http://www.squidoo.com/applescript
How to Write AppleScript Code without any Prior Knowledge

AppleScript, with tutorial emphasis to benefit those new to scripting. Free example scripts of general utility. This site is dedicated to those who want to learn about the machanics of the AppleScript language.
If you are a long-term Mac-Fan, you may be interested in my site on HyperCard and AppleScript and the Classic (OS9) environment.
Note that I approach things as a step-by-step progression, therefore, for the more basic scripts, please scroll to the posts toward the bottom of the page. For an explanation of some common terms with examples, check out: AppleScript Explained
For more advanced scripts: Advanced AppleScript Code or More Applescript Code.
For some basic JavaScript, check out: How to Write JavaScriptError Handling for Dates

try
set AppleScript's text item delimiters to ","
set testDate to text returned of (display dialog "Enter a date:" default answer "Tuesday, October 10, 2012" buttons "Test" default button "Test")
set dateCharCount to (count characters of testDate)
set commaCount to 0
repeat with x from 1 to dateCharCount
if character x of testDate = "," then set commaCount to commaCount + 1
end repeat
set testWeekday to (text item 1 of testDate)
set validWeekdays to {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"}
set validMonths to {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"}
set testMonth to (word 1 of text item 2 of testDate)
set testDay to (word 2 of text item 2 of testDate)
set testYear to (word 1 of text item 3 of testDate)
set compareDate to date string of (date testDate)
if testDate ≠ compareDate then
display alert "Invalid Date" message "The date, " & testDate & ", which was entered, is invalid ! The correct date is: " & compareDate buttons "Abort" default button "Abort" as warning
else
display alert "Valid Date" message "The date " & testDate & " is valid." buttons "OK" default button "OK"as informational
end if
on error theError number theNumber
if theNumber = -30720 then
if testWeekday is not in validWeekdays then
display alert "Error number " & theNumber & ": Invalid Weekday" message "The weekday, " &testWeekday & ", entered for this date is invalid !" buttons "Abort" default button "Abort" as warning
else if testMonth is not in validMonths then
display alert "Error number " & theNumber & ": Invalid Month" message "The month " & testMonth & " entered for this date is invalid !" buttons "Abort" default button "Abort" as warning
else if not (testDay is number) then
display alert "Error number " & theNumber & ": Invalid Day" message "The day " & testDay & " entered for this date is invalid !" buttons "Abort" default button "Abort" as warning
else if not (testYear is number) then
display alert "Error number " & theNumber & ": Invalid Year" message "The year " & testYear & " entered for this date is invalid !" buttons "Abort" default button "Abort" as warning
end if
else if theNumber = -1728 then
display alert "Error number " & theNumber & ": Invalid Date Format" message "Commas have been omitted for the date " & testDate & " !" buttons "Abort" default button "Abort" as warning
end if
end try
Most of this script is easy to follow, but just a few script lines to take note of when testing for validity:
1. To test for a general instance of validity, we use:
set testDate to text returned of (display dialog "Enter a date:" default answer"Tuesday, October 10, 2012" buttons "Test" default button "Test") -->Here is where I have placed an erroneous date; October 10, 2012 which actually falls on a Wednesday
set compareDate to date string of (date testDate)
if testDate ≠ compareDate then
We save the entered date in the variable testDate because, if we don't, AppleScript will coerce the entered text to a valid date (if it is invalid) and therefore no comparison can be made. With the variable testDate already set, we can set a second variable compareDatethat can be coerced to a valid date, if necessary, so that we can compare the values of the two variables.
If an invalid weekday or month is entered it triggers an appropriate error handler for error number -30720.
3. Finally, just a punctuation test, which uses a count variable dateCharCount to count how many commas are found in the entered text, because there should be 2 in a valid date and, if not, error number -1728 is triggered.
Concatenation or Parsing and Editing of Text Strings
Everything, well almost everything you need to know about text
This is sort of lengthy, but it is pretty easy to follow
First, to extract text from a list. Both of these result in the text "Computer":
set theTextList to {"Apple", "Computer"} as list
last item of theTextList
last text item of theTextList --> both statements yield 'Computer'
And then using the 'reverse' keyword:
set theTextList to {"Apple", "Computer", "Inc"} as list
last text item of theTextList --> yields "Inc"
set theTextList to reverse of theTextList --> yields {"Inc", "Computer", "Apple"}
last text item of theTextList --> yields "Apple"
As you can see, the keyword 'reverse' changes the order of items in a list.
If we want to extract a specific word:
set theTextString to "Apple Computer, Inc"
set theAppleString to word 1 of theTextString
display dialog theAppleString --> yields "Apple"
If we want to extract a specific paragraph:
set theTextString to "Apple Computer, Inc" & return & "Home of Mac OS X"
set theAppleString to paragraph 2 of theTextString
display dialog theAppleString --> yields "Home of Mac OS X"
Extracting by numerical index:
set theTextString to "Apple Computer"
set computerStringStart to offset of "Computer" in theTextString
display dialog computerStringStart --> yields 7: position of first letter in the word 'Computer'
set computerText to (text computerStringStart thru -1 of theTextString)
display dialog computerText --> yields 'Computer'-1 is the index for the last letter of the text string, no matter how long the string is; a lot easier than having to determine how long the string is every time!
