node6.setName( "小儿子的小儿子" ); |
45 | node0.getChildren().add(node1); |
46 | node0.getChildren().add(node2); |
47 | node1.getChildren().add(node3); |
48 | node1.getChildren().add(node4); |
49 | node1.setParent(node0); |
50 | node2.getChildren().add(node5); |
51 | node2.getChildren().add(node6); |
52 | node2.setParent(node0); |
53 | node3.setParent(node1); |
54 | node4.setParent(node1); |
55 | node5.setParent(node2); |
56 | node6.setParent(node2); |
58 | session.beginTransaction(); |
60 | session.getTransaction().commit(); |
64 | public void testLoad() { |
66 | Session session = sessionFactory.openSession(); |
67 | session.beginTransaction(); |
68 | Node node = (Node)session.load(Node. class , 1 ); |
70 | session.getTransaction().commit(); |
74 | private void print(Node node, int level) { |
76 | for ( int i= 0 ;i<level;i++){ |
79 | System.out.println(preStr+node.getName()); |
80 | for (Node children:node.getChildren()){ |
81 | print(children,level+ 1 ); |
5)Console:
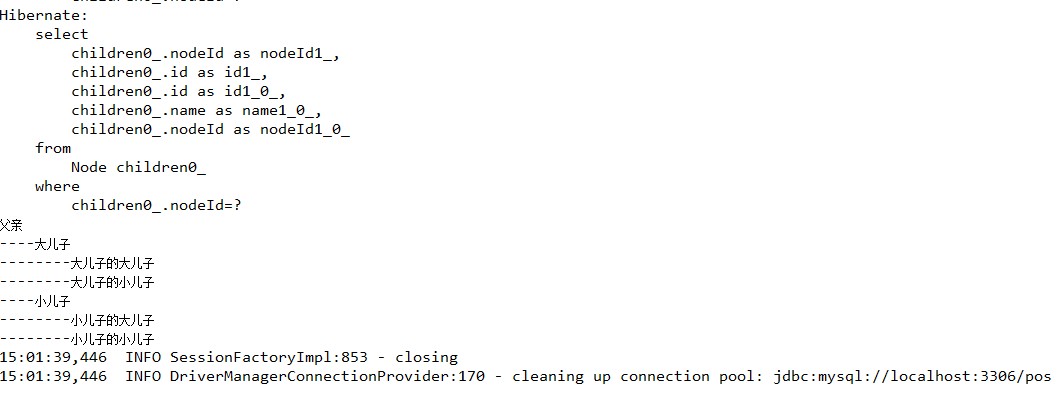
原文地址:http://my.oschina.net/huangcongmin12/blog/77577