引言
是否在寻找一个强大的Opc Ua .NET客户端库,以方便你在.NET环境下开发OPC UA应用程序?今天,将为你揭示一款备受好评的Opc Ua .NET客户端库!
什么是Opc Ua客户端
Opc Ua(Open Platform for Control and Unification)是一种广泛应用于工业自动化领域的通信协议标准。它旨在提供统一的接口和数据模型,以实现不同设备之间的无缝连接和高效的数据传输。而这款MIT License的Opc Ua客户端,正是为了帮助用户更好地理解和利用这一协议标准而开发的。
此库作用
使用OPC统一体系结构和Visual Studio进行通信。有了这个库,应用程序可以浏览、读取、写入和订阅网络上OPC UA服务器发布的实时数据。
支架.NETCore、通用Windows平台(UWP)、Windows演示框架(WPF)和Xamarin应用程序。
功能特性
易于集成:该库提供简单易用的API,方便开发者快速集成到现有项目中。
高效稳定:经过严格的测试和实际应用验证,确保在各种复杂环境中稳定高效运行。
支持OPC UA协议:支持所有OPC UA基础协议和扩展,满足各种应用需求。
灵活的配置与定制:提供丰富的配置选项和扩展点,方便根据项目需求进行定制。
强大的性能优化:针对.NET环境进行了性能优化,显著提升数据传输和处理速度。
MIT License:作为一款开源软件,这款客户端采用了MIT License许可模式,这意味着用户可以免费获取、修改和使用它。这极大地降低了使用成本,让更多人能够受益于它的功能和服务。
如何使用
通过nuget安装 Workstation.UaClient
以下是从公共OPC UA服务器读取变量ServerStatus的示例。
using System;
using System.Threading.Tasks;
using Workstation.ServiceModel.Ua;
using Workstation.ServiceModel.Ua.Channels;
public class Program
{
/// <summary>
/// Connects to server and reads the current ServerState.
/// </summary>
public static async Task Main()
{
// describe this client application.
var clientDescription = new ApplicationDescription
{
ApplicationName = "Workstation.UaClient.FeatureTests",
ApplicationUri = $"urn:{System.Net.Dns.GetHostName()}:Workstation.UaClient.FeatureTests",
ApplicationType = ApplicationType.Client
};
// create a 'ClientSessionChannel', a client-side channel that opens a 'session' with the server.
var channel = new ClientSessionChannel(
clientDescription,
null, // no x509 certificates
new AnonymousIdentity(), // no user identity
"opc.tcp://opcua.umati.app:4840", // the public endpoint of the umati sample server.
SecurityPolicyUris.None); // no encryption
try
{
// try opening a session and reading a few nodes.
await channel.OpenAsync();
Console.WriteLine($"Opened session with endpoint '{channel.RemoteEndpoint.EndpointUrl}'.");
Console.WriteLine($"SecurityPolicy: '{channel.RemoteEndpoint.SecurityPolicyUri}'.");
Console.WriteLine($"SecurityMode: '{channel.RemoteEndpoint.SecurityMode}'.");
Console.WriteLine($"UserIdentityToken: '{channel.UserIdentity}'.");
// build a ReadRequest. See 'OPC UA Spec Part 4' paragraph 5.10.2
var readRequest = new ReadRequest {
// set the NodesToRead to an array of ReadValueIds.
NodesToRead = new[] {
// construct a ReadValueId from a NodeId and AttributeId.
new ReadValueId {
// you can parse the nodeId from a string.
// e.g. NodeId.Parse("ns=2;s=Demo.Static.Scalar.Double")
NodeId = NodeId.Parse(VariableIds.Server_ServerStatus),
// variable class nodes have a Value attribute.
AttributeId = AttributeIds.Value
}
}
};
// send the ReadRequest to the server.
var readResult = await channel.ReadAsync(readRequest);
// DataValue is a class containing value, timestamps and status code.
// the 'Results' array returns DataValues, one for every ReadValueId.
var serverStatus = readResult.Results[0].GetValueOrDefault<ServerStatusDataType>();
Console.WriteLine("\nServer status:");
Console.WriteLine(" ProductName: {0}", serverStatus.BuildInfo.ProductName);
Console.WriteLine(" SoftwareVersion: {0}", serverStatus.BuildInfo.SoftwareVersion);
Console.WriteLine(" ManufacturerName: {0}", serverStatus.BuildInfo.ManufacturerName);
Console.WriteLine(" State: {0}", serverStatus.State);
Console.WriteLine(" CurrentTime: {0}", serverStatus.CurrentTime);
Console.WriteLine($"\nClosing session '{channel.SessionId}'.");
await channel.CloseAsync();
}
catch(Exception ex)
{
await channel.AbortAsync();
Console.WriteLine(ex.Message);
}
}
}
// Server status:
// ProductName: open62541 OPC UA Server
// SoftwareVersion: 1.4.0-rc1
// ManufacturerName: open62541
// State: Running
Model, View, ViewModel (MVVM)
对于HMI应用程序,您可以使用XAML绑定将UI元素连接到实时数据。
首先添加一个UaApplication实例,并在启动过程中对其进行初始化:
public partial class App : Application
{
private UaApplication application;
protected override void OnStartup(StartupEventArgs e)
{
// Build and run an OPC UA application instance.
this.application = new UaApplicationBuilder()
.SetApplicationUri($"urn:{Dns.GetHostName()}:Workstation.StatusHmi")
.SetDirectoryStore($"{Environment.GetFolderPath(Environment.SpecialFolder.LocalApplicationData)}\\Workstation.StatusHmi\\pki")
.SetIdentity(this.ShowSignInDialog)
.Build();
this.application.Run();
// Create and show the main view.
var view = new MainView();
view.Show();
}
...