The same as saying:
set computerText to (text 7 thru -1 of theTextString)
Of course, if we wanted to, we could say:
'set computerText to (text 7 thru 14 of theTextString)' but why?
set theTextString to "Apple Computer"
set theAppleString to text 7 thru -2 of theTextString
display dialog theAppleString --> yields 'Compute'-2 gets the numeric index of the letter in 'Computer' that is 2 from the end.
set theTextString to "Apple Computer, Inc"
set theAppleString to text 1 thru -1 of theTextString
display dialog theAppleString --> yields: "Apple Computer, Inc"
set theTextString to "Apple Computer, Inc"
set theAppleString to (words 1 thru -1 of theTextString) as text
display dialog theAppleString --> yields "AppleComputerInc"
set AppleScript's text item delimiters to ","
set theTextString to "Apple Computer, Inc"
set theAppleString to (text items 1 thru -2 of theTextString) as text
display dialog theAppleString --> yields "Apple Computer"
The following would give the same result:
set AppleScript's text item delimiters to ","
set theTextString to "Apple Computer, Inc"
set theAppleString to (text item 1 of theTextString) as text
display dialog theAppleString
Why? Because with 'set AppleScript's text item delimiters to ","' , what is found before a comma is a text item and what is after a comma is another text item - this can be very useful.
Look at this:
set AppleScript's text item delimiters to " "
set theTextString to "Apple Computer, Inc"
set theAppleString to (text item 1 of theTextString) as text
display dialog theAppleString --> this yields "Apple", because the space determines the value of text items.
set AppleScript's text item delimiters to " "
set theTextString to "Apple Computer, Inc"
set theAppleString to (text item 2 of theTextString) as text
display dialog theAppleString --> this yields "Computer,", because the space determines the value of text items.
This illustrates how to concatenate some text to display a dialog:
set AppleScript's text item delimiters to " "
set theTextString to "Apple Computer, Inc"
set theAppleString to (text item 1 of theTextString) as text
--> the result is "Apple", because the space determines the value of text items.
set theComputerString to (text 1 thru -2 of text item 2 of theTextString) as text
--> the result is "Computer", because the space determines the value of text items and 'text 1 thru -2' eliminates the comma.
Now we can put it all together:
set dialogString to "Too bad all " & theComputerString & "'s aren't " & theAppleString & "s!"
display dialog dialogString with icon note buttons {"No Doubt"} default button {"No Doubt"} giving up after 5
Contact me if you have any questions or comments at: hyperscripter@gmail.com
Great Deals and Steals for AppleScripters
AppleScript Reference Manuals from Amazon
Using Say and Listen Commands with iTunes
In this article, we look at the say and listen commands. It is a natural transition from the previous iTunes script.

Before you try to run this script, go to the Speech Preferences and click on the Speech Recognition tab and click speech on. Also, if you have not used speech recognition before, click on the 'Calibrate...' button. It adjusts speech recognition to your personal voice to optimize its responsiveness. To refresh your memory, here is the script from the previous post, which we will use (in part) in this post. The key statements used in the script to follow are in bold text:
tell application "iTunes"
activate
set visible of front window to true
set view of front window to playlist "Hall & Oates"
copy (get view of front window) to thePlaylist
set soundValue to (choose from list {"25", "50", "75", "100"} with prompt "Select volume level:" OK button name "Play" cancel button name "Abort")
set soundValue to soundValue as integer
set sound volume to soundValue
play thePlaylist
end tell
The new script:
tell application "iTunes"
activate
set visible of front window to true
set myMusic to (name of every playlist)
end tell
set userResponse to voiceQuery("Which playlist would you like to hear?", myMusic, 30)
if userResponse is false then error number -128
say "Playing " & userResponse & " please wait"
tell application "iTunes"
activate
set thePlaylist to userResponse
set view of front window to playlist thePlaylist
copy (get view of front window) to thePlaylist
play thePlaylist
end tell
on voiceQuery(userPrompt, theseItems, timeoutValue)
set cancelCmds to {"Cancel"}
set matchItems to cancelCmds & theseItems
try
tell application "SpeechRecognitionServer"
set userResponse to listen for matchItems with prompt userPrompt giving up aftertimeoutValue displaying theseItems
end tell
if userResponse is in cancelCmds then
error "user cancelled"
end if
return userResponse
on error
display dialog "The music did not play!"
return false
end try
end voiceQuery
The statement '
set myMusic to (name of every playlist)' gets all of the playlists on your hard drive and puts them into the variable myMusic.
The statement 'voiceQuery("Which playlist would you like to hear?", myMusic, 30)' calls the function 'on voiceQuery(userPrompt, theseItems, timeoutValue)' and places the values into the corresponding variable parameters.
voiceQuery(...) returns a value in the variable userResponse and, if it is not false, plays the desired playlist.
If you have questions or would like to suggest a post on another AppleScript issue, please go toAppleScript Guestbook or if you like this site, click on the twitter icon at the top or bottom of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
Deals from Amazon
Check out these Deals on iPads, iPhones and iPods
AppleScript for iTunes Playlists
A Simple AppleScript for iTunes

Chooses a pre-determined playlist from your iTunes library, then prompts for the volume level. After all of this is done, it plays the selected playlist at the specified volume level:
tell application "iTunes"
activate
set visible of front window to true
set view of front window to playlist "Hall & Oates"
copy (get view of front window) to thePlaylist
set soundValue to (choose from list {"25", "50", "75", "100"} with prompt "Select volume level:" OK button name "Play" cancel button name "Abort")
set soundValue to soundValue as integer
set sound volume to soundValue
play thePlaylist
end tell
The line copy (get view of front window) to thePlaylist assigns, in this case, "Hall & Oates" to the variable thePlaylist (the name assigned must be the actual name of an existing playlist). The second important thing to note here: set soundValue to soundValue as integer . This is required because the list dialog returns a text value, so we must coerce the value to an integer.
