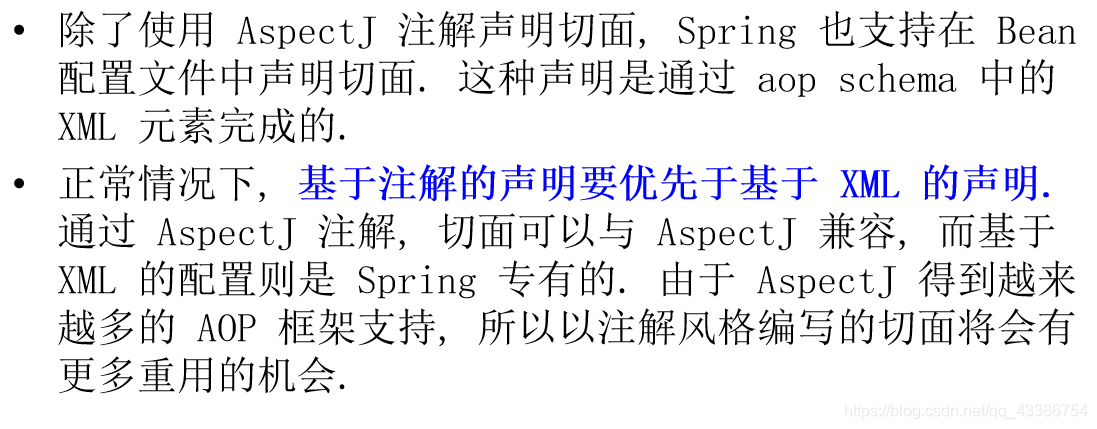
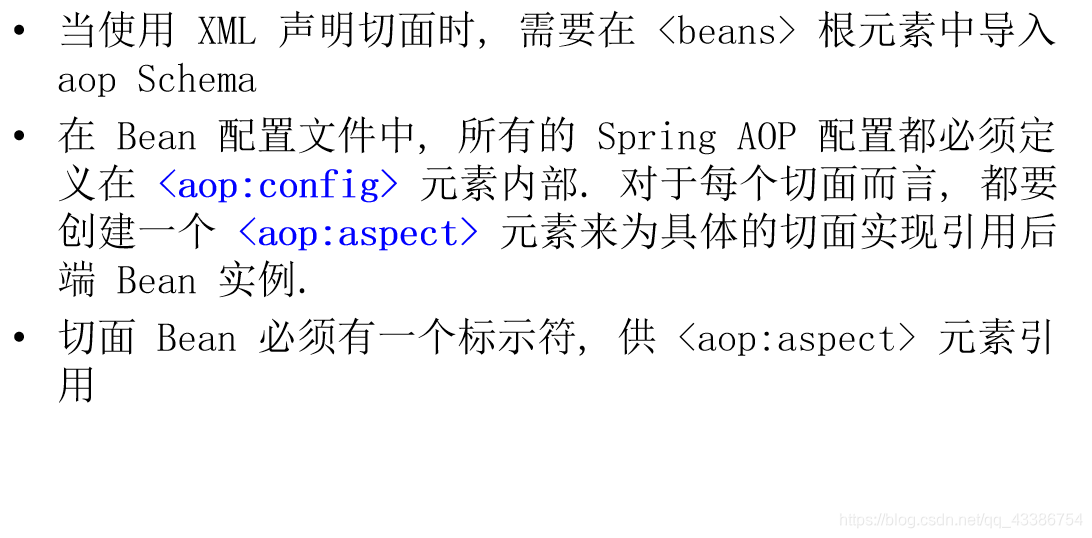
示例
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd">
<bean class="mao.shu.spring.aop.aspectJXMl.IntCalc" id="intCalc"/>
<bean class="mao.shu.spring.aop.aspectJXMl.LogAspect" id="logAspect"/>
<bean class="mao.shu.spring.aop.aspectJXMl.ValidateAspect" id="validateAspect"/>
<aop:config proxy-target-class="true">
<aop:pointcut id="intCalcPoint" expression="execution(* mao.shu.spring.aop.aspectJXMl.IntCalc.*(..))"/>
<aop:aspect ref="logAspect" order="1" >
<aop:after method="afterMethod" pointcut-ref="intCalcPoint"/>
<aop:before method="beforeMethod" pointcut-ref="intCalcPoint"/>
<aop:after-returning method="afterRunningMethod" returning="result" pointcut-ref="intCalcPoint"/>
<aop:after-throwing method="throwingMethod" pointcut-ref="intCalcPoint" throwing="t"/>
<aop:around method="aroundMethod" pointcut-ref="intCalcPoint"/>
</aop:aspect>
<aop:aspect ref="validateAspect" order="0">
<aop:after method="validate" pointcut-ref="intCalcPoint"/>
</aop:aspect>
</aop:config>
</beans>
package mao.shu.spring.aop.aspectJXMl;
import org.junit.Before;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import static org.junit.Assert.*;
public class IntCalcTest {
private ApplicationContext app;
private IntCalc intCalc;
@Before
public void before(){
this.app = new ClassPathXmlApplicationContext("mao/shu/spring/aop/aspectJXMl/aspectJXML.xml");
this.intCalc = this.app.getBean("intCalc",IntCalc.class);
