大家可能都会遇到这样一个设计:某个界面设计有个ShowMore按钮,点击是文本的展开与收缩。Android默认的效果大家可能都会通过setMaxLines来实现,但直接这么做是没有动画的,就是一个文本的突变。很早之前也想过要实现一个动画,但对TextView的Layout不熟悉就一直没做过。最近在一次SpringDemoReview的时候,iOS实现了这个效果,这不行啊,我们Android不能落后啊。所以最后作出了下面的效果。
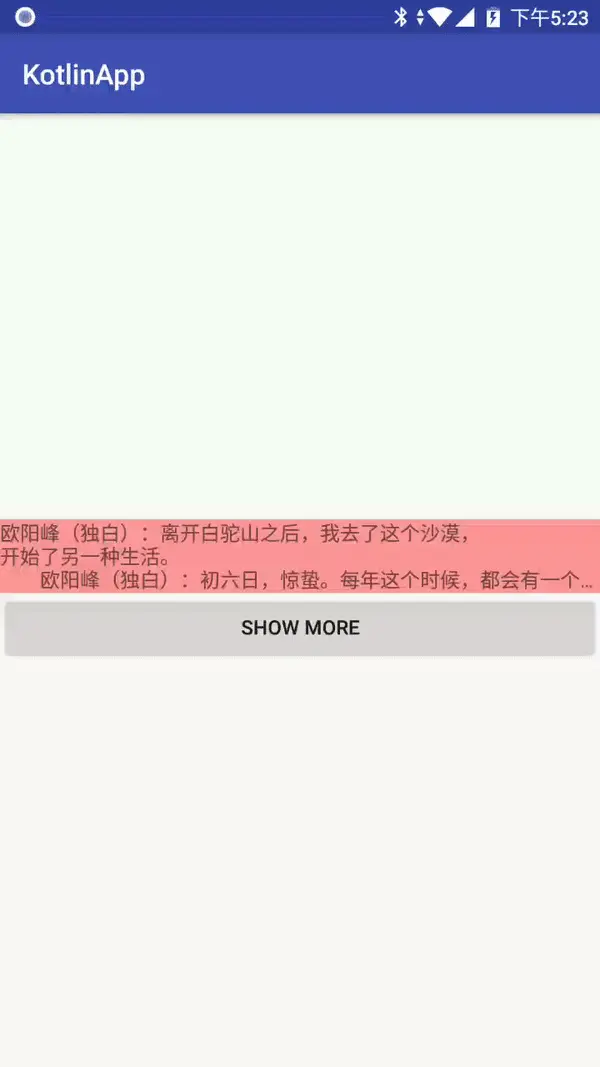
基本是完整兼容TextView本身。收缩小图有...,而且这个点点还是TextView本身的实现,没做过任何修改。
不关心原理可以直接使用TextViewUtils
https://github.com/aesean/ActivityStack/blob/master/app/src/main/java/com/aesean/activitystack/utils/TextViewUtils.java
使用方法很简单:
int lines = mTextView.getMaxLines() == 3 ? Integer.MAX_VALUE : 3;
mTextView.setMaxLineWithAnimation(lines);
但其实比起代码本身来说,实现代码的过程更加重要。
AutoSizeTextView
第一次看到这个效果的话,我们先思考下,如何实现这个效果?但就动画来说就是TextView的高度从3行的高度变到了最大行的高度。如果我们能拿到这两个高度的话,结合ValueAnimator应该大致可以做到这个效果。那问题来了怎么拿到3行或者多行的文字高度?拿View本身的高度吗?那就麻烦了,那样必须先绘制View然后才能拿到,但我们显然是想要在设置前就知道这个高度的。麻烦了,简单看下TextView实现会发现,TextView会通过Layout来测量文本位置,代码非常复杂。我不是很熟悉TextView的绘制和Layout。所以虽然简单浏览了还是对测量TextView高度毫无头绪。
怎么办?不知道有没有人知道,Android O时候Google让TextView实现了类似iOS上的自动改变文字大小的功能。
https://developer.android.com/guide/topics/ui/look-and-feel/autosizing-textview.html
看到这个有没有什么灵感?虽然没有看过具体实现,但猜测这里的AutoSize,应该是需要对文本进行测量的,不断用不同字号,对文本进行测量,直到测量到合适的字号,然后设置。
猜测归猜测,我们实际去看下具体实现。不用想具体实现应该在AppCompatTextView类中。
然后很容易找到三个setAutoSizeText开头的方法和一个AppCompatTextHelper
private final AppCompatTextHelper mTextHelper;
public void setAutoSizeTextTypeWithDefaults
public void setAutoSizeTextTypeUniformWithConfiguration
public void setAutoSizeTextTypeUniformWithPresetSizes
这三个方法都是调用类AppCompatTextHelper中对应的方法。跟到AppCompatTextHelper很容易就找到了AppCompatTextViewAutoSizeHelper
class AppCompatTextHelper {
private final @NonNull AppCompatTextViewAutoSizeHelper mAutoSizeTextHelper;
大致浏览下这个类会发现这样一个方法
/**
* Automatically computes and sets the text size.