There is one problem with this script. If we click on the 'Abort' button the playlist is still played. This problem can be handled with a conditional which will 'trap' for this such as: if soundValue is not "false" then play thePlaylist.
An enhancement of the above script:
tell application "iTunes"
activate
set visible of front window to true
set thePlaylist to (choose from list {"Hall & Oates", "Aja", "Elton John", "ELO"} with prompt "Select a playlist:" OK button name "Choose" cancel button name "Abort") as text
if thePlaylist is not "false" then
set view of front window to playlist thePlaylist
copy (get view of front window) to thePlaylist
set soundValue to (choose from list {0, 25, 50, 75, 100} with prompt "Select volume level (0 for mute):"OK button name "Play" cancel button name "Abort")
if soundValue is not "false" then
set sound volume to soundValue
play thePlaylist
end if
end if
end tell
In this second script we use a choose list command to get the playlist we will use. Also in this script, we assign names to the button choices: OK button name "Play" cancel button name "Abort"
Finally, we use 0 for when we want to make the sound mute: {0, 25, 50, 75, 100} and since the number list items are not enclosed in quotes, AppleScript does not need to coerce the chosen value to integer.
If you wanted to get all of your playlists to appear in a choose from list dialog it would go something like this:
set thePlaylists to (name of every playlist)
set thePlaylist to (choose from list thePlaylists with prompt "Select a playlist:" OK button name "Choose"cancel button name "Abort") as text
If you have further questions on scripting with iTunes or would like to suggest a post on another AppleScript issue, please go to AppleScript Guestbook. For another iTunes script, go to: Using Say and Listen Commands with iTunes
Bookmark Free AppleScript Code Examples
Choosing a Color from the Color Picker
set colorPref to choose color default color {31270, 38327, 65535}
If the user clicks on the "OK" button without choosing a color, the RGB value {31270,38327,65535} is assigned to the variable colorPref, otherwise its value is set to whatever RGB value results from where the user clicked in the color wheel.
We can then use that value in a script such as this which changes the background color of the frontmost finder window:
set colorPref to choose color default color {31270, 38327, 65535}
tell application "Finder"
activate
tell the icon view options of the front Finder window
set the background color to colorPref
end tell
set the current view of the front Finder window to icon view
end tell
This changes the background color for the icon view only. All of the other views remain unchanged.
Contact me with your questions or suggestions at: hyperscripter@gmail.com or visit AppleScript Guestbook.
If you are interested in similar subject matter to this, be sure to scroll up and down this area of the page for other examples using 'choose'
The Simpsons - Steve Mobs
Mapple Computer ? Steve Mobs ?
Essential AppleScript Reference
This Book is Quite a Find
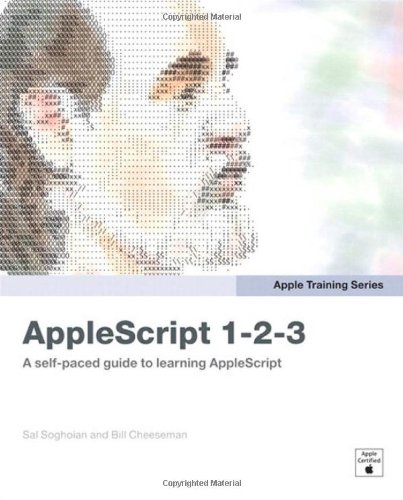
Apple Training Series: AppleScript 1-2-3
This a highly autoritative guide for anyone who is serious about learning AppleScript. It is presented in logical order and in clear concise language, easily understood by the novice as well as the more accomplished. You will want to refer back to this manual for years to come, because of its straight forward presentation and the wealth of example scripts contained therein.
"I give this reference a five-star rating"
iPads, iPods and iPhones
The Essentials from Amazon, Highly Recommended...
Choose Application
The 'choose application' command is very similar in syntax to the 'choose file' command.
set theApp to choose application with title "Installed Applications:" with prompt "Select an application:"
With a result in the form: application "Adobe ImageReady 7.0". The application is automatically opened.
Both of the parameters here, you should recognize.
Here is an example of how the 'choose file' and 'choose application' commands can be used together to open a text file:
set theFile to choose file of type "TEXT" with prompt "Locate a text document to open"
set theApp to choose application with title "Installed Applications:" with prompt "Select an application to open '" & theFile & "':" as alias
tell application "Finder"
open theFile using theApp
end tell
This is all pretty straightforward, so if you carefully look over the syntax of this, I don't expect you will have any difficulty with this.
If you have questions or would like to suggest a post on another AppleScript issue, please visit myGuestbook or if you like this site, click on the twitter icon at the top or bottom of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
Enhanced Choose from List (Tiger and Leopard)
set theName to (choose from list {"John", "Joe", "Bill"} with prompt"Choose a name:")
if theName is false then
display dialog "You clicked cancel to exit." with icon stop buttons {"Exit"} default button {"Exit"}
else
set theName to (item 1 of theName)
display dialog theName with icon note buttons {"Info"} default button {"Info"}
end if
Since there are a number of enhancements to cover here, I will start by presenting a script which uses all of them (they are highlighted), and then explain their significance:
set theName to (choose from list {"John", "Joe", "Bill"} with prompt "Choose a name:" with title "Database Names" default items {"Joe"} OK button name "Choose" cancel button name "Abort" with multiple selections allowed)
if theName is false then
display dialog "You clicked cancel to exit." with icon stop buttons {"Exit"} default button {"Exit"}
else
set theName to (item 1 of theName)
display dialog theName with icon note buttons {"Info"} default button {"Info"}
end if
with title "Database Names": sets the text in the titlebar to "Database Names"
default items {"Joe"}: The dialog selection 'Joe' is selected when the dialog opens.
with multiple selections allowed: If you click on more than 1 item while holding down the shift key a comma-delimited list of the items is returned as the result.
