- 当我们在开发中,使用数据库连接的时候,往往是测试的时候连接测试数据库,生产环境用的是生产环境的数据库。
这两个数据库的用户名密码往往是不同的。但是当我们打完包上线app到生产环境中去的时候,发现数据库的用户名密码用的是测试数据库的用户名密码。
这时候就很麻烦了。所以从Spring3开始提供了profile的功能。
就是当环境符合那个profile就激活哪个profile。
这里我们用Dao去演示,里面的成员变量封装了数据库的类型,数据库的用户名和密码
正式开发的时候,这里应该是DataSource
首先我们先写一个dao
package org.huluo.springapiprofile;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class SpringProfileDao {
@Value("#{config.username}")
private String username;
@Value("#{config.password}")
private String password;
@Value("#{config.dbtype}")
private String dbType;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getDbType() {
return dbType;
}
public void setDbType(String dbType) {
this.dbType = dbType;
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
在这里我们先贴上配置文件的代码。配置文件的配置分别是生产环境下的和开发环境下的数据库用户名和密码以及数据库的类型。
dev.properties配置文件 –> 配置的是开发环境下的数据库用户名密码和数据库的类型
username=hello
password=world
dbtype=derby
produce.properties配置文件 —> 自然就是对应的生产环境下的配置文件
username=root
password=root
dbtype=mysql
再进行Spring配置,这里我们选择xml的配置方式
<beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p"
xmlns:util="http://www.springframework.org/schema/util"
xmlns:context="http://www.springframework.org/schema/context" xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:jpa="http://www.springframework.org/schema/data/jpa" xmlns="http://www.springframework.org/schema/beans"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/data/jpa http://www.springframework.org/schema/data/jpa/spring-jpa.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.2.xsd">
<beans profile="development">
<context:component-scan base-package="org.huluo.springapiprofile"/>
<util:properties id="config" location="classpath:springprofileapitest/dev.properties"/>
</beans>
<beans profile="produce">
<context:component-scan
base-package="org.huluo.springapiprofile"/>
<util:properties id="config" location="classpath:springprofileapitest/produce.properties"/>
</beans>
</beans>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
我们可以看到笔者这里配置了两个profile他们的名字分别是:development和produce
现在我们写一个单元测试去测试一下
package org.huluo.springapiprofile
import org.junit.Test
import org.junit.runner.RunWith
import org.springframework.beans.factory.annotation.Autowired
import org.springframework.test.context.ActiveProfiles
import org.springframework.test.context.ContextConfiguration
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = "classpath*:spring/ctx-profiletest.xml")
@ActiveProfiles(value = "produce")
public class SpringProfileDaoTest {
@Autowired
private SpringProfileDao springProfileDao
@Test
public void testName() throws Exception {
System.out.println(springProfileDao.getUsername())
System.out.println(springProfileDao.getPassword())
System.out.println(springProfileDao.getDbType())
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
这里我们激活的是produce的profile
所以打印出来的应该是:
root
root
mysql
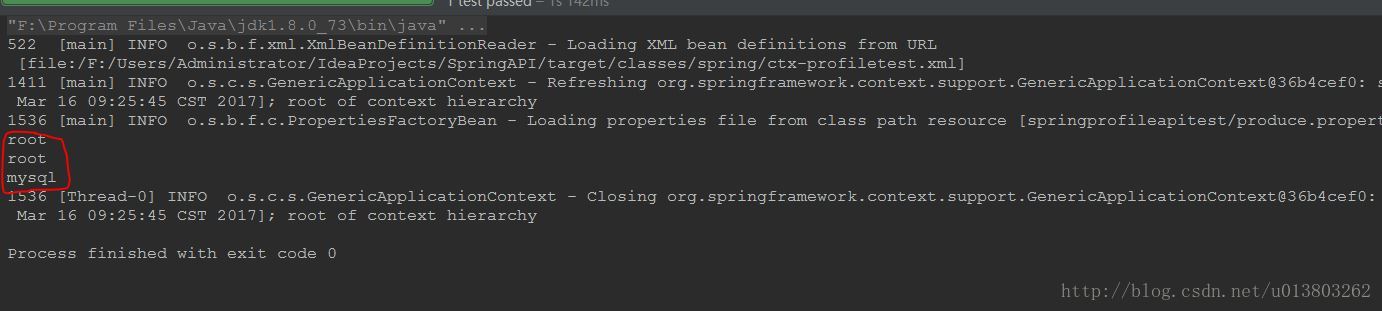
当然还有其他的方式去激活profile,比如说
<context-param>
<param-name>spring.profiles.active</param-name>
<param-value>webenv</param-value>
</context-param>