1. “Cannot Find Symbol”
这是一个非常常见的问题,因为Java中的所有标识符都需要在使用之前进行声明。出现这个错误是因为,在编译代码时,编译器不明白该标识符的含义。
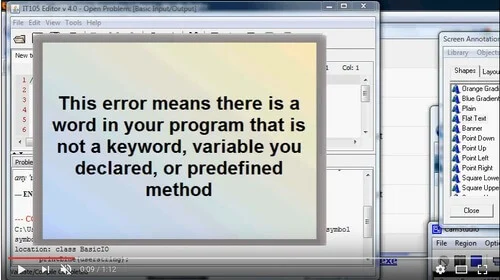 {
xlocation = xxlocation;
ylocation = yylocation;
name = nname;
ccount++;
}
}
public class JavaApplication1 {
public static void main(String[] args) {
robot firstRobot = new Robot(34,51,"yossi");
System.out.println("numebr of robots is now " + Robot.ccount);
}
}
要解决这个问题,可以:
把类和文件命名为相同的名字。
确保两个名称始终保持一致。
3. “Incompatible Types”
“Incompatible Types”是赋值语句尝试对变量与表达式进行类型匹配时发生的逻辑错误。通常,将字符串赋值给一个整数时会产生这个错误,反之亦然。这不是一个Java语法错误。
test.java:78: error: incompatible types
return stringBuilder.toString();
^
required: int
found: String
1 error
当编译器抛出“incompatible types”消息时,确实不太容易解决这个问题:
- 使用类型转换函数。
- 开发人员可能需要修改代码原有的功能。
4. “Invalid Method Declaration; Return Type Required”
这个错误消息的意思是,在方法声明中未显示地声明方法的返回类型。
public class Circle
{
private double radius;
public CircleR(double r)
{
radius = r;
}
public diameter()
{
double d = radius * 2;
return d;
}
}
有这几种情况会触发“invalid method declaration; return type required”错误:
- 忘记声明类型。
- 如果方法没有返回值,那么需要在方法声明中指定“void”作为返回类型。
- 构造函数不需要声明类型。但是,如果构造函数名称中存在错误,那么编译器会把构造函数看成是没有指定类型的方法。
5. “Missing Return Statement”
当一个方法缺少return语句时,会触发“Missing Return Statement”错误消息。有返回值(非void类型)的方法必须要有一条返回某个值的语句,以便在方法之外调用该值。
public String[] OpenFile() throws IOException {
Map<String, Double> map = new HashMap();
FileReader fr = new FileReader("money.txt");
BufferedReader br = new BufferedReader(fr);
try{
while (br.ready()){
String str = br.readLine();
String[] list = str.split(" ");
System.out.println(list);
}
} catch (IOException e){
System.err.println("Error - IOException!");
}
}
编译器抛出“missing return statement”消息有这几个原因:
- 返回语句被错误地省略了。
- 该方法没有返回任何值,但是在方法声明中未声明类型为void。
6.“Reached End of File While Parsing”
这个错误消息通常在程序缺少右大括号(“}”)时触发。有时,在代码的末尾增加右大括号可以快速地修复此错误。
public class mod_MyMod extends BaseMod
public String Version()
{
return "1.2_02";
}
public void AddRecipes(CraftingManager recipes)
{
recipes.addRecipe(new ItemStack(Item.diamond), new Object[] {
"#", Character.valueOf('#'), Block.dirt
});
}
上述代码会产生以下这个错误:
java:11: reached end of file while parsing }
编码工具和适当的代码缩进可以更容易地找到这些不匹配的大括号。
7.“Unreachable Statement”
当一条语句出现在一个它不可能被执行的地方时,会触发“Unreachable statement”错误。通常,是在一个break或return语句之后。
for(;;){
break;
... // unreachable statement
}
int i=1;
if(i==1)
...
else
... // dead code
通常,简单地移动return语句即可修复此错误。
8.“Variable Might Not Have Been Initialized”
在方法中声明的局部变量如果没有初始化,就会发生这种错误。如果在if语句中包含没有初始值的变量时,就会发生这种错误。
int x;
if (condition) {
x = 5;
}
System.out.println(x); // x可能尚未初始化
9.“Missing Return Value”
当返回语句包含不正确的类型时,你会收到“Missing Return Value”消息。例如,查看以下代码:
public class SavingsAcc2 {
private double balance;
private double interest;
public SavingsAcc2() {
balance = 0.0;
interest = 6.17;
}
public SavingsAcc2(double initBalance, double interested) {
balance = initBalance;
interest = interested;
}
public SavingsAcc2 deposit(double amount) {
balance = balance + amount;
return;
}
public SavingsAcc2 withdraw(double amount) {
balance = balance - amount;
return;
}
public SavingsAcc2 addInterest(double interest) {
balance = balance * (interest / 100) + balance;
return;
}
public double getBalance() {
return balance;
}
}
返回以下错误:
SavingsAcc2.java:29: missing return value
return;
^
SavingsAcc2.java:35: missing return value
return;
^
SavingsAcc2.java:41: missing return value
return;
^
3 errors
通常,这个错误的出现是因为有某个返回语句没有返回任何东西。
10.“Cannot Return a Value From Method Whose Result Type Is Void”
当一个void方法尝试返回任何值时,会发生此Java错误,例如在以下代码中:
public static void move()
{
System.out.println("What do you want to do?");
Scanner scan = new Scanner(System.in);
int userMove = scan.nextInt();
return userMove;
}
public static void usersMove(String playerName, int gesture)
{
int userMove = move();
if (userMove == -1)
{
break;
}
```
通常,更改方法的返回类型与返回语句中的类型一致,可以解决这个问题。例如,下面的void可以改为int:
```
public static int move()
{
System.out.println("What do you want to do?");
Scanner scan = new Scanner(System.in);
int userMove = scan.nextInt();
return userMove;
}
11.“Non-Static Method … Cannot Be Referenced From a Static Context”
当Java代码尝试在静态类中调用非静态方法时,会发生此问题。例如,以下代码:
class Sample
{
private int age;
public void setAge(int a)
{
age=a;
}
public int getAge()
{
return age;
}
public static void main(String args[])
{
System.out.println("Age is:"+ getAge());
}
}
会触发这个错误:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot make a static reference to the non-static method getAge() from the type Sample
要在静态方法中调用非静态方法,需要是声明一个要调用的非静态方法的类的实例。
12.“(array) Not Initialized”
当数组已经声明但未初始化时,你会得到“(array) Not Initialized”这样的错误消息。数组的长度是固定的,因此每个数组都需要以所需的长度进行初始化。
以下代码是正确的:
AClass[] array = {object1, object2}
这样也可以:
AClass[] array = new AClass[2];
...
array[0] = object1;
array[1] = object2;
但这样是不正确的:
AClass[] array;
...
array = {object1, object2};