OK button name "Choose": The OK button is renamed "Choose" and behaves as the "OK" button would.
cancel button name "Abort": The cancel button is renamed "Abort" and behaves as the "Cancel" button would.
The 'choose application' command will be covered in the next post. It is somewhat similar to the 'choose file' dialog.
Please see my Guestbook and leave a comment or suggestion on this or any other AppleScript topic.
The Mactini - Smallest Mac in the World
Humor from BBC Two
AppleScript Guestbook
Give me your opinion, topics you'd like covered, or whatever else you want to say about AppleScript...
Choose File Name Command
The 'choose file name' command is similar to the 'choose file' command
set theFile to choose file name with prompt "Set file name and location:"
With a result such as: file "Macintosh HD:Users:administrator:Desktop:Untitled"
Choose file name dialogs have 3 optional parameters: 'with prompt' , 'default name' and 'default location'.
Here with the optional parameters:
set theFile to choose file name with prompt "Set file name and location:" default name "Document 1" default location (path to documents folder)
It is pretty simple to see that the last two parameters determine the name of the yet to be created document and its location.
Finally, a TextEdit example that actually creates a document:
set fileReference to choose file name with prompt ("Create new document:") default name "My Text Document" default location (path to desktop)
set newDocText to text returned of (display dialog "Enter initial text for document '" & fileReference & "':"default answer "" with icon note buttons "OK" default button "OK")
open for access fileReference
close access fileReference
tell application "TextEdit"
activate
open fileReference
set text of document 1 to newDocText
end tell
Where fileReference is the reference for the new document and newDocText is the initial text for the new document.
These lines create the new document and then close it:
open for access fileReference
close access fileReference
TextEdit reopens the document and inserts the text stored in the variable newDocText. TextEdit refers to the document as document 1 because it is the front document.
In the next post, I will backtrack a little bit to show you the enhanced parameters for 'choose from list' which are available in Tiger and Leopard.
If you have questions or would like to suggest a post on another AppleScript issue, please go to myAppleScript Guestbook or if you like this site, click on the twitter icon at the left of the page.
Choose File Dialogs
Getting a reference to a specific document for further script execution.

In this post, we continue with the 'choose file'command. Like the 'choose folder' command, it returns a reference pointer to the actual file that it represents. In this case, it returns an alias reference to a document such as:
set theFile to choose file with prompt "Select a file to open:"
With the following result: alias "Macintosh HD:Users:administrator:Documents:My Document.cwk"
Choose file dialogs have 3 of the same 4 optional parameters, which function identically to the ones used with choose folder dialogs (there is no showing package contents parameter): default location, with (or without) invisibles and with multiple selections allowed.
One additional parameter, not available with the choose folder command is the 'of type' parameter. This is used when you want to specify what types of files to show:
set theFile to choose file of type {"public.jpg", "public.gif"} with prompt "Select a file to open:"
If you like this site, leave a comment at the AppleScript Guestbook or click on the twitter icon at the left of the page
Google on Technology and Apple
News on Tech Issues
Choose Folder Dialogs
First, a simple 'choose folder' dialog:
set theFolder to choose folder with prompt "Select a folder:"
Which brings up the following dialog with the result: alias "HyperMac HD:Users:administrator:Documents:"

Where 'prompt' is the text string that appears at the top of the dialog window. When the user selects a folder the alias to that folder is placed in the variable 'thefolder'
There are 4 optional parameters: default location, with (or without) invisibles, with multiple selections allowed and showing package contents. Together they look like this:
set theFolder to choose folder with prompt ("Select a folder:") default location (path to documents folder)with invisibles, multiple selections allowed and showing package contents
Although you aren't likely to use all of the possible parameters, I will explain each one here:
default location: The dialog opens with the specified path shown
with invisibles: This shows files that aren't ordinarily visible. Most of you will not need to use this parameter, it can be dangerous to tinker with these files, but if you have a reason...
multiple selections allowed: This allows you to choose more than one folder; each folder alias is separated by a comma in the form: {alias 1,alias 2.....}
showing package contents: This is also not ordinarily visible to the user; it displays bundle contents of application packages.
The next post will cover the 'choose file' command, which allows you to select a specific document and get its alias for further script execution. It has parameters that are very similar to the 'choose folder' command, so it should be very easy to pick up how to use it.
If you have questions or would like to suggest a post on another AppleScript issue, visit my AppleScript Guestbook or if you like this site, please 'star rate' me at the top of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
Discerning Between When to Use Set and When to Use Copy
Sometimes set and copy can be used interchangeably, sometimes not!
Even if you have been using AppleScript for a while, as I have, you may still be unclear as to the difference between using set and using copy. In fact you may, as I thought for the longest time, think that they are synonyms. This is in many cases true, such as in statements like:
set clientName to "Joe Gonzalez"
copy "Joe Gonzalez" to clientName
In both cases, the variable clientName is set to the value of "Joe Gonzalez".
If we try to use these interchangeably in the following statements, however, we get very different results. To illustrate this, have a look at these two scripts, which, on the surface would appear to have the same result:
set todaysDate to current date
set tomorrowsDate to todaysDate
set day of tomorrowsDate to (day of todaysDate) + 1
return date string of todaysDate
The previous statement, it would seem, would give us the current date: say 'Tuesday, June 9, 2009'. Unexpectedly, the value of the variable 'todaysDate' is now 'Wednesday, June 10, 2009' - not what we want. The reason for this anomaly is that when we use set to 'set' the value of a second variable, any changes to the value of the second are also changed in the former. This is because 'set' makes the second variable a clone of the original.