*
* @hide
*/
@RestrictTo(LIBRARY_GROUP)
void autoSizeText() {
if (!isAutoSizeEnabled()) {
return;
}
if (mNeedsAutoSizeText) {
if (mTextView.getMeasuredHeight() <= 0 || mTextView.getMeasuredWidth() <= 0) {
return;
}
final boolean horizontallyScrolling = invokeAndReturnWithDefault(
mTextView, "getHorizontallyScrolling", false);
final int availableWidth = horizontallyScrolling
? VERY_WIDE
: mTextView.getMeasuredWidth() - mTextView.getTotalPaddingLeft()
- mTextView.getTotalPaddingRight();
final int availableHeight = mTextView.getHeight() - mTextView.getCompoundPaddingBottom()
- mTextView.getCompoundPaddingTop();
if (availableWidth <= 0 || availableHeight <= 0) {
return;
}
synchronized (TEMP_RECTF) {
TEMP_RECTF.setEmpty();
TEMP_RECTF.right = availableWidth;
TEMP_RECTF.bottom = availableHeight;
final float optimalTextSize = findLargestTextSizeWhichFits(TEMP_RECTF);
if (optimalTextSize != mTextView.getTextSize()) {
setTextSizeInternal(TypedValue.COMPLEX_UNIT_PX, optimalTextSize);
}
}
}
// Always try to auto-size if enabled. Functions that do not want to trigger auto-sizing
// after the next layout pass should set this to false.
mNeedsAutoSizeText = true;
}
自动计算和设置文本大小。看到findLargestTextSizeWhichFits这个方法了吗?
/**
* Performs a binary search to find the largest text size that will still fit within the size
* available to this view.
*/
private int findLargestTextSizeWhichFits(RectF availableSpace) {
final int sizesCount = mAutoSizeTextSizesInPx.length;
if (sizesCount == 0) {
throw new IllegalStateException("No available text sizes to choose from.");
}
int bestSizeIndex = 0;
int lowIndex = bestSizeIndex + 1;
int highIndex = sizesCount - 1;
int sizeToTryIndex;
while (lowIndex <= highIndex) {
sizeToTryIndex = (lowIndex + highIndex) / 2;
if (suggestedSizeFitsInSpace(mAutoSizeTextSizesInPx[sizeToTryIndex], availableSpace)) {
bestSizeIndex = lowIndex;
lowIndex = sizeToTryIndex + 1;
} else {
highIndex = sizeToTryIndex - 1;
bestSizeIndex = highIndex;
}
}
return mAutoSizeTextSizesInPx[bestSizeIndex];
}
大致意思就是二分搜索找到可用的最大文本大小。其实不看注释,只看方法名字也能明白。方法实现也很简单,while循环,二分搜索查找合适的大小。
private boolean suggestedSizeFitsInSpace(int suggestedSizeInPx, RectF availableSpace) {
final CharSequence text = mTextView.getText();
final int maxLines = Build.VERSION.SDK_INT >= 16 ? mTextView.getMaxLines() : -1;
if (mTempTextPaint == null) {
mTempTextPaint = new TextPaint();
} else {
mTempTextPaint.reset();
}
mTempTextPaint.set(mTextView.getPaint());
mTempTextPaint.setTextSize(suggestedSizeInPx);
// Needs reflection call due to being private.