}
@Test
public void plus() throws Exception {
System.out.println(this.intCalc.plus(1,12));
}
@Test
public void subtract() throws Exception {
System.out.println(this.intCalc.subtract(1,12));
}
@Test
public void multiplication() throws Exception {
System.out.println(this.intCalc.division(134,13424));
}
@Test
public void division() throws Exception {
System.out.println(this.intCalc.multiplication(554,78475));
}
}
声明切面
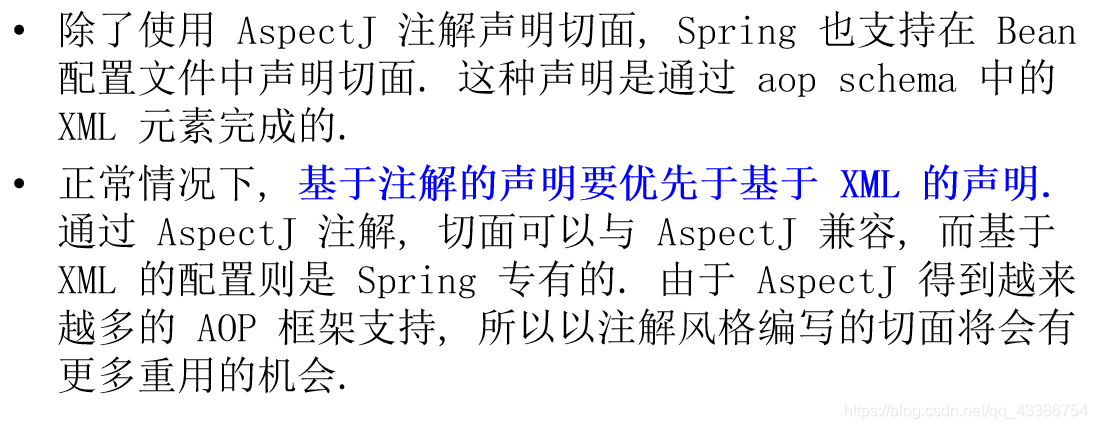
<bean class="mao.shu.spring.aop.aspectJXMl.LogAspect" id="logAspect"/>
<bean class="mao.shu.spring.aop.aspectJXMl.ValidateAspect" id="validateAspect"/>
<aop:config>
<aop:aspect ref="validateAspect" order="0">
<aop:after method="validate" pointcut-ref="intCalcPoint"/>
</aop:aspect>
</aop:config>
声明切入点
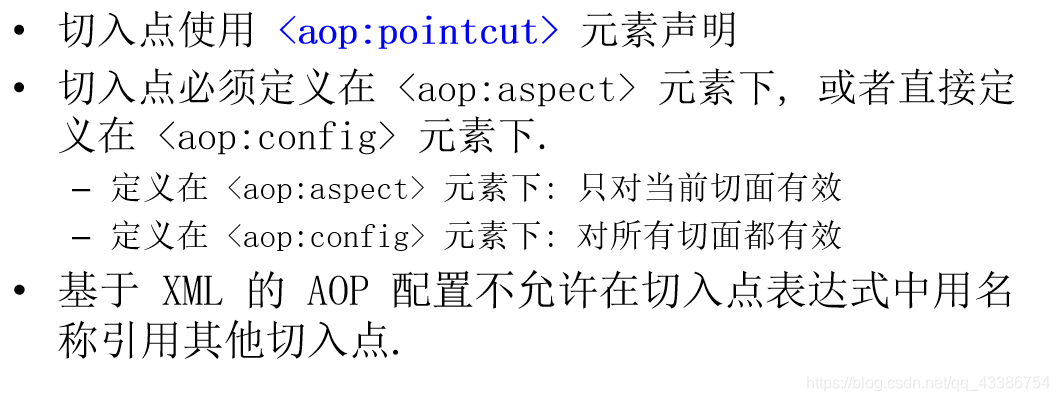
<aop:config proxy-target-class="true">
<aop:pointcut id="intCalcPoint" expression="execution(* mao.shu.spring.aop.aspectJXMl.IntCalc.*(..))"/>
</aop:config>
声明通知
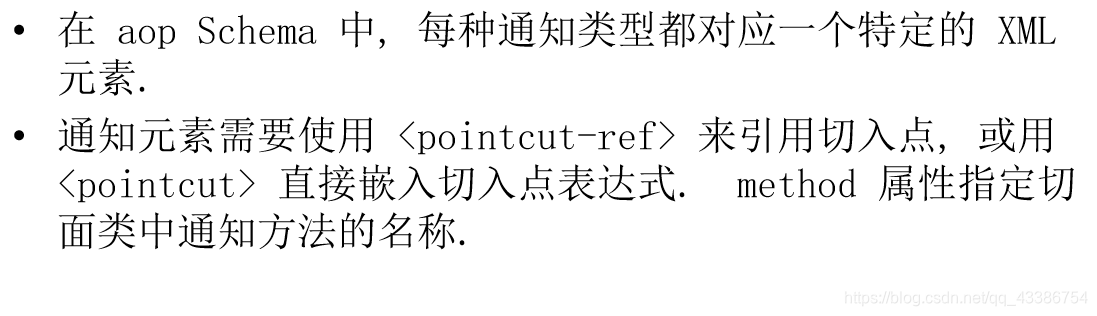
<aop:aspect ref="logAspect" order="1" >
<aop:after method="afterMethod" pointcut-ref="intCalcPoint"/>
<aop:before method="beforeMethod" pointcut-ref="intCalcPoint"/>
<aop:after-returning method="afterRunningMethod" returning="result" pointcut-ref="intCalcPoint"/>
<aop:after-throwing method="throwingMethod" pointcut-ref="intCalcPoint" throwing="t"/>
<aop:around method="aroundMethod" pointcut-ref="intCalcPoint"/>
</aop:aspect>