If we want to use the original variable as an initial value for the second without effecting the value of the original then we must use the keyword 'copy':
set todaysDate to current date
copy todaysDate to tomorrowsDate
set day of tomorrowsDate to (day of todaysDate) + 1
return date string of todaysDate
In the second case with 'copy', todaysdate retains its original value; using copy instead of set makes the new variable tomorrowsDate an independent variable.
This principle holds true for lists, records and script objects as well. In other words, the set keyword is a reference to the original item which is why, with ordinary text strings they can be used interchangeably.
This is one of those things that you will encounter with AppleScript, where you can get the correct result every time you run a script and think that it will always work, and then you try to use it with another data type and get a big surprise.
Questions or comments are always welcome at my AppleScript Guestbook or contact me on twitter at:http://twitter.com/hyperscripter.
Search Amazon for Stuff from Apple
Order Folder Items by Creation Date
List items of a specified folder numerically by date of creation

tell application "Finder"
set itemGroup to sort (get every document file of the front Finder window) by creation date
repeat with x from 1 to count of itemGroup
set groupItem to item x of itemGroup
set the name of groupItem to ((x as string) & "-" & (the name of groupItem))
end repeat
end tell
First note that in statements such as the second line above, what is enclosed in parentheses is executed first (a list of the documents contained in the specified folder). Next that list is sorted by creation date and the value is placed in the variable itemGroup.
The repeat block takes that sorted list and places a number and a hyphen before each item name:
'((x as string) & "-" & (the name of groupItem))'
In place of creation date, you could also use 'name', 'size' or 'kind' as your sort criteria.
If after running this script, you decide that you want to restore things back to the way they were, this script will do it (BE SURE THAT YOU HAVE THE CORRECT WINDOW AS THE FRONT FINDER WINDOW):
tell application "Finder"
set itemGroup to (every document file of the front Finder window)
repeat with x from 1 to count of itemGroup
set groupItem to item x of itemGroup
set nameItem to the name of groupItem
set the hyphenOffset to (the offset of "-" in nameItem) + 1
set the originalName to text from the hyphenOffset to -1 of nameItem
set the name of groupItem to the originalName
end repeat
end tell
If you like this site, click on the twitter icon or squidlike me at the left of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
RSS: HTML Example Code
Exploring the World of HTML and CSS Scripting
-
HTML to Create Document Menus
- Sometimes you want the user to choose from a list of possible items (web pages, operating systems, e... Adding Some Pizazz to Your Text
- Making a Statement with Initial Text of Document Image HTML
- This Displays an Image on Your Page. As with a regular link it is a reference to a location, but it...
The Finder's Standard Suite
These are the core commands that the Finder and every scriptable application should be able to handle
While the last two are pretty simple to implement, the open command requires some explantion. First, the basic open syntax:
tell application "Finder" to open home
This is easy to understand, it simply tells the Finder to open home of your HD.
If you want to open a specific document, you would use a form such as:
tell application "Finder" to open document file "My Document.cwk" of folder "Documents" of home
end tell
Here, 'My Document.cwk' is replaced with your document residing in your 'Documents' folder (or which ever folder you desire).
To get the full path of a document, open the document and hold down the command key while clicking on the window's title bar. The result is something like:
(alias "Macintosh HD:Users:administrator:Documents:My Document.cwk")
Each level of the path is separated by a colon. The script would look like this:
tell application "Finder" to open document file (alias "Macintosh HD:Users:administrator:Documents:My Document.cwk")
end tell
The basic quit and activate (bring app to front) commands take no parameters:
tell application "Finder" to quit
tell application "Finder" to activate
tell application "FileMaker Pro" to activate
tell application "AppleWorks 6" to activate
Remember that I covered the print command in my previous post.
If you have questions or would like to suggest a post on another AppleScript issue, please go to my AppleScript Guestbook or if you like this site, please 'star rate' me at the top of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
A Fun AppleScript for iTunes
tell application "iTunes"
activate
set visible of front window to false
set view of front window to playlist "Hall & Oates"
copy (get view of front window) to thePlaylist
set soundValue to text returned of (display dialog "Enter sound volume (a number between 0 and 100-full volume):" default answer "25" buttons {"Cancel", "Play"} default button {"Play"})
set soundValue to soundValue as integer
set sound volume to soundValue
play playlist
end tell
Basic Printing Scripts
A few simple AppleScripts to automate your printing routine
tell application "TextEdit"
print the front document with properties {copies:2,collating:true, starting page:1, ending page:1, pages across:1, pages down:1, error handling:standard} withoutprint dialog
end tell
Most of what appears in 'with properties {....}' above should be fairly obvious. If you want to specify the printer, you could follow the last parameter in the list with a comma and 'target printer:' and the actual name of the desired printer enclosed in quotes.
If you want to use different printers in certain cases, replace 'without print dialog'with 'print dialog'
This second script, so that you aren't required to specify the application file targeted by the print command:
tell application "Printer Setup Utility"
set the current printer to printer "Deskjet D2400 series"
(alias "Macintosh HD:Users:administrator:Documents:My Document.cwk")
end tell
Replace 'Deskjet D2400 series' with the name of your printer and replace '(alias "Macintosh HD:Users:administrator:Documents:My Document.cwk")' with the path to the document that you wish to print (Don't forget to precede the path name by 'alias' - this is important).