Layout.Alignment alignment = invokeAndReturnWithDefault(
mTextView, "getLayoutAlignment", Layout.Alignment.ALIGN_NORMAL);
final StaticLayout layout = Build.VERSION.SDK_INT >= 23
? createStaticLayoutForMeasuring(
text, alignment, Math.round(availableSpace.right), maxLines)
: createStaticLayoutForMeasuringPre23(
text, alignment, Math.round(availableSpace.right));
// Lines overflow.
if (maxLines != -1 && (layout.getLineCount() > maxLines
|| (layout.getLineEnd(layout.getLineCount() - 1)) != text.length())) {
return false;
}
// Height overflow.
if (layout.getHeight() > availableSpace.bottom) {
return false;
}
return true;
}
通过调用suggestedSizeFitsInSpace方法来判断大小是否合适,最终找到最合适的大小。看到中间的StaticLayout了吗?
final StaticLayout layout = Build.VERSION.SDK_INT >= 23
? createStaticLayoutForMeasuring(
text, alignment, Math.round(availableSpace.right), maxLines)
: createStaticLayoutForMeasuringPre23(
text, alignment, Math.round(availableSpace.right));
StaticLayout可以用来对文本进行排版测量。这个方法最后很明显就是通过StaticLayout的测量结果和实际可绘制的大小进行比对来判断是否合适的。
// Lines overflow.
if (maxLines != -1 && (layout.getLineCount() > maxLines
|| (layout.getLineEnd(layout.getLineCount() - 1)) != text.length())) {
return false;
}
// Height overflow.
if (layout.getHeight() > availableSpace.bottom) {
return false;
}
return true;
OK到这里AutoSizeTextView基本原理就清楚了。那对于我们上面的效果有什么帮助呢?
帮助大了,我们可以顺着Google的思路,也创建出一个StaticLayout然后我们就可以自由的计算任意文字在指定TextView上的绘制出的大小了。拿到大小后,动画就非常简单了。通过ValueAnimator不断改变View的heigh来实现展开和收拢动画。
@Nullable
public static ValueAnimator setMaxLinesWithAnimation(@NotNull final TextView textView, final int maxLine) {
measureTextHeight(textView, textView.getText().toString());
final int textHeight = measureTextHeight(textView, textView.getText(), maxLine);
if (textHeight < 0) {
// measure failed. setMaxLines directly.
textView.setMaxLines(maxLine);
return null;
}
final int minLines = textView.getMinLines();
final int targetHeight = textHeight + textView.getCompoundPaddingBottom() + textView.getCompoundPaddingTop();
return animatorToHeight(textView, targetHeight, new AnimatorListenerAdapter() {
public void onAnimationStart(Animator animation) {
super.onAnimationStart(animation);
}
public void onAnimationEnd(Animator animation) {
super.onAnimationEnd(animation);
textView.setMinLines(minLines);
textView.setMaxLines(maxLine);
}
});
}
@Nullable
public static ValueAnimator animatorToHeight(@NotNull final TextView textView, int h, @Nullable Animator.AnimatorListener listener) {
final int height = textView.getHeight();
if (height == h) {
return null;
}
ValueAnimator valueAnimator = new ValueAnimator();
valueAnimator.setIntValues(height, h);
long duration = makeDuration(h, height);
valueAnimator.setDuration(duration);
valueAnimator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
int value = (int) animation.getAnimatedValue();
textView.setHeight(value);
}
});
if (listener != null) {
valueAnimator.addListener(listener);
}
valueAnimator.start();
return valueAnimator;
}
但是一定注意在动画结束后手动恢复MinLines和MaxLines
,不恢复会怎样呢?这里我就不解释了,你可以自己实际尝试下,看会导致什么问题。
最终代码就是:https://github.com/aesean/ActivityStack/blob/master/app/src/main/java/com/aesean/activitystack/utils/TextViewUtils.java
200多行,不算多。下面代码和github完全一致方便404用户。创建StaticLayout部分的代码都来自AppCompatTextViewAutoSizeHelper,修修补补就变成了下面的类。Google在构建StaticLayout时候反射了部分TextView方法,虽然都在说不推荐反射,但既然Google都用了反射那肯定是有原因的,而且Google用了MethodCacheMap做了Method缓存降低了反射对性能的影响,反射时候用defaultValue来处理反射失败的情况。你可以尝试将反射部分的代码改成下面的代码来测试反射失败的情况。
private static <T> T invokeAndReturnWithDefault(@NonNull Object object, @NonNull final String methodName, @NonNull final T defaultValue) {
if (1 == 1) {
return defaultValue;
}
}
@SuppressLint("PrivateApi")
@Nullable
private static Method getTextViewMethod(@NonNull final String methodName) {
if (1 == 1) {
return null;
}
}
import android.animation.Animator;
import android.animation.AnimatorListenerAdapter;
import android.animation.ValueAnimator;
import android.annotation.SuppressLint;
import android.graphics.RectF;
import android.os.Build;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.support.annotation.RequiresApi;
import android.text.Layout;
import android.text.StaticLayout;
import android.text.TextDirectionHeuristic;
import android.text.TextDirectionHeuristics;
import android.text.TextPaint;
import android.util.Log;
import android.widget.TextView;
import org.jetbrains.annotations.NotNull;
import java.lang.reflect.Method;
import java.util.Hashtable;
/**
* TextViewUtils.
