题目描述:单链表可能有环,也可能无环。给定两个单链表的头节点 head1 和 head2, 这两个链表可能相交,也可能不相交。请实现一个函数,如果两个链表相交,请返回相交 的第一个节点;如果不相交,返回 null 即可。
首先,感谢程云老师的分享!以下是本问题的解决方法整理。
思路:
链表分有环链表和无环链表,如果两个链表存在相交,则只有两种可能,两个链表都无环或者都有环。
(1)如果链表都无环,则先判断链表的尾指针是否一样,如果不一样,则没有相交。如果一样,则找出两个链表的长度差,将两个链表从距离尾节点同样的距离进行扫描,如果相交,则必然有一处扫描节点相同。实例数据List1:1->2->3->4->5->6->7->null,List2:0->9->8->6->7->null,第一个相交节点为6
示例图如下,
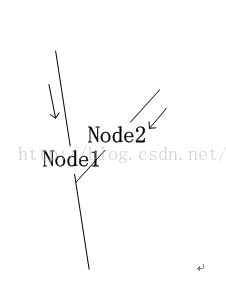
(2)如果链表都有环,则只肯能有下面两种情况(如下图)。两种情况区分的方法为:入环点是否相同。
如果相同则为第一种情况:那么查找第一个相交点与无环的单链表相交找第一个交点的方法一样。
如果入环点不同,则为第二种情况,这个相交点或者为list1 的入环点loop1或者为list2的入环点loop2。
情况1实例数据(List1:1->2->3->4->5->6->7->4,List2:0->9->8->2->3->4->5->6->7->4,第一个交点为2)
情况2实例数据(List1:1->2->3->4->5->6->7->4,List2:0->9->8->6->7->4->5->6,第一个交点为4或6)
下面为实现代码
- public static class Node {
- public int value;
- public Node next;
-
- public Node(int data) {
- this.value = data;
- }
- }
-
- public static Node getIntersectNode(Node head1, Node head2) {
- if (head1 == null || head2 == null) {
- return null;
- }
- Node loop1 = getLoopNode(head1);
- Node loop2 = getLoopNode(head2);
- if (loop1 == null && loop2 == null) {
- return noLoop(head1, head2);
- }
- if (loop1 != null && loop2 != null) {
- return bothLoop(head1, loop1, head2, loop2);
- }
- return null;
- }
-
-
-
-
- public static Node getLoopNode(Node head) {
- if (head == null || head.next == null || head.next.next == null) {
- return null;
- }
- Node n1 = head.next;
- Node n2 = head.next.next;
- while (n1 != n2) {
- if (n2.next == null || n2.next.next == null) {
- return null;
- }
- n2 = n2.next.next;
- n1 = n1.next;
- }
- n2 = head;
- while (n1 != n2) {
- n1 = n1.next;
- n2 = n2.next;
- }
- return n1;
- }
-
- public static Node noLoop(Node head1, Node head2) {
- if (head1 == null || head2 == null) {
- return null;
- }
- Node cur1 = head1;
- Node cur2 = head2;
- int n = 0;
- while (cur1.next != null) {
- n++;
- cur1 = cur1.next;
- }
- while (cur2.next != null) {
- n--;
- cur2 = cur2.next;
- }
- if (cur1 != cur2) {
- return null;
- }
- cur1 = n > 0 ? head1 : head2;
- cur2 = cur1 == head1 ? head2 : head1;
- n = Math.abs(n);
- while (n != 0) {
- n--;
- cur1 = cur1.next;
- }
- while (cur1 != cur2) {
- cur1 = cur1.next;
- cur2 = cur2.next;
- }
- return cur1;
- }
-
- public static Node bothLoop(Node head1, Node loop1, Node head2, Node loop2) {
- Node cur1 = null;
- Node cur2 = null;
- if (loop1 == loop2) {
- cur1 = head1;
- cur2 = head2;
- int n = 0;
- while (cur1 != loop1) {
- n++;
- cur1 = cur1.next;
- }
- while (cur2 != loop2) {
- n--;
- cur2 = cur2.next;
- }
- cur1 = n > 0 ? head1 : head2;
- cur2 = cur1 == head1 ? head2 : head1;
- n = Math.abs(n);
- while (n != 0) {
- n--;
- cur1 = cur1.next;
- }
- while (cur1 != cur2) {
- cur1 = cur1.next;
- cur2 = cur2.next;
- }
- return cur1;
- } else {
- cur1 = loop1.next;
- while (cur1 != loop1) {
- if (cur1 == loop2) {
- return loop1;
- }
- cur1 = cur1.next;
- }
- return null;
- }
- }
另:判断是否单链表是否有环可以使用哈希表进行判断。
最后,关于如何找环的入口点问题,可以参考文章:http://blog.csdn.net/wongson/article/details/8019228,