The print command is part of the 'Standard Suite' of the Finder's Dictionary, and so, in my next post, We will look at the remaining commands that make up the Standard Suite (there are 3). If you have questions on printing or would like to suggest a post on an AppleScript issue you need help with, Please visit myAppleScript Guestbook
Display Dialog with Password
A password dialog where text entered appears as bullets
Seriously, though, the main purpose of this type of dialog is as a security measure to restrict access to critical files.
set thePass to "pass90805"
Here, depending upon the application, you could have 'thePass' set to the value stored in a global field, In FileMaker it would be a statement something like 'set thePass to data of cell "passwordStorage"'
considering case
repeat with x from 1 to 3
display dialog ("Enter the password for access to this file:") default answer "" with icon stop with hidden answer
if the text returned of the result is thePass then
exit repeat
end if
if x is 3 then return "You have attempted to access this file too many times. Your access is denied. Please try again."
end repeat
end considering
The considering case block tells AppleScript to require that the text entered match the particular case of the pre-determined password (upper or lower).
By the way, the part that gives the bullets instead of the actual text entered is 'with hidden answer'
The repeat block allows the user 3 attempts at entering the password for access and after that, terminates the script.
If you have questions or would like to suggest a post on another AppleScript issue, visit AppleScript Guestbook or if you like this site, please 'star rate' me at the top of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
'Display Alert' Dialogs
Dialogs that display additional information to user

try
display dialog ("Enter a number") default answer ("") buttons {"OK"}default button "OK"
set userEntry to text returned of result
return userEntry as number
on error
set alertString to "The text entered is not a number"
set messageString to ("" & userEntry & "is not a number. ") & "Run the script again and use only number keys."
display alert alertString message messageString buttons {"OK"} default button "OK" giving up after 20
end try
This is fairly straightforward, below the resulting dialog when the user happens to enter 'abc' (a non-numerical value):

Contact me if you have any questions or comments at: hyperscripter@gmail.com orhttp://twitter.com/hyperscripter or to subscribe, click the By Email link at the top of the page
If you like this post, check out this one: Invoking actions with dialogs
Creating a New Document with Microsoft Word 2004
Simple AppleScript to create a new text document with default text

tell application "Microsoft Word"
activate
try
set newDoc to make new document
set name of font object of text object of newDoc to "Arial"
--Here you could have a 'text returned of' dialog to set the text font for the new document to a font of your choice. Of course, the font would have to exist on the target hard drive.
set documentName to text returned of (display dialog "Enter name for new document:" default answer"Untitled Document" with icon note buttons {"Cancel", "Save"} default button {"Save"})
save as newDoc file name documentName
--By default, the document is created in the currently active folder.
set initialText to text returned of (display dialog "Enter initial text for new document:" default answer "" with icon note buttons {"Cancel", "Insert"} default button {"Insert"})
if initialText ≠ "" then
set documentRange to create range active document start 0 end 0
insert text initialText at documentRange
end if
--If initialText has no value, then a text document is opened with no initial text.
on error
if documentName = "" then
display dialog "Document creation aborted!" with icon stop buttons {"OK"} default button {"OK"}
end if
end try
end tell
The else part of the conditional above instructs AppleScript to ignore the fact that no initial text was requested for the new document and creates the document anyway. The value of 0 for start and end tells Word that it is a document that does not yet have any text or if there is existing text, to place it before the existing text .
If you have questions or would like to suggest a post on another AppleScript issue, please visit my AppleScript Guestbook or if you like this site, please 'star rate' me at the top of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
Trapping for List Dialog Errors
Intercepting the Dreaded 'Cancel' error (Error Number -128)
set x to (choose from list {"Joe", "Amy", "Bill"} with prompt "Choose a record:")
if x is false then
else
set targetItem to (x as text)
show (every record whose cell "Name" contains x)
end if
When the user clicks on 'Cancel', the variable x is assigned the boolean value false. So all you have to do is set up a conditional clause to deal with that (notice it does nothing at all) and to perform the usual statements otherwise.
If you have questions or would like to suggest a post on another AppleScript issue, go to my Guestbook or if you like this site, please 'star rate' me at the top of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
FileMaker Pro AppleScript to Backup Files
Backup a specific folder of FileMaker documents, replacing previous backup files
Most of what appears here builds upon the information presented in previous posts to this point.
tell application "Finder"
try
set todaysDate to (current date)
set bkpYear to (year of todaysDate)
set monthlyBkp to ("Monthly Reports " & bkpYear) as text
if not (folder "Database Backups" exists) then
set targetFolder to (make new folder at folder "Desktop" of startup disk with properties {name:"Database Backups"})
end if
set replaceExecute to button returned to (display dialog "Are you sure you want to replace current backup contents with the selected items?" with icon caution buttons {"Cancel", "OK"} default button {"OK"} giving up after 60) --give user the chance to exit in case there is already a folder 'database backups' containing items that the user does not want to replace
if replaceExecute = "OK" then
select {file "Home Database" of folder "Database Programs)", ¬
file "Appointments" of folder "Database Programs", ¬
file monthlyBkp of folder "Database Programs", ¬
file "Accounts Receivable" of folder "Database Programs", ¬
file "Calendar" of folder "Database Programs"}
duplicate the selection to folder "Database Backups" with replacing --if we proceed, 'with replacing' replaces the contents of the folder "Database Backups"
end if
on error
display dialog "Backup cancelled!" with icon stop buttons {"OK"} default button {"OK"} giving up after 3
end try
end tell
tell application "FileMaker Pro" to activate
The next subject I will deal with addresses scripting for text formatting with AppleWorks 6 specifically, it is a simple but powerful script that can get you started with what you may want to do with text documents in general. Even if you use other text programs, you may find with a little knowledge of applescripting of the specific app that you use, this can be adapted to work with your document scripts.
