Time Limit: 1000MS | Memory Limit: 65536K | |||
Total Submissions: 8378 | Accepted: 2456 | Special Judge |
Description
Thousands of thousands years ago there was a small kingdom located in the middle of the Pacific Ocean. The territory of the kingdom consists two separated islands. Due to the impact of the ocean current, the shapes of both the islands became convex polygons. The king of the kingdom wanted to establish a bridge to connect the two islands. To minimize the cost, the king asked you, the bishop, to find the minimal distance between the boundaries of the two islands.
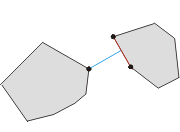
Input
The input consists of several test cases.
Each test case begins with two integers N, M. (3 ≤ N, M ≤ 10000)
Each of the next N lines contains a pair of coordinates, which describes the position of a vertex in one convex polygon.
Each of the next M lines contains a pair of coordinates, which describes the position of a vertex in the other convex polygon.
A line with N = M = 0 indicates the end of input.
The coordinates are within the range [-10000, 10000].
Output
For each test case output the minimal distance. An error within 0.001 is acceptable.
Sample Input
4 4 0.00000 0.00000 0.00000 1.00000 1.00000 1.00000 1.00000 0.00000 2.00000 0.00000 2.00000 1.00000 3.00000 1.00000 3.00000 0.00000 0 0
Sample Output
1.00000
Source
POJ Founder Monthly Contest – 2008.06.29, Lei Tao
//AC代码
#include<iostream>
#include<queue>
#include<cstdio>
#include<algorithm>
#include<cstring>
#include<iomanip>
#include<map>
#include<cstdlib>
#include<cmath>
#include<vector>
#define LL long long
#define IT __int64
#define zero(x) fabs(x)<eps
#define mm(a,b) memset(a,b,sizeof(a))
const int INF=0x7fffffff;
const double inf=1e8;
const double eps=1e-8;
const double PI=acos(-1.0);
const int Max=50001;
using namespace std;
int sign(double x)
{
return (x>eps)-(x<-eps);
}
typedef struct Node
{
double x;
double y;
Node(const double &_x=0, const double &_y=0) : x(_x), y(_y) {}
void input()
{
scanf("%lf%lf",&x,&y);
}
void output()
{
printf("%lf %lf\n",x,y);
}
} point;
point list[Max],stack[Max];
int n,m;
int top;
int cnt;
int curcnt;
double xmult(point p0,point p1,point p2)
{
return(p1.x-p0.x)*(p2.y-p0.y)-(p2.x-p0.x)*(p1.y-p0.y);
}
double dmult(point p0,point p1,point p2)
{
return(p1.x-p0.x)*(p2.x-p0.x)+(p1.y-p0.y)*(p2.y-p0.y);
}
double Distance(point p1,point p2)// 返回两点之间欧氏距离
{
return( sqrt( (p1.x-p2.x)*(p1.x-p2.x)+(p1.y-p2.y)*(p1.y-p2.y) ) );
}
bool cmp(point p1,point p2)
{
double temp;
temp=xmult(list[0],p1,p2);
if(temp>0)
return true;
if(temp==0&&(Distance(list[0],p1)<Distance(list[0],p2)))
return true;
return false;
}
void convex_hull()//凸包模板
{
int i;
for(i=1; i<n; i++)
{
point temp;
if((list[i].y<list[0].y)||(list[i].y==list[0].y&&list[i].x<list[0].x))
swap(list[0],list[i]);
}
sort(list+1,list+n,cmp);
top=1;
stack[0]=list[0];
stack[1]=list[1];
for(i=2; i<n; i++)
{
while(top>=1&&xmult(stack[top-1],stack[top],list[i])<=0)
top--;
top++;
stack[top]=list[i];
}
}
void anticlockwise(point p[],int n)
{
for(int i=0; i<n-2; i++)
{
double tmp=xmult(p[i],p[i+1],p[i+2]);
if(tmp>eps) return;
else if(tmp<-eps)
{
reverse(p,p+n);
return;
}
}
}
//计算C点到直线AB的最短距离
double GetDist(point A,point B,point C)
{
if(Distance(A,B)<eps) return Distance(B,C);
if(dmult(A,B,C)<-eps) return Distance(A,C);
if(dmult(B,A,C)<-eps) return Distance(B,C);
return fabs(xmult(A,B,C)/Distance(A,B));
}
//求一条直线的两端点到另外一条直线的距离
double MinDist(point A,point B,point C,point D)
{
return min(min(GetDist(A,B,C),GetDist(A,B,D)),min(GetDist(C,D,A),GetDist(C,D,B)));
}
double rotating_calipers(point p[],point q[],int n,int m)//旋转卡壳
{
int i,j=1;
double temp,ans=inf;
int yminp=0,ymaxq=0;
for(i=0;i<n;i++)
{
if(p[i].y<p[yminp].y)
yminp=i;
}
for(i=0;i<m;i++)
{
if(q[i].y>q[ymaxq].y)
ymaxq=i;
}
p[n]=p[0];
q[m]=q[0];
for(i=0;i<n;i++)
{
while(temp=xmult(p[yminp+1],q[ymaxq+1],p[yminp])-xmult(p[yminp+1],q[ymaxq],p[yminp])>eps)
ymaxq=(ymaxq+1)%m;
if(temp<-eps)
ans=min(ans,GetDist(p[yminp],p[yminp+1],q[ymaxq]));
else
ans=min(ans,MinDist(p[yminp],p[yminp+1],q[ymaxq],q[ymaxq+1]));
yminp=(yminp+1)%n;
}
return ans;
}
int main()
{
int i,j;
double dis;
while(cin>>n>>m&&(n||m))
{
for(i=0;i<n;i++)
list[i].input();
for(j=0;j<m;j++)
stack[j].input();
anticlockwise(list,n);
//for(i=0;i<n;i++)
//list[i].output();
anticlockwise(stack,m);
//for(j=0;j<m;j++)
//stack[j].output();
//sort(list,list+n,cmp);
//sort(stack,stack+m,cmp);
dis=min(rotating_calipers(list,stack,n,m),rotating_calipers(stack,list,m,n));
cout<<setprecision(5)<<setiosflags(ios::fixed)<<dis<<endl;
}
return 0;
}
/*
4 3
1 3
0 4
1 2
4 1
2 1
2 3
3 2
*/