原文参考:http://blog.bossma.cn/csharp/code-first-dwith-ado-net-entity-framework-4-1/
.NET的实体框架越来越完善了,前几天看到Entity Framework 4.1已经正式发布了,新添加了一种称为Code First的开发模式。字面上的意思就是代码优先;按照微软对于它的说明就是:Code First聚焦于定义你的model类,这些类可以映射到一个现有的数据库,或者根据这些类生成数据库,并且提供了数据注解功能和一个易用的API。
下面将对如何使用这种开发模式做一个简单的说明:
准备:您需要已经安装VS2010以及Entity Framework 4.1
1、新建项目或网站
无论是网站,还是项目都可以使用Code First的开发模式。
2、添加类库引用
- EntityFramework
- System.Data.Entity
- System.ComponentModel.DataAnnotations
3、编写Model类
如果是网站需要把代码写到App_Code文件夹下才能够被编译执行。
[Column(TypeName = "varchar" )] |
public string CategoryId { get ; set ; } |
[Column( "CategoryName" , TypeName = "nvarchar" )] |
public string Name { get ; set ; } |
public virtual ICollection<Product> Products { get ; set ; } |
public int ProductId { get ; set ; } |
[Column( "ProductName" , TypeName = "nvarchar" )] |
public string Name { get ; set ; } |
public string CategoryId { get ; set ; } |
public virtual Category Category { get ; set ; } |
public string SupplierCode { get ; set ; } |
[Column( "SupplierName" , TypeName = "nvarchar" )] |
public string Name { get ; set ; } |
public DateTime AddTime { get ; set ; } |
这些Model类和我们平常用的没什么两样,不需要任何基类,只不过增加了一些属性注解来做持久化映射。
这些属性定义在System.ComponentModel.DataAnnotations中,比如:Column用于映射数据表中的字段(字段名、字段类型等)。
如果我们不添加这些映射,则框架会按照惯例进行相关的映射处理,开发者不需要做什么显示配置。
关于这些属性可以参考:http://msdn.microsoft.com/en-us/library/dd901590(v=vs.95).aspx
EF4.1中支持的包括如下属性:
- KeyAttribute 主键
- StringLengthAttribute 字符串长度
- MaxLengthAttribute 最大长度
- ConcurrencyCheckAttribute
- RequiredAttribute 必需
- TimestampAttribute 时间戳
- ComplexTypeAttribute 复合类型
- ColumnAttribute 映射列:column name, ordinal & data type
- TableAttribute 映射表:name 和schema
- InversePropertyAttribute
- ForeignKeyAttribute 外键
- DatabaseGeneratedAttribute
- NotMappedAttribute 在数据库中排除
4、创建DbContext
继承DbContext,为上边创建的Model添加数据集。
public class ProductContext : DbContext |
public DbSet<Category> Categories { get ; set ; } |
public DbSet<Product> Products { get ; set ; } |
public DbSet<Supplier> Suppliers { get ; set ; } |
这可以理解成一个数据操作类,而且DbContext提供了一些方法很不错,如Find可以根据主键查找数据。
5、添加数据库连接字符串
providerName = "System.Data.SqlClient" |
connectionString = "Server=192.168.100.100;Database=ProductContext;User ID=sa;Password=sa;Trusted_Connection=False;Persist Security Info=True " /> |
注意这个数据连接的名称要和继承自DbContext的类的名称一致,数据库ProductContext在SQLServer中尚未创建。
6、编写数据处理
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %> |
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> |
< html xmlns = "http://www.w3.org/1999/xhtml" > |
< form id = "form1" runat = "server" > |
Category Name:< asp:TextBox ID = "TextBox1" runat = "server" ></ asp:TextBox > |
< asp:Button ID = "Button1" runat = "server" OnClick = "Button1_Click" Text = "添 加" /> |
< asp:Button ID = "Button2" runat = "server" Text = "查 询" OnClick = "Button2_Click" /> |
< asp:Repeater ID = "Repeater1" runat = "server" > |
<%# Eval("CategoryId") %> <%# Eval("Name") %>< br /> |
using System.Web.UI.WebControls; |
using System.Data.Entity; |
using System.Collections; |
public partial class _Default : System.Web.UI.Page |
protected void Page_Load( object sender, EventArgs e) |
protected void Button1_Click( object sender, EventArgs e) |
using (ProductContext db = new ProductContext()) |
Category c = new Category() |
CategoryId = Guid.NewGuid().ToString(), |
protected void Button2_Click( object sender, EventArgs e) |
using (ProductContext db = new ProductContext()) |
IQueryable<Category> rs = from c in db.Categories |
Repeater1.DataSource = rs.ToList(); |
有两个数据操作,添加数据和查询数据。
在执行数据操作的时候, 如果数据库不存在则程序首先创建数据库,再进行数据操作。默认的情况下,如果数据库已经存在,则不执行更新数据库结构的操作。
但是我们有时候更改了Model类,希望能够同步到数据库中。
完美的实现方式就是我们改了某个字段或者某个表,数据库中只更新相应的部分就行了。但是这一想法没有在这个版本中实现。
微软提供的是如果发现Model类有变化,则重新创建数据库。微软的方法无疑会将我们的测试数据清理掉,还好我们可以在初始化的过程中添加测试数据,这样每次重新创建数据库的时候,测试数据就会自动加进去了,算是解决了一些问题。
public class ProductContextInitializer : DropCreateDatabaseIfModelChanges<ProductContext> |
protected override void Seed(ProductContext context) |
context.Categories.Add( new Category() { CategoryId = Guid.NewGuid().ToString(), Name = "Category1" }); |
context.Categories.Add( new Category() { CategoryId = Guid.NewGuid().ToString(), Name = "Category2" }); |
因为Code First是一种开发模式,也就是开发的过程,所以测试数据对于开发者来说并不是太重要,微软的解决方案可以接受。只不过实际运作起来就不能这样改了,只能手动改数据库了。
7、上边提到的微软的解决方案还需要通过一个方法开启:
创建文件Global.asax,在Application_Start中添加一个初始化操作:
void Application_Start( object sender, EventArgs e) |
System.Data.Entity.Database.SetInitializer<ProductContext>( new ProductContextInitializer()); |
8、现在运行程序,试试吧:
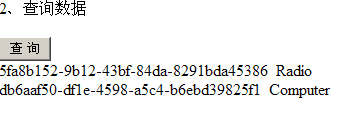
OK!使用流程大体就是这样的。
个人认为Code First对于理顺开发流程还是很有帮助的,我们先根据需求编写Model及相应的操作,大部分时间只关于业务层面就行了,至于数据库生成就可以了。当然使用这种开发模式还需要根据项目类型和个人喜好。
更多细节本文没有介绍,有什么问题欢迎与我讨论。
提供几篇文章参考:
http://msdn.microsoft.com/zh-cn/ff954205.aspx
http://blogs.msdn.com/b/adonet/archive/2011/03/15/ef-4-1-code-first-walkthrough.aspx