最近需要用到EASYUI中的TREE功能,以前我是直接拼接成<UL><LI>发现这样拼完之后在更改树后对树的刷新不是很理想,现改用JSON格式,首先分析TREE中JOSN格式如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
[{
"id":1,
"text":"流程分类",
"children":[{
"id":11,
"text":"门禁流程分类",
"checked":true
},{
"id":113,
"text":"子门禁流程分类",
"children":[{
"id":1131,
"text":"子子门禁流程分类"
},{
"id": 8,
"text":"Async Folder",
"state":"closed"
}]
}]
},{
"id":3
"text":"行政",
"children":[{
"id":"31",
"text":"加班"
},{
"id":"33",
"text":"请假"
}]
}]
|
可以看出这种模式是由三个属性所组成,ID TEXT 集合,根据分析 我们需要对此模式建立一个BEAN的结构模型,建立TREENODE:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
|
package
com.odbpo.beans;
import
java.util.List;
public
class
TreeNode {
private
int
id;
private
String text;
private
int
pid;
private
List<TreeNode> children;
public
int
getPid() {
return
pid;
}
public
void
setPid(
int
pid) {
this
.pid = pid;
}
public
int
getId() {
return
id;
}
public
void
setId(
int
id) {
this
.id = id;
}
public
String getText() {
return
text;
}
public
void
setText(String text) {
this
.text = text;
}
public
List<TreeNode> getChildren() {
return
children;
}
public
void
setChildren(List<TreeNode> children) {
this
.children = children;
}
}
|
BEAN构建完成,那么接下来分析如何往BEAN里传数据,首先分析 数据库表中结构
1
2
3
4
5
|
create
table
depatment(
id,
--当前ID
pid,
--父ID
name
--显示名称
)
|
接下来我们要建立一个COM.UTIL包,所递归方法放置在这个包下,以便后续多次调用方便
建立类名为:JSONFACTORY
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
/*
* 以对象形式传回前台
*/
public
static
List<TreeNode> buildtree(List<TreeNode> nodes,
int
id){
List<TreeNode> treeNodes=
new
ArrayList<TreeNode>();
for
(TreeNode treeNode : nodes) {
TreeNode node=
new
TreeNode();
node.setId(treeNode.getId());
node.setText(treeNode.getText());
if
(id==treeNode.getPid()){
node.setChildren(buildtree(nodes, node.getId()));
treeNodes.add(node);
}
}
return
treeNodes;
}
|
完成以上工作后我们就要在控制器中使用在DAO中建立好的查询方法,这里DAO中写法就不细说了;
控制器写法如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
@RequestMapping
(
"/flow_tree"
)
@ResponseBody
public
List<TreeNode> getTree(){
List<TreeNode> nodes=
new
ArrayList<TreeNode>();
List<FlowSortTable> list_all=flowSortTableServiceImpl.findAll();
for
(FlowSortTable flowSortTable : list_all) {
TreeNode treeNode=
new
TreeNode();
treeNode.setId(flowSortTable.getSortId());
treeNode.setPid(flowSortTable.getSortPartmentId());
treeNode.setText(flowSortTable.getSortName());
nodes.add(treeNode);
}
List<TreeNode> treeNodes=JsonTreeFactory.buildtree(nodes,
0
);
return
treeNodes;
}
|
以上工作结束,我们就可以在前台使用EASYUITREE模式了
将此代码 放在$(document).ready(function(){})中
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
$(
"#tt1"
).tree({
url:
'${contextPath}/main/flow/flow_tree.html'
,
onClick:
function
(node){
$(
"#sort"
).css(
"display"
,
"block"
);
$(
"#save"
).hide();
$(
"#update"
).show();
odbpo_combobox(
"#flowType"
,
'${contextPath}/main/flow/flowSelect.html'
,
"flowId"
,
"flowName"
);
var
pnode=$(
this
).tree(
'getParent'
,node.target);
$(
"#flowType"
).combobox(
'setValue'
, pnode.id);
$(
"#node_id"
).val(node.id);
$(
"#node_text"
).val(node.text);
console.debug(node.id);
console.debug(node.text);
}
})
|
HTML构建:
1
2
3
|
<
ul
id
=
"tt1"
>
</
ul
>
|
启动TOMCAT预览就可以看到一个树形图的效果了!
关注公众号,分享干货,讨论技术
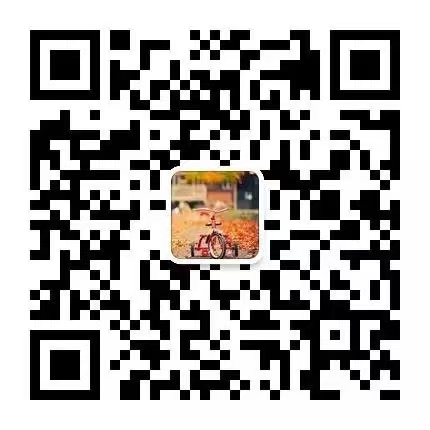