}
然后,任何视图模型都可以转换为OPC UA订阅。
[Subscription(endpointUrl: "opc.tcp://localhost:48010", publishingInterval: 500, keepAliveCount: 20)]
public class MainViewModel : SubscriptionBase
{
/// <summary>
/// Gets the value of ServerServerStatus.
/// </summary>
[MonitoredItem(nodeId: "i=2256")]
public ServerStatusDataType ServerServerStatus
{
get { return this.serverServerStatus; }
private set { this.SetProperty(ref this.serverServerStatus, value); }
}
private ServerStatusDataType serverServerStatus;
}
在运行时配置EndpointUrl(MVVM)
可以使用配置文件在运行时替换EndpointUrl的所有实例。使用这种方法可以为开发和生产指定不同的端点。
using Microsoft.Extensions.Configuration;
var config = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appSettings.json", true)
.Build();
var app = new UaApplicationBuilder()
...
.AddMappedEndpoints(config)
.Build();
[Subscription(endpointUrl: "MainPLC", publishingInterval: 500, keepAliveCount: 20)]
public class MainViewModel : SubscriptionBase
{
/// <summary>
/// Gets the value of ServerServerStatus.
/// </summary>
[MonitoredItem(nodeId: "i=2256")]
public ServerStatusDataType ServerServerStatus
{
get { return this.serverServerStatus; }
private set { this.SetProperty(ref this.serverServerStatus, value); }
}
private ServerStatusDataType serverServerStatus;
}
appSettings.json
{
"MappedEndpoints": [
{
"RequestedUrl": "MainPLC",
"Endpoint": {
"EndpointUrl": "opc.tcp://192.168.1.100:48010",
"SecurityPolicyUri": "http://opcfoundation.org/UA/SecurityPolicy#None"
}
}
]
}
源码地址
https://github.com/convertersystems/opc-ua-client
推荐阅读
欢迎扫描下方二维码加我的微信,获取更多技术解决方案并入群交流
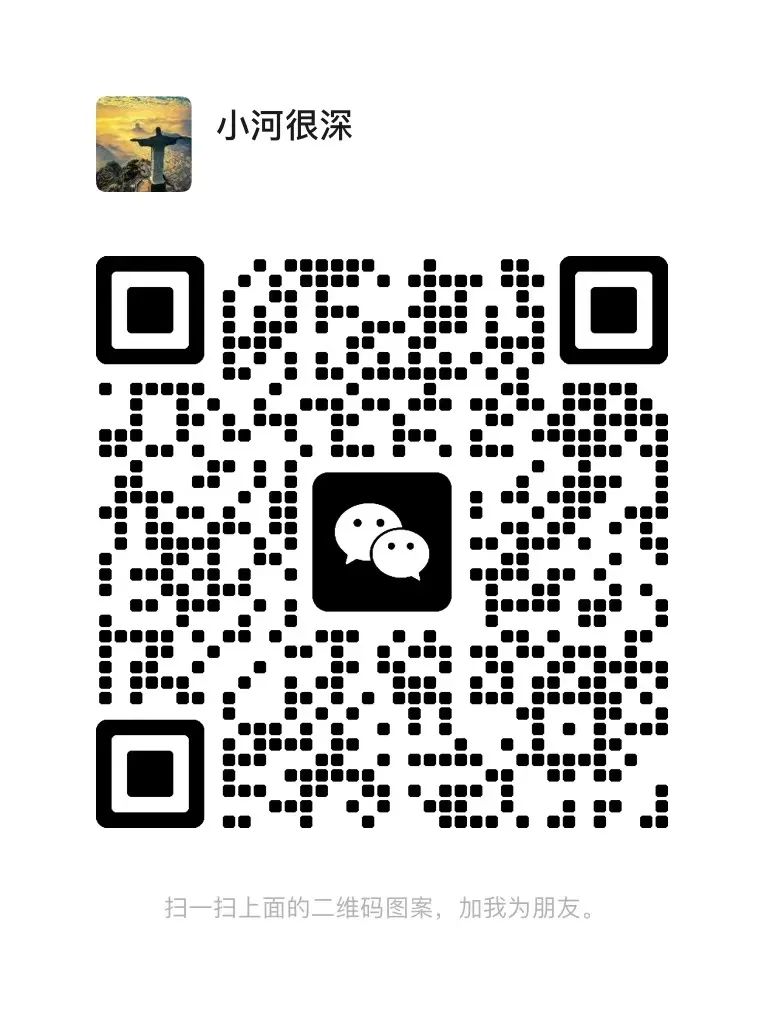
版权声明:本文来源于网络素材收集整理或网友供稿,版权归版权所有者所有,如有侵权请联系小编予以删除