If you have questions or would like to suggest a post on another AppleScript issue, visit my AppleScript Guestbook If you would like to see more FileMaker-specific AppleScripts, be sure to visit: AppleScript-and-FileMaker.
More on List Dialogs
Continuing with list dialogs with an example that goes into more detail

This post will deal with what I consider a very concrete use of the 'choose from list' dialog. After reading this (and perhaps trying it out in the Script Editor) you will probably be totally confused (just kidding!!) It gets a little complicated, but it is basically a cut-and-paste script.
If you pay close attention to my comments, I'm sure you'll understand most of what is going on and, after all, it's understanding how a script works that allows you adapt it to other applications that you may have in mind.
So anyway, here it is:
set AppleScript's text item delimiters to ","
This first line is needed, because it sets things up for extracting the info we need.
set theLongDate to (current date)
set theLongDate to (date string of theLongDate)
The next two lines set us up for calculating the numerical data for month and day.
set currentMonth to (word 1 of text item 2 of theLongDate)
set currentDay to (word 2 of text item 2 of theLongDate)
This gets the numerical value for the month.
set currentYear to (word 1 of text item 3 of theLongDate)
set monthList to {January, February, March, April, May, June, July, August, September, October, November,December}
repeat with x from 1 to 12
if currentMonth = ((item x of monthList) as string) then
set theRequestNumber to (text -2 thru -1 of ("0" & x))
exit repeat
end if
end repeat
set currentMonth to theRequestNumber
set currentDay to (text -2 thru -1 of ("0" & currentDay))
set theShortDate to (currentMonth & "/" & currentDay & "/" & currentYear) as string
Previous code sets up the default text (current date in the form 'MM/DD/YYYY' - something like '02/03/2009') for dialog below :
set theDefaultDate to text returned of (display dialog "Enter a date in the form MM/DD/YYYY:" default answertheShortDate buttons {"Calc Date"} default button {"Calc Date"} giving up after 20)
This line converts the text supplied by the dialog to class 'date' (the long form of the date, including the time):
set calcLongDate to date theDefaultDate
This line extracts the date portion and leaves the time portion behind:
set calcLongDateTrunc to (date string of calcLongDate)
This converts calcLongDateTrunc back into the form 'text' so that it can be displayed in a dialog:
set calcLongDateTrunc to calcLongDateTrunc as string
display dialog calcLongDateTrunc buttons {"Done"} default button {"Done"} giving up after 15
Yields something like- 'Tuesday, February 3, 2009'
In my next post, I will shift gears and present an AppleScript which I have derived from one of my Filemaker programs, which serves as a convenient file backup program.
If you have questions or would like to suggest a post on another AppleScript issue, please visitAppleScript Guestbook or if you like this site, please 'star rate' me at the top of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
Dialogs for Lists
Making a Choice from a List of Options

Take a look at this example:
set theName to (choose from list {"John", "Joe", "Bill"})
if theName is false then
display dialog "You clicked cancel to exit." with icon stop buttons{"Exit"} default button {"Exit"}
else
set theName to (item 1 of theName)
display dialog theName with icon note buttons {"Info"} default button {"Info"}
end if
First note in the first line that 'choose from list' prompts you to choose from the three names given {"john", "joe", "bill"}. the name chosen is assigned to the variable 'thename'.
if the user clicks cancel from a 'choose from list' dialog such as this, then the variable 'thename' takes on the value false indicating that the user decided not to make a choice of the items listed in the dialog.
If the user makes a choice, say for instance "bill", then 'thename' takes on that value and can be used for further script execution.
Adapting the previous dialog, assuming you had a list of items (whatever criteria relevant to what you want) compiled from a database of your own design, in this case we will call the list 'recentOrders' , a list of customer names who have ordered products or services from you in recent months. Another version of the list dialog that could be used based on these premises:
set recentOrders to recentOrdersArray as list
try
set recentCustomer to (choose from list recentOrders)
if recentCustomer ≠ false then
set recentCustomer to (item 1 of recentCustomer)
set scriptAction to button returned of (display dialog "Get information for last order from '" & recentCustomer& "' ?" with icon note buttons {"Cancel", "Info"} default button {"Info"})
display dialog "Info for last order from: " & recentCustomer
-here you would return to the user the info from your database on 'recentCustomer'
end if
end try
Here, we choose a particular item (customer) from 'recentorders'. next, if we do not cancel execution, the variable 'recentcustomer' is assigned. if we do not cancel the next request dialog, the data on the requested customer can be displayed (based upon info stored in whatever the referenced database).
As list type dialogs deviate from the standard dialogs dealt with up until now, it is important to note that, although computers are supposed to follow some sort of logic, you sometimes have to 'go with the flow' on things that don't seem to follow that logic.
I can't emphasize enough, that the whole process of learning how to applescript builds upon itself. In my coming posts, I will strive to make things easier to grasp by continuing in a step-by-step manner, as I have found it to be effective in learning scripting.
I hope this post has been useful in furthering your knowledge of scripting with AppleScript. As always, if you have any questions or comments or would like to suggest a post on another AppleScript issue, please go to myAppleScript Guestbook or if you like this site, please 'star rate' me at the top of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
Getting Results of Dialogs
Once you get down the basics of writing a simple dialog for interaction with the user, the next step is to be able to use the returned data to its fullest extent to invoke other actions based upon the actions of the user.