* {@link android.support.v7.widget.AppCompatTextViewAutoSizeHelper}
*
* @author danny
* @version 1.0
* @since 1/17/18
*/
@RequiresApi(Build.VERSION_CODES.ICE_CREAM_SANDWICH)
public class TextViewUtils {
private static final String TAG = "TextViewUtils";
private static Hashtable<String, Method> sTextViewMethodByNameCache = new Hashtable<>();
private static final long DURATION = 600; // ms
private static final long MIN_DURATION = 100; // ms
private static final long MAX_DURATION = 1000; // ms
private static final long BASE_HEIGHT = 1000; // px
// horizontal scrolling is activated.
private static final int VERY_WIDE = 1024 * 1024;
private static RectF TEMP_RECT_F = new RectF();
private static TextPaint sTempTextPaint;
@Nullable
public static ValueAnimator setMaxLinesWithAnimation(@NotNull final TextView textView, final int maxLine) {
measureTextHeight(textView, textView.getText().toString());
final int textHeight = measureTextHeight(textView, textView.getText(), maxLine);
if (textHeight < 0) {
// measure failed. setMaxLines directly.
textView.setMaxLines(maxLine);
return null;
}
final int minLines = textView.getMinLines();
final int targetHeight = textHeight + textView.getCompoundPaddingBottom() + textView.getCompoundPaddingTop();
return animatorToHeight(textView, targetHeight, new AnimatorListenerAdapter() {
public void onAnimationStart(Animator animation) {
super.onAnimationStart(animation);
}
public void onAnimationEnd(Animator animation) {
super.onAnimationEnd(animation);
textView.setMinLines(minLines);
textView.setMaxLines(maxLine);
}
});
}
@Nullable
public static ValueAnimator animatorToHeight(@NotNull final TextView textView, int h, @Nullable Animator.AnimatorListener listener) {
final int height = textView.getHeight();
if (height == h) {
return null;
}
ValueAnimator valueAnimator = new ValueAnimator();
valueAnimator.setIntValues(height, h);
long duration = makeDuration(h, height);
valueAnimator.setDuration(duration);
valueAnimator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
int value = (int) animation.getAnimatedValue();
textView.setHeight(value);
}
});
if (listener != null) {
valueAnimator.addListener(listener);
}
valueAnimator.start();
return valueAnimator;
}
private static long makeDuration(int h, int height) {
long d = (long) (DURATION * (Math.abs(h - height) * 1f / BASE_HEIGHT));
return Math.max(MIN_DURATION, Math.min(d, MAX_DURATION));
}
public static int measureTextHeight(@NotNull TextView textView, @NotNull CharSequence text) {
return measureTextHeight(textView, text, Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN ? textView.getMaxLines() : Integer.MAX_VALUE);
}
public static int measureTextHeight(@NotNull TextView textView, @NotNull CharSequence text, int maxLines) {
if (sTempTextPaint == null) {
sTempTextPaint = new TextPaint();
}
sTempTextPaint.set(textView.getPaint());
sTempTextPaint.setTextSize(textView.getTextSize());
final int targetHeight = measureTextHeight(textView, text, sTempTextPaint, maxLines);
sTempTextPaint.reset();
return targetHeight;
}
public static int measureTextHeight(@NotNull TextView textView, @NotNull CharSequence text, @NotNull TextPaint textPaint, int maxLines) {
final boolean horizontallyScrolling = invokeAndReturnWithDefault(textView, "getHorizontallyScrolling", false);
final int availableWidth = horizontallyScrolling
? VERY_WIDE
: textView.getMeasuredWidth() - textView.getTotalPaddingLeft()
- textView.getTotalPaddingRight();
final int availableHeight = textView.getHeight() - textView.getCompoundPaddingBottom()
- textView.getCompoundPaddingTop();
if (availableWidth <= 0 || availableHeight <= 0) {
return -1;
}
TEMP_RECT_F.setEmpty();
TEMP_RECT_F.right = availableWidth;
// TEMP_RECT_F.bottom = availableHeight;
TEMP_RECT_F.bottom = VERY_WIDE;
// Needs reflection call due to being private.
Layout.Alignment alignment = invokeAndReturnWithDefault(
textView, "getLayoutAlignment", Layout.Alignment.ALIGN_NORMAL);
final StaticLayout layout = createStaticLayoutForMeasuringCompat(text, alignment, Math.round(TEMP_RECT_F.right), Integer.MAX_VALUE, textPaint, textView);
return layout.getLineTop(Math.min(layout.getLineCount(), maxLines));
}
private static StaticLayout createStaticLayoutForMeasuringCompat(CharSequence text, Layout.Alignment alignment, int availableWidth, int maxLines, TextPaint textPaint, TextView textView) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
return createStaticLayoutForMeasuring(text, alignment, availableWidth, maxLines, textPaint, textView);
} else {
return createStaticLayoutForMeasuringPre23(text, alignment, availableWidth, textPaint, textView);
}
}
@RequiresApi(Build.VERSION_CODES.M)
private static StaticLayout createStaticLayoutForMeasuring(CharSequence text, Layout.Alignment alignment, int availableWidth, int maxLines, TextPaint textPaint, TextView textView) {
// Can use the StaticLayout.Builder (along with TextView params added in or after
// API 23) to construct the layout.
final TextDirectionHeuristic textDirectionHeuristic = invokeAndReturnWithDefault(textView, "getTextDirectionHeuristic", TextDirectionHeuristics.FIRSTSTRONG_LTR);
final StaticLayout.Builder layoutBuilder = StaticLayout.Builder.obtain(text, 0, text.length(), textPaint, availableWidth);
return layoutBuilder.setAlignment(alignment)
.setLineSpacing(textView.getLineSpacingExtra(), textView.getLineSpacingMultiplier())
.setIncludePad(textView.getIncludeFontPadding())
.setBreakStrategy(textView.getBreakStrategy())
.setHyphenationFrequency(textView.getHyphenationFrequency())
.setMaxLines(maxLines == -1 ? Integer.MAX_VALUE : maxLines)
.setTextDirection(textDirectionHeuristic)
.build();
}
@RequiresApi(Build.VERSION_CODES.ICE_CREAM_SANDWICH)
private static StaticLayout createStaticLayoutForMeasuringPre23(CharSequence text, Layout.Alignment alignment, int availableWidth, TextPaint textPaint, TextView textView) {
// Setup defaults.
float lineSpacingMultiplier = 1.0f;
float lineSpacingAdd = 0.0f;
boolean includePad = true;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) {
// Call public methods.
lineSpacingMultiplier = textView.getLineSpacingMultiplier();
lineSpacingAdd = textView.getLineSpacingExtra();
includePad = textView.getIncludeFontPadding();
} else {
// Call private methods and make sure to provide fallback defaults in case something
// goes wrong. The default values have been inlined with the StaticLayout defaults.
lineSpacingMultiplier = invokeAndReturnWithDefault(textView,
"getLineSpacingMultiplier", lineSpacingMultiplier);
lineSpacingAdd = invokeAndReturnWithDefault(textView,
"getLineSpacingExtra", lineSpacingAdd);
//noinspection ConstantConditions
includePad = invokeAndReturnWithDefault(textView,
"getIncludeFontPadding", includePad);
}
// The layout could not be constructed using the builder so fall back to the
// most broad constructor.
return new StaticLayout(text, textPaint, availableWidth,
alignment,
lineSpacingMultiplier,
lineSpacingAdd,
includePad);
}
private static <T> T invokeAndReturnWithDefault(@NonNull Object object, @NonNull final String methodName, @NonNull final T defaultValue) {
T result = null;
boolean exceptionThrown = false;
try {
// Cache lookup.
Method method = getTextViewMethod(methodName);
if (method != null) {
//noinspection unchecked
result = (T) method.invoke(object);
}
} catch (Exception ex) {
exceptionThrown = true;
Log.w(TAG, "Failed to invoke TextView#" + methodName + "() method", ex);
} finally {
if (result == null && exceptionThrown) {
result = defaultValue;
}
}
return result;
}
@SuppressLint("PrivateApi")
@Nullable
private static Method getTextViewMethod(@NonNull final String methodName) {
try {
Method method = sTextViewMethodByNameCache.get(methodName);
if (method == null) {
method = TextView.class.getDeclaredMethod(methodName);
if (method != null) {
method.setAccessible(true);
// Cache update.
sTextViewMethodByNameCache.put(methodName, method);
}
}
return method;
} catch (Exception ex) {
Log.w(TAG, "Failed to retrieve TextView#" + methodName + "() method", ex);
return null;
}
}
}