If you read through the script below, you will see the kinds of information returned to AppleScript from a dialog and how it can be useful to save that data to variables for further processing. First a dialog that directly assigns the text result to a variable:
set returnedText to text returned of (display dialog "Enter some text:" default answer "" with icon note)
-->"some text"
In this example, the variable: returnedText is set after the dialog has been invoked:
display dialog "Enter some text:" default answer "" with icon note
set returnedText to text returned of the result
-->"some text"
In this final example, all of the result data is stored in a list with a variable assigned for each. Note here that I include the giving up after parameter which returns the 'true or false' of whether or not the user responded to the dialog or 'gave up' within the specified time:
display dialog "Enter some text:" default answer "" with icon note giving up after 15
set {returnedText, returnedButton, gaveupBoolean} to the result as list
-->{"some text", "OK", false}
And here, some of the things you can do with the data derived from this:
returnedText
-->"some text"
returnedButton
-->"OK"
display dialog gaveupBoolean
-->{button returned:"OK"}
if gaveupBoolean = true then display dialog "You gave up dude !" with icon 0
-->{button returned:"OK"}, true is unquoted because it is not a string, but a boolean value
Questions or comments ? Have a topic of interest that you would like to see covered here ? Go to: AppleScript Guestbook
Using AppleScript Dialogs to Invoke Actions
Attaching an action to user interaction dialog

try
display dialog "Your text here" with icon caution buttons {"OK", "Cancel"} default button {"Cancel"} giving up after 30
end try
Taking our original script I will make a few additions:
try
set theAction to button returned of (display dialog "Quit application AppleWorks 6" with icon caution buttons{"OK", "Cancel"} default button {"Cancel"} giving up after 30)
if theAction = "OK" then tell application "AppleWorks 6" to quit
end try
You can see above, that I have set the variable theAction to take on the value of the name of the button chosen (note parentheses). As before, if the user clicks on the 'Cancel' button the script halts without any action being taken. If, however the 'OK' button is chosen, then AppleScript sends the quit message to AppleWorks 6.
Now suppose we wanted a dialog that quits an application, but we want to be able to enter in the name of a specific application ahead of time.
try
set dialogResult to (display dialog "Enter name of application to quit:" default answer "" with iconcaution buttons {"OK", "Cancel"} default button {"Cancel"} giving up after 30)
set appToQuit to (text returned of dialogResult)
if (button returned of dialogResult) = "OK" then tell application appToQuit to quit
end try
There are a few changes to be noted here, but it's really not complicated at all.
First, I have enclosed the dialog part of the script in parentheses and before that I have inserted 'set dialogResult to'. This tells AppleScript to give the variable dialogResult the values that will be derived from executing the dialog part of the script.
The text default answer "", inserted into the dialog section tells AppleScript to give us a blank text field where the name of the app to quit will be entered. The variabledialogResult will contain both the text entered and the name of the button clicked.
Next, the variable appToQuit extracts the text part of dialogResult (the application name)
The next line determines if the 'OK' button was activated and, if so, quits the application supplied by the variable appToQuit, otherwise the script ends with no further action.
I hope you're having a little bit of fun now and seeing just how powerful the AppleScript language can be. If you have any questions or comments, visit my AppleScript Guestbookand leave a comment.
Getting Started with AppleScript Dialogs
A Basic Notification Dialog

Note in the script below, that you could substitute 'note' or 'caution' for 'stop' (in purple) to indicate varying degrees of importance or urgence.
display dialog "Your text here" with icon stop buttons "OK" default button"OK"
Taking the above script a step further, you could include 'giving up after 5 (or 15, 20, 60 etc) so that a dialog will automatically close itself after the indicated interval of time:
display dialog "Your text here" with icon stop buttons {"OK"} default button {"OK"} giving up after 5
You can also have multiple button choices (up to 3). take special note of the formatting required for multiple buttons:
display dialog "Your text here" with icon note buttons {"OK", "Cancel"} default button {"Cancel"} giving up after 30
Note in the above example, if you click on 'cancel' or strike the enter or return keys, it will generate an error message. To avoid this, and to get your dialog to close 'silently', the above must be enclosed within a 'try clause' such as:
try
display dialog "Your text here" with icon caution buttons {"OK", "Cancel"} default button {"Cancel"} giving up after 30
end try
I hope that those of you who are new to appleScripting will find these sample dialog scripts useful as you begin to explore the magic of AppleScript. If you still don't understand how these scripts work, I strongly urge you to cut and paste them into your Script Editor and run them - this should clear up any questions you may still have.
If you have questions, go to: AppleScript Guestbook or if you like this site, please 'star rate' me at the top of the page. For RSS feed, click the RSS icon at the top or bottom of the page.
If you want a more detailed script on dialogs like this with an action attached to it, see:Using AppleScript Dialogs to Invoke Actions above. Please subscriibe to my RSS feed at the top or bottom of the page (look for the icon)
Amazon Digital Cameras
Rate a Digital Camera or Webcam
Logitech Z-2300 THX-Certified 200-Watt 2.1 Speaker System (Silver)
If you love music, youll love the Logitech Z-2300, more...0 points
Sony Cyber-shot DSC-W290 12.1 MP Digital Camera with 5x Optical Zoom and Super Steady Shot Image Stabilization (Black)
The Sony Cyber-shot DSC-W290 camera combines style more...0 points
Olympus Stylus Tough-8000 12 MP Digital Camera with 3.6x Wide Angle Optical Dual Image Stabilized Zoom and 2.7-Inch LCD (Black)
The STYLUS TOUGH-8000 is virtually indestructible. more...0 points
Olympus Stylus Tough-6000 10 MP Waterproof Digital Camera with 3.6x Wide Angle Optical Dual Image Stabilized Zoom and 2.7-Inch LCD (Blue)
For the first time, Dual Image Stabilization